This content originally appeared on DEV Community and was authored by Info general Hazedawn
Creating a RESTful API is a fundamental skill for modern web developers, especially when working with the MERN stack (MongoDB, Express.js, React, and Node.js). In this blog post, weโll guide you through the process of setting up a basic API using Node.js and Express.js, connecting it to MongoDB, and performing CRUD (Create, Read, Update, Delete) operations. Letโs get started! ๐
*What is RESTful Architecture? *๐
REST (Representational State Transfer) is an architectural style that defines a set of constraints for creating web services. It uses standard HTTP methods like GET, POST, PUT, and DELETE to interact with resources. A RESTful API allows clients to perform operations on resources identified by URLs.
Key Principles of REST:
Stateless: Each request from the client must contain all the information needed to process the request.
Client-Server Separation: The client and server operate independently.
Cacheable: Responses must define themselves as cacheable or non-cacheable.
Step 1: Initialize Your Project
1. Create a New Directory:
bash
mkdir mern-api
cd mern-api
2.Initialize Node.js Project:
bash
npm init -y
3. Install Required Packages:
bash
npm install express mongoose body-parser cors
Step 2: Create Your Server
Create server.js File:
In your project root, create a file named server.js and add the following code:
javascript
const express = require('express');
const bodyParser = require('body-parser');
const cors = require('cors');
const mongoose = require('mongoose');
const app = express();
const PORT = process.env.PORT || 5000;
app.use(bodyParser.json());
app.use(cors());
// Connect to MongoDB
mongoose.connect('mongodb://localhost/mern-api', {
useNewUrlParser: true,
useUnifiedTopology: true,
})
.then(() => console.log("MongoDB connected"))
.catch(err => console.error(err));
app.get('/', (req, res) => {
res.send('Hello from MERN API!');
});
app.listen(PORT, () => {
console.log(Server is running on port ${PORT}
);
});
Step 3: Define Your Data Model with Mongoose ๐
Create a Model:
Create a folder named models and inside it create a file named Item.js:
javascript
const mongoose = require('mongoose');
const ItemSchema = new mongoose.Schema({
name: { type: String, required: true },
quantity: { type: Number, required: true },
description: { type: String },
});
module.exports = mongoose.model('Item', ItemSchema);
Implementing CRUD Operations ๐
Step 4: Set Up Routes for CRUD Operations
1. Create Routes:
Create a folder named routes and inside it create a file named itemRoutes.js:
javascript
const express = require('express');
const Item = require('../models/Item');
const router = express.Router();
// Create an item
router.post('/', async (req, res) => {
const newItem = new Item(req.body);
try {
await newItem.save();
res.status(201).json(newItem);
} catch (error) {
res.status(400).json({ message: error.message });
}
});
// Get all items
router.get('/', async (req, res) => {
try {
const items = await Item.find();
res.json(items);
} catch (error) {
res.status(500).json({ message: error.message });
}
});
// Update an item
router.put('/:id', async (req, res) => {
try {
const updatedItem = await Item.findByIdAndUpdate(req.params.id, req.body, { new: true });
res.json(updatedItem);
} catch (error) {
res.status(400).json({ message: error.message });
}
});
// Delete an item
router.delete('/:id', async (req, res) => {
try {
await Item.findByIdAndDelete(req.params.id);
res.json({ message: 'Item deleted' });
} catch (error) {
res.status(500).json({ message: error.message });
}
});
module.exports = router;
2. Integrate Routes into Server:
In your server.js, import and use the routes:
javascript
const itemRoutes = require('./routes/itemRoutes');
app.use('/api/items', itemRoutes);
Testing Your API ๐งช
You can test your API using tools like Postman or cURL. Here are some example requests:
- Create an Item:
- POST to http://localhost:5000/api/items
- Body:
json
{
"name": "Apple",
"quantity": 10,
"description": "Fresh apples"
}
Get All Items:
Update an Item:
Body:
json
{
"quantity": 20
}Delete an Item:
****DELETE to http://localhost:5000/api/items/{id}
Conclusion ๐
Congratulations! You have successfully built a basic RESTful API using Node.js and Express.js. Connecting to MongoDB and implementing CRUD operations gives you a solid foundation for developing more complex applications.
The MERN stack empowers you to create full-stack applications efficiently using JavaScript across both the client and server sides. Explore and enhance your API with features like authentication or advanced query handling!
NodeJS #ExpressJS #RESTAPI #MERNStack #WebDevelopment #JavaScript #MongoDB #API #CodingJourney
This content originally appeared on DEV Community and was authored by Info general Hazedawn
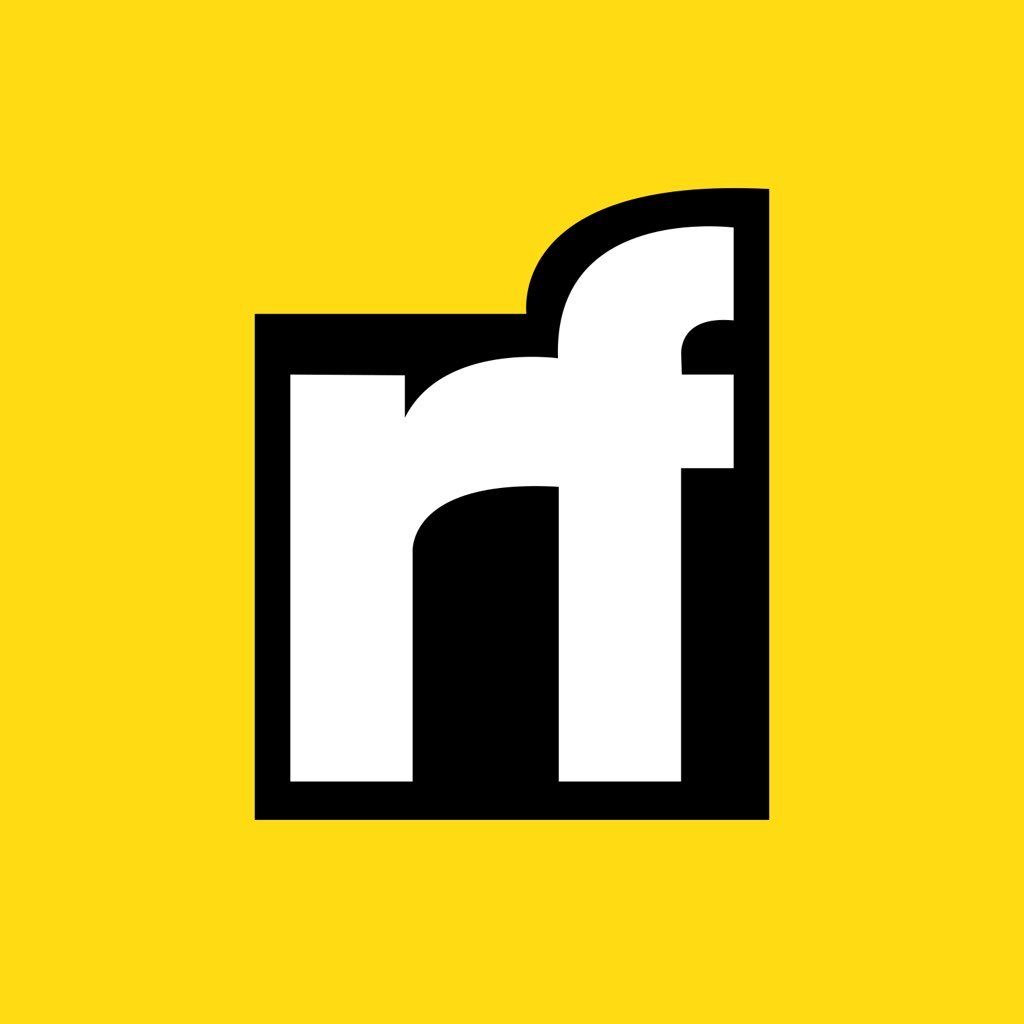
Info general Hazedawn | Sciencx (2024-09-24T06:06:00+00:00) Building a RESTful API with Node.js and Express.js: A Step-by-Step Guide ๐. Retrieved from https://www.scien.cx/2024/09/24/building-a-restful-api-with-node-js-and-express-js-a-step-by-step-guide-%f0%9f%8c%90/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.