This content originally appeared on Telerik Blogs and was authored by Jefferson S. Motta
In this article, I want to share how to use Progress Telerik Smart AI components using a custom OpenAI key, replacing Azure OpenAI and Microsoft Word as Context to ChatGPT using a sample from Progress Telerik on GitHub.
DALL-E image
Success Case
A briefing history about this solution test using Telerik Smart AI component: Before I started this article, I asked some lawyer friends to send me documents in DOCX and give me questions that a technical revisor would ask about them.
After some adjustments, passing all document words as text without “---” as the original used to do, the AI started answering the questions as expected, up to the limitations of a professional lawyer.
Document as Context
I am using DOCX as the context for Azure OpenAI. The example provided by Progress Telerik was a .txt file, Context.txt, which was supposed to help the company share the same context to build documentation, contracts, new documents, press releases, documentation or marketing campaigns.
The context given to the AI is fundamental to a better response and contextualizing the business needs of the company, solution or project.
This can be evolved, and we can have multiple documents shared in a single place for contextualization—for example, one for the company, one for the product and one for the team. So, anyone in the company can work based on the same information to guarantee the quality and consistency of the documentation produced by the enterprise and the public.
I also have technical training as a social media analyst. And I know how developers have communication limitations in some cases to express verbally to other company team members, like the marketing team, about how things work and how they can help the end user. So if IT professionals create technical documentation of the products, they will be more accessible to the non-technical co-workers to explore concepts using AI, sharing the same context mentioned before.
I’m sharing the project on GitHub, and anyone can start creating applications based on it that can be adjusted for any professional.
Changing the Template
I changed the template to use OpenAI APIs, changed the Context.txt to an Microsoft Word file, changed the ChatGPT call and passed all documents to the API.
Let’s See It Step by Step
This is the main point I would like to demonstrate: the replacement of Azure OpenAI to work with Telerik AI products.
Changing the original function that uses Azure OpenAI, that is:
1.private static string CallOpenAIApi(string systemPrompt, string message)
2.{
3. // Add your key and endpoint to use the OpenAI API.
4. OpenAIClient client = new OpenAIClient(
5. new Uri("AZURE_ENDPOINT"),
6. new AzureKeyCredential("AZURE_KEY"));
7.
8. ChatCompletionsOptions chatCompletionsOptions = new ChatCompletionsOptions()
9. {
10. DeploymentName = "DeploymentName",
11. Messages =
12. {
13. new ChatRequestSystemMessage(systemPrompt),
14. new ChatRequestUserMessage(message),
15. }
16. };
17.
18. Response<ChatCompletions> response = client.GetChatCompletions(chatCompletionsOptions);
19. ChatResponseMessage responseMessage = response.Value.Choices[0].Message;
20.
21. return responseMessage.Content;
22.}
To this:
1.private static string CallOpenAIApi(string systemPrompt, string message)
2.{
3. // Your OpenAI API key
4. var apiKey = Environment.GetEnvironmentVariable(“API_KEY_OPENAI”) ??
5. throw new Exception("Environment API Key is Missing");
6.
7. // OpenAI API endpoint for chat completions
8. var endpoint = "https://api.openai.com/v1/chat/completions";
9.
10. using var httpClient = new HttpClient();
11.
12. // Set the authorization header with your API key
13. httpClient.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", apiKey);
14.
15. // Prepare the request body
16. var requestBody = new
17. {
18. model = "gpt-3.5-turbo", // Specify the model you want to use
19. messages = new[]
20. {
21. new { role = "system", content = systemPrompt },
22. new { role = "user", content = message }
23. }
24. };
25.
26. // Serialize the request body to JSON
27. var jsonRequestBody = JsonSerializer.Serialize(requestBody);
28. var content = new StringContent(jsonRequestBody, System.Text.Encoding.UTF8, "application/json");
29.
30. // Prepare the request message
31. var requestMessage = new HttpRequestMessage(HttpMethod.Post, endpoint) { Content = content };
32.
33. // Send the POST request to the OpenAI API
34. var response = httpClient.Send(requestMessage);
35.
36. // Ensure the request was successful
37. response.EnsureSuccessStatusCode();
38.
39. // Read and parse the response body
40. var responseBody = response.Content.ReadAsStringAsync().Result; // Use .Result in synchronously wait on the task
41. using var doc = JsonDocument.Parse(responseBody);
42. // Extract the content of the first response message
43. var responseMessage = doc.RootElement.GetProperty("choices")[0].GetProperty("message").GetProperty("content").GetString();
44.
45. return responseMessage ?? throw new Exception("Error reading response.");
46.}
Note that you need to add an API Key API_KEY_OPENAI to the environment.
This code above was generated by GitHub Copilot; I just created the read of the API Key from the Environment. You can change this to any other method you like.
This is the content for Context.docx used in this example based on the original Context.txt.
Section | Content |
---|---|
Title | How Does Telerik DevCraft Cut Development Time? |
Description | Telerik DevCraft is the most comprehensive software development tools collection among the .NET and JavaScript technologies. It features 1,250+ modern, feature-rich, and professionally designed UI components for web, desktop, mobile, and cross-platform applications, embedded reporting and report management solutions, document processing libraries, automated testing, and mocking tools from the Telerik and Kendo UI suites. DevCraft will arm you with everything you need to deliver engaging and inclusive applications in less time and with less effort. |
Statistics |
|
Resources |
|
Key Benefits |
|
Product Features |
|
Call to Action Start 30 day FREE Trial | About Progress Progress (NASDAQ: PRGS) provides the leading products to develop, deploy, and manage high-impact business applications. Our comprehensive product stack is designed to make technology teams more productive and enable organizations to accelerate the creation and delivery of strategic business applications, automate the process by which apps are configured, deployed, and scaled, and make critical data and content more accessible and secure—leading to competitive differentiation and business success. Learn about Progress at www.progress.com or +1-800-477-6473. |
Testimonials |
|
Commitment to Quality | Uncompromised quality from 15+ years of helping millions of developers create beautiful user experiences for mission-critical applications. We live with your daily challenges, striving to solve them with the best products, predictable release cycles and support within hours. |
Changed the MainForm initialize to:
1. var allTextContent = CopyTextFromDocx(@"..\..\..\..\SampleData\Context.docx");
2.
3. Chunks = allTextContent.Split(new[] { ' ' }, StringSplitOptions.RemoveEmptyEntries).Distinct().ToArray();
Code to read the DOCX file (I shared this code on a C# Corner post):
1. // Extract text from a .docx file
2. public static string CopyTextFromDocx(string file)
3. {
4. var docxFormatProvider = new DocxFormatProvider();
5. using var input = File.OpenRead(file);
6. var document = docxFormatProvider.Import(input);
7. var txtFormatProvider = new TxtFormatProvider();
8. return txtFormatProvider.Export(document);
9. }
And changed the way the question is made:
1.private string AnswerQuestion(string question)
2.{
3. // Use all document content:
4.
5. string allContext = string.Join(“ “, PageEmbeddings.Keys);
6.
7. var answer = CallOpenAIApi("You are a helpful assistant. Use the provided context to answer the user question. Context: " + allContext, question);
8.
9. return answer;
10.}
The Result of the Operation
When you start the solution, this is the content of the opened file (New_App_specification.docx):
New app specification:
- I would suggest using Telerik UI for WinForms
- What about Telerik support?
This is the result we get when typing a space after the sentences. I just formatted it after the generation for a better view in this post:
New app specification:
- I would suggest using Telerik UI for WinForms
Great suggestion! Telerik UI for WinForms is a part of the comprehensive Telerik DevCraft suite, providing modern, feature-rich UI components for desktop applications. With Telerik UI for WinForms, developers can create engaging and user-friendly interfaces for their Windows desktop applications. It offers a wide range of controls and themes to standardize the look and feel, while also allowing for limitless customization options. This can significantly reduce development time and effort, increasing productivity. If you are interested, you can start a 30-day free trial to explore all the features and benefits of Telerik UI for WinForms. - What about Telerik support?
Telerik offers industry-leading and flexible technical support resources to simplify everyday tasks for developers. With this support, developers can save up to 50% of their time, increase productivity, and stay up to date with the latest technology and user experience trends. Additionally, Telerik provides standardization of look and feel across different applications, out-of-the-box themes, and limitless customization options to quickly present to users. If you are interested, you can start a 30-day FREE trial to experience the support and other benefits firsthand.
Conclusion
Including artificial intelligence in your products today is not a plus but a minimal requirement and a standard need.
This article guided you to use an alternative to Azure OpenAI which could be expensive regarding account management; working with Azure requires a specialized workforce.
We are at the beginning of society’s transformation using AI generation power, and in the future, this article could make no sense. But today, we can start building an innovative work method, and Progress Telerik is here to help.
We talked about Telerik UI for WinForms, but the SmartAI component is available across all Telerik and Kendo UI libraries, so pick your favorite or choose all of them with Telerik DevCraft.
Feel free to reach out to me on LinkedIn to discuss this content. I’m happy to converse in Portuguese or English.
References
- SmartAI GitHub sample: https://github.com/telerik/smart-ai-components/tree/master/winforms/RichTextEditor
- Sample used in this article: https://github.com/jssmotta/telerikAIComponentRichText
This content originally appeared on Telerik Blogs and was authored by Jefferson S. Motta
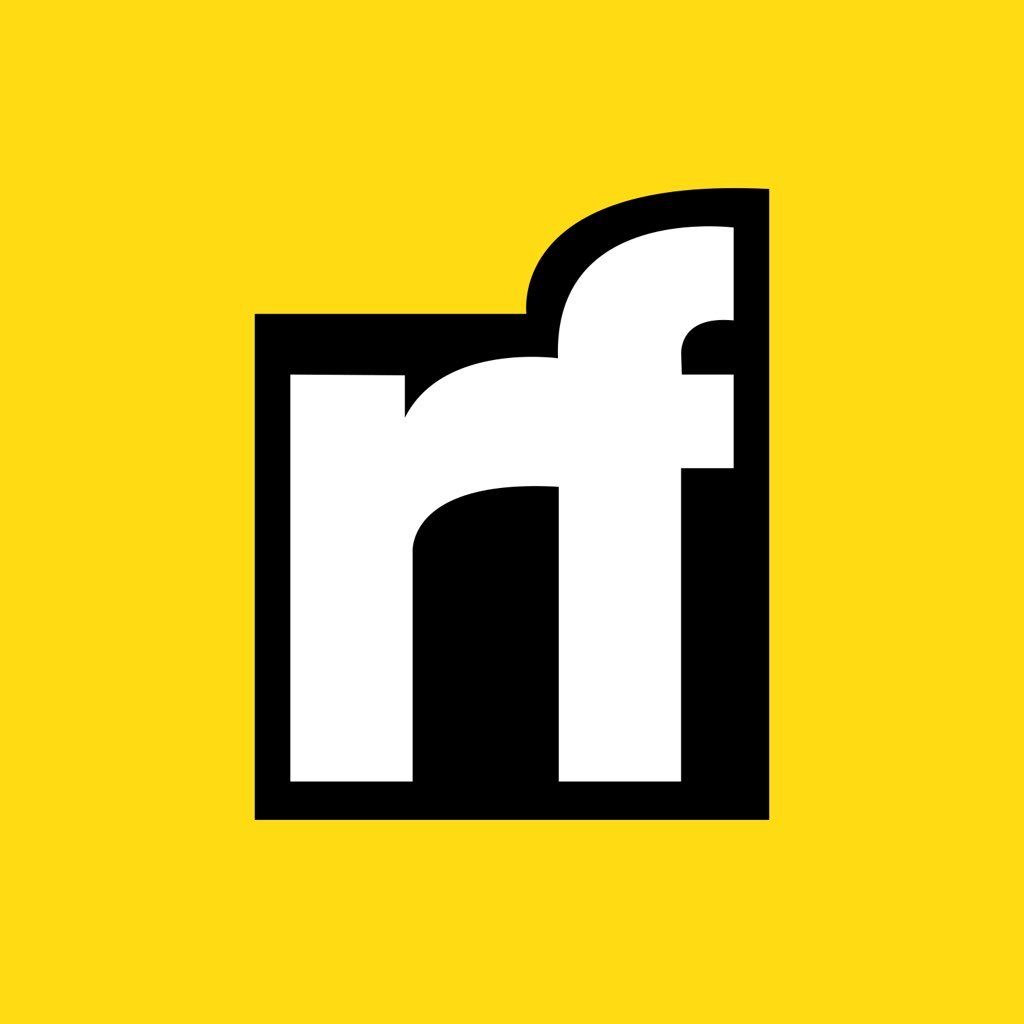
Jefferson S. Motta | Sciencx (2024-08-01T07:34:10+00:00) Using Smart AI Components with OpenAI API and MS Word Document as Context. Retrieved from https://www.scien.cx/2024/08/01/using-smart-ai-components-with-openai-api-and-ms-word-document-as-context/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.