This content originally appeared on Level Up Coding - Medium and was authored by Radhakishan Surwase
Processing a high volume of messages in real-time is a common challenge for systems interacting with event-driven queues, like Kafka or RabbitMQ. Go’s concurrency model, powered by goroutines and channels, is ideal for creating scalable pipelines that process messages with low latency and high throughput.
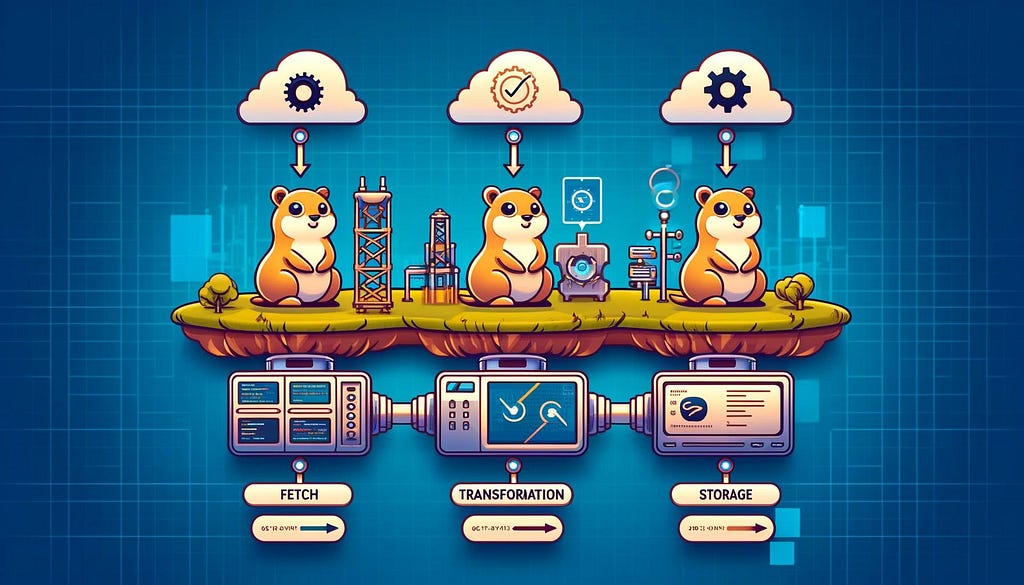
Here, we’ll build a 4-step data processing pipeline using an object-oriented approach with interfaces, a worker pool to manage concurrency, and channels to maintain efficient data flow between stages.
Overview of the Pipeline
In this pipeline, we’ll structure the processing into four stages:
- Fetching — Retrieve messages from a queue or a source.
- Validation — Validate the fetched data to ensure it meets certain criteria.
- Transformation — Transform the data for the final processing.
- Storage — Store the processed data in a database or external system.
Each stage will be an independent worker pool using a pool of goroutines to handle messages concurrently, ensuring the system can scale effectively as message volume increases.
Key Components and Architecture
Our solution involves creating modular and reusable components that communicate via channels. Each stage will implement a Processor interface, ensuring any future stages can be easily added or modified. We’ll also design a generic WorkerPool to manage processing for each stage.
1. Defining the Message Struct
The Message struct represents a single message that flows through the pipeline. It could contain any type of data; in this example, we keep it simple:
type Message struct {
ID int
Data string
}
2. Creating the Processor Interface
Each processing stage (Fetch, Validate, Transform, Store) implements a Processor interface. This interface defines a single Process method, which will receive and return a Message. Implementing stages as separate structs keeps the code modular and flexible.
type Processor interface {
Process(msg Message) Message
}
3. Implementing Pipeline Stages
Each pipeline stage (Fetcher, Validator, Transformer, Storer) will implement the Processor interface. Here’s how each stage would look:
type Fetcher struct{}
func (f Fetcher) Process(msg Message) Message {
msg.Data = fmt.Sprintf("Fetched: %s", msg.Data)
return msg
}
type Validator struct{}
func (v Validator) Process(msg Message) Message {
msg.Data = fmt.Sprintf("Validated: %s", msg.Data)
return msg
}
type Transformer struct{}
func (t Transformer) Process(msg Message) Message {
msg.Data = fmt.Sprintf("Transformed: %s", msg.Data)
return msg
}
type Storer struct{}
func (s Storer) Process(msg Message) Message {
fmt.Printf("Stored: %s\n", msg.Data)
return msg
}
Each struct’s Process method performs its specific task. The Storer stage represents the final output and simply prints the stored message. In a real application, it would likely store the message in a database.
4. Implementing the WorkerPool Struct
To handle each stage concurrently, we create a WorkerPool struct. Each pool will spin up multiple goroutines to process messages as they come through the pipeline.
type WorkerPool struct {
processor Processor
numWorkers int
in chan Message
out chan Message
wg *sync.WaitGroup
}
func NewWorkerPool(processor Processor, numWorkers int) *WorkerPool {
return &WorkerPool{
processor: processor,
numWorkers: numWorkers,
in: make(chan Message),
out: make(chan Message),
wg: &sync.WaitGroup{},
}
}
func (wp *WorkerPool) Start() {
for i := 0; i < wp.numWorkers; i++ {
go func() {
for msg := range wp.in {
processedMsg := wp.processor.Process(msg)
wp.out <- processedMsg
}
wp.wg.Done()
}()
}
}
Each WorkerPool manages a set of goroutines. These goroutines read messages from the in channel, process them, and then send the processed messages to the out channel. The WorkerPool struct includes:
- Processor: The stage-specific processor implementing Processor.
- numWorkers: Number of concurrent workers in the pool.
- Channels: Channels for input and output.
- WaitGroup: Used to synchronize worker shutdown.
5. Building the Pipeline
Finally, we define the RunPipeline function to link each stage. This function sets up channels between stages, initializes each WorkerPool, and handles the flow from start to finish:
func RunPipeline(messages []Message) {
fetcher := NewWorkerPool(Fetcher{}, 3)
validator := NewWorkerPool(Validator{}, 3)
transformer := NewWorkerPool(Transformer{}, 3)
storer := NewWorkerPool(Storer{}, 3)
fetcher.wg.Add(1)
go func() {
for _, msg := range messages {
fetcher.in <- msg
}
close(fetcher.in)
fetcher.wg.Wait()
close(fetcher.out)
}()
validator.wg.Add(1)
go func() {
for msg := range fetcher.out {
validator.in <- msg
}
close(validator.in)
validator.wg.Wait()
close(validator.out)
}()
transformer.wg.Add(1)
go func() {
for msg := range validator.out {
transformer.in <- msg
}
close(transformer.in)
transformer.wg.Wait()
close(transformer.out)
}()
storer.wg.Add(1)
go func() {
for msg := range transformer.out {
storer.in <- msg
}
close(storer.in)
storer.wg.Wait()
close(storer.out)
}()
fetcher.Start()
validator.Start()
transformer.Start()
storer.Start()
time.Sleep(2 * time.Second)
}
This RunPipeline function:
- Initializes Each Worker Pool: Each stage in the pipeline is represented by a worker pool.
- Links Stages Using Channels: Channels link the output of one stage to the input of the next.
- Starts Worker Pools: Begins processing with concurrent workers in each stage.
6. Running the Pipeline
To test the pipeline, we define some sample messages and call RunPipeline in the main function:
func main() {
messages := []Message{
{ID: 1, Data: "Message 1"},
{ID: 2, Data: "Message 2"},
{ID: 3, Data: "Message 3"},
}
RunPipeline(messages)
}
Each message flows through the pipeline, and its data is processed at each stage, demonstrating the concurrent processing of each message.
Key Benefits of This Approach
- Scalability: The worker pool design allows for parallel processing in each stage. You can adjust the number of workers per stage to optimize performance based on message volume.
- Modularity: Using the Processor interface for each stage keeps the pipeline components isolated. You can easily add new stages or modify existing ones without affecting the whole pipeline.
- Concurrent and Efficient: Each stage operates independently, allowing high throughput as messages move smoothly through the pipeline.
Conclusion
With Go’s powerful concurrency primitives, we can build a data processing pipeline capable of handling high message volumes in a scalable and maintainable manner. This pipeline design demonstrates the principles of modularity, scalability, and reusability, making it easy to expand and adapt to different processing requirements.
This approach is ideal for applications requiring real-time processing, such as ETL (Extract, Transform, Load) pipelines, data streaming, and event-driven architectures, allowing us to harness the full power of Golang’s concurrency model.
Mastering Real-Time Data Pipelines in Golang with Channels and Worker Pools was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Radhakishan Surwase
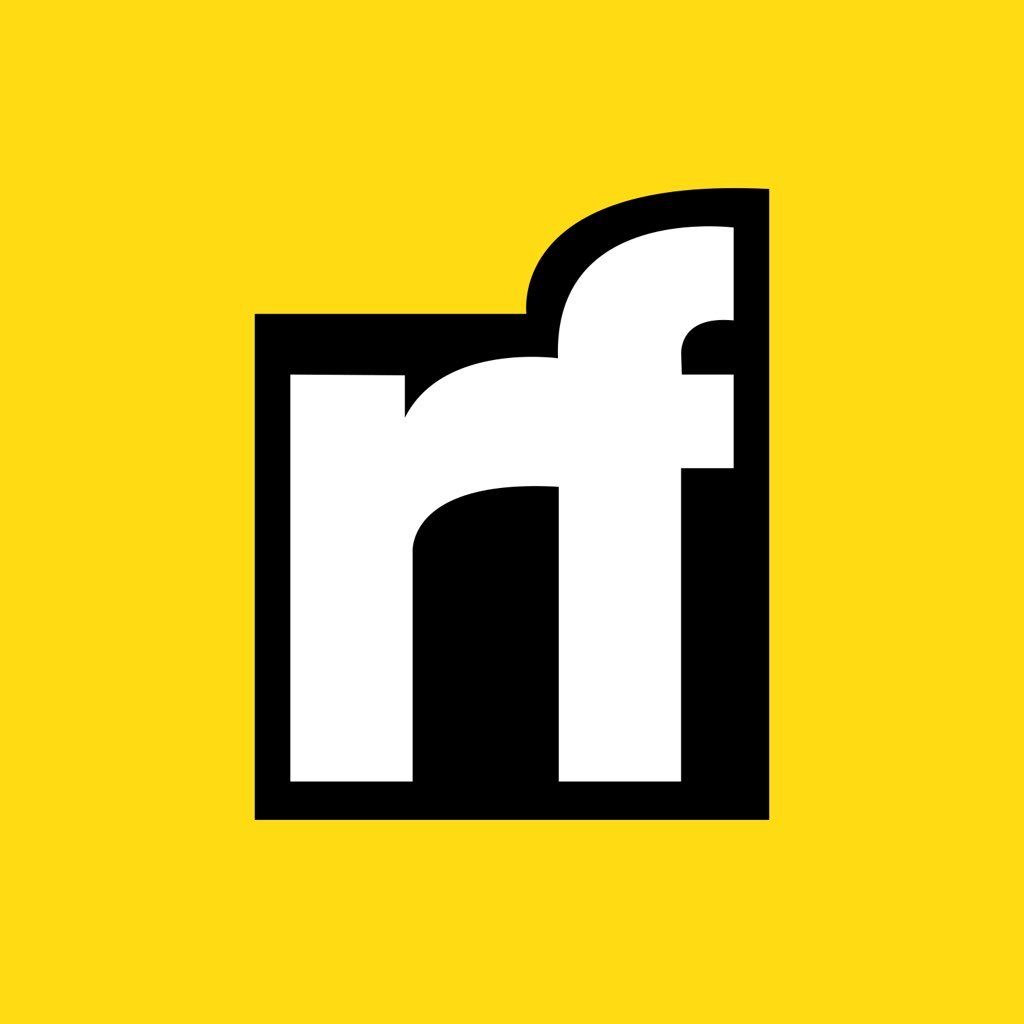
Radhakishan Surwase | Sciencx (2024-10-28T00:59:23+00:00) Mastering Real-Time Data Pipelines in Golang with Channels and Worker Pools. Retrieved from https://www.scien.cx/2024/10/28/mastering-real-time-data-pipelines-in-golang-with-channels-and-worker-pools/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.