This content originally appeared on DEV Community and was authored by Olatunji Ayodele Abidemi
To build web applications using Python 3, you can use the Flask framework. Flask is lightweight, flexible, and great for creating web apps quickly. Here are the steps to get started:
Install Flask:
First, activate your Python environment and install Flask using pip:
source env/bin/activate # Activate your environment
pip install flask
Create a Simple Flask Application:
Create a Python file (e.g., app.py
) and write a basic Flask app:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
Run the Application:
Run your Flask app:
python app.py
Routes and View Functions:
Define routes (URL paths) and view functions (what to display for each route). For example:
@app.route('/about')
def about():
return 'About page'
@app.route('/contact')
def contact():
return 'Contact us'
Dynamic Routes:
Create dynamic routes that accept parameters (e.g., user IDs):
@app.route('/user/<int:user_id>')
def user_profile(user_id):
return f'User {user_id} profile'
Debugging:
Enable debug mode during development for helpful error messages:
app.run(debug=True)
Remember to adapt this example to your specific project.
This content originally appeared on DEV Community and was authored by Olatunji Ayodele Abidemi
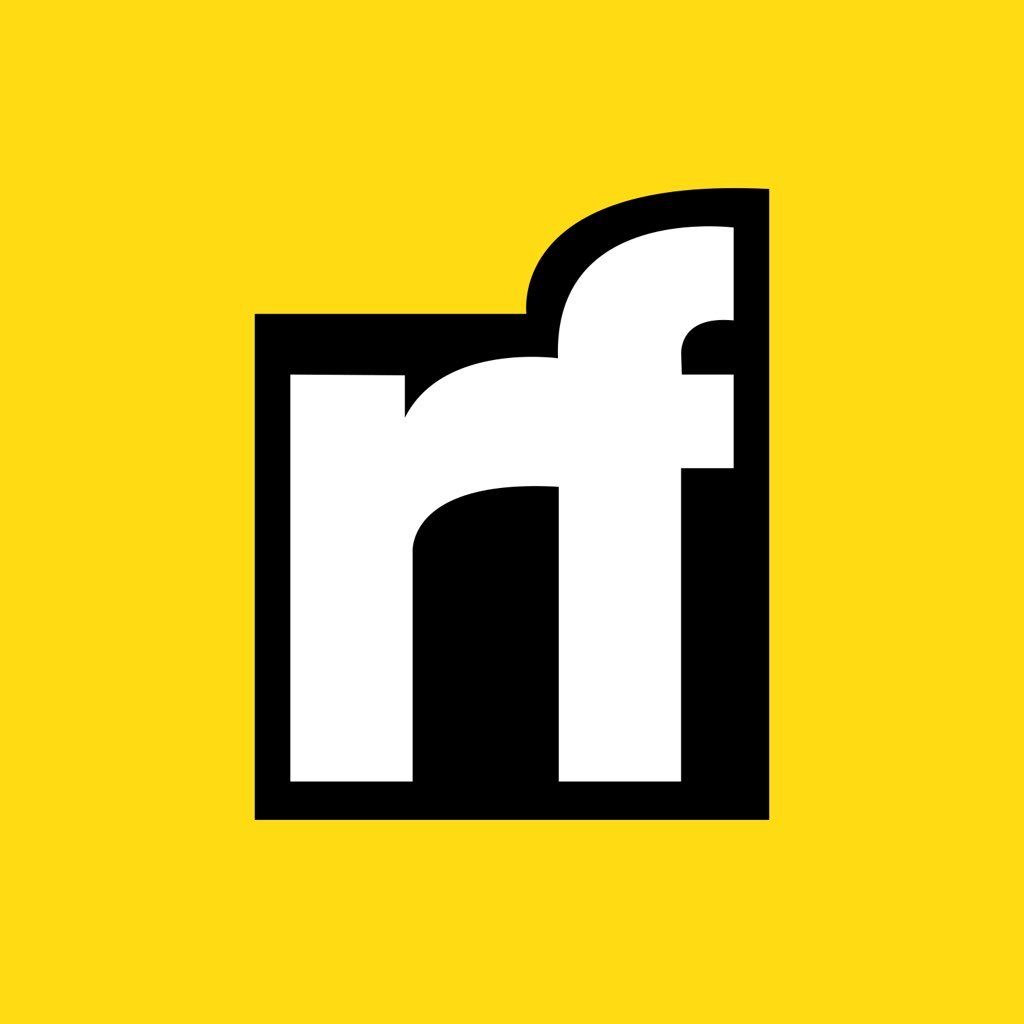
Olatunji Ayodele Abidemi | Sciencx (2024-07-29T10:15:16+00:00) To build web applications using Python3. Retrieved from https://www.scien.cx/2024/07/29/to-build-web-applications-using-python3/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.