This content originally appeared on Level Up Coding - Medium and was authored by Simuratli
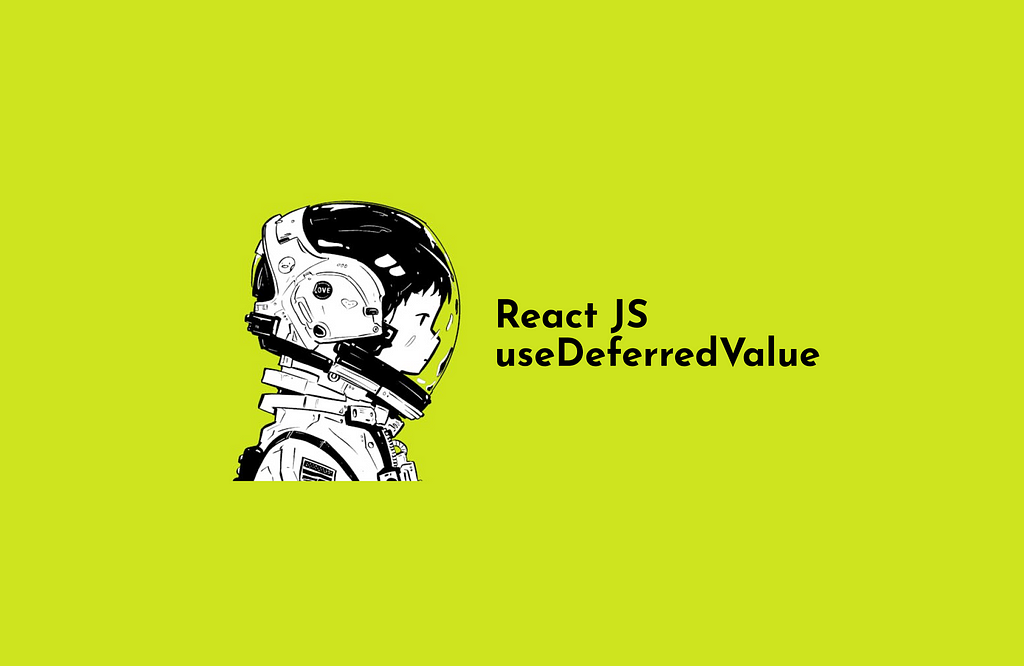
The useDeferredValuehook, introduced with React 18. It is a powerful tool you can use to provide better performance and smoother user experiences in your React applications.
What is useDeferredValue?
The useDeferredValue hook allows us to postpone updating a certain part of the UI for some time while the page continues to load.
How can we use it?
const deferredValue = useDeferredValue(value)
1. Example
This example simply displays the current search query with a slight delay, simulating how useDeferredValue can help smooth out UI updates during fast typing.
import React, { useState, useDeferredValue } from "react";
function App() {
const [query, setQuery] = useState("");
const deferredQuery = useDeferredValue(query, { timeoutMs: 500 });
return (
<div>
<input
type="text"
value={query}
onChange={(e) => setQuery(e.target.value)}
placeholder="Search..."
/>
<SearchResults query={deferredQuery} />
</div>
);
}
function SearchResults({ query }) {
return <div>Searching for: {query}</div>;
}
export default App;
- query: The current search input from the user.
- deferredQuery: A delayed version of query that update after 500 milliseconds.
2. Example
When building a dynamic search feature on our website that handles a large amount of data, we can enhance the user experience by utilizing useDeferredValue. This React hook helps defer updating the displayed values until the user has finished typing, optimizing performance, and reducing unnecessary renders.
We have 2 components App.tsx and List.tsx.
App.tsx:
import { useState } from "react";
import List from "./List";
export default function App() {
const [inputValue, setInputValue] = useState("");
return (
<div className="App">
<input
onChange={(e) => {
setInputValue(e.target.value);
}}
value={inputValue}
type="text"
placeholder="type..."
/>
<List inputValue={inputValue} />
</div>
);
}
List.tsx:
import React, { useMemo } from "react";
const List = ({ inputValue }) => {
console.log(inputValue);
const LIST_SIZE = 10000;
const list = useMemo(() => {
const l = [];
for (let i = 0; i < LIST_SIZE; i++) {
l.push(<div key={i}>{inputValue}</div>);
}
return l;
}, [inputValue]);
return <div>{list}</div>;
};
export default List;
As you see in here when we type something. It repeats itself 10000 times and writes it to the screen but it affects performance.
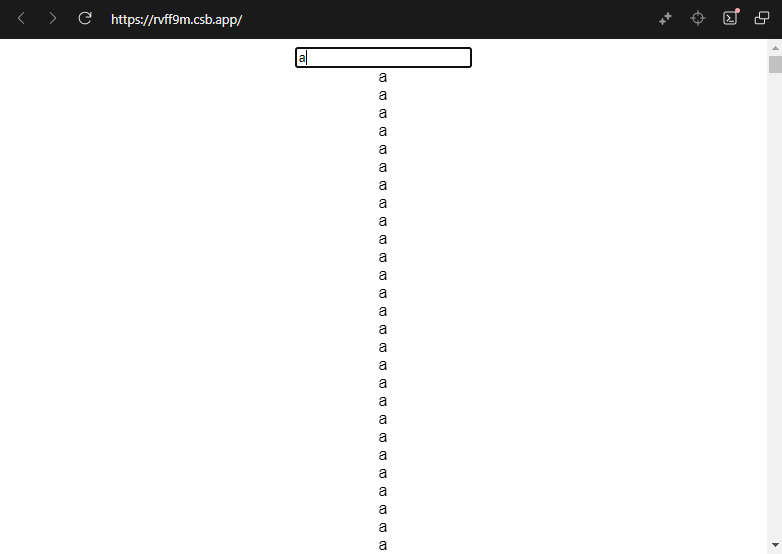
but we if use useDeferredValue it defers our list component and it helps use to fix performance issues.
import React, { useMemo, useDeferredValue } from "react";
const List = ({ inputValue }) => {
const deferredInput = useDeferredValue(inputValue);
const LIST_SIZE = 10000;
const list = useMemo(() => {
const l = [];
for (let i = 0; i < LIST_SIZE; i++) {
l.push(<div key={i}>{deferredInput}</div>);
}
return l;
}, [deferredInput]);
return <div>{list}</div>;
};
export default List;
You can test by yourself in Code Sandbox.
Thanks For reading…
Please connect with me on LinkedIn.
Enhancing User Experience in React with useDeferredValue: A Practical Guide was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Simuratli
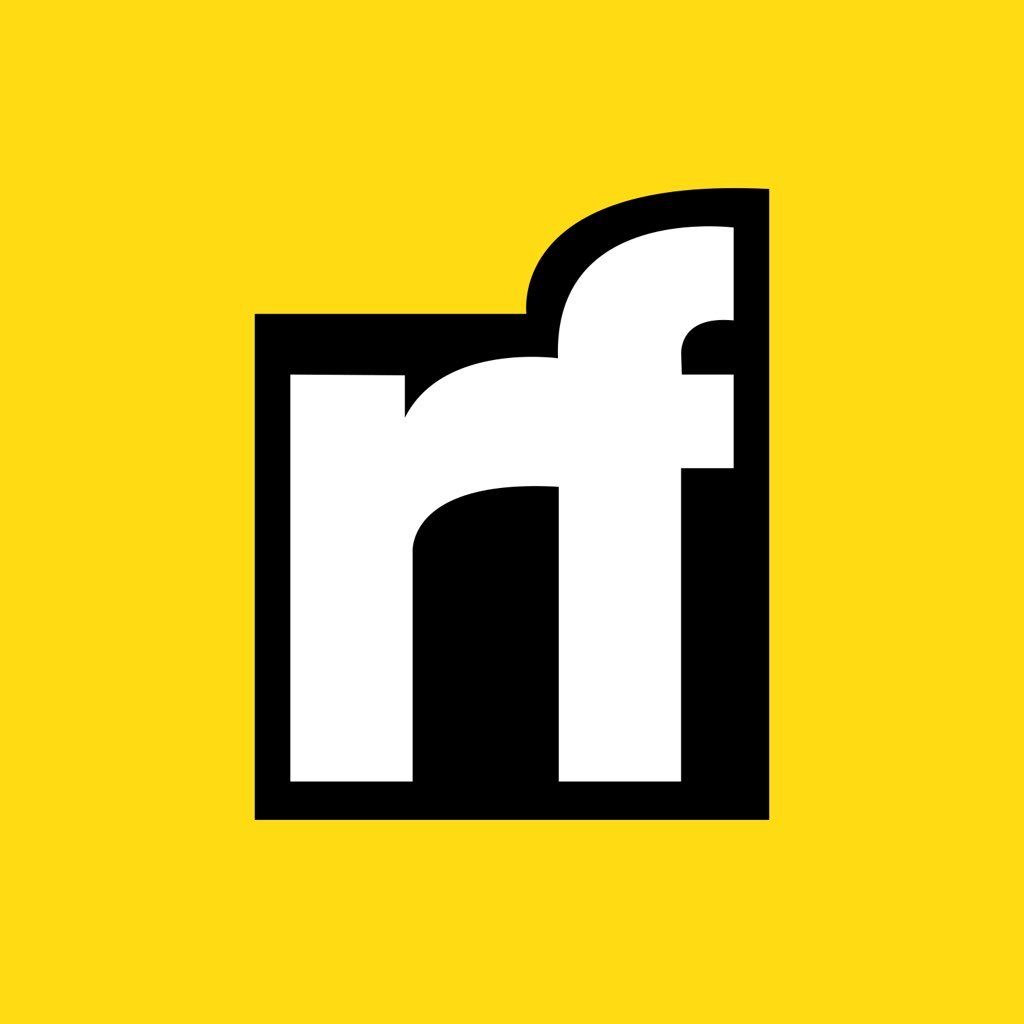
Simuratli | Sciencx (2024-09-05T20:26:24+00:00) Enhancing User Experience in React with useDeferredValue: A Practical Guide. Retrieved from https://www.scien.cx/2024/09/05/enhancing-user-experience-in-react-with-usedeferredvalue-a-practical-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.