This content originally appeared on Bits and Pieces - Medium and was authored by Mike Chen
Learn how to use Bit to share Zod validation schemas across repositories. Simplify collaboration, versioning, and updates while maintaining consistency in your codebase.
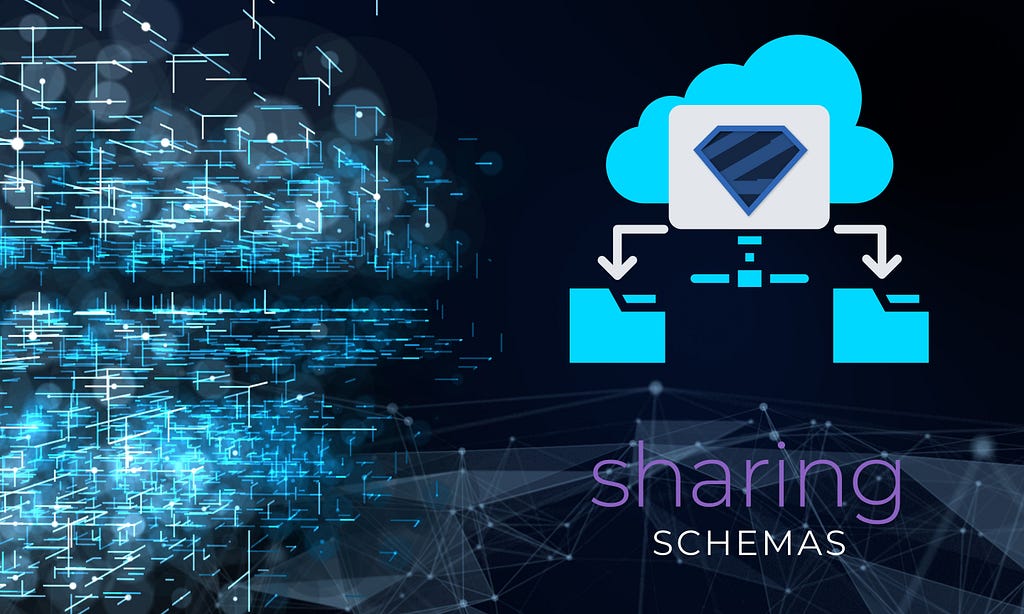
As modern web applications grow increasingly modular and complex, sharing code between repositories becomes an essential aspect of efficient development. Zod, a powerful TypeScript-first schema declaration and validation library, is a popular choice for managing and validating data structures.
However, sharing Zod schemas between repositories can become challenging in a fragmented environment. This is where Bit comes into play — a tool designed for sharing, maintaining, and reusing components effortlessly.
In this post, we’ll explore how to leverage Bit to share Zod schemas between repositories, ensuring consistency and reducing redundant work.
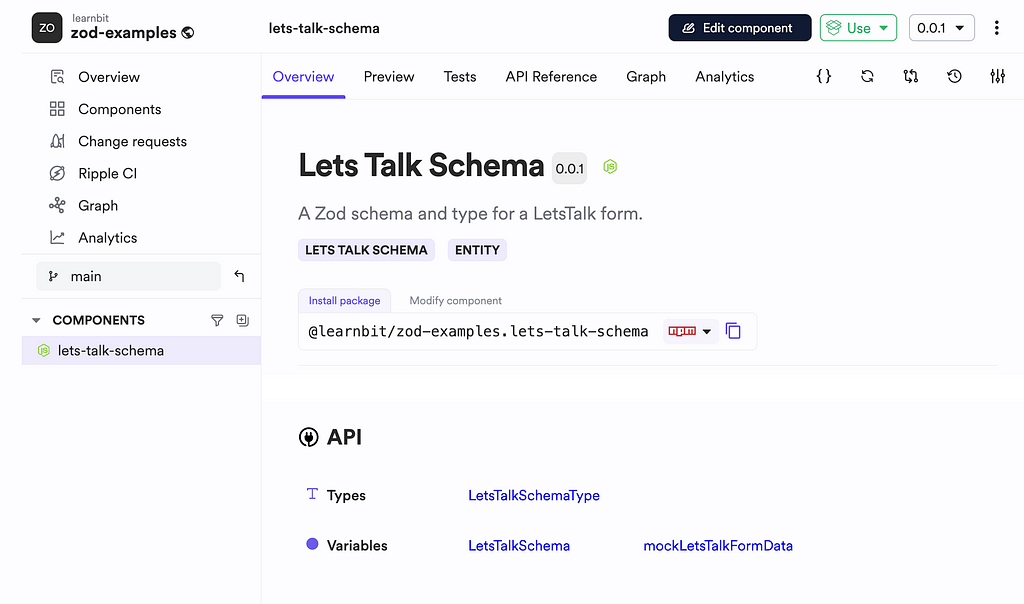
We’ll go over a demo project that uses a Zod schema to validate the data sent from a “Let’s Talk” form to a corresponding Express service that handles that request.
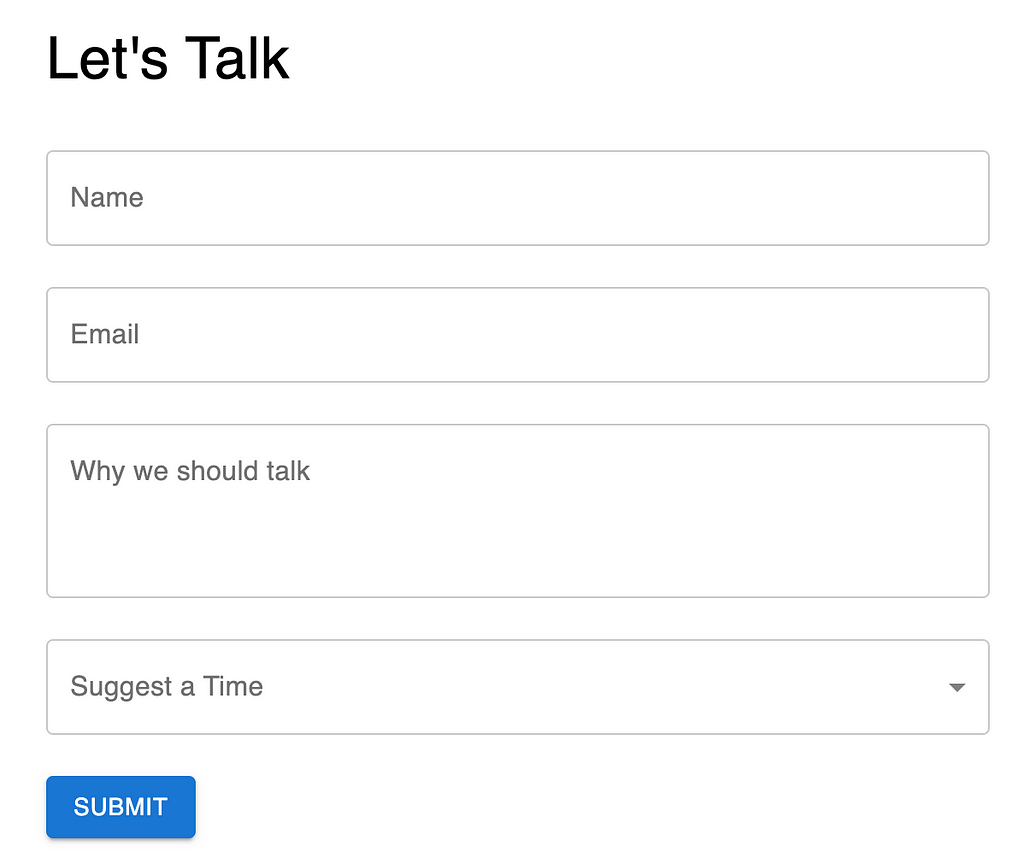
The Zod schema will be maintained as a Bit component, shared on Bit platform and installed in the various repositories (to make the demo easy to navigate, all projects are maintained in the same project, however they do not share dependencies).
GitHub - bitdev-community/zod-with-bit: A demo of a shared Zod schema
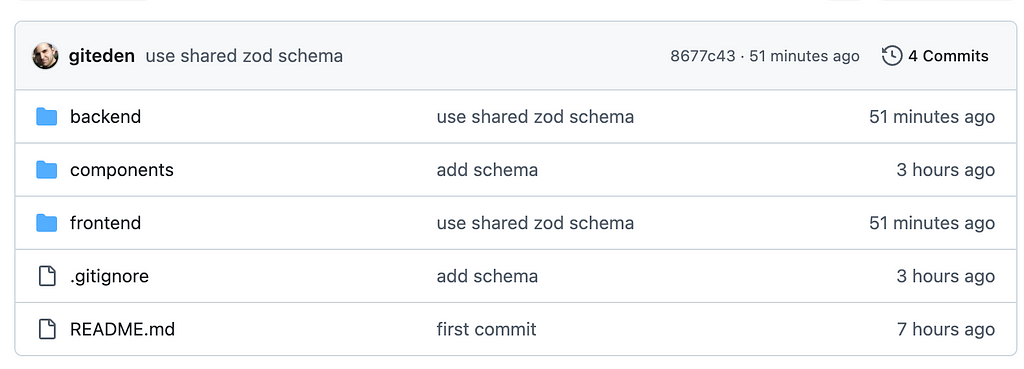
Why Share Zod Schemas?
Zod schemas are often used to define the shape of data and ensure its integrity. These schemas can validate incoming requests, enforce types in API contracts, or act as a source of truth for both backend and frontend systems.
Benefits of sharing Zod schemas include:
- Consistency: Avoid mismatched data validation logic across projects.
- Reusability: Reduce redundant schema definitions.
- Maintenance: Centralize schema updates and propagate changes seamlessly.
However, traditional approaches to sharing schemas — such as publishing npm packages — can become cumbersome to manage as projects scale.
The Bit Advantage
Bit simplifies the process of sharing Zod schemas across repositories. It allows you to:
- Isolate each schema as a Bit component.
- Version schemas independently to manage updates and maintain backward compatibility.
- Share schemas seamlessly across projects using Bit’s platform or self-hosted scopes.
- Collaborate with built-in tools for CI, testing, and change requests.
Bit eliminates the overhead of managing monorepos or custom package registries, streamlining your workflow.
Getting Started: Sharing Zod Schemas with Bit
Step 1: Install and Set Up Bit
Install Bit using the CLI:
npx @teambit/bvm install
Initialize a Bit workspace in a separate repository or inside an existing repository to allow for modules to be shared as Bit components:
bit init --default-scope my-org.schemas
Note that maintaining the Zod schema (Bit component) outside your project’s repo means that it will not necessarily use the latest version of that schema as it depends by which version of it was installed.
Having said that, since Bit components are not tied to any repository, you can change your mind at any time and maintain the components in any repository you’d like.
Step 2: Create Zod Schemas as Components
Install Zod as a dependency in your workspace:
bit install zod
Create a new Bit component for your schema. We’ll use the template for “entity components”:
bit create entity lets-talk-schema
Define your Zod schema in the generated component. In our example, we’ll use Zod to validate the information sent by a form that requests a meeting:
/**
* @filename: lets-talk-schema.ts
*/
import { z } from 'zod';
export const LetsTalkSchema = z.object({
name: z.string().min(1, 'Name is required'),
email: z.string().email('Invalid email address'),
whyWeShouldTalk: z.string().min(1, 'Reason is required'),
suggestTime: z.string().min(1, 'Suggested time is required'),
});
We’ll also use Zod’s infer method to generate a Typescript type based on our schema:
/**
* @filename: lets-talk-schema.ts
*/
export type LetsTalkSchemaType = z.infer<typeof LetsTalkSchema>;
In addition, it’s recommended to add mock data that can be used for testing. This mock data will always be up-to-date with the latest changes of that schema as it is versioned along with it as a single Bit component:
/**
* @filename: lets-talk.mock.ts
*/
import type { LetsTalkSchemaType } from './lets-talk-schema.js';
export const mockLetsTalkFormData: LetsTalkSchemaType = {
name: 'Alice Example',
email: 'alice@example.com',
whyWeShouldTalk:
'We want to discuss a potential business partnership opportunity.',
suggestTime: '2024-12-30 02:00 PM',
};
We’ll then export all three (*Zod schema,. Typescript type, and mock data) from the component’s entry file, to make it available for external consumers:
/**
* @filename: index.ts
*/
export { LetsTalkSchema } from './lets-talk-schema.js';
export type { LetsTalkSchemaType } from './lets-talk-schema.js';
export { mockLetsTalkFormData } from './lets-talk.mock.js';
Step 3: Tag and Export Components
- Tag the component with a version:
bit tag lets-talk-schema --message "initial version"
- Export the component to an access-controlled scope in Bit platform (create a scope if you don’t have one already).
bit export
See this shared Zod schema for example:
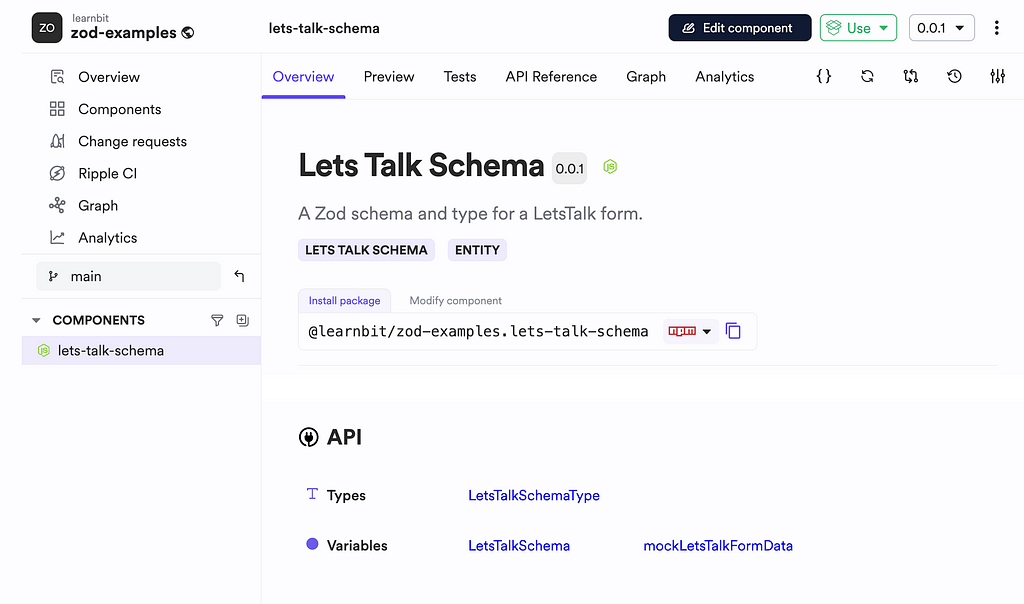
Step 4: Consume the Schema in Another Repository
Install the schema in the relevant projects using bit or a standard package manager like npm, pnpm or yarn:
npm i @learnbit/zod-examples.lets-talk-schema
Use the schema in your projects. For example:
import {
LetsTalkSchemaType,
LetsTalkSchema,
} from '@learnbit/zod-examples.lets-talk-schema';
// ...
const LetsTalkForm:= () => {
const [submitStatus, setSubmitStatus] = useState<FormStatus>('idle');
const {
register,
handleSubmit,
formState: { errors },
reset,
} = useForm<LetsTalkSchemaType>({
resolver: zodResolver(LetsTalkSchema),
});
const onSubmit = async (data: LetsTalkSchemaType) => {
// ...
}
Advantages of Using Bit for Zod Schemas
- Streamlined publishing: Bit handles your package (Bit component) dependencies, build and package.json configuration for you
- Version Control: Manage schema versions independently, ensuring updates don’t break dependent projects.
- Discoverability: Use Bit’s web platform to browse schemas, view usage examples, and documentation.
- Seamless Updates: Propagate updates to dependent repositories with minimal effort.
- Collaborative Development: Use Bit’s change requests to review and approve updates collaboratively.
- Integration with CI: Automatically build and test schemas to maintain quality and reliability.
Conclusion
Sharing Zod schemas across repositories becomes seamless with Bit. By treating schemas as composable components, Bit enables efficient collaboration, consistency, and maintainability. Whether you’re managing a monorepo, polyrepo, or entirely decentralized codebase, Bit offers the tools you need to make your Zod schemas a cornerstone of your development process.
Ready to streamline your schema sharing? Start using Bit today and transform how you manage and reuse your Zod schemas!
Share Zod Validation Schemas Between Repositories was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Mike Chen
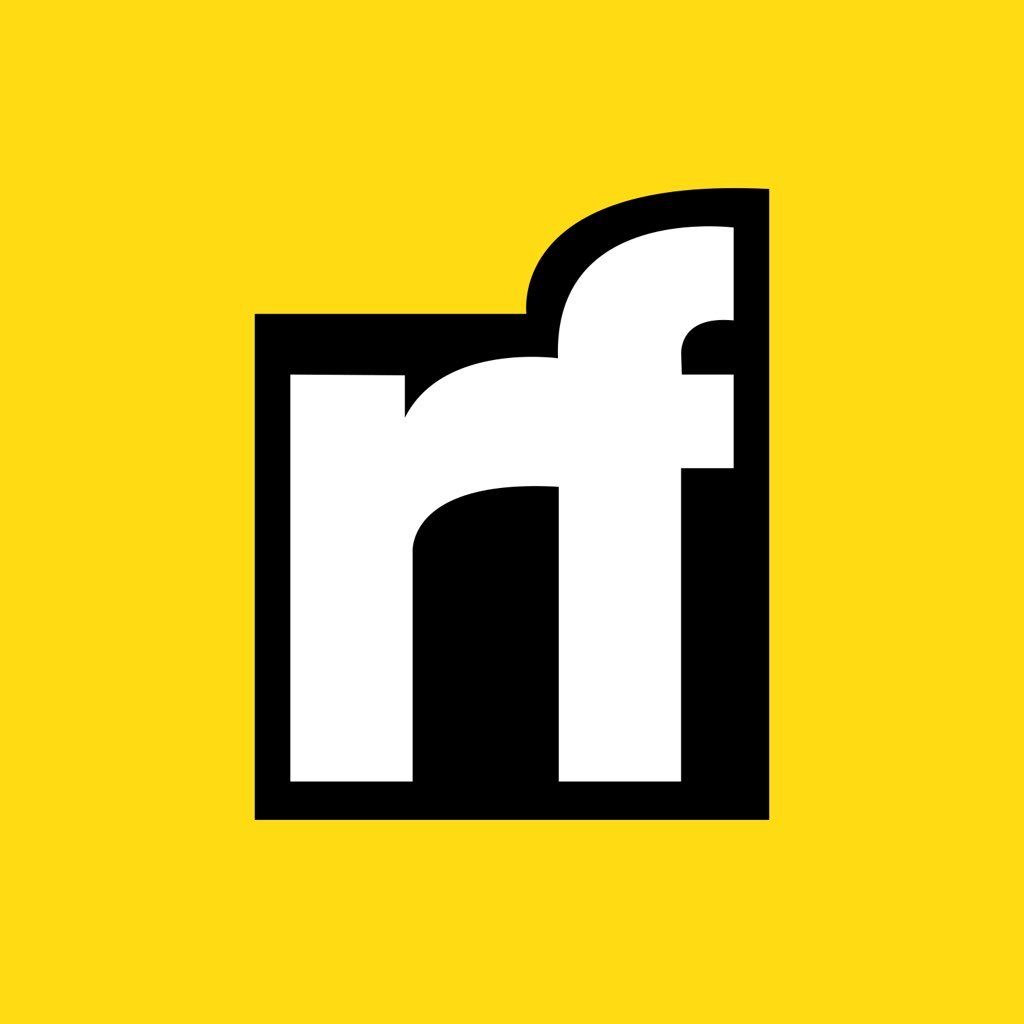
Mike Chen | Sciencx (2024-12-25T21:57:07+00:00) Share Zod Validation Schemas Between Repositories. Retrieved from https://www.scien.cx/2024/12/25/share-zod-validation-schemas-between-repositories/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.