This content originally appeared on DEV Community and was authored by Aadarsh Kunwar
In Flutter, there are two common ways to navigate between pages or views: using the Navigator and implementing a page view with PageView.
- Using Navigator for Navigation Between Pages The Navigator is the primary way to manage a stack of routes, allowing you to push and pop routes (pages) to navigate between them.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Home Page')),
body: Center(
child: ElevatedButton(
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(builder: (context) => SecondPage()),
);
},
child: Text('Go to Second Page'),
),
),
);
}
}
class SecondPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Second Page')),
body: Center(
child: ElevatedButton(
onPressed: () {
Navigator.pop(context);
},
child: Text('Go back to Home Page'),
),
),
);
}
}
- Using PageView for Swiping Between Pages PageView is useful for scenarios where you want to allow users to swipe between pages, similar to a carousel or a stepper.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: PageViewExample(),
);
}
}
class PageViewExample extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('PageView Example')),
body: PageView(
children: <Widget>[
Container(
color: Colors.red,
child: Center(child: Text('Page 1', style: TextStyle(fontSize: 24))),
),
Container(
color: Colors.green,
child: Center(child: Text('Page 2', style: TextStyle(fontSize: 24))),
),
Container(
color: Colors.blue,
child: Center(child: Text('Page 3', style: TextStyle(fontSize: 24))),
),
],
),
);
}
}
This content originally appeared on DEV Community and was authored by Aadarsh Kunwar
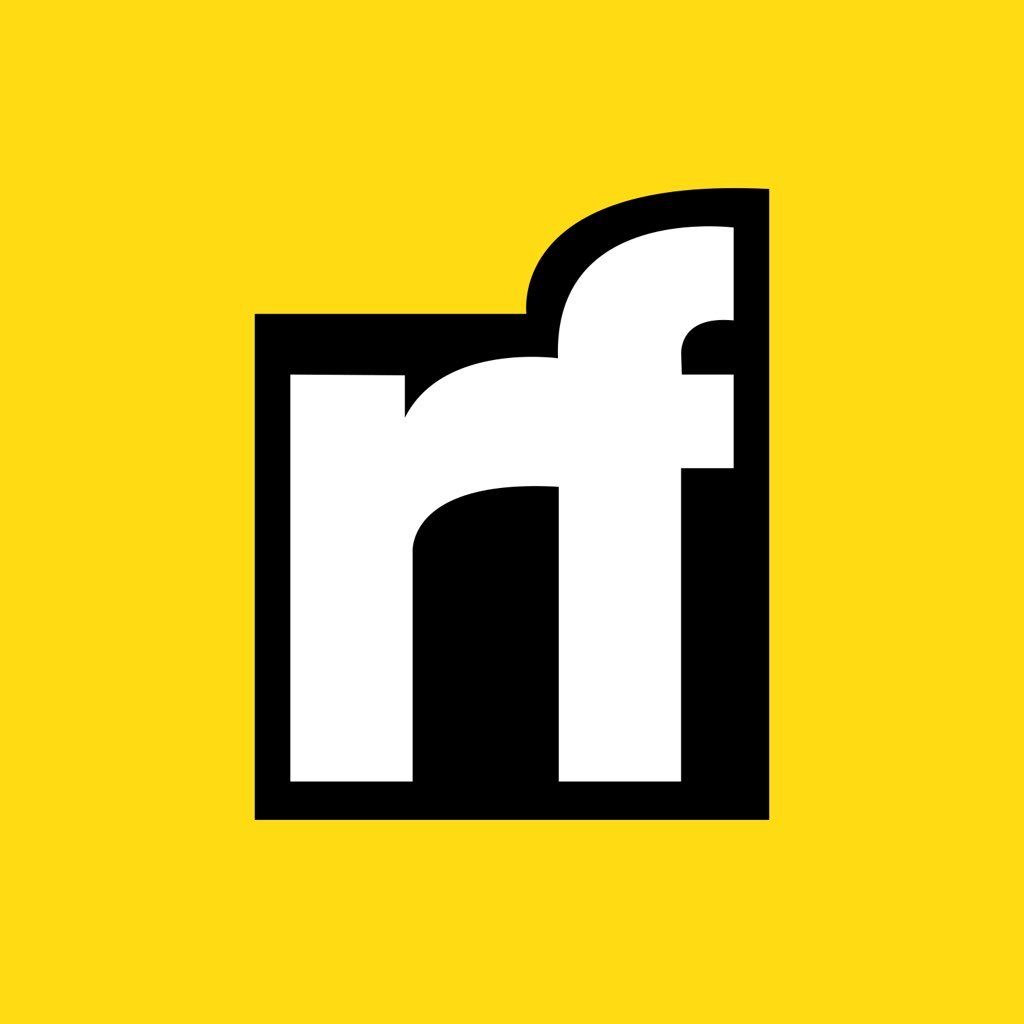
Aadarsh Kunwar | Sciencx (2024-08-17T17:36:16+00:00) Flutter PageView or Navigator. Retrieved from https://www.scien.cx/2024/08/17/flutter-pageview-or-navigator/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.