This content originally appeared on HackerNoon and was authored by chaooshi
\
If you’ve ever wondered why you write import './Button.css';
at the top of your JSX or TSX files (or even within other CSS files using @import
), here’s why.
\ When you import a CSS file in a React application like this:
import './Button.css';
\
you’re not importing JavaScript code or a module with exports. Instead, you’re instructing the build system (like Webpack or Vite) to include the styles defined in Button.css
into your application. Here’s how it works:
\ In a typical React setup, a bundler like Webpack is configured to handle various types of files, including CSS. When you import a CSS file, Webpack (or another bundler) processes this file and injects its styles into the DOM.
What Does “Processing� Mean?
When we say the bundler “processes� the CSS file, we mean that it reads the content of the file and converts it into a format that can be used by the browser. For instance:
Webpack:
Imagine you have the following CSS in Button.css
:
\
@import './base.css';
.button {
background-image: url('./button-background.png');
padding: 10px;
}
\
And in base.css
:
\
body {
margin: 0;
font-family: Arial, sans-serif;
}
\
Then you import Button.css
in a .jsx
file:
\
// MyComponent.jsx
import React from 'react';
import './Button.css';
function MyComponent() {
return <button className="button">Click Me</button>;
}
export default MyComponent;
\ Here’s how Webpack processes it:
@import
Resolution: The@import './base.css';
statement is resolved during the build process, and the contents ofbase.css
are inlined intoButton.css
.url()
Resolution: Theurl('./button-background.png')
path is resolved, potentially being transformed into a hashed file path (e.g.,button-background.abc123.png
) for better caching in production.
\ ==These two steps are handled by css-loader in Webpack.==
- Bundling: Webpack then bundles the CSS into a single file or injects it into the HTML. In production, the final output might look like this in the generated CSS file:
body {
margin: 0;
font-family: Arial, sans-serif;
}
.button {
background-image: url('button-background.abc123.png');
padding: 10px;
}
\
- Injection: This CSS is either included as a separate file (e.g.,
styles.css
) linked in the HTML:
<link rel="stylesheet" href="/assets/styles.css">
\
Or it may be injected directly into a <style>
tag within the <head>
of the HTML during development. This approach allows for faster development because the styles are dynamically updated without needing to reload the entire page.
Vite:
Vite processes the CSS similarly. During development, it injects the styles into the document’s <head>
tag. For production, Vite uses Rollup to bundle the CSS into a separate file. The final production HTML might include:
\
<link rel="stylesheet" href="/assets/style.css">
The contents of style.css
would be the bundled and optimized version of your CSS, ready to be deployed.
\
Global vs. Scoped Styles
The styles defined in Button.css
are applied globally across your application unless you use something like CSS Modules to scope them. If it's plain CSS, the styles are global and can affect any matching elements throughout your application. So, be cautious when naming your classes to avoid unintended styling conflicts across components.
\ By understanding this, you can better manage how and where your styles are applied, keeping your React application’s styling organized and effective.
Conclusion
Understanding how CSS is processed in React helps you manage styles effectively. Tools like Webpack and Vite bundle and link your styles, ensuring they’re applied as intended across your app. By knowing how global and scoped styles work, you can avoid styling conflicts and keep your app looking sharp.
This content originally appeared on HackerNoon and was authored by chaooshi
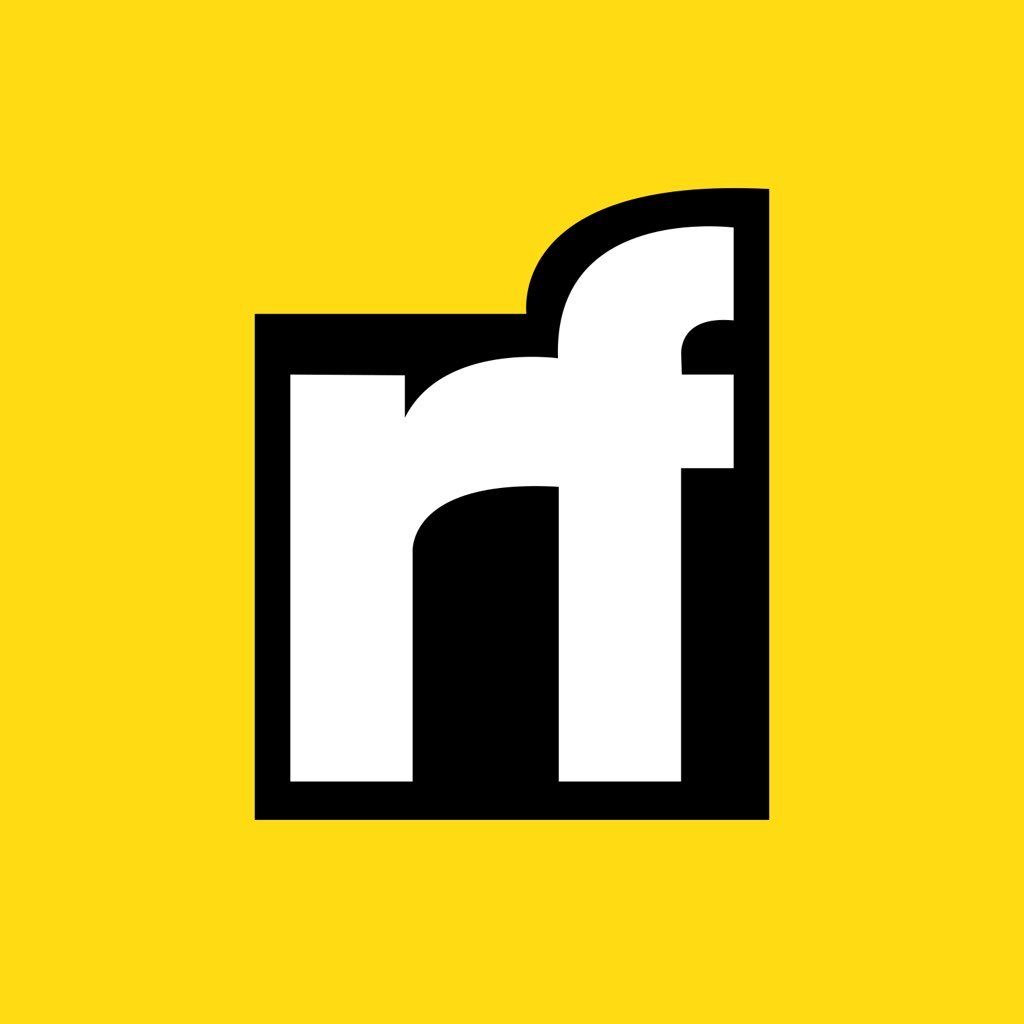
chaooshi | Sciencx (2024-10-08T08:43:39+00:00) Importing CSS into Your React File: But Hold On, What Is Exported?. Retrieved from https://www.scien.cx/2024/10/08/importing-css-into-your-react-file-but-hold-on-what-is-exported/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.