This content originally appeared on DEV Community and was authored by Imran Khan
We’ve covered variables, data types, control structures, functions, lists, and dictionaries. Now, let's introduce another crucial concept: file handling. File handling allows you to read from and write to files, which is essential for managing data that persists beyond the duration of a program’s execution. Understanding file handling will enable you to work with data stored on your computer or any other storage medium.
What is File Handling?
File handling involves operations for reading from and writing to files. This capability is crucial for applications that need to save data, such as configuration settings, user data, or logs. Think of file handling as a way to communicate with the file system on your computer, allowing your program to store and retrieve information efficiently.
Basic File Operations
Here are the fundamental operations you can perform with files:
- Opening a File: Before you can read from or write to a file, you need to open it.
- Reading from a File: Extract information from a file.
- Writing to a File: Save or update information in a file.
- Closing a File: Always close a file after finishing operations to free up system resources.
How to Handle Files in Python
1. Opening a File:
Use the open()
function to open a file. This function takes the filename and the mode as arguments. Common modes include:
-
"r"
: Read (default mode). -
"w"
: Write (creates a new file or overwrites an existing one). -
"a"
: Append (adds to an existing file).
Example:
file = open("example.txt", "w") # Open a file in write mode
2. Writing to a File:
Use the write()
method to write data to the file.
Example:
file = open("example.txt", "w")
file.write("Hello, world!") # Write text to the file
file.close() # Close the file
3. Reading from a File:
Use methods like read()
, readline()
, or readlines()
to read data from a file.
Example:
file = open("example.txt", "r")
content = file.read() # Read the entire file
print(content) # Output: Hello, world!
file.close()
4. Using Context Managers:
Context managers simplify file handling by automatically closing the file after operations are completed. Use the with
statement for better resource management.
Example:
with open("example.txt", "w") as file:
file.write("Hello, world!") # File is automatically closed when done
Practical Applications of File Handling
1. Storing User Data:
Save user preferences, settings, or data to files so that they persist between sessions.
Example:
user_data = {"name": "Alice", "age": 25}
with open("user_data.txt", "w") as file:
file.write(str(user_data)) # Convert dictionary to string and write to file
2. Reading Configuration Files:
Load configuration settings from a file to customize program behavior.
Example:
with open("config.txt", "r") as file:
config = file.read() # Read configuration settings
print(config)
3. Analyzing Log Files:
Read and analyze log files to monitor program behavior or errors.
Example:
with open("log.txt", "r") as file:
for line in file:
print(line.strip()) # Print each line from the log file
Practice Exercises
To practice file handling, try these exercises:
- Create and Write to a File: Write a program that creates a file and writes your name and age to it.
- Read File Content: Write a program that reads the content of a file and prints it to the console.
- Append Data: Write a program that appends new data to an existing file.
- Save and Load User Preferences: Write a program that saves user preferences (like theme or font size) to a file and loads them when the program starts.
Conclusion
File handling is a vital skill for managing data in programming. By understanding how to open, read, write, and close files, you can store and retrieve data efficiently, making your programs more versatile and practical. Keep practicing these techniques, and you'll be able to handle data storage tasks with confidence.
Happy coding!
7. File Handling
These resources will provide you with detailed explanations, tutorials, and examples on Object-Oriented Programming and its core concepts. Happy learning!
This content originally appeared on DEV Community and was authored by Imran Khan
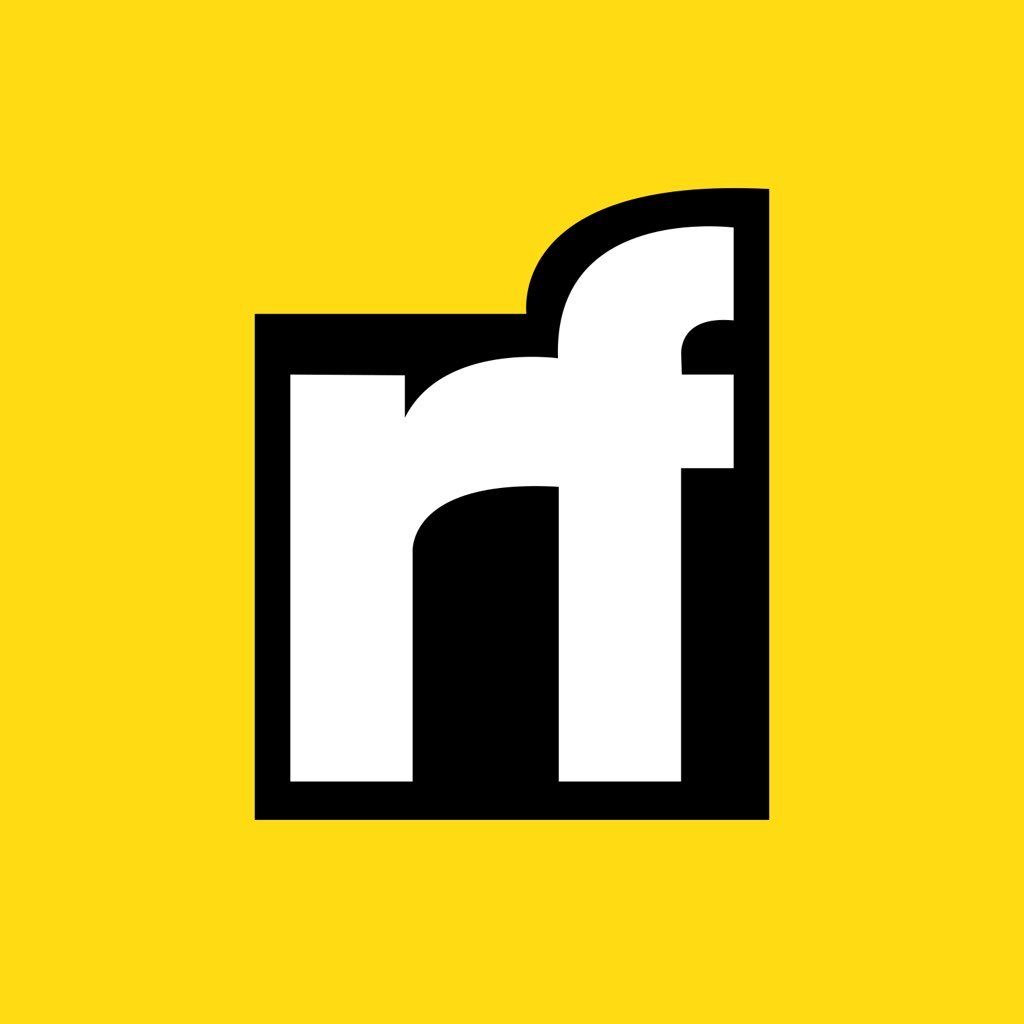
Imran Khan | Sciencx (2024-08-10T16:52:49+00:00) ### Introduction to Programming: Exploring Dictionaries and Beyond. Retrieved from https://www.scien.cx/2024/08/10/introduction-to-programming-exploring-dictionaries-and-beyond/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.