This content originally appeared on DEV Community and was authored by TechEazy Consulting
In today’s fast-paced world of software development, building scalable web applications is crucial. With Spring Boot and Spring Data JPA, you can create robust REST APIs and manage data with ease. This blog will walk you through the essential concepts, annotations, and strategies for efficient web application development. Learn how to handle HTTP requests, responses, and database operations with minimal boilerplate code, all while following a real-world example. Check out our detailed video tutorial on our YouTube channel TechEazy Consulting and register for our full course at TechEazy Consulting.
In the modern software landscape, RESTful APIs have become a standard for building web applications that scale well and serve both web and mobile clients. With Spring Boot’s rapid development capabilities and Spring Data JPA’s seamless data management, you can quickly build a backend that powers your entire system. In this post, we’ll cover the key elements of Spring Boot REST API development and database management using Spring Data JPA, with code samples to get you started.
Why Spring Boot for REST APIs?
Spring Boot simplifies the process of developing REST APIs by providing key annotations and utilities that abstract much of the repetitive code required for handling HTTP requests and managing dependencies.
Key Spring Boot Annotations for REST API Development:
@RestController: This annotation designates a class as a REST controller, capable of handling web requests and returning responses directly in JSON or XML format.
@RequestMapping: Maps HTTP requests to specific methods within a controller.
@GetMapping, @PostMapping, @PutMapping, @DeleteMapping: These annotations are shorthand for mapping HTTP methods (GET, POST, PUT, DELETE) to Java methods within the controller.
These annotations form the backbone of any RESTful service built with Spring Boot.
Sample Code:
@RestController
@RequestMapping("/api/orders")
public class OrderController {
@GetMapping("/{id}")
public ResponseEntity<Order> getOrder(@PathVariable Long id) {
Order order = orderService.findById(id);
return new ResponseEntity<>(order, HttpStatus.OK);
}
@PostMapping
public ResponseEntity<String> createOrder(@RequestBody Order order) {
orderService.save(order);
return new ResponseEntity<>("Order created successfully!", HttpStatus.CREATED);
}
}
In this example, we have a REST controller that handles requests to get and create orders. By leveraging annotations like @GetMapping and @PostMapping, we map specific HTTP methods to Java methods, making our code clean and readable.
Handling HTTP Responses and Errors
In Spring Boot, handling HTTP responses and errors is straightforward, thanks to @ResponseBody and ResponseEntity.
@ResponseBody: Automatically serializes the return value of a method to JSON or XML and writes it to the HTTP response body.
ResponseEntity: Provides more flexibility, allowing you to customize HTTP status codes, headers, and response bodies.
Sample Code for Custom Response:
@PostMapping
public ResponseEntity<String> createOrder(@RequestBody Order order) {
orderService.save(order);
return new ResponseEntity<>("Order created successfully!", HttpStatus.CREATED);
}
This method handles POST requests to create new orders. The ResponseEntity allows us to return a custom success message along with the HTTP status code 201 Created, which indicates that the resource was successfully created.
Common HTTP Status Codes:
200 OK: Request was successful.
201 Created: Resource was successfully created.
400 Bad Request: The request was invalid or malformed.
404 Not Found: The requested resource could not be found.
500 Internal Server Error: An unexpected error occurred on the server.
Spring Data JPA: Simplifying Database Access
Spring Data JPA is a key module that abstracts much of the boilerplate code required for database operations. It allows developers to focus on writing business logic rather than worrying about data persistence.
Benefits of Spring Data JPA:
Repository Pattern: By extending the JpaRepository interface, you can perform CRUD operations without writing complex SQL queries.
Minimal Boilerplate Code: Common database interactions, such as saving and retrieving data, are handled automatically.
Entity Mapping: Java objects are mapped to database tables using JPA annotations like @Entity and @id.
Sample Code for Repository and Entity Setup:
@Entity
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String status;
// Getters and Setters
}
public interface OrderRepository extends JpaRepository<Order, Long> {}
In this example, Order is an entity that represents a table in the database, and OrderRepository extends JpaRepository to handle CRUD operations. Spring Data JPA abstracts the complexity, allowing you to interact with your database using simple method calls like save(), findById(), and deleteById().
Real-World Use Case: Last Mile Delivery System
To demonstrate the power of Spring Boot and Spring Data JPA, let’s walk through a real-world example—a Last Mile Delivery System. This system processes orders containing parcel information, groups parcels by location and dimension for efficient logistics planning, and provides role-based access for clients, drivers, and supervisors.
Key features of the system:
Client Access: Clients can upload order documents and track their parcels.
Driver Portal: Drivers can log in to view their delivery status and track drive time.
Supervisor Dashboard: Supervisors can see all orders and manage deliveries.
Automated Notifications: SMS and email updates are sent to customers to track their orders.
This use case demonstrates how Spring Boot and Spring Data JPA can be used to build complex, role-based applications with minimal code.
Next Steps
Ready to build your own scalable, efficient web applications using Spring Boot and Spring Data JPA? Visit our YouTube channel TechEazy Consulting for a detailed video tutorial that walks you through these concepts. For more code samples, hands-on exercises, and best practices, check out our Full Blog.
If you’re interested in diving deeper into Spring Boot and mastering web development, register for our full course at TechEazy Consulting and start your journey today!
This content originally appeared on DEV Community and was authored by TechEazy Consulting
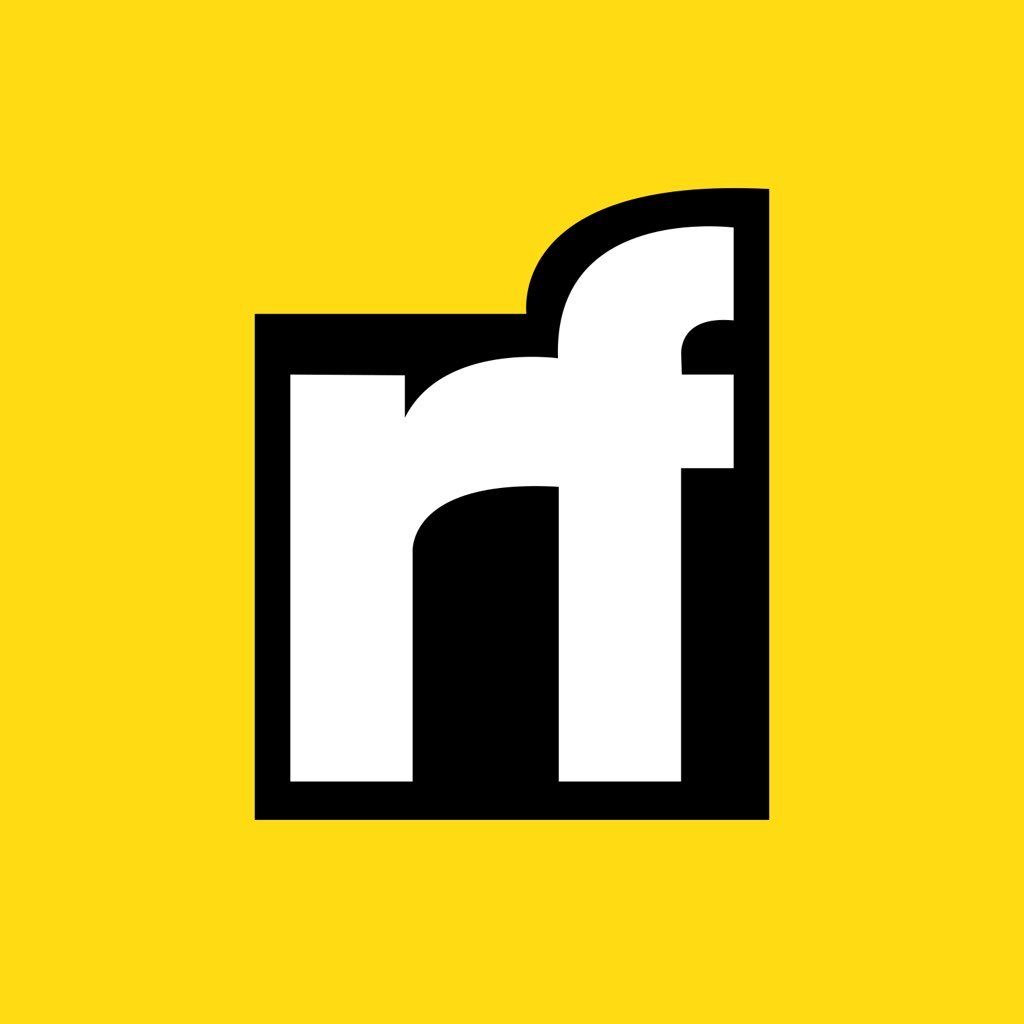
TechEazy Consulting | Sciencx (2024-09-10T17:27:00+00:00) Master REST API Development and Streamline Data Access with Spring Boot and Spring Data JPA. Retrieved from https://www.scien.cx/2024/09/10/master-rest-api-development-and-streamline-data-access-with-spring-boot-and-spring-data-jpa/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.