This content originally appeared on Level Up Coding - Medium and was authored by Sonika | @Walmart | FrontEnd Developer | 10 Years
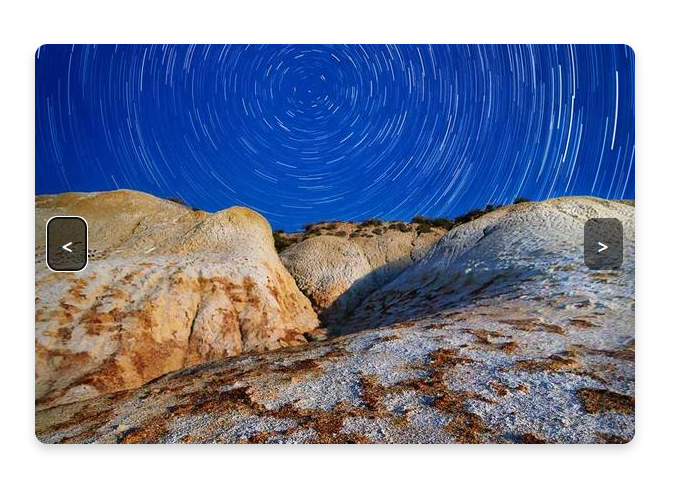
import React, { useState } from 'react';
import './App.css';
const App = () => {
const [slideIndex, setSlideIndex] = useState(0);
const slides = [
'https://picsum.photos/seed/img1/600/400',
'https://picsum.photos/seed/img2/600/400',
'https://picsum.photos/seed/img3/600/400',
];
const showSlide = (n) => {
if (n >= slides.length) {
setSlideIndex(0);
} else if (n < 0) {
setSlideIndex(slides.length - 1);
} else {
setSlideIndex(n);
}
};
const nextSlide = () => {
showSlide(slideIndex + 1);
};
const prevSlide = () => {
showSlide(slideIndex - 1);
};
return (
<div className="carousel-container">
<button className="arrow arrow-left" onClick={prevSlide}>
<
</button>
<div className="slides-container">
{slides.map((slide, index) => (
<img
key={index}
src={slide}
alt={`Image ${index + 1} for carousel`}
className={`slide ${slideIndex === index ? 'active' : ''}`}
/>
))}
</div>
<button className="arrow arrow-right" onClick={nextSlide}>
>
</button>
</div>
);
};
export default App;
/* Carousel.css */
.carousel-container {
position: relative;
width: 80%;
max-width: 600px;
margin: auto;
overflow: hidden;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.2);
}
.slides-container {
display: flex;
transition: transform 0.5s ease-in-out;
}
.slide {
min-width: 100%;
display: none;
}
.slide.active {
display: block;
}
.arrow {
position: absolute;
top: 50%;
transform: translateY(-50%);
background-color: rgba(0, 0, 0, 0.5);
color: white;
border: none;
padding: 10px;
cursor: pointer;
}
.arrow-left {
left: 10px;
}
.arrow-right {
right: 10px;
}
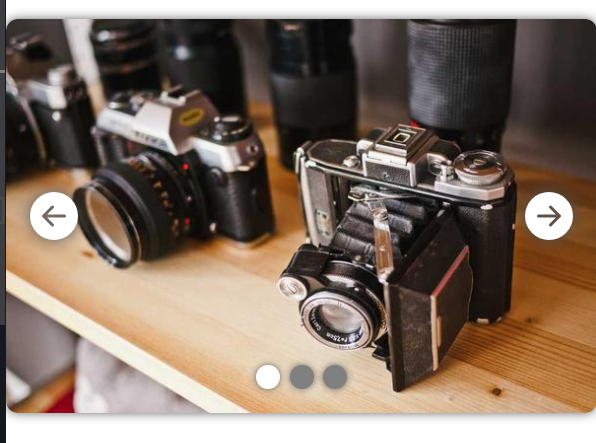
REACT:
app.js
import './App.css';
import { Carousel } from './components/Carousel';
import { slides } from './data/carousel.json';
function App() {
return (
<div className="App">
<Carousel data={slides} />
</div>
);
}
export default App;
data,
{
"slides": [
{
"src": "https://picsum.photos/seed/img1/600/400",
"alt": "Image 1 for carousel"
},
{
"src": "https://picsum.photos/seed/img2/600/400",
"alt": "Image 2 for carousel"
},
{
"src": "https://picsum.photos/seed/img3/600/400",
"alt": "Image 3 for carousel"
}
]
}
import React, { useState } from 'react';
import { BsArrowLeftCircleFill, BsArrowRightCircleFill } from 'react-icons/bs';
import './Carousel.css';
export const Carousel = ({ data }) => {
const [slide, setSlide] = useState(0);
const nextSlide = () => {
setSlide(slide === data.length - 1 ? 0 : slide + 1);
};
const prevSlide = () => {
setSlide(slide === 0 ? data.length - 1 : slide - 1);
};
return (
<div className="carousel">
<BsArrowLeftCircleFill onClick={prevSlide} className="arrow arrow-left" />
{data.map((item, idx) => {
return (
<img
src={item.src}
alt={item.alt}
key={idx}
className={slide === idx ? 'slide' : 'slide slide-hidden'}
/>
);
})}
<BsArrowRightCircleFill
onClick={nextSlide}
className="arrow arrow-right"
/>
<span className="indicators">
{data.map((_, idx) => {
return (
<button
key={idx}
className={
slide === idx ? 'indicator' : 'indicator indicator-inactive'
}
onClick={() => setSlide(idx)}
></button>
);
})}
</span>
</div>
);
};
CSS,
.carousel {
position: relative;
display: flex;
justify-content: center;
align-items: center;
}
.slide {
border-radius: 0.5rem;
box-shadow: 0px 0px 7px #666;
width: 100%;
height: 100%;
}
.slide-hidden {
display: none;
}
.arrow {
position: absolute;
filter: drop-shadow(0px 0px 5px #555);
width: 2rem;
height: 2rem;
color: white;
}
.arrow:hover {
cursor: pointer;
}
.arrow-left {
left: 1rem;
}
.arrow-right {
right: 1rem;
}
.indicators {
display: flex;
position: absolute;
bottom: 1rem;
}
.indicator {
background-color: white;
height: 1rem;
width: 1rem;
border-radius: 100%;
border: none;
outline: none;
box-shadow: 0px 0px 5px #555;
margin: 0 0.2rem;
cursor: pointer;
}
.indicator-inactive {
background-color: grey;
}
.indicators button {
padding: 0;
}
My YouTube Channel: FrontEnd Interview Preparation
JAVASCRIPT:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Carousel</title>
<style>
.carousel-container {
position: relative;
width: 80%;
max-width: 600px;
margin: auto;
overflow: hidden;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.2);
}
.slides-container {
display: flex;
transition: transform 0.5s ease-in-out;
}
.slide {
min-width: 100%;
display: none;
}
.slide.active {
display: block;
}
.arrow {
position: absolute;
top: 50%;
transform: translateY(-50%);
background-color: rgba(0, 0, 0, 0.5);
color: white;
border: none;
padding: 10px;
cursor: pointer;
}
.arrow-left {
left: 10px;
}
.arrow-right {
right: 10px;
}
</style>
</head>
<body>
<div class="carousel-container">
<button class="arrow arrow-left" onclick="prevSlide()"><</button>
<div class="slides-container">
<img src="https://picsum.photos/seed/img1/600/400" alt="Image 1 for carousel" class="slide active">
<img src="https://picsum.photos/seed/img2/600/400" alt="Image 2 for carousel" class="slide">
<img src="https://picsum.photos/seed/img3/600/400" alt="Image 3 for carousel" class="slide">
</div>
<button class="arrow arrow-right" onclick="nextSlide()">></button>
</div>
<script>
let slideIndex = 0;
function showSlide(n) {
const slides = document.querySelectorAll('.slide');
if (n >= slides.length) {
slideIndex = 0;
} else if (n < 0) {
slideIndex = slides.length - 1;
} else {
slideIndex = n;
}
slides.forEach(slide => slide.classList.remove('active'));
slides[slideIndex].classList.add('active');
}
function nextSlide() {
showSlide(slideIndex + 1);
}
function prevSlide() {
showSlide(slideIndex - 1);
}
// Initially show the first slide
showSlide(slideIndex);
</script>
</body>
</html>
OR
// Data for the carousel slides
const data = [
{
src: "https://picsum.photos/seed/img1/600/400",
alt: "Image 1 for carousel"
},
{
src: "https://picsum.photos/seed/img2/600/400",
alt: "Image 2 for carousel"
},
{
src: "https://picsum.photos/seed/img3/600/400",
alt: "Image 3 for carousel"
}
];
// Carousel component
function Carousel({ data }) {
// State to keep track of current slide index
let slide = 0;
// Function to go to the next slide
const nextSlide = () => {
slide = (slide + 1) % data.length;
updateCarousel();
};
// Function to go to the previous slide
const prevSlide = () => {
slide = slide === 0 ? data.length - 1 : slide - 1;
updateCarousel();
};
// Function to update the carousel display
const updateCarousel = () => {
const slides = document.querySelectorAll('.slide');
const indicators = document.querySelectorAll('.indicator');
slides.forEach((slide, idx) => {
if (idx === slide) {
slide.classList.remove('slide-hidden');
} else {
slide.classList.add('slide-hidden');
}
});
indicators.forEach((indicator, idx) => {
if (idx === slide) {
indicator.classList.remove('indicator-inactive');
} else {
indicator.classList.add('indicator-inactive');
}
});
};
// Initial render of carousel
const renderCarousel = () => {
const carouselContainer = document.createElement('div');
carouselContainer.classList.add('carousel');
const leftArrow = document.createElement('button');
leftArrow.classList.add('arrow', 'arrow-left');
leftArrow.innerHTML = '<';
leftArrow.addEventListener('click', prevSlide);
carouselContainer.appendChild(leftArrow);
data.forEach((item, idx) => {
const img = document.createElement('img');
img.src = item.src;
img.alt = item.alt;
img.classList.add('slide');
if (idx !== 0) {
img.classList.add('slide-hidden');
}
carouselContainer.appendChild(img);
});
const rightArrow = document.createElement('button');
rightArrow.classList.add('arrow', 'arrow-right');
rightArrow.innerHTML = '>';
rightArrow.addEventListener('click', nextSlide);
carouselContainer.appendChild(rightArrow);
const indicatorsContainer = document.createElement('span');
indicatorsContainer.classList.add('indicators');
data.forEach((_, idx) => {
const indicator = document.createElement('button');
indicator.classList.add('indicator', idx === 0 ? 'active' : 'inactive');
indicator.addEventListener('click', () => {
slide = idx;
updateCarousel();
});
indicatorsContainer.appendChild(indicator);
});
carouselContainer.appendChild(indicatorsContainer);
document.body.appendChild(carouselContainer);
};
// Call render function
renderCarousel();
// Return empty div (component doesn't render through ReactDOM.render)
return null;
}
// Initialize the carousel component
Carousel({ data });
Build Carousel | React & JavaScript was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Sonika | @Walmart | FrontEnd Developer | 10 Years
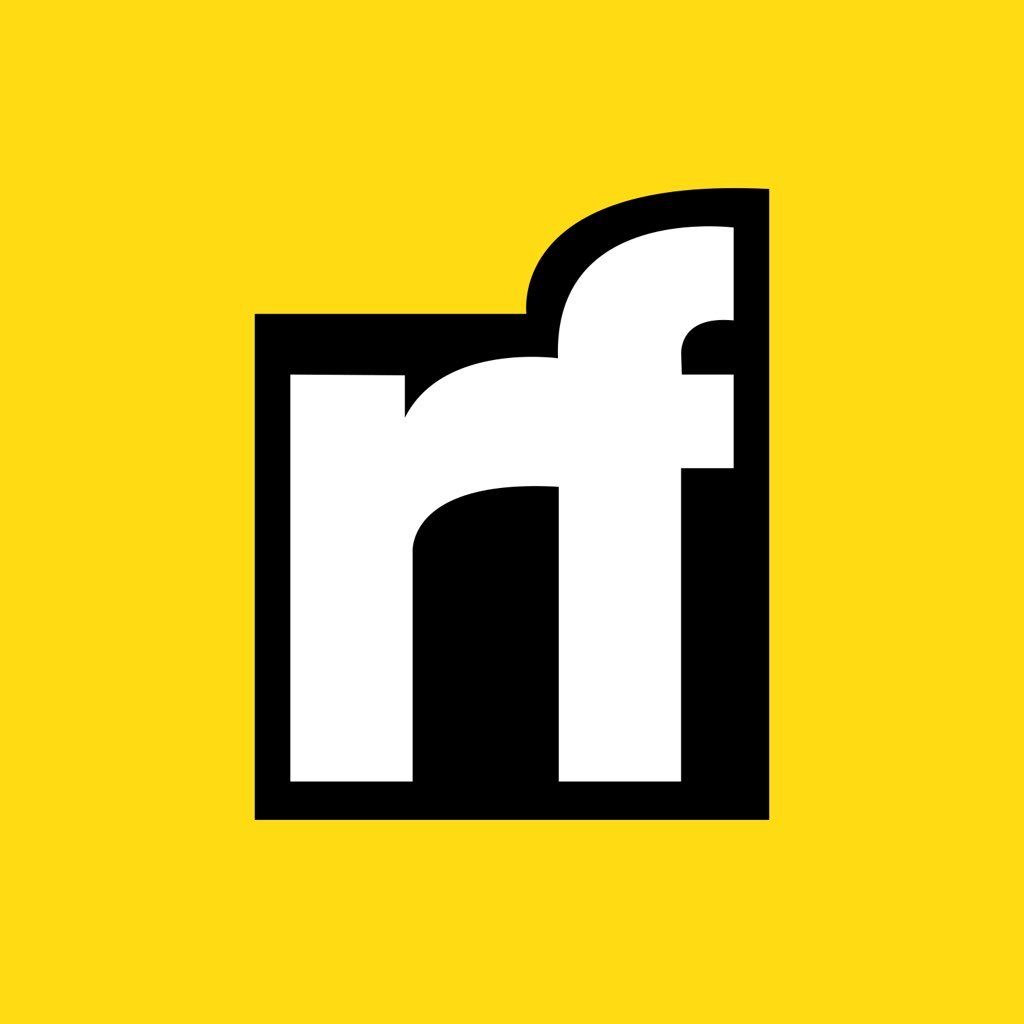
Sonika | @Walmart | FrontEnd Developer | 10 Years | Sciencx (2024-08-09T12:57:22+00:00) Build Carousel | React & JavaScript. Retrieved from https://www.scien.cx/2024/08/09/build-carousel-react-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.