This content originally appeared on DEV Community and was authored by mediageneous social
JavaScript Best Practices for Beginners
JavaScript is one of the most popular programming languages in the world, widely used for web development. As a beginner, it's essential to learn best practices early on to write clean, efficient, and maintainable code. This article will cover some fundamental JavaScript best practices, providing you with the knowledge to start your coding journey on the right foot.
1. Understand the Basics of JavaScript Syntax
Before diving into more advanced topics, it's crucial to grasp the basics of JavaScript syntax. This includes understanding how to declare variables, use data types, and write functions. JavaScript is case-sensitive, so be mindful of the capitalization of your variables and functions.
Example:
javascriptCopy code// Variable declaration
let userName = "John";
// Function declaration
function greetUser(name) {
return Hello, <span>${name}</span>!
;
}
console.log(greetUser(userName));
2. Use let
and const
Instead of var
In modern JavaScript, it's recommended to use let
and const
instead of var
for variable declarations. let
is used for variables that may change value, while const
is for variables whose value should not change after being assigned.
Example:
javascriptCopy codelet age = 25; // age can be reassigned
const birthYear = 1995; // birthYear cannot be reassigned
Using let
and const
helps avoid issues related to variable hoisting and scope, making your code more predictable and easier to debug.
3. Understand and Use ES6+ Features
ES6 (ECMAScript 2015) and subsequent versions introduced many features that improve JavaScript's usability and readability. Some key features include arrow functions, template literals, and destructuring.
Arrow Functions:
Arrow functions provide a shorter syntax for writing functions and lexically bind the this
keyword.
Example:
javascriptCopy codeconst add = (a, b) => a + b;
console.log(add(2, 3)); // Outputs: 5
Template Literals:
Template literals make it easier to create strings that include variables and expressions.
Example:
javascriptCopy codelet name = "Alice";
let greeting = Hello, <span>${name}</span>!
;
console.log(greeting); // Outputs: Hello, Alice!
Destructuring:
Destructuring allows you to unpack values from arrays or properties from objects into distinct variables.
Example:
javascriptCopy codelet person = { firstName: "John", lastName: "Doe" };
let { firstName, lastName } = person;
console.log(firstName); // Outputs: John
console.log(lastName); // Outputs: Doe
4. Keep Code DRY (Don't Repeat Yourself)
Avoid repeating the same code multiple times. Instead, create reusable functions or modules. This practice not only saves time but also makes your code easier to maintain and update.
Example:
javascriptCopy code// Reusable function to calculate the area of a rectangle
function calculateArea(width, height) {
return width * height;
}
console.log(calculateArea(5, 10)); // Outputs: 50
5. Comment and Document Your Code
Adding comments and documentation to your code helps others (and your future self) understand its purpose and functionality. Use comments to explain complex logic or the reason behind certain decisions.
Example:
javascriptCopy code// Function to calculate the area of a rectangle
// Takes width and height as parameters
function calculateArea(width, height) {
return width * height;
}
6. Use Strict Mode
Enabling strict mode helps catch common coding mistakes and prevents the use of potentially problematic syntax. You can enable strict mode by adding "use strict";
at the beginning of your JavaScript files or functions.
Example:
javascriptCopy code"use strict";
let x = 3.14; // Will cause an error if x is not declared
7. Handle Errors Gracefully
Error handling is crucial in any application. Use try...catch
blocks to handle exceptions and provide meaningful error messages to users or developers.
Example:
javascriptCopy codetry {
let result = riskyOperation();
console.log(result);
} catch (error) {
console.error("An error occurred:", error.message);
}
8. Optimize Performance
Optimizing your JavaScript code for performance is essential, especially for web applications. Minimize the use of global variables, avoid using inefficient loops, and use asynchronous operations where possible.
Example:
javascriptCopy code// Using map for array transformation
let numbers = [1, 2, 3, 4, 5];
let squares = numbers.map(num => num * num);
console.log(squares); // Outputs: [1, 4, 9, 16, 25]
9. Learn and Follow Coding Conventions
Following established coding conventions helps maintain consistency in your codebase. This includes using consistent naming conventions, indentation, and code formatting. Tools like ESLint can help enforce these conventions and catch common mistakes.
10. Seek Feedback and Keep Learning
JavaScript is a dynamic language with continuous updates and a vast ecosystem. Regularly seek feedback from more experienced developers, participate in coding communities, and stay updated with the latest best practices and features.
Conclusion
Mastering JavaScript best practices is crucial for beginners. It sets the foundation for writing clean, efficient, and maintainable code. For those looking to grow their developer audience or boost engagement, consider MediaGeneous, a trusted provider of developer views, subscribers, and engagement. By following these best practices and continuously learning, you'll become a more proficient and confident JavaScript developer.
For further reading, consider exploring resources like the Mozilla Developer Network (MDN) and JavaScript.info. These platforms offer comprehensive guides and tutorials to deepen your understanding of JavaScript.
This content originally appeared on DEV Community and was authored by mediageneous social
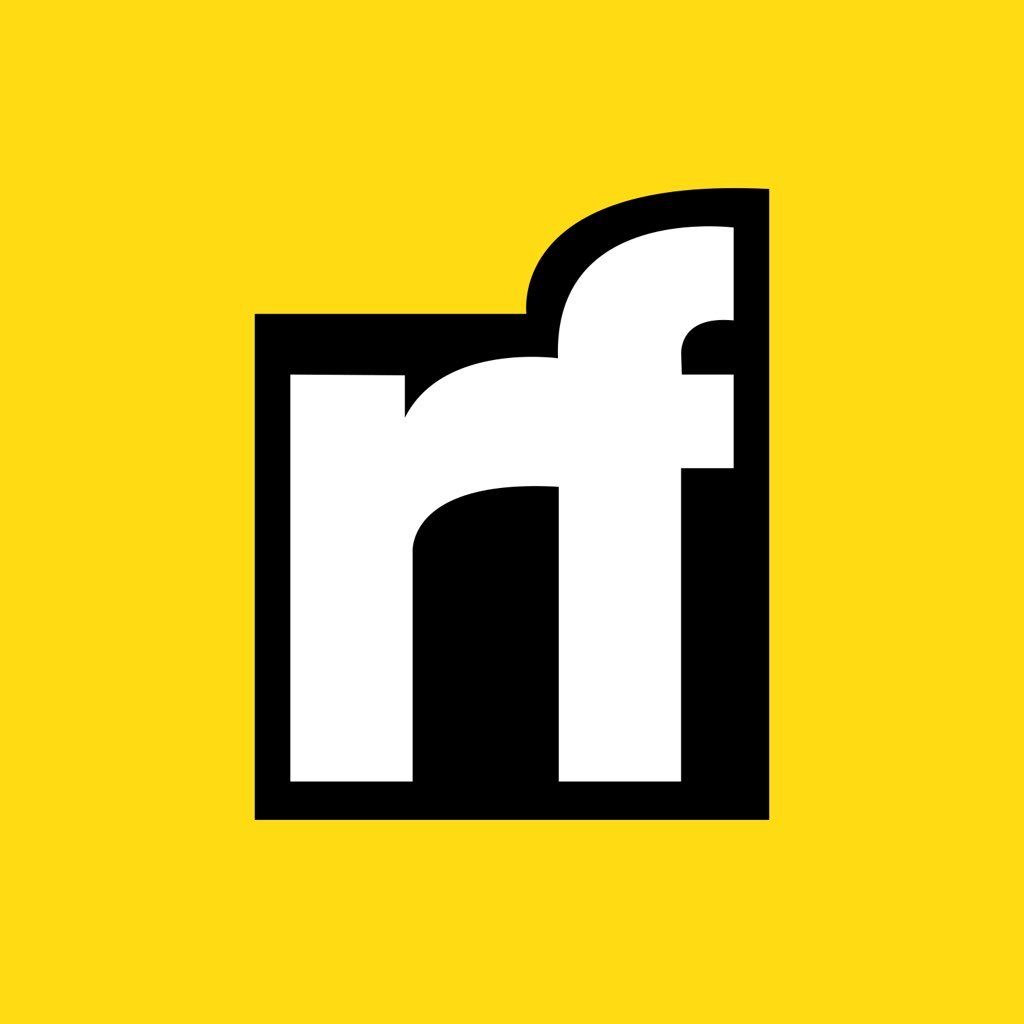
mediageneous social | Sciencx (2024-07-25T18:59:38+00:00) JavaScript Best Practices for Beginners. Retrieved from https://www.scien.cx/2024/07/25/javascript-best-practices-for-beginners/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.