This content originally appeared on DEV Community and was authored by mibii
Building a Full-Stack Web App: From Authentication to API Integration
Hello, fellow code enthusiasts! Today, I'm excited to share my journey of building a full-stack web application that combines user authentication, backend API integration, and frontend development. This project is perfect for beginners looking to expand their skills across different technologies. Let's dive in!
The Big Picture
Our goal was to create a web app that allows users to:
Sign up and log in
Submit text to an AI model (in this case, the Gemini API)
View the AI-generated response
Sounds simple, right? Well, there's a lot going on under the hood!
Backend Magic with Node.js and Express
We started by setting up our backend using Node.js and Express. Here are the key components:
User Authentication: We implemented signup and login routes using JSON Web Tokens (JWT) for secure authentication.
File-based User Storage: Instead of a database, we used a JSON file to store user information. It's a simple solution for small projects or prototypes.
API Integration: We created a route to handle requests to the Gemini API, acting as a middleman between our frontend and the AI service.
app.post('/process-input', authenticateJWT, async (req, res) => {
// API call logic here
});
Frontend: Where It All Comes Together
For the frontend, we used plain HTML, CSS, and JavaScript. Here's what we focused on:
- Forms for User Interaction: We created forms for signup, login, and text input.
- Asynchronous Requests: We used fetch to make asynchronous calls to our backend.
- Dynamic Content Updates: We updated the DOM to display the API response without reloading the page.
- Error Handling: We implemented basic error handling to improve user experience.
The Secret Sauce: JWT Authentication
JSON Web Tokens (JWT) were crucial for maintaining user sessions. Here's how we used them:
- Generate a token upon successful login
- Send the token with each request to protected routes
- Verify the token on the backend before processing requests
const authenticateJWT = (req, res, next) => {
const token = req.headers.authorization.split(' ')[1];
// Verify token logic here
};
App working results
Do sign up and log in. Then ask any question:
Key Takeaways for Beginners
Start Small, Then Expand: Begin with core functionality and add features incrementally.
Security Matters: Always consider security, even in small projects. JWT is a great starting point.
User Experience is Key: Small touches like loaders can significantly improve your app's feel.
Learn by Doing: This project touched on frontend, backend, API integration, and authentication – all valuable skills!
What's Next?
There's always room for improvement! Here are some ideas to take this project further:
- Implement a proper database (e.g., MongoDB)
- Add more robust error handling and input validation
- Enhance the UI with a frontend framework like React
- Implement user profiles and history of API requests
Remember, the journey of a developer is all about continuous learning. Each project teaches you something new, so keep coding and exploring!
Deployment link to see the app: https://askai-56ol.onrender.com/
App code source: https://buymeacoffee.com/techmobilebox/e/291063
This content originally appeared on DEV Community and was authored by mibii
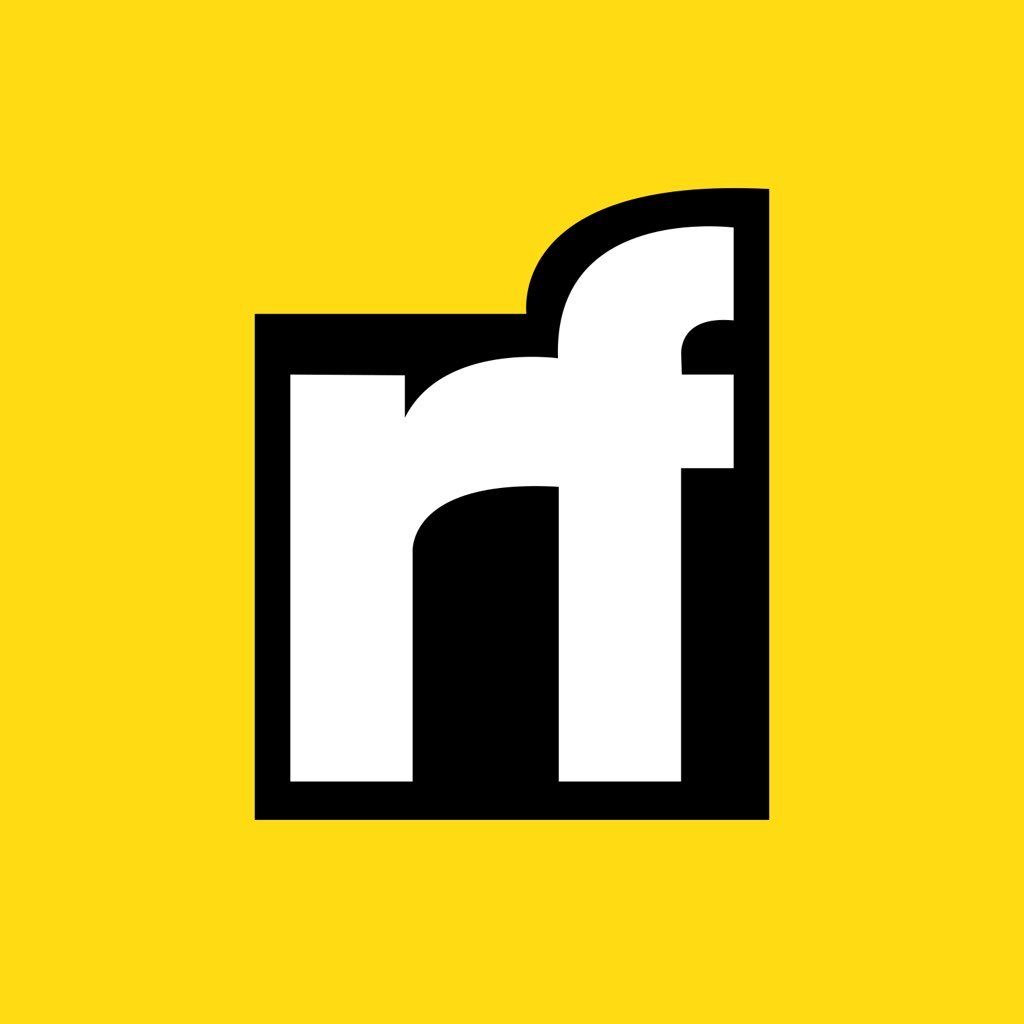
mibii | Sciencx (2024-08-18T02:23:10+00:00) Create your own AI asking App (Submit text to an AI model View the AI-generated response). Retrieved from https://www.scien.cx/2024/08/18/create-your-own-ai-asking-app-submit-text-to-an-ai-model-view-the-ai-generated-response/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.