This content originally appeared on Level Up Coding - Medium and was authored by Sonika | @Walmart | FrontEnd Developer | 10 Years
Build a Rock Paper Scissors game where the user plays against the computer.
Requirements
- Create a Rock Paper Scissors game where the user can choose Rock, Paper, or Scissors.
- The computer randomly selects Rock, Paper, or Scissors.
- Display the user’s choice, the computer’s choice, and the result (win, lose, or draw).
- Provide a button to play again.
Notes
- The focus of this challenge is on game logic and handling user interactions.
- You may use simple styling to make the game visually appealing.
Rock Paper Scissors Game
Choices:
- Rock
- Paper
- Scissors
Buttons:
- Rock
- Paper
- Scissors
- Play Again
Expected Output
- A game where the user can select Rock, Paper, or Scissors.
- The computer randomly selects Rock, Paper, or Scissors.
- Display the choices and the result.
- A button to play again.
React Implementation,
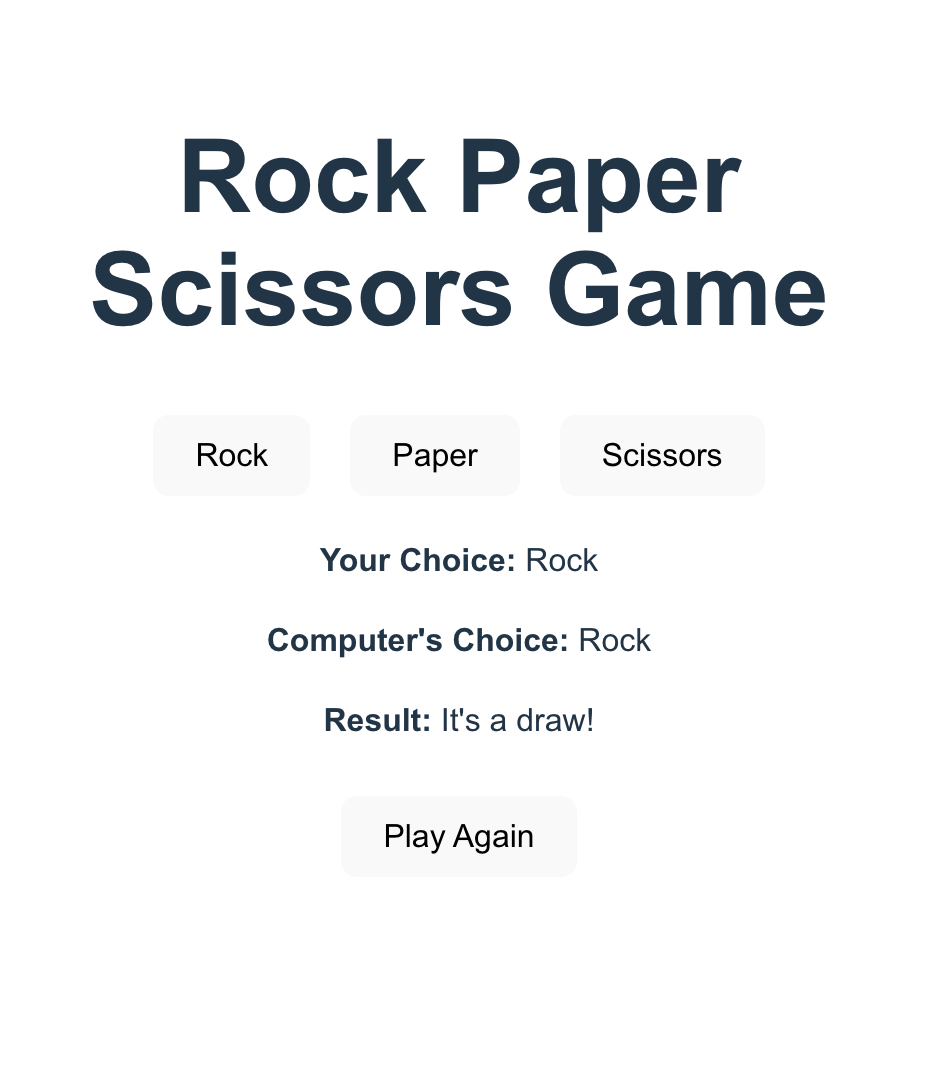
import React, { useState } from 'react';
const choices = ['Rock', 'Paper', 'Scissors'];
const getRandomChoice = () => {
return choices[Math.floor(Math.random() * choices.length)];
};
const determineWinner = (userChoice, computerChoice) => {
if (userChoice === computerChoice) {
return "It's a draw!";
}
if (
(userChoice === 'Rock' && computerChoice === 'Scissors') ||
(userChoice === 'Paper' && computerChoice === 'Rock') ||
(userChoice === 'Scissors' && computerChoice === 'Paper')
) {
return 'You win!';
}
return 'You lose!';
};
const RockPaperScissors = () => {
const [userChoice, setUserChoice] = useState('');
const [computerChoice, setComputerChoice] = useState('');
const [result, setResult] = useState('');
const handleUserChoice = (choice) => {
const computerSelectedChoice = getRandomChoice();
setUserChoice(choice);
setComputerChoice(computerSelectedChoice);
setResult(determineWinner(choice, computerSelectedChoice));
};
const handlePlayAgain = () => {
setUserChoice('');
setComputerChoice('');
setResult('');
};
return (
<div style={styles.container}>
<h1>Rock Paper Scissors Game</h1>
<div style={styles.choicesContainer}>
<button
onClick={() => handleUserChoice('Rock')}
style={styles.choiceButton}
>
Rock
</button>
<button
onClick={() => handleUserChoice('Paper')}
style={styles.choiceButton}
>
Paper
</button>
<button
onClick={() => handleUserChoice('Scissors')}
style={styles.choiceButton}
>
Scissors
</button>
</div>
{userChoice && computerChoice && (
<div style={styles.resultContainer}>
<p>
<strong>Your Choice:</strong> {userChoice}
</p>
<p>
<strong>Computer's Choice:</strong> {computerChoice}
</p>
<p>
<strong>Result:</strong> {result}
</p>
<button onClick={handlePlayAgain} style={styles.playAgainButton}>
Play Again
</button>
</div>
)}
</div>
);
};
const styles = {
container: {
textAlign: 'center',
fontFamily: 'Arial, sans-serif',
padding: '20px',
},
choicesContainer: {
display: 'flex',
justifyContent: 'center',
marginBottom: '20px',
},
choiceButton: {
margin: '0 10px',
padding: '10px 20px',
fontSize: '16px',
cursor: 'pointer',
},
resultContainer: {
marginTop: '20px',
},
playAgainButton: {
marginTop: '10px',
padding: '10px 20px',
fontSize: '16px',
cursor: 'pointer',
},
};
export default RockPaperScissors;
Thanks for reading
- 👏 Please clap for the story and follow me 👉
- 📰 View more content on Coding and System Design Interviews
- 🔔 Follow me: LinkedIn!
React | Rock Paper Scissors Game was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Sonika | @Walmart | FrontEnd Developer | 10 Years
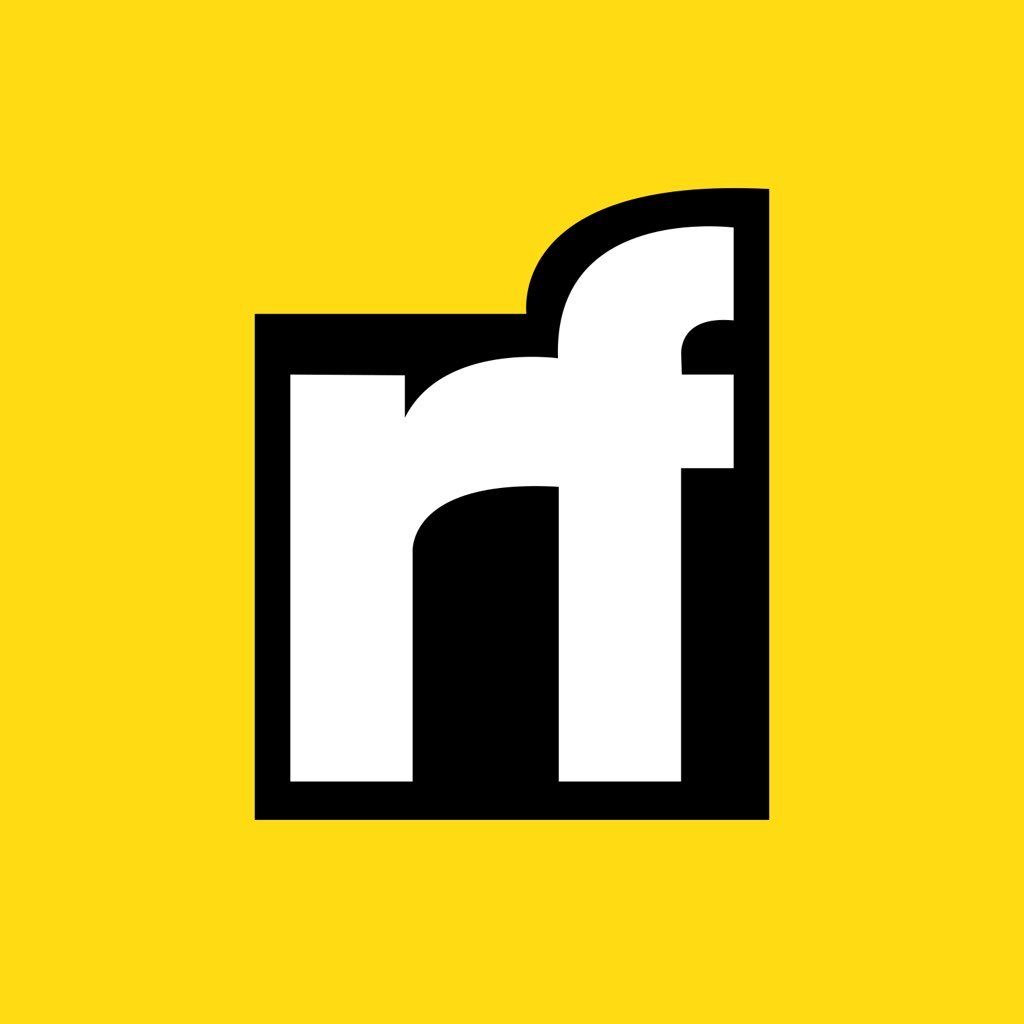
Sonika | @Walmart | FrontEnd Developer | 10 Years | Sciencx (2024-08-15T18:04:20+00:00) React | Rock Paper Scissors Game. Retrieved from https://www.scien.cx/2024/08/15/react-rock-paper-scissors-game/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.