This content originally appeared on Bits and Pieces - Medium and was authored by Eden Ella
Build your UI, data, logic, and infrastructure with small, reusable components
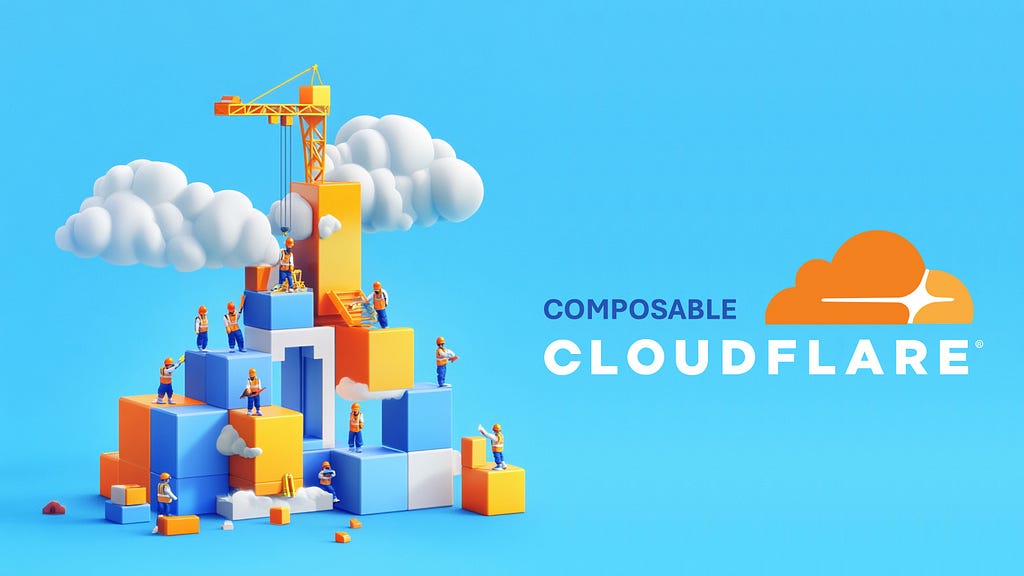
Composable architecture is a software design approach that emphasizes the separation of concerns and the composition of small, reusable building blocks to create complex systems. This approach is particularly well-suited for cloud-native applications, where scalability, reliability, and flexibility are key requirements.
While Cloudflare provides a wide range of services and tools for the composition of cloud-native applications, our codebase is often designed in a monolithic way that does not allow us to reuse and re-compose services efficiently.
In this guide, we’ll explore how to design a composable architecture for Cloudflare applications using Bit and Bit Platform. Our goal is to create a set of reusable Cloudflare components that can be easily composed to build complex applications.
Each component will be a small, self-contained unit of functionality that can be shared and reused across different projects.
Components and App Components
Software built with Bit is composed of two types of components: components and app components.
‘Components’ or ‘Bit components’ are small, reusable building blocks that encapsulate a specific piece of functionality. They are, in essence, packages that can be maintained without the need for a repository or a complex development environment.
‘App components’ are Bit components that are not only published as standalone packages but are also deployed and, therefore, are accessible at runtime.
Cloudflare Workers, for example, can be deployed as app components.
Creating a Bit Workspace
To get started with Bit, we need to create a Bit workspace.
Head over to Bit Platform, create a free account, create a scope to manage your components, and then create a new workspace.
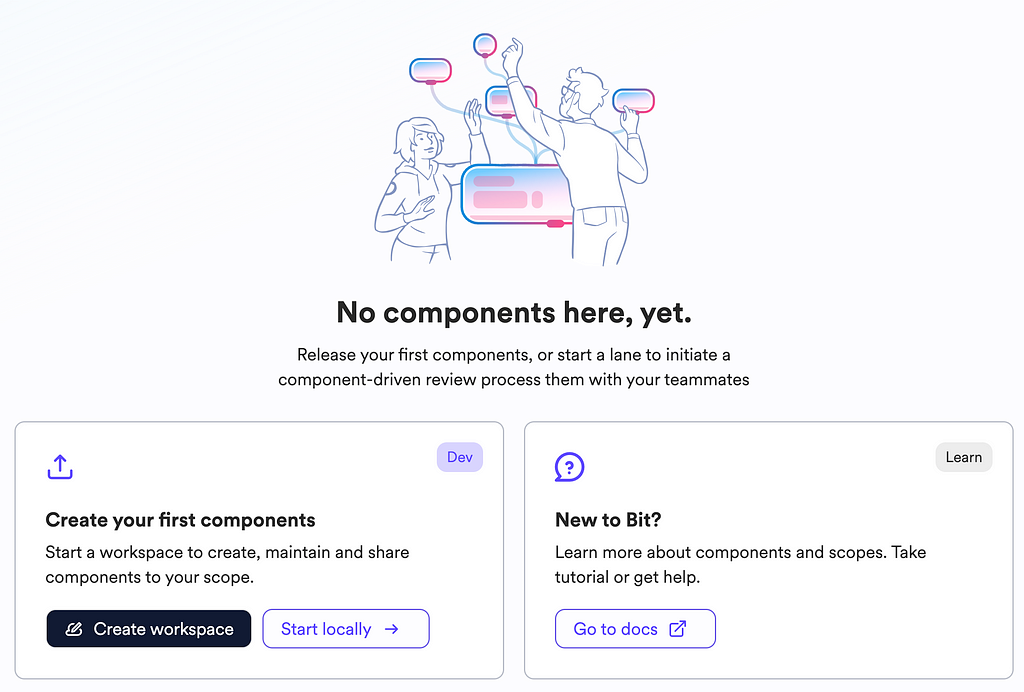
The Bit workspace will open in your browser, and you can start creating and managing components right away.
Note that this guide follows a completely cloud-based workflow, but you can also use Bit locally.
Creating a Cloudflare Worker Component
Let’s start by creating a Cloudflare Worker component using Bit. We’ll “fork” (or clone) an existing Cloudflare Worker component from Bit Platform and modify it to suit our needs.
Once the component is ready, we’ll publish it to Bit Platform and deploy it as a Cloudflare Worker. The Cloudflare Worker component will be available for reuse in other projects, saving us the need to rewrite the same functionality or configurations.
To fork a demo Cloudflare Worker component, run the following command:
bit fork backend.cloudflare/examples/cloudflare-worker
The forked Cloudflare Worker component will be added to your workspace, and you can start modifying it right away. It contains three files: the worker functionality ( cloudflare-worker.ts) , the worker configuration (cloudflare-worker.bit-app.ts), and the entry or main file for the worker component ( index.ts ).
The main file is used only when a component is consumed in build time, as a package.
The worker logs an environment variable and returns a basic HTTP response:
/**
* @filename cloudflare-worker.ts
* @componentId: backend.cloudflare/examples/cloudflare-worker
*/
import type {
Request as WorkerRequest,
ExecutionContext,
} from '@cloudflare/workers-types/experimental';
interface Env {
MESSAGE: string;
}
export default {
fetch(request: WorkerRequest, env: Env, ctx: ExecutionContext) {
console.log(env.MESSAGE);
return new Response('OK');
},
};
The configuration file with the `.bit-app.ts` extension which defines this component as an app component, contains the worker’s configuration:
/**
* @filename cloudflare-worker.bit-app.ts
* @componentId: backend.cloudflare/examples/cloudflare-worker
*/
import { WorkerApp } from '@backend/cloudflare.app-types.cloudflare-workers';
import {
CloudflareWorker,
type CloudflareWorkerOptions,
} from '@backend/cloudflare.deployers.cloudflare-workers';
const cloudflareOptions = {
accountId: 'dbd9f5dfb3d70b3002b8a5b8c45bf08a',
apiToken: process.env.CLOUDFLARE_TOKEN as string,
workerName: 'bit-worker-example',
mainFile: 'worker.js',
compatibility_date: '2024-09-02',
bindings: [
{
type: 'plain_text',
name: 'MESSAGE',
text: 'Hello, world!',
},
],
} satisfies CloudflareWorkerOptions;
export default WorkerApp.from({
name: 'cloudflare-worker',
mainPath: import.meta.resolve('./cloudflare-worker.js'),
deploy: CloudflareWorker.deploy(cloudflareOptions),
options: {
bindings: {
MESSAGE: 'Hello, world!',
},
},
});
Run Bit’s dev server to explore your components using the ‘Workspace UI’:
bit start
Running the Cloudflare Worker Component Locally
To list the app components in your workspace, run the following command:
bit app list
The output should include the Cloudflare Worker component we just forked:
┌─────────────────────────────────────────────────────┬───────────────────┐
│ id │ name │
├─────────────────────────────────────────────────────┼───────────────────┤
│ backend.cloudflare/examples/cloudflare-worker@0.1.1 │ cloudflare-worker │
└─────────────────────────────────────────────────────┴───────────────────┘
Use the app name to run the Cloudflare Worker component locally:
bit run cloudflare-worker
The worker will start running locally:
starting cloudflare-worker on http://localhost:3000
Hello, world!
Releasing and Deploying the Cloudflare Worker Component
To release the component, store in your scope on Bit Platform, and deploy it as a Cloudflare Worker, run the following command:
bit tag - message "Initial release"
The component will be built on Bit Platform, and deployed as a Cloudflare Worker.
Adding a logic component
You might want to separate the logic of your Cloudflare Worker into another component that can be used in multiple workers or other parts of your application.
In this case you can create a new NodeJS component and add the logic there.
For example, let’s create an error handling component that can be used in multiple Cloudflare Workers:
bit create module examples/error-handler
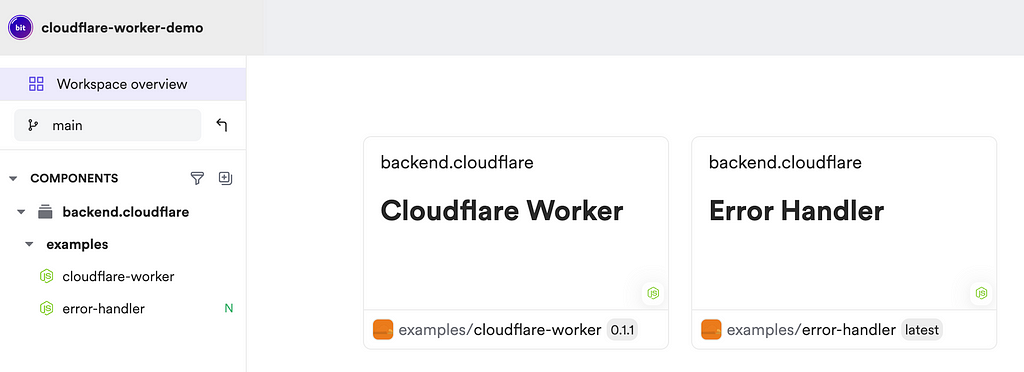
We can implement the logic in the error handler component:
/**
* @filename error-handler.ts
* @componentId: backend.cloudflare/examples/error-handler
*/
export class ErrorHandler extends Error {
// Implement error handling logic here
}
An use the error handler in the Cloudflare Worker component:
/**
* @filename cloudflare-worker.ts
* @componentId: backend.cloudflare/examples/cloudflare-worker
*/
import { ErrorHandler } from '@backend/cloudflare.examples.error-handler';
// ...
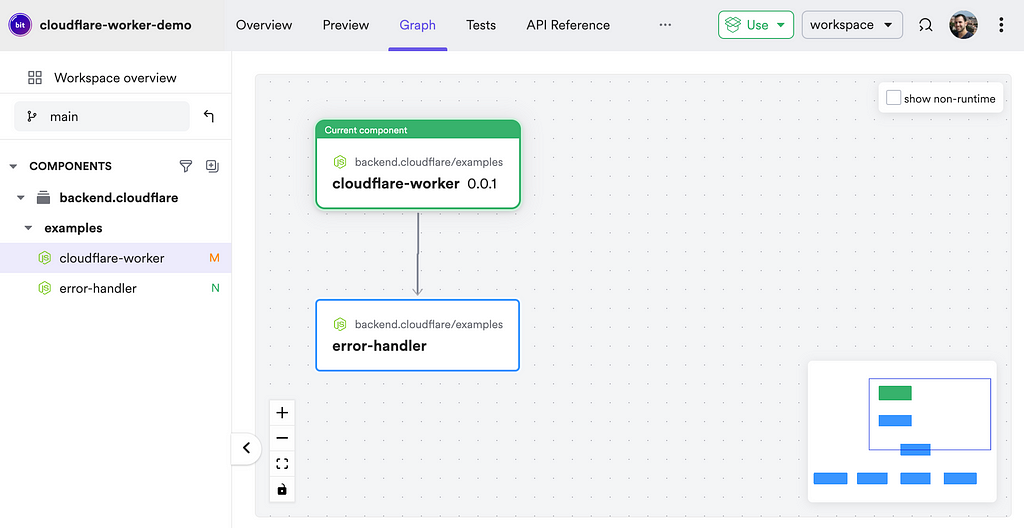
The release process is the same as before, you can tag the component with a new version:
bit tag - message "Add error handling"
Unlike the Cloudflare Worker component, the error handler component is not deployed as an app component. It is a regular component that is only published and can be used as a regular package (in build time) by other components.
However, since the the error handler component is now a dependency of the Cloudflare Worker component, its release will automatically trigger a new build and release of the Cloudflare Worker component.
Discussion
This guide provides a taste of how to design a composable architecture for Cloudflare applications using Bit.
A composable design with Bit means that everything in your codebase is a reusable component, including services, configurations, and even infrastructure. Logic, UI, data, and other concerns are separated into small components that can be used to accelerate development and improve maintainability.
To explore additional Cloudflare solutions implemented with Bit see the Cloudflare Bit Scope.
Learn More
- Moving Beyond IaC: Infrastructure into Components
- How to Publish NPM Packages: A Modern Approach
- Building Service-Oriented Platforms with Bit
Composable Cloudflare Architecture: A Guide was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Eden Ella
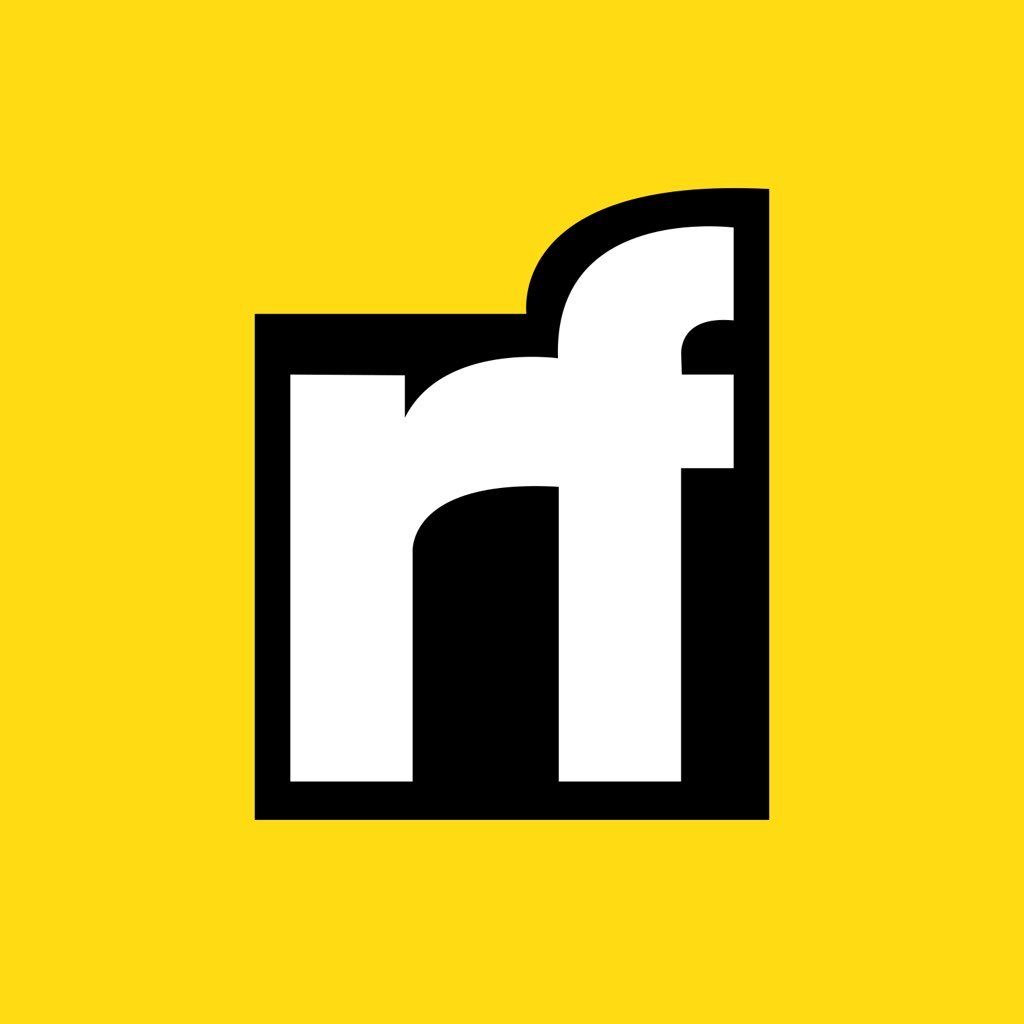
Eden Ella | Sciencx (2024-09-24T21:28:29+00:00) Composable Cloudflare Architecture: A Guide. Retrieved from https://www.scien.cx/2024/09/24/composable-cloudflare-architecture-a-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.