This content originally appeared on Level Up Coding - Medium and was authored by Pavel Pogosov
The Most Useful JavaScript Feature In 2024
In JavaScript development, especially when dealing with async tasks and error handling, we often rely on try-catch blocks and async-await. These work well but can make your code look bloated and harder to read.
To solve this, a new operator, ?=, has been proposed. It simplifies error handling and improves code readability.
In this article, I’ll explain how it works and why it can make a difference for developers.
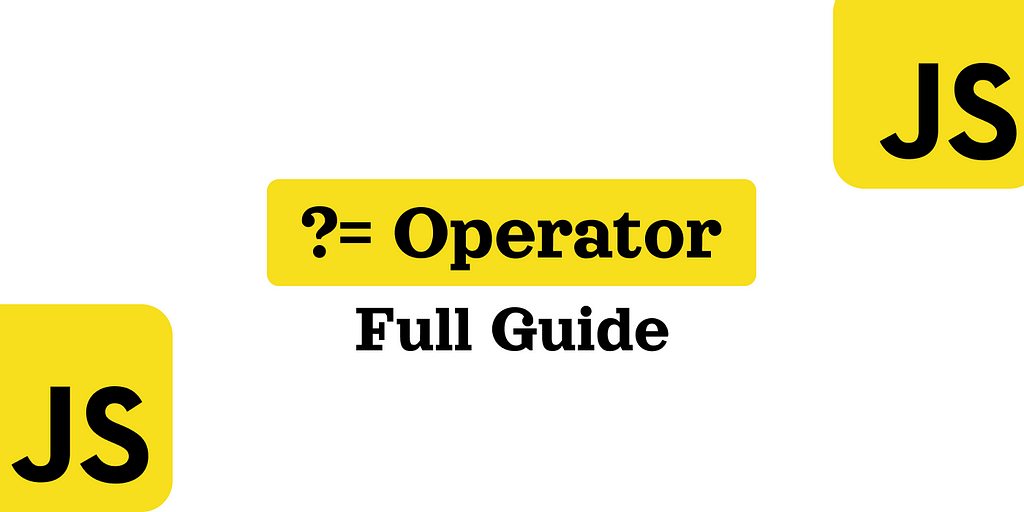
Table of Contents
- Simple Error Handling
- Works Seamlessly with Async Functions and Promises
- Cleaner and More Readable Code
- Unified Approach
- What is Symbol.result?
- Why Do We Need This Operator?
Simple Error Handling
One of the biggest advantages of ?= is that it simplifies error control. Instead of wrapping every potential “dangerous” line in a try-catch block, you can use this safe assignment operator.
It returns results in the format [error, result]. If no error occurs, you get [null, result]. Otherwise, it returns [error, null].
Here’s an example:
const [error, data] ?= await fetchData();
if (error) {
console.log("An error occurred:", error);
} else {
console.log("Data:", data);
}
Works Seamlessly with Async Functions and Promises
This operator plays nicely with promises and async functions, making it perfect for managing errors in async tasks. Think about API requests, file reading, or any operation where things might go wrong.
For example:
async function getData() {
const [fetchError, response] ?= await fetch(
"https://api.example.com/data"
);
if (fetchError) {
handleFetchError(fetchError);
return;
}
const [jsonError, data] ?= await response.json();
if (jsonError) {
handleJsonError(jsonError);
return;
}
return data;
}
🚨 For daily coding insights, follow me on Twitter!
Cleaner and More Readable Code
Using ?= helps reduce code nesting and gets rid of excessive try-catch blocks.
It also makes error handling more explicit and intuitive, which leads to better maintainability and fewer missed critical errors.
Let’s compare the old approach with the new one.
Traditional approach:
try {
const response = await fetch("https://api.example.com/data");
const json = await response.json();
const data = parseData(json);
} catch (error) {
handleError(error);
}
With ?=:
const [fetchError, response] ?= await fetch("https://api.example.com/data");
if (fetchError) {
handleFetchError(fetchError);
return;
}
Much cleaner, right?
Unified Approach
The ?= operator works with any object that implements the Symbol.result method.
This means you can use the same approach for error and result handling, no matter the data type or API. It adds flexibility when dealing with complex data structures or interacting with various services.
Here’s an example:
const obj = {
[Symbol.result]() {
return [new Error("An error occurred"), null];
},
};
const [error, result] ?= obj;
if (error) {
console.log("Error:", error);
}
What is Symbol.result?
Symbol.result is a method you can define on objects or functions to control how they return their results when used with the safe assignment operator.
When an object or function is called through ?=, Symbol.result processes the return value into a tuple of [error, result].
Imagine we have an object that implements Symbol.result to handle its own errors:
const obj = {
[Symbol.result]() {
return [new Error("Error in object"), null];
}
};
const [error, result] ?= obj;
console.log(error); // Outputs: Error in object
This feature allows developers to customize how objects and functions behave with the ?= operator.
It’s especially useful for libraries or frameworks where a unified error-handling approach is needed.
For example, an API response object could implement Symbol.result to return errors or successful data in a standardized format
Why Do We Need This Operator?
The ?= operator simplifies error handling and improves code readability. It reduces the need for repetitive try-catch blocks, handling errors at different stages like network requests, JSON parsing, or data validation in a single, clean format.
By using ?=, your code becomes cleaner and more predictable, especially when working with promises and async operations.
I’ll definitely be using this operator more in my projects!
Thanks for reading!
I hope you found this article useful. If you have any questions or suggestions, please leave comments. Your feedback helps me to become better.
Don’t forget to subscribe⭐
?= Operator in JavaScript was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Pavel Pogosov
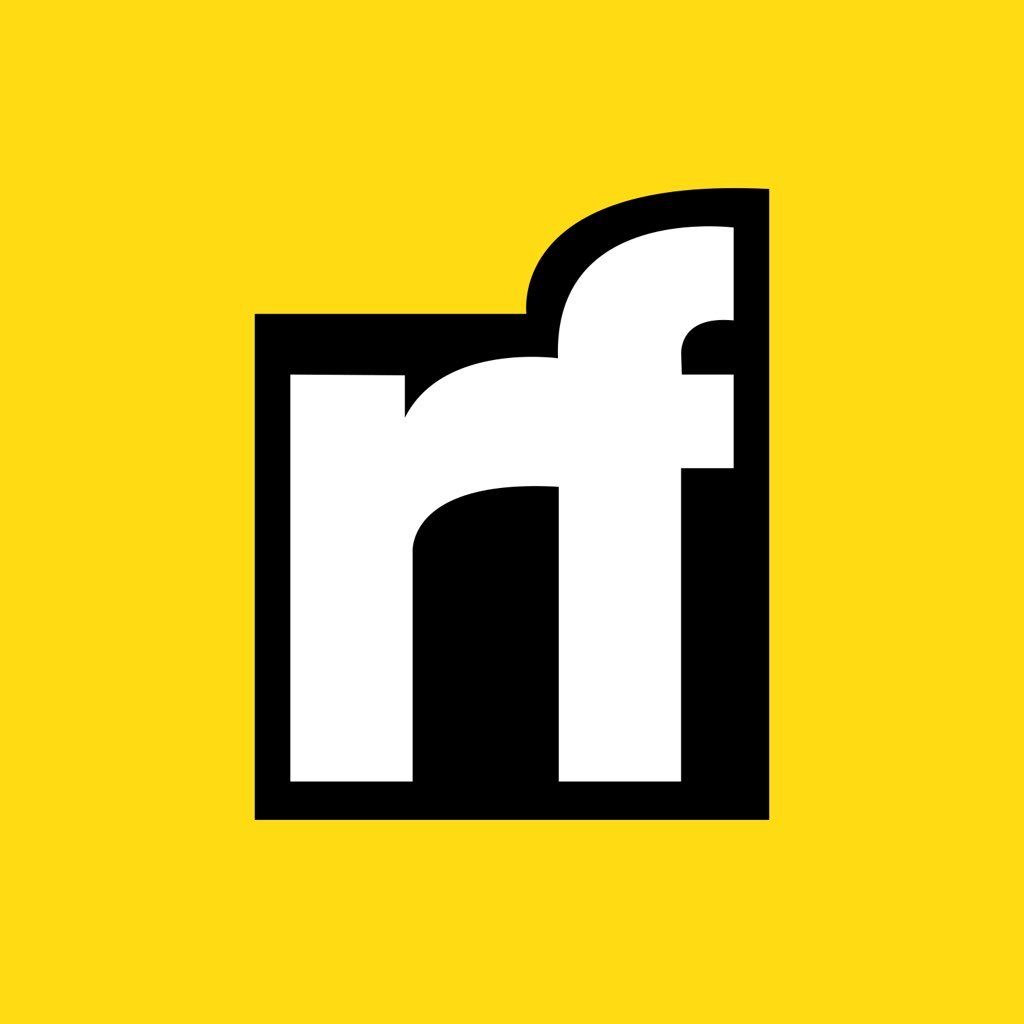
Pavel Pogosov | Sciencx (2024-10-24T01:05:55+00:00) ?= Operator in JavaScript. Retrieved from https://www.scien.cx/2024/10/24/operator-in-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.