This content originally appeared on Level Up Coding - Medium and was authored by Aman Saxena
In today’s digital landscape, securing applications is more critical than ever. As Golang (Go) continues to rise in popularity for developing scalable and high-performance applications, it’s essential to ensure that these applications are secure from potential threats. This article provides a comprehensive guide to securing Go applications, covering architecture, best practices, and practical code examples.
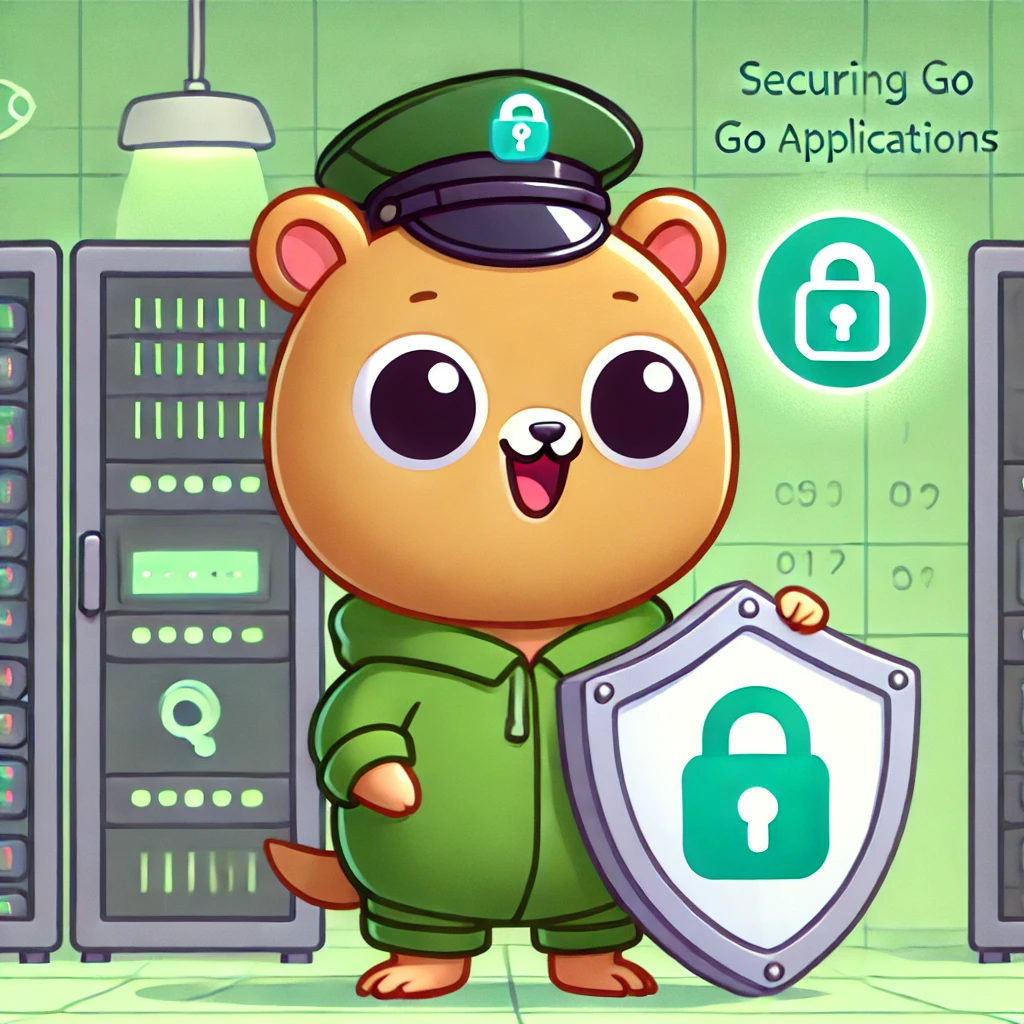
Architecture Overview
Before diving into the specifics, let’s start with a high-level architecture diagram that outlines the typical components of a secure Go application.
Architecture Diagram Description:
- Client Side: This includes user devices like desktops, mobile phones, or tablets that interact with the Go application via a web or mobile interface.
- API Gateway: Acts as an entry point for client requests, performing initial security checks such as rate limiting, IP whitelisting, and routing to appropriate services.
- Authentication and Authorization Service: Responsible for managing user identities, roles, and permissions. This service ensures that only authenticated and authorized users can access specific resources.
- Application Logic Layer: The core of your Go application, where business logic is implemented. This layer is secured by validating input, implementing secure coding practices, and using middleware to handle security concerns.
- Database: Stores application data. Secure this layer with encryption, access control, and regular audits to prevent unauthorized access.
- Monitoring and Logging: Continuous monitoring and logging of application activities help in early detection of anomalies or security breaches.
+---------------------------+
| Client Side |
| (Desktops, Mobiles, etc.) |
+--------------+------------+
|
v
+--------------+---------------+
| API Gateway |
| (Rate Limiting, IP Whitelist)|
+---------------+--------------+
|
v
+-----------------------+-----------------------+
| |
v v
+-------------------------------+ +-----------------------------+
| Authentication & Authorization| | Application Logic Layer |
| (Identity, Roles, Access) | | (Business Logic, Middleware)|
+-------------------------------+ +-----------------------------+
| |
v v
+----------------------+ +------------------------------+
| Database (Encrypted| | Monitoring & Logging |
| ,Access Controlled) | |(Continuous Activity Tracking)|
+----------------------+ +------------------------------+
Best Practices for Securing Go Applications
1. Input Validation and Sanitization
Always validate and sanitize inputs from users to prevent injection attacks such as SQL injection or cross-site scripting (XSS).
import (
"net/http"
"regexp"
)
func validateInput(input string) bool {
validInput := regexp.MustCompile(`^[a-zA-Z0-9_]+$`)
return validInput.MatchString(input)
}
func handler(w http.ResponseWriter, r *http.Request) {
userInput := r.URL.Query().Get("input")
if !validateInput(userInput) {
http.Error(w, "Invalid input", http.StatusBadRequest)
return
}
// Process input
}
Explanation: This code snippet shows a simple input validation function using a regular expression to ensure that the input only contains alphanumeric characters and underscores. Invalid input is rejected, preventing potential injection attacks.
2. Use Secure Authentication Mechanisms
Implement robust authentication mechanisms such as OAuth2, JWT (JSON Web Tokens), or TLS client certificates. Never store passwords in plain text — always use secure hashing algorithms like bcrypt.
import (
"golang.org/x/crypto/bcrypt"
)
func hashPassword(password string) (string, error) {
bytes, err := bcrypt.GenerateFromPassword([]byte(password), bcrypt.DefaultCost)
return string(bytes), err
}
func checkPasswordHash(password, hash string) bool {
err := bcrypt.CompareHashAndPassword([]byte(hash), []byte(password))
return err == nil
}
Explanation: This example demonstrates how to securely hash and verify passwords using bcrypt, which is resistant to brute-force attacks.
3. Implement Proper Error Handling
Avoid exposing internal error messages to users, as they can reveal sensitive information. Instead, log detailed errors internally and display generic messages to users.
func handler(w http.ResponseWriter, r *http.Request) {
_, err := someSensitiveOperation()
if err != nil {
log.Printf("Internal error: %v", err)
http.Error(w, "Something went wrong", http.StatusInternalServerError)
return
}
// Continue processing
}
Explanation: The code logs the detailed error message internally but returns a generic error message to the client, minimizing the risk of information leakage.
4. Secure Data at Rest and in Transit
Encrypt sensitive data at rest using tools like AWS KMS or custom encryption methods in Go. Ensure data in transit is encrypted using HTTPS/TLS.
import (
"crypto/aes"
"crypto/cipher"
"crypto/rand"
"io"
)
func encrypt(data []byte, passphrase string) ([]byte, error) {
block, _ := aes.NewCipher([]byte(passphrase))
gcm, err := cipher.NewGCM(block)
if err != nil {
return nil, err
}
nonce := make([]byte, gcm.NonceSize())
if _, err = io.ReadFull(rand.Reader, nonce); err != nil {
return nil, err
}
ciphertext := gcm.Seal(nonce, nonce, data, nil)
return ciphertext, nil
}
Explanation: This code snippet shows how to encrypt data using AES-GCM, a strong encryption standard. The data is encrypted before being stored, ensuring its security at rest.
5. Use Dependency Management and Monitor Vulnerabilities
Regularly update dependencies using Go Modules and monitor for vulnerabilities. Use tools like gosec to scan your Go code for security issues.
go get -u all
gosec ./...
Explanation: Keeping dependencies updated and scanning for security vulnerabilities ensures that your application is protected from known exploits.
6. Implement Role-Based Access Control (RBAC)
Use RBAC to ensure that users only have the permissions necessary for their roles. This minimizes the risk of unauthorized access.
type User struct {
ID string
Roles []string
}
func hasRole(user User, role string) bool {
for _, r := range user.Roles {
if r == role {
return true
}
}
return false
}
Explanation: The example checks if a user has a specific role before allowing access to certain functionality, ensuring that permissions are properly enforced.
7. Regular Security Audits and Penetration Testing
Conduct regular security audits and penetration testing to identify and fix vulnerabilities. Use both automated tools and manual testing for comprehensive coverage.
Conclusion
Securing Go applications is an ongoing process that involves implementing best practices, regular updates, and continuous monitoring. By following the guidelines outlined in this article, you can significantly reduce the risk of security breaches and ensure that your Go applications are robust and secure.
Feel free to connect with me on LinkedIn!
Securing Go Applications: A Comprehensive Guide to Best Practices was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Aman Saxena
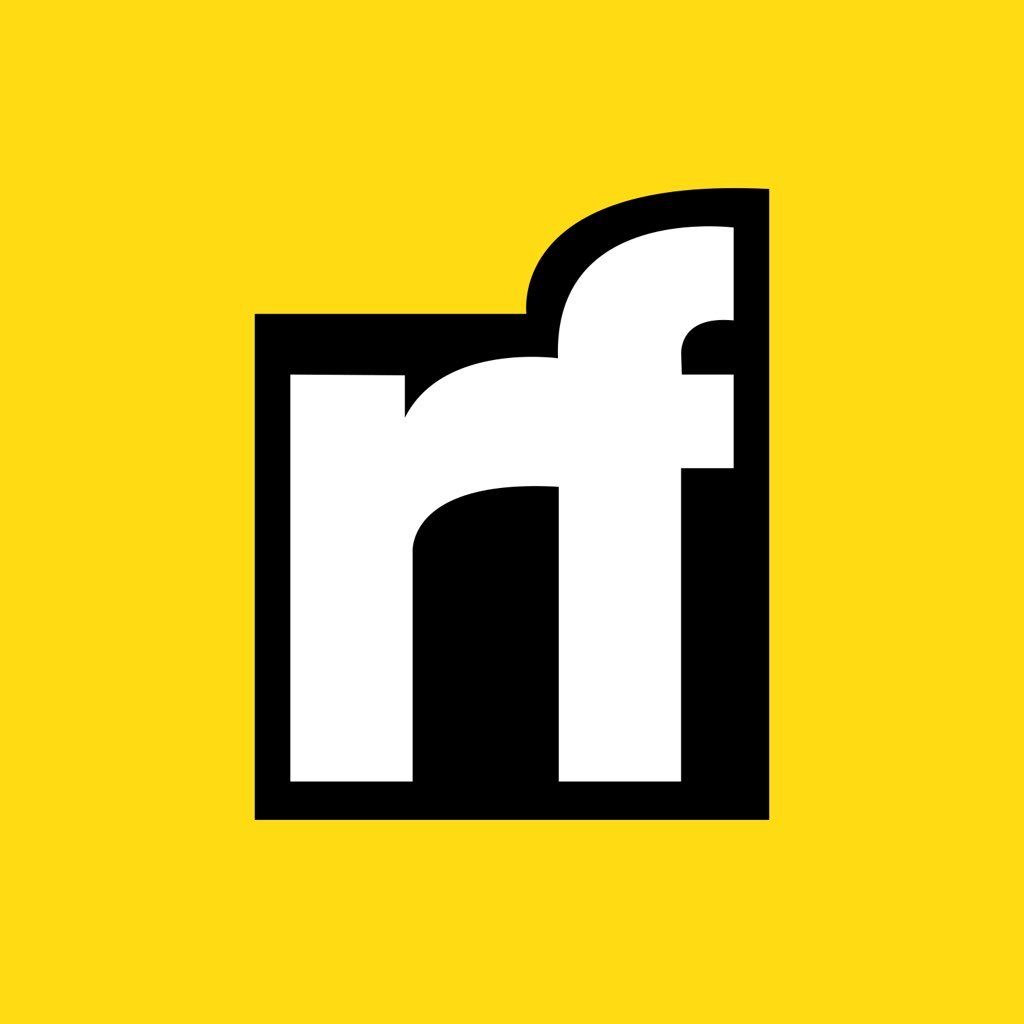
Aman Saxena | Sciencx (2024-08-25T15:31:52+00:00) Securing Go Applications: A Comprehensive Guide to Best Practices. Retrieved from https://www.scien.cx/2024/08/25/securing-go-applications-a-comprehensive-guide-to-best-practices/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.