This content originally appeared on DEV Community and was authored by Pranav Bakare
An Immediately Invoked Function Expression (IIFE) is a JavaScript function that runs as soon as it is defined. It is commonly used to avoid polluting the global scope or to create a private scope for variables.
Here’s a simple example of an IIFE:
(function() {
var message = "Hello from IIFE!";
console.log(message);
})();
Explanation:
- The function is wrapped in parentheses:
(function() { ... })
. This makes the JavaScript engine treat it as an expression. - Immediately after the closing parenthesis of the function, another set of parentheses
()
is added to invoke the function immediately. - The function runs right after it is defined, logging
"Hello from IIFE!"
to the console.
Output:
Hello from IIFE!
Usage:
IIFEs are useful when you want to create a new scope, especially to protect variables from being accessed or modified outside of the function:
(function() {
var counter = 0; // This counter is private and can't be accessed from outside
function increment() {
counter++;
console.log(counter);
}
increment(); // Logs: 1
increment(); // Logs: 2
})();
console.log(typeof counter); // Logs: "undefined", because `counter` is not accessible here.
This ensures that variables like counter
remain private and are not accidentally modified or accessed from other parts of the code.
This content originally appeared on DEV Community and was authored by Pranav Bakare
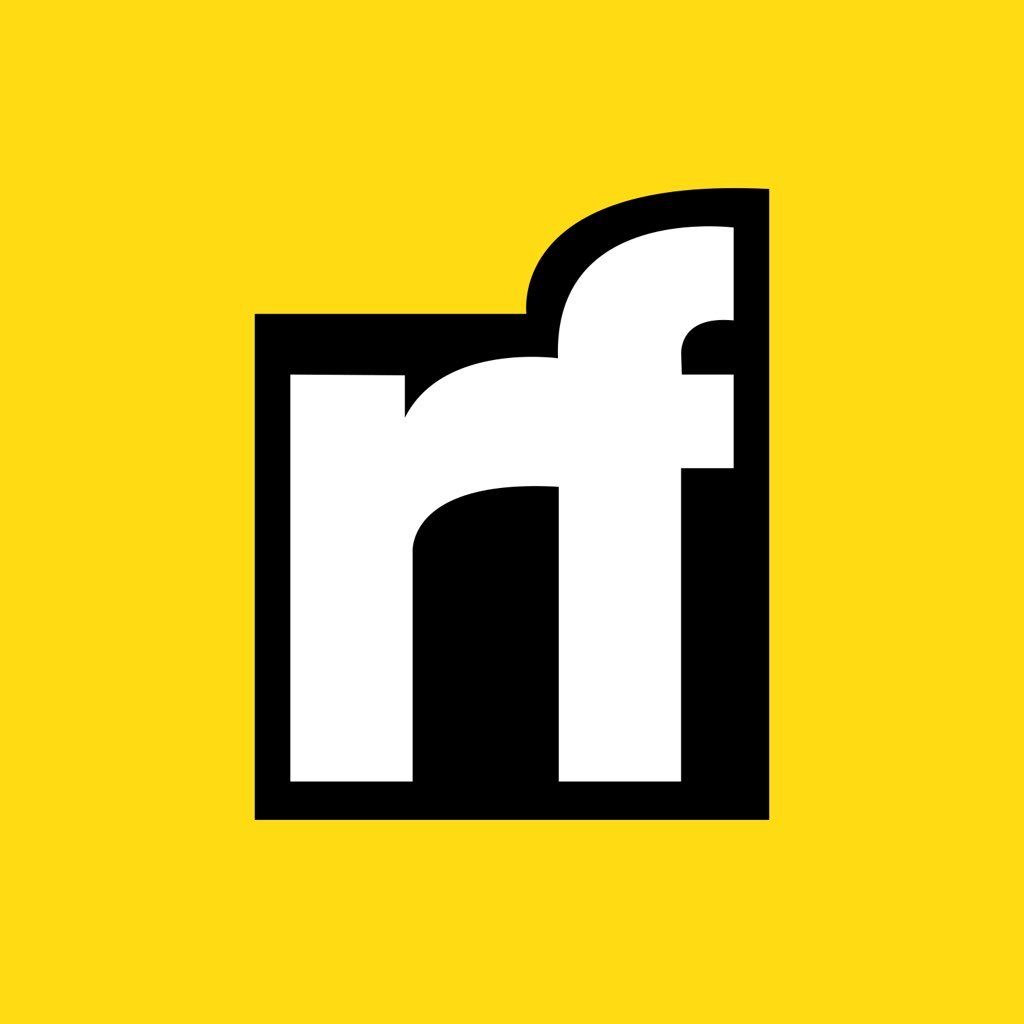
Pranav Bakare | Sciencx (2024-09-01T13:44:05+00:00) Immediately Invoked Function Expression (IIFE). Retrieved from https://www.scien.cx/2024/09/01/immediately-invoked-function-expression-iife/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.