This content originally appeared on Level Up Coding - Medium and was authored by Joctan
Get ahead in Java programming by understanding the key distinctions that every developer must master.
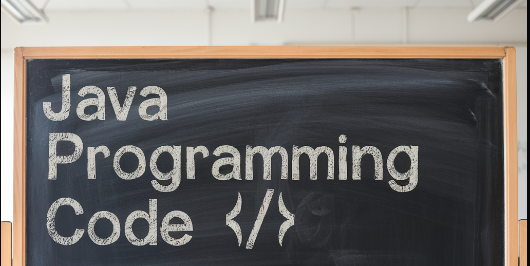
They are not the same!? Yes they aren’t the same. In the Java programming, there’s a critical distinction that can make or break your code: the difference between parameters and arguments. Though often used interchangeably, these terms have unique roles that can significantly impact how effectively you use methods. You’d know the difference between them to write cleaner, and more efficient Java code. Keep reading this article to unravel these essential concepts and discover how mastering them can elevate your programming prowess with practical, easy-to-follow examples.
What are Parameters?
Parameters are the variables defined in a method’s declaration. They act as placeholders for the values that will be passed to the method when it is called. Parameters specify the type and number of inputs that the method can accept.
Key Characteristics of Parameters:
- Defined in Method Declaration: Parameters are declared within parentheses in the method signature.
- Variable Scope: Parameters are local to the method in which they are declared. They can be used within the method but are not accessible outside of it.
- Data Types: Each parameter has a defined data type, which determines what kind of value can be passed to it.
- Multiple Parameters: A method can have multiple parameters, separated by commas.
Example of Parameters
Here’s an example of a method that uses parameters:
public class ParameterExample {
// Method to add two numbers
public int add(int a, int b) { // int a and int b are parameters
return a + b; // Returns the sum of a and b
}
public static void main(String[] args) {
ParameterExample example = new ParameterExample();
// Calling the add method
int sum = example.add(5, 10); // 5 and 10 are arguments
System.out.println("Sum: " + sum); // Output: Sum: 15
}
}
In this example, int a and int b are parameters of the add method. They define the method’s input, which allows it to accept two integer values when called.
What are Arguments?
Arguments are the actual values or data that are passed to a method when it is invoked. They correspond to the parameters defined in the method. In simple terms, arguments are the specific values supplied to the method during a call. Think about how to you assign a value to a variable.
Key Characteristics of Arguments:
- Values Passed to Methods: Arguments are the specific values that you pass to a method’s parameters when calling it.
- Matching Data Types: The type of the argument must match the type of the parameter it is being passed to. If they do not match, a compilation error occurs.
- Multiple Arguments: You can pass multiple arguments to a method, corresponding to each of the parameters defined.
- Variable Arguments (Varargs): Methods can accept a variable number of arguments using the varargs syntax.
Example of Arguments
Here’s an example that demonstrates arguments:
public class ArgumentExample {
// Method to calculate the product of two numbers
public int multiply(int x, int y) { // int x and int y are parameters
return x * y; // Returns the product of x and y
}
public static void main(String[] args) {
ArgumentExample example = new ArgumentExample();
// Calling the multiply method with arguments
int product = example.multiply(4, 5); // 4 and 5 are arguments
System.out.println("Product: " + product); // Output: Product: 20
}
}
In this example, when we call the multiply method, the values 4 and 5 are passed as arguments to the parameters int x and int y, respectively.
Example Demonstrating Both
Let’s look at a more comprehensive example that incorporates both parameters and arguments:
public class Person {
private String name;
private int age;
// Constructor with parameters
public Person(String name, int age) {
this.name = name; // Parameters assigned to instance variables
this.age = age;
}
// Method to display person details
public void displayInfo() {
System.out.println("Name: " + name + ", Age: " + age);
}
public static void main(String[] args) {
// Creating a Person object and passing arguments to the constructor
Person person1 = new Person("Alice", 30); // "Alice" and 30 are arguments
person1.displayInfo(); // Output: Name: Alice, Age: 30
// Creating another Person object
Person person2 = new Person("Bob", 25); // "Bob" and 25 are arguments
person2.displayInfo(); // Output: Name: Bob, Age: 25
}
}
In this example:
- The Person class has a constructor with parameters String name and int age.
- When creating a new Person object, the actual values "Alice" and 30 (as well as "Bob" and 25 for the second object) are passed as arguments to the constructor.
Conclusion
Understanding the difference between parameters and arguments is crucial for effective method utilization in Java. Parameters define the inputs a method can accept, while arguments are the actual values provided when the method is called. This distinction allows for the creation of flexible and reusable code, ultimately making your Java applications more robust and maintainable. By mastering these concepts, you will be better equipped to write efficient and organized Java programs.
Happy reading :)
The Crucial Difference Between Parameters and Arguments In JavaYou Need to Know was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Joctan
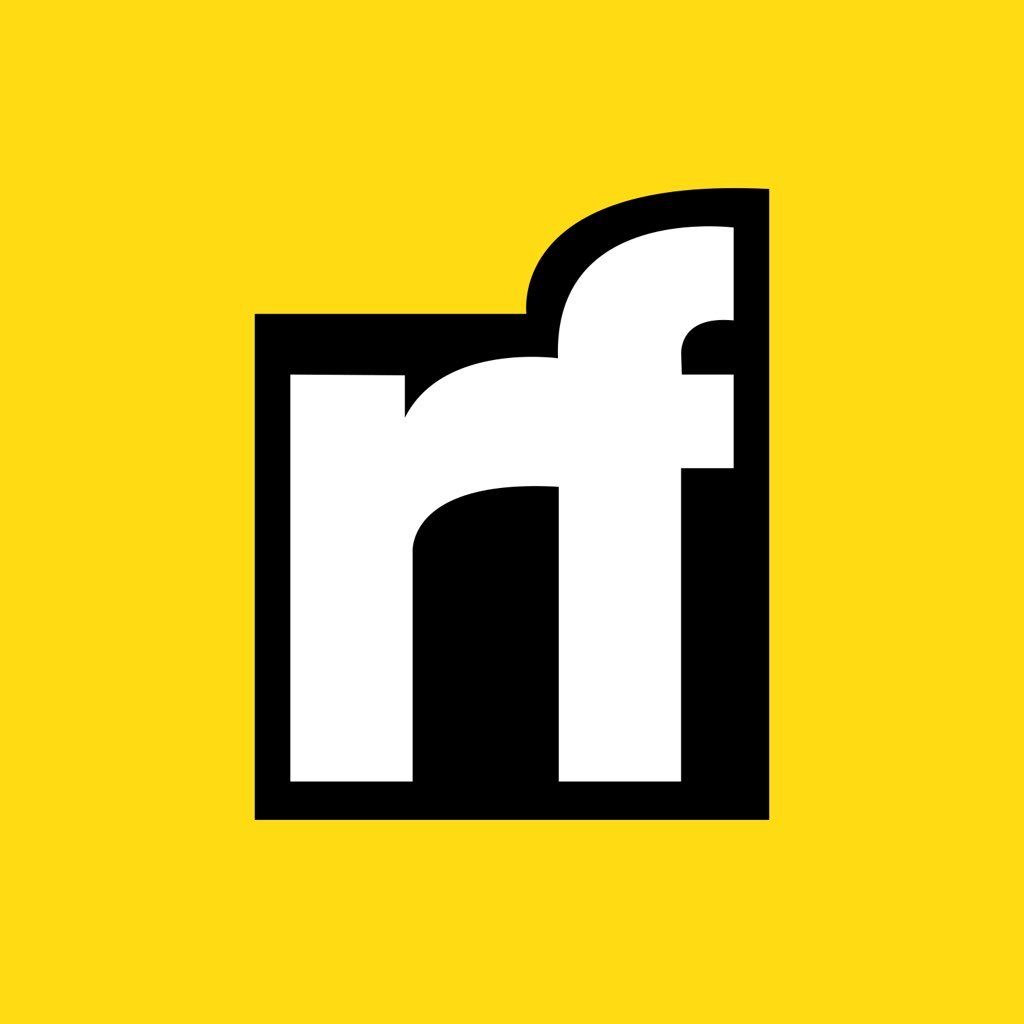
Joctan | Sciencx (2024-09-17T16:13:18+00:00) The Crucial Difference Between Parameters and Arguments In JavaYou Need to Know. Retrieved from https://www.scien.cx/2024/09/17/the-crucial-difference-between-parameters-and-arguments-in-javayou-need-to-know/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.