This content originally appeared on Telerik Blogs and was authored by Hassan Djirdeh
Coding style guides offer a standardized approach to writing code so that a team or organization maintains consistency and scalability in its codebase.
In the digital landscape, consistency is not just a principle for user interface design but can also be a fundamental requirement in coding practices. Coding style guides represent an essential framework within software development, particularly for frontend developers engaged with design systems. These guides offer a standardized approach to writing code, so a team or organization can maintain uniformity, readability and scalability in its codebase.
In this article, we explore the significance of coding style guides, some popular methodologies and how they contribute to a cohesive development environment.
What Are Coding Style Guides?
Coding style guides are comprehensive sets of rules and conventions that dictate how code should be written and organized. They serve as a blueprint for developers, enabling them to write code that not only functions efficiently but is also easy to read and maintain. This consistency is especially crucial in large teams where multiple developers collaborate on the same project, as it prevents discrepancies that can lead to errors or complicated merges in version control systems.
Coding style guides can cover various aspects of coding, including but not limited to:
- Naming conventions: Establishing rules for naming variables, functions, classes and other code elements, promoting clarity and consistency.
- Code formatting: Defining standards for indentation, whitespace usage, line breaks and other formatting rules to enhance readability.
- Code organization: Providing guidelines for structuring code files, directories and modules, improving maintainability and scalability.
- Commenting practices: Establishing conventions for writing clear and concise code comments, facilitating collaboration and knowledge sharing.
- Language-specific conventions: Addressing language-specific coding practices, such as bracket styles, variable declarations and control flow structures.
Why Are Coding Style Guides Important?
For design engineers, coding style guides are more than just rules; they can be a pivotal tool for translating design systems into functional code. A well-defined style guide helps keep the implementation of design tokens and systems consistent across various parts of an application, which is crucial for maintaining the integrity of the design throughout the development process.
In addition to streamlining collaboration among team members, a coding style guide lets all developers follow the same design principles, which is crucial when implementing UI components.
Consistency in code leads to consistency in user interfaces, making the application more intuitive and user-friendly. When every part of an application adheres to the same design guidelines, it creates a more seamless experience for users, reducing the learning curve and increasing user satisfaction.
Popular Coding Style Guides
While coding style guides can be tailored to the specific needs of an organization, there are several popular methodologies that have gained widespread adoption in the software development community. To illustrate how coding style guides can be applied and used, we’ll discuss a few below:
BEM
As an example, one popular coding style guide that many use when structuring CSS classes is the BEM methodology. BEM stands for Block, Element, Modifier and it’s a naming convention that helps developers create modular and reusable CSS classes.
Consider a scenario where CSS classes are named spontaneously without a clear system:
/* CSS without clear structure */
.card {}
.featured {}
.title {}
.description {}
.link {}
In the above simple example, the class names are generic and could easily overlap in style properties across different sections of a website. This can lead to styles being accidentally overridden and makes the CSS difficult to maintain, especially in larger projects.
Now, let’s contrast this with BEM methodology. Using BEM, class names are structured like the following:
.block {}
.block__element {}
.block--modifier {}
The block is the main component or container, the element is a part of the block and the modifier is a variation of the block or element. Each of these serves a clear purpose and reduces the risk of style conflicts.
Contrasting with the poorly structured example, BEM offers a clear and systematic way to name CSS classes, which helps in avoiding conflicts and enhances modularity:
/* BEM structure for the card component */
.card {}
.card__title {}
.card__description {}
.card__link {}
.card--featured {}
Here, each class has a specific purpose:
- Block:
.card
acts as the foundational class for the card component. - Element:
.card__title
,.card__description
and.card__link
are elements that belong to the.card
block, indicating their specific roles within the card component. - Modifier:
.card--featured
is a modifier that represents a different version of the.card
, specifically styling it as a featured card.
To show how these BEM classes are applied in HTML, consider the following example:
<div class="card card--featured">
<h2 class="card__title">Featured Product</h2>
<p class="card__description">
Lorem ipsum dolor sit amet, consectetur adipiscing elit.
</p>
<a href="#" class="card__link">Learn More</a>
</div>
This structure clearly separates the styling concerns, making the CSS easier to manage and extend.
For more details on BEM, be sure to check out the official BEM documentation.
Google HTML/CSS Style Guide
Coding style guides encompass more than just CSS organization; as mentioned earlier, they can also cover aspects like naming conventions, indentation, formatting, and commenting. For example, Google’s HTML/CSS Style Guide provides comprehensive guidelines on formatting and style rules for HTML and CSS. It covers best practices for accessibility, like having alternative content always be provided for multimedia:
<!-- Not recommended -->
<img src="spreadsheet.png" />
<!-- Recommended -->
<img src="spreadsheet.png" alt="Spreadsheet screenshot." />
<!-- Not recommended -->
<title>Test</title>
<article>
This is only a test.
<!-- Recommended -->
<!DOCTYPE html>
<meta charset="utf-8" />
<title>Test</title>
<article>This is only a test.</article>
</article>
And writing semantic HTML where possible:
<!-- Not recommended -->
<div onclick="goToRecommendations();">All recommendations</div>
<!-- Recommended -->
<a href="recommendations/">All recommendations</a>
For a more detailed list of guidelines, be sure to check out the Google HTML/CSS Style Guide documentation.
Airbnb JavaScript Style Guide
The Airbnb JavaScript Style Guide is another widely recognized coding standard that emphasizes clarity, predictability and organization in JavaScript coding practices. It advocates for modern JavaScript syntax and idiomatic expressions, promoting best practices that lead to clean and understandable code.
This style guide is particularly meticulous about variable and function naming, the use of arrow functions and structuring components in React applications. For example, it prefers constants over let
and var
for variables that do not change and provides certain guidelines when using components and props in React.
Here’s how the Airbnb JavaScript Style Guide would suggest structuring this piece of JavaScript code:
// Bad
function handleThing() {
return true;
}
// Good
const handleThing = () => true;
In React, as an example, the guide encourages the use of PascalCase for React component names and camelCase for instances of these components:
// bad
import reservationCard from "./ReservationCard";
// good
import ReservationCard from "./ReservationCard";
// bad
const ReservationItem = <ReservationCard />;
// good
const reservationItem = <ReservationCard />;
For a list of coding style guides for different programming languages, check out the GitHub repository awesome-guidelines by GitHub user Kristories.
Tailwind
When developing your own design system, your team can either create these guidelines from the ground up or adopt widely recognized frameworks and conventions from the broader development community.
One such popular framework is Tailwind, a utility-first CSS framework that has become a favorite in the development scene. Unlike traditional approaches like BEM, which focus on structured naming conventions, Tailwind provides a set of utility classes that can be applied directly to HTML elements to style them efficiently.
<div class="p-3 bg-white shadow rounded-lg">
<h3 class="text-xs border-b">font-mono</h3>
<p class="font-mono">The quick brown fox jumps over the lazy dog.</p>
</div>
Tailwind’s design philosophy aims to offer a flexible and customizable method for designing interfaces. Its utility-first approach reduces the amount of CSS developers need to write, fostering a more consistent and scalable design. Each class in Tailwind specifies a particular style or a set of CSS properties, for example:
- p-3 denotes
padding: 0.75rem
- bg-white denotes
background-color: white
- rounded-lg denotes
border-radius: 0.25rem
- And so forth
Wrap-up
In conclusion, coding style guides are essential tools in the realm of software development, especially for design engineers who bridge visual design and technical implementation. These guides provide a structured approach to coding that enhances collaboration, promotes consistency and elevates the quality of the final product.
Whether adopting established standards like Google’s HTML/CSS guidelines, BEM for CSS structuring or the Airbnb JavaScript Style Guide, or creating customized guidelines tailored to the specific needs of a project, the benefits are significant. For design engineers looking to enhance their team’s efficiency and output quality, integrating a comprehensive coding style guide can be important in achieving these goals.
This content originally appeared on Telerik Blogs and was authored by Hassan Djirdeh
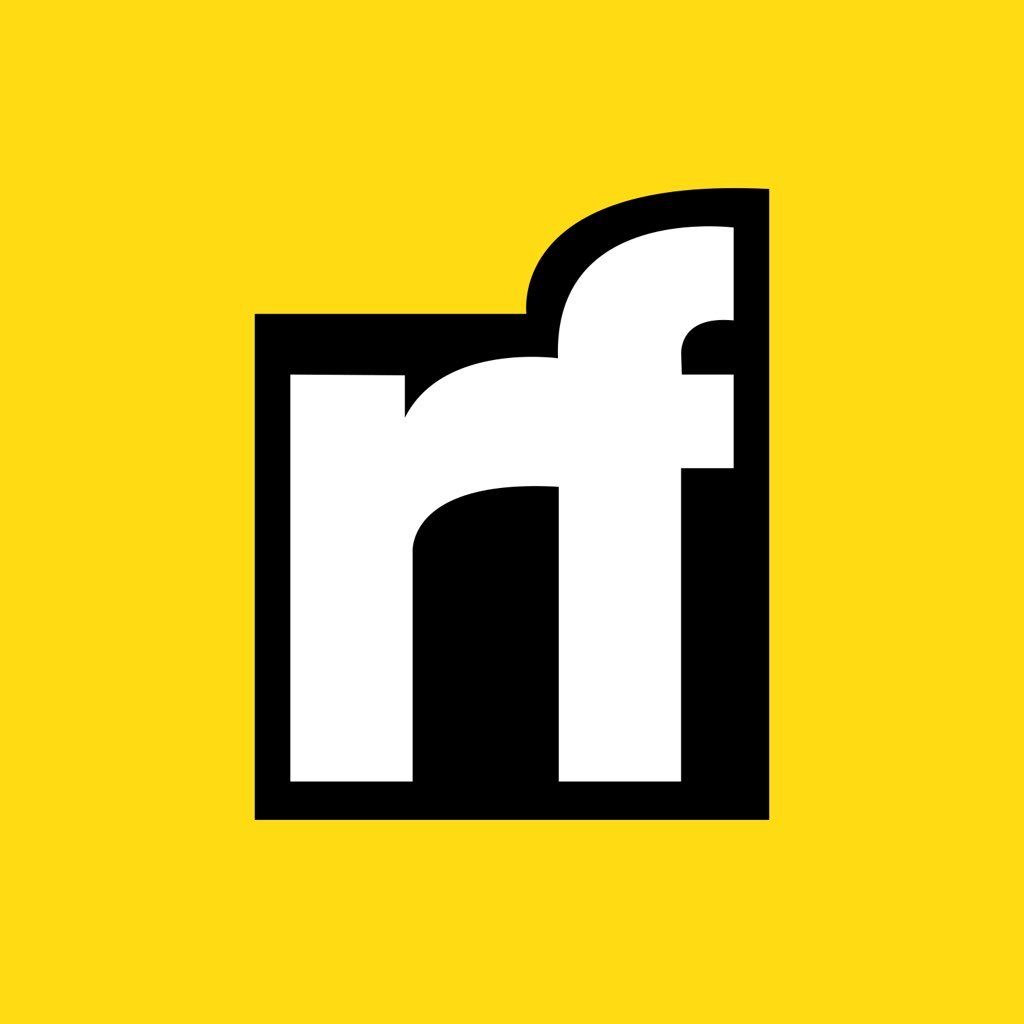
Hassan Djirdeh | Sciencx (2024-10-09T08:42:56+00:00) Coding Style Guides—A Standardized Approach to Writing Code. Retrieved from https://www.scien.cx/2024/10/09/coding-style-guides-a-standardized-approach-to-writing-code/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.