This content originally appeared on DEV Community and was authored by Saepul Malik
Selama kurang lebih 5 tahun saya bekerja sebagai web developer, 3 fungsi array ini adalah yang paling sering saya gunakan untuk mengelola data dan berinteraksi dengan array. untuk react project sendiri 3 fungsi array ini sangatlah powerfull untuk pengolahan data, berikut kurang lebih penggunaan yang efektif dari ke 3 fungsi tersebut.
Map untuk renderList
import React from 'react';
const users = ['Alice', 'Bob', 'Charlie'];
function UserList() {
return (
<ul>
{users.map((user, index) => (
<li key={index}>{user}</li>
))}
</ul>
);
}
export default UserList;
Filter untuk kondisional rendering
import React from 'react';
const users = ['Al', 'Bob', 'Charlie'];
function UserList() {
const filteredUsers = users.filter(user => user.length > 3);
return (
<ul>
{filteredUsers.map((user, index) => (
<li key={index}>{user}</li>
))}
</ul>
);
}
export default UserList;
Reduce untuk Menghitung Data
import React from 'react';
const products = [
{ id: 1, name: 'Laptop', price: 1500 },
{ id: 2, name: 'Phone', price: 800 },
{ id: 3, name: 'Tablet', price: 1200 }
];
function TotalPrice() {
const totalPrice = products.reduce((acc, product) => acc + product.price, 0);
return (
<div>
<h2>Total Price: ${totalPrice}</h2>
</div>
);
}
export default TotalPrice;
Menggabungkan map, filter, dan reduce dalam React
import React from 'react';
const products = [
{ id: 1, name: 'Laptop', price: 1500, discount: 200 },
{ id: 2, name: 'Phone', price: 800, discount: 50 },
{ id: 3, name: 'Tablet', price: 1200, discount: 100 }
];
function DiscountedProducts() {
const discountedProducts = products.filter(product => product.discount > 0);
const totalDiscount = discountedProducts.reduce((acc, product) => acc + product.discount, 0);
return (
<div>
<h2>Total Discount: ${totalDiscount}</h2>
<ul>
{discountedProducts.map(product => (
<li key={product.id}>{product.name} - Discount: ${product.discount}</li>
))}
</ul>
</div>
);
}
export default DiscountedProducts;
Kesimpulan
Dalam aplikasi React, map, filter, dan reduce bukan hanya alat untuk memanipulasi data, tetapi juga cara untuk merender UI secara dinamis dan efisien. Dengan memahami dan menguasai fungsi-fungsi ini, kita bisa membuat aplikasi yang lebih modular, mudah dibaca, dan scalable. Jadi, terus eksplorasi dan terapkan fungsi-fungsi ini dalam proyek React kita untuk hasil yang maksimal
This content originally appeared on DEV Community and was authored by Saepul Malik
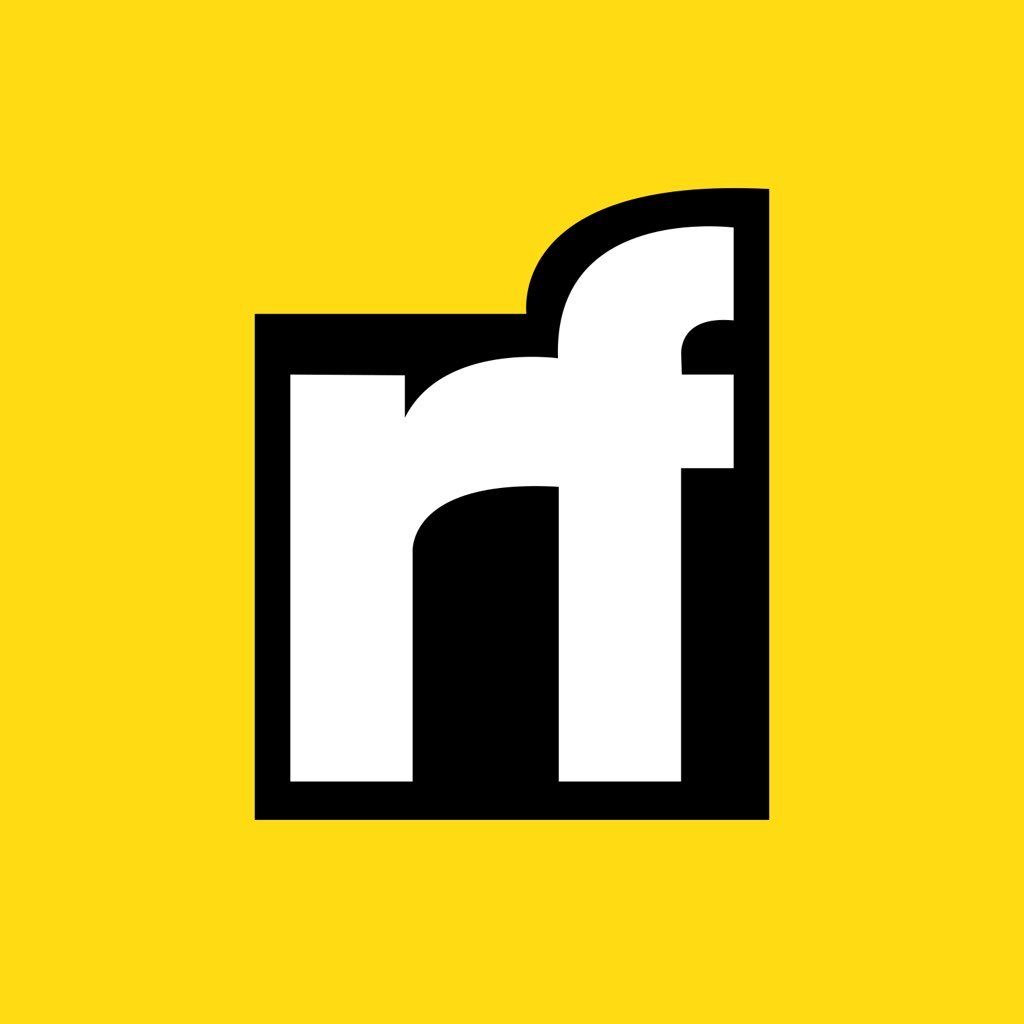
Saepul Malik | Sciencx (2024-08-27T02:04:31+00:00) Memanfaatkan reduce, map, dan filter untuk Pengolahan Data dalam React Project. Retrieved from https://www.scien.cx/2024/08/27/memanfaatkan-reduce-map-dan-filter-untuk-pengolahan-data-dalam-react-project/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.