This content originally appeared on DEV Community and was authored by Manal Ghanim
I’m working on a Spring Boot application where I need to implement recursive health checks using Spring Boot Actuator. The challenge is that Service A depends on Service B, and Service B depends on Service A, creating a circular dependency. I want to avoid entering an infinite loop during health checks.
Requirements:
Use Spring Boot Actuator’s /health endpoint.
Ensure that the health check does not enter an infinite loop due to circular dependencies.
Cache the health check results to minimize repeated calls.
What I’ve Tried:
Implementing custom health indicators for each service. Using a cache to store health check results temporarily. Example Code: Here’s a simplified version of what I’m trying to achieve:
import org.springframework.boot.actuate.health.HealthIndicator;
import org.springframework.stereotype.Component;
import org.springframework.web.client.RestTemplate;
@Component
public class ServiceAHealthIndicator implements HealthIndicator {
private final RestTemplate restTemplate;
public ServiceAHealthIndicator(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
@Override
public Health health() {
return checkHealth("http://service-b/actuator/health");
}
private Health checkHealth(String url) {
try {
String response = restTemplate.getForObject(url, String.class);
if (response.contains("\"status\":\"UP\"")) {
return Health.up().build();
} else {
return Health.down().build();
}
} catch (Exception e) {
return Health.down().withDetail("Error", e.getMessage()).build();
}
}
}
Questions:
How can I prevent the infinite loop in this circular dependency scenario?
What is the best way to cache the health check results to avoid repeated calls?
Any guidance or examples would be greatly appreciated!
This content originally appeared on DEV Community and was authored by Manal Ghanim
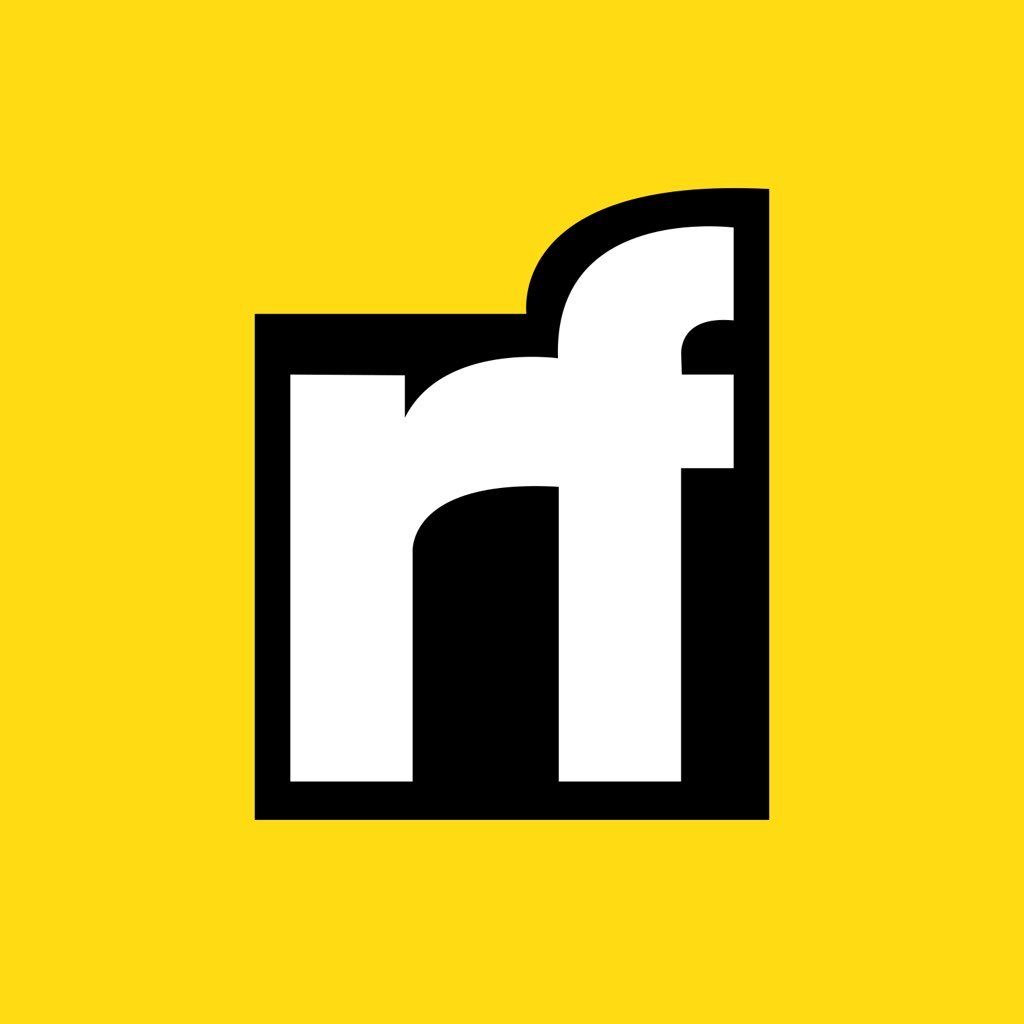
Manal Ghanim | Sciencx (2024-07-30T00:00:00+00:00) How to Implement Recursive Health Check in Spring Boot Actuator Without Infinite Loop in Circular Dependencies?. Retrieved from https://www.scien.cx/2024/07/30/how-to-implement-recursive-health-check-in-spring-boot-actuator-without-infinite-loop-in-circular-dependencies/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.