This content originally appeared on DEV Community and was authored by Md Yusuf
Integration testing with React Testing Library involves testing how various components of your application work together. Here's a basic guide to get you started:
Setting Up
- Install Dependencies: Ensure you have the necessary libraries installed. If you haven't done so already, install React Testing Library and Jest:
npm install --save-dev @testing-library/react @testing-library/jest-dom
-
Create a Test File:
Typically, you would create a test file alongside the component you're testing, following a naming convention like
ComponentName.test.js
.
Writing Integration Tests
Here’s a step-by-step example of how to write an integration test:
Example Scenario
Let's say you have a simple form component that takes a user’s name and displays it when submitted.
Form.js
:
import React, { useState } from 'react';
const Form = () => {
const [name, setName] = useState('');
const [submittedName, setSubmittedName] = useState('');
const handleSubmit = (e) => {
e.preventDefault();
setSubmittedName(name);
setName('');
};
return (
<div>
<form onSubmit={handleSubmit}>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
placeholder="Enter your name"
/>
<button type="submit">Submit</button>
</form>
{submittedName && <p>Hello, {submittedName}!</p>}
</div>
);
};
export default Form;
Writing the Test
Form.test.js
:
import React from 'react';
import { render, screen, fireEvent } from '@testing-library/react';
import Form from './Form';
describe('Form Component', () => {
test('displays the submitted name', () => {
render(<Form />);
// Input field and button
const input = screen.getByPlaceholderText(/enter your name/i);
const button = screen.getByRole('button', { name: /submit/i });
// Simulate user typing and submitting the form
fireEvent.change(input, { target: { value: 'John Doe' } });
fireEvent.click(button);
// Check if the submitted name is displayed
expect(screen.getByText(/hello, john doe!/i)).toBeInTheDocument();
});
});
Explanation of the Test
Render the Component: The
render
function mounts theForm
component in a virtual DOM.Select Elements: Use
screen
to query elements. Here, we select the input and button using accessible queries.Simulate User Actions: Use
fireEvent
to simulate typing in the input field and clicking the submit button.Assert Expected Behavior: Finally, check if the expected output (the greeting message) appears in the document.
Running the Tests
Run your tests using the following command:
npm test
Best Practices
- Focus on User Interactions: Write tests that simulate how users interact with your app, not just the implementation details.
- Keep Tests Isolated: Each test should focus on a specific functionality to ensure clarity and maintainability.
- Use Descriptive Names: Make your test descriptions clear to convey what is being tested.
By following this approach, you can effectively test how different parts of your React application work together, ensuring a smoother user experience. Let me know if you need further assistance!
This content originally appeared on DEV Community and was authored by Md Yusuf
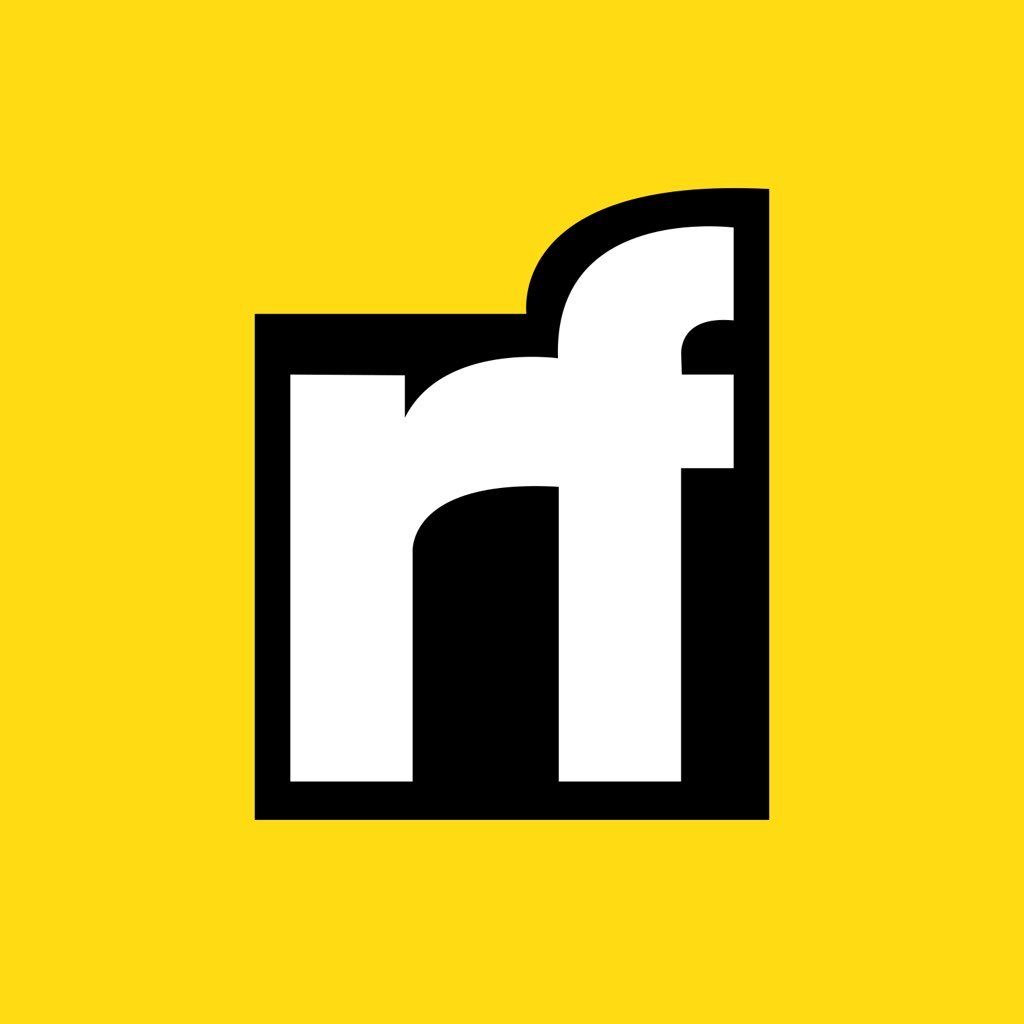
Md Yusuf | Sciencx (2024-09-30T04:38:21+00:00) Integration Testing in React: Best Practices with Testing Library. Retrieved from https://www.scien.cx/2024/09/30/integration-testing-in-react-best-practices-with-testing-library/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.