This content originally appeared on DEV Community and was authored by Alessandro Maclaine
Effect-TS provides several powerful ways to combine optional values, or Option
s, in a functional programming context. Whether you want to pair multiple Option
s together or apply functions inside Option
s to other values, the library offers several methods to simplify these operations. In this article, we'll explore four key functions for combining Option
s: O.product
, O.productMany
, O.all
, and O.ap
.
Example 1: Combining Two Options into a Tuple with O.product
Concept
The O.product
function allows you to combine two Option
s into a tuple. If both Option
s are Some
, it returns an Option
containing the tuple of both values. If either Option
is None
, it returns None
.
Code
function combining_ex01() {
const some1 = O.some(1); // Create an Option containing the value 1
const some2 = O.some(2); // Create an Option containing the value 2
const none = O.none(); // Create an Option representing no value
console.log(O.product(some1, some2)); // Output: Some([1, 2]) (combines both values into a tuple)
console.log(O.product(some1, none)); // Output: None (since the second Option is None)
console.log(O.product(none, some2)); // Output: None (since the first Option is None)
}
Explanation
-
O.product(some1, some2)
: Bothsome1
andsome2
areSome
, so the function returnsSome([1, 2])
, a tuple containing both values. -
O.product(some1, none)
: Since the secondOption
isNone
, the function returnsNone
. -
O.product(none, some2)
: Since the firstOption
isNone
, the function returnsNone
.
This function is useful when you need to combine the values of two Option
s into a pair, but you still want to ensure both values exist before proceeding.
Example 2: Combining Multiple Options into a Tuple with O.productMany
Concept
The O.productMany
function allows you to combine one Option
with multiple Option
s, producing a tuple if all Option
s are Some
. If any of the Option
s is None
, the function returns None
.
Code
function combining_ex02() {
const some1 = O.some(1); // Create an Option containing the value 1
const some2 = O.some(2); // Create an Option containing the value 2
const some3 = O.some(3); // Create an Option containing the value 3
const none = O.none(); // Create an Option representing no value
console.log(O.productMany(some1, [some2, some3])); // Output: Some([1, 2, 3]) (combines all values into a tuple)
console.log(O.productMany(some1, [none, some3])); // Output: None (since one of the Options is None)
}
Explanation
-
O.productMany(some1, [some2, some3])
: All theOption
s areSome
, so the function returnsSome([1, 2, 3])
, combining all values into a tuple. -
O.productMany(some1, [none, some3])
: Since one of theOption
s isNone
, the function returnsNone
.
This function is useful when you need to combine multiple Option
s into a single tuple but want to ensure that all values are present before proceeding.
Example 3: Combining a Structure of Options with O.all
Concept
The O.all
function combines multiple Option
s from an array or an object into a single Option
. If all Option
s are Some
, it returns a new Option
containing the combined structure. If any Option
is None
, it returns None
.
Code
function combining_ex03() {
const optionsArray = [O.some(1), O.some(2), O.some(3)]; // Create an array of Options
const optionsArrayWithNone = [O.some(1), O.none(), O.some(3)]; // Create an array of Options with a None
const optionsObject = { a: O.some(1), b: O.some(2) }; // Create an object of Options
const optionsObjectWithNone = { a: O.some(1), b: O.none() }; // Create an object of Options with a None
console.log(O.all(optionsArray)); // Output: Some([1, 2, 3]) (combines all array values)
console.log(O.all(optionsArrayWithNone)); // Output: None (since one of the array Options is None)
console.log(O.all(optionsObject)); // Output: Some({ a: 1, b: 2 }) (combines all object values)
console.log(O.all(optionsObjectWithNone)); // Output: None (since one of the object Options is None)
}
Explanation
-
O.all(optionsArray)
: All theOption
s in the array areSome
, so the function returnsSome([1, 2, 3])
, combining all array values. -
O.all(optionsArrayWithNone)
: One of theOption
s in the array isNone
, so the function returnsNone
. -
O.all(optionsObject)
: All theOption
s in the object areSome
, so the function returnsSome({ a: 1, b: 2 })
, combining all object values. -
O.all(optionsObjectWithNone)
: One of theOption
s in the object isNone
, so the function returnsNone
.
This function is useful when dealing with multiple Option
s in a structure, and you want to ensure all values are present before combining them.
Example 4: Applying a Function in an Option with O.ap
Concept
The O.ap
function allows you to apply a function contained in one Option
to a value contained in another Option
. If both Option
s are Some
, it returns an Option
containing the result of applying the function. If either Option
is None
, it returns None
.
Code
function combining_ex04() {
const someFn = O.some((n: number) => n * 2); // Create an Option containing a function
const someValue = O.some(3); // Create an Option containing the value 3
const none = O.none(); // Create an Option representing no value
console.log(pipe(someFn, O.ap(someValue))); // Output: Some(6) (applies the function to the value)
console.log(pipe(someFn, O.ap(none))); // Output: None (since the value Option is None)
console.log(pipe(none, O.ap(someValue))); // Output: None (since the function Option is None)
}
Explanation
-
pipe(someFn, O.ap(someValue))
: BothOption
s areSome
, so the function is applied to the value, resulting inSome(6)
. -
pipe(someFn, O.ap(none))
: Since the valueOption
isNone
, the function returnsNone
. -
pipe(none, O.ap(someValue))
: Since the functionOption
isNone
, the result isNone
.
This function is useful when you need to apply a function wrapped in an Option
to a value also wrapped in an Option
, ensuring both exist before performing the operation.
Conclusion
Combining Option
s in Effect-TS allows for robust handling of optional values in a functional style. Whether you're creating tuples with O.product
, combining multiple Option
s with O.productMany
, merging structures with O.all
, or applying functions with O.ap
, these techniques ensure your operations are safe and predictable. By leveraging these methods, you can simplify your code while maintaining safety around missing values, making your logic more concise and reliable.
This content originally appeared on DEV Community and was authored by Alessandro Maclaine
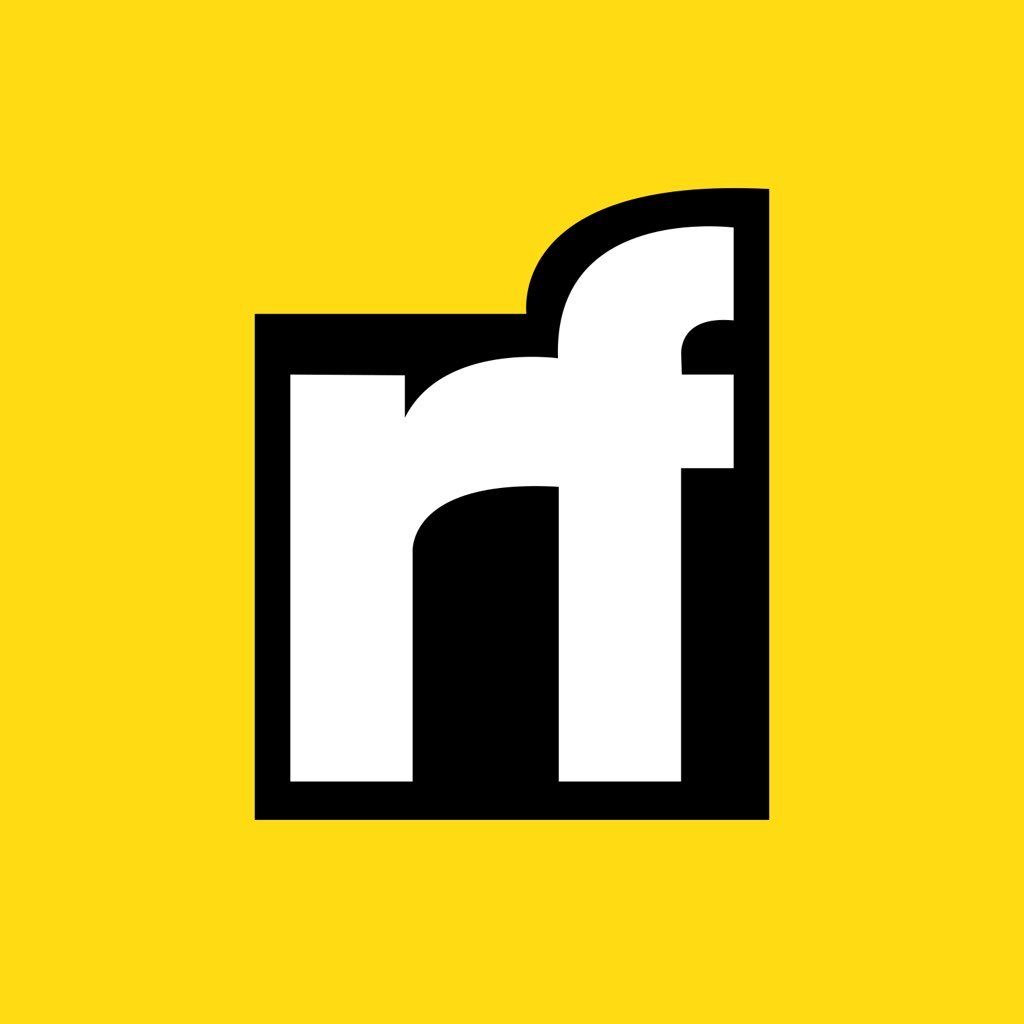
Alessandro Maclaine | Sciencx (2024-09-11T16:53:19+00:00) Combining Options in Effect-TS: A Practical Guide. Retrieved from https://www.scien.cx/2024/09/11/combining-options-in-effect-ts-a-practical-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.