This content originally appeared on DEV Community and was authored by TD!
It's Day #4 of #100daysofMiva. See Git repository for Random color project.
The provided React component, RandomColor, generates and displays random colors in either HEX or RGB format. Users can switch between these formats and generate new colors using buttons.
I did not encounter much trouble with the project because I already did most of the battle yesterday. So let's dive in:
getRandomNumber
Function
function getRandomNumber(limit) {
return Math.floor(Math.random() * limit);
This function generates a random integer between '0' and 'limit - 1'.
generateRandomHexColor
Function
function generateRandomHexColor() {
const hexChars = "0123456789ABCDEF";
let hexColor = "#";
for (let i = 0; i < 6; i++) {
hexColor += hexChars[getRandomNumber(16)];
}
return hexColor;
}
This function generates a random HEX color by randomly selecting characters from the string '0123456789ABCDEF'.
generateRandomRgbColor
Function
function generateRandomRgbColor() {
const r = getRandomNumber(256);
const g = getRandomNumber(256);
const b = getRandomNumber(256);
return `rgb(${r},${g},${b})`;
}
This function generates a random RGB color by generating random values for red, green, and blue channels.
Rendering the Component
The component is rendered with three buttons: one to switch to HEX, one to switch to RGB, and one to generate a new color in the current format. The color type and value are displayed in a visually appealing manner.
return (
<div style={containerStyles}>
<button style={buttonStyles} onClick={() => setTypeOfColor("hex")}>
Create HEX Color
</button>
<button style={buttonStyles} onClick={() => setTypeOfColor("rgb")}>
Create RGB Color
</button>
<button style={buttonStyles} onClick={generateColor}>
Generate Random Color
</button>
<div style={colorDisplayStyles}>
<h3>{typeOfColor === "rgb" ? "RGB Color" : "HEX Color"}</h3>
<h1>{color}</h1>
</div>
</div>
);
This content originally appeared on DEV Community and was authored by TD!
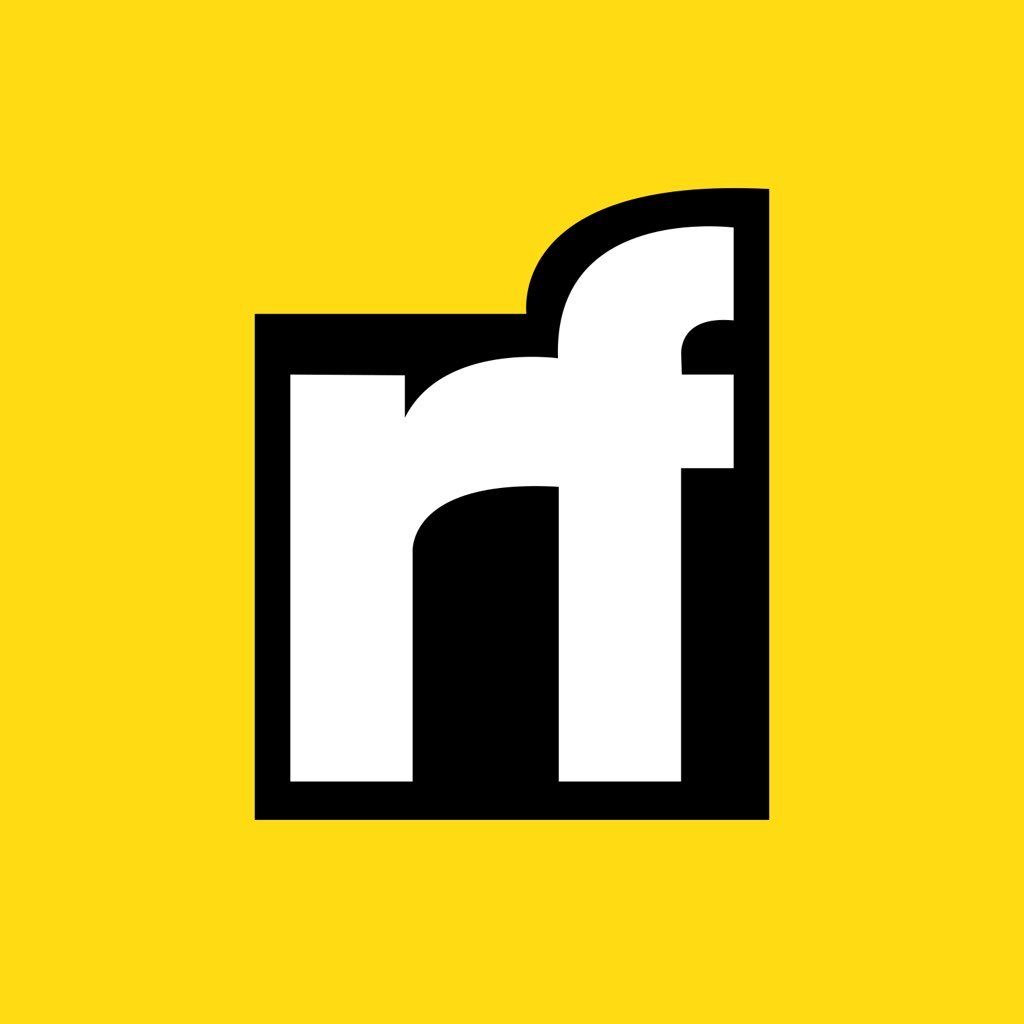
TD! | Sciencx (2024-08-24T05:04:52+00:00) Simple REACT Projects || Random color project. Retrieved from https://www.scien.cx/2024/08/24/simple-react-projects-random-color-project/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.