This content originally appeared on DEV Community and was authored by Talles L
from asyncio import run, sleep
class AlternatingGenerator:
def __init__(self, gen1, gen2):
self.gen1 = gen1
self.gen2 = gen2
self.next = gen1
def __aiter__(self):
return self
async def __anext__(self):
# if we are done we both generators
if not self.gen1 and not self.gen2:
raise StopAsyncIteration
try:
# saving the current generator into a variable for later
current = self.next
# if the other genrerator is not null, alternating to it for the next iteration
if self.next is self.gen1 and self.gen2:
self.next = self.gen2
elif self.next is self.gen2 and self.gen1:
self.next = self.gen1
# calling the generator of the current iteration and returning the next result
return await anext(current)
except StopAsyncIteration:
# unsetting the generator that just finished
if current is self.gen1:
self.gen1 = None
else:
self.gen2 = None
# recursive call, trying again with the other generator
return await self.__anext__()
async def three():
for i in range(3, 31, 10):
await sleep(0.25)
yield i
async def five():
for i in range(5, 101, 10):
await sleep(0.25)
yield i
async def main():
gen = AlternatingGenerator(three(), five())
try: # or just use "async for item in gen:"
while True:
print(await anext(gen))
except StopAsyncIteration:
pass
if __name__ == '__main__':
run(main())
$ python alternating_generator.py
3
5
13
15
23
25
35
45
55
65
75
85
95
This content originally appeared on DEV Community and was authored by Talles L
Print
Share
Comment
Cite
Upload
Translate
Updates
There are no updates yet.
Click the Upload button above to add an update.
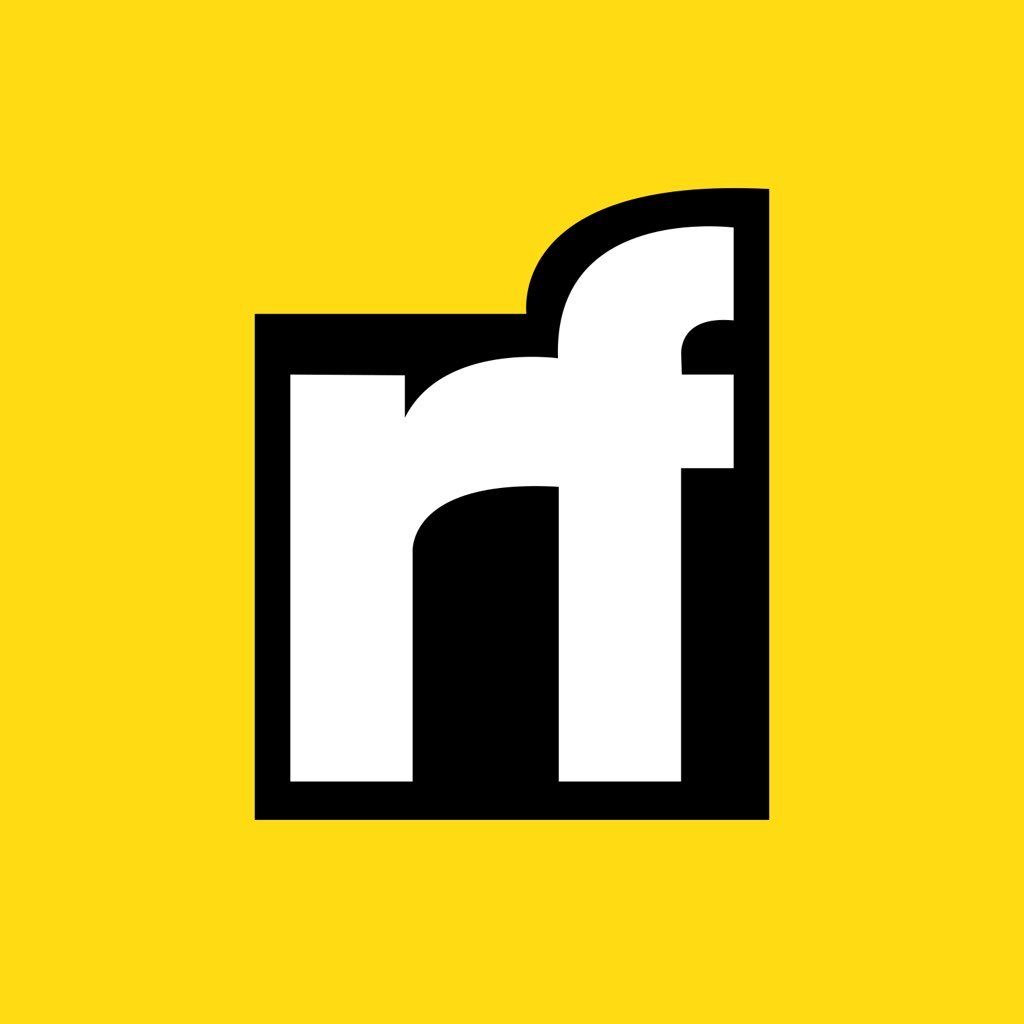
APA
MLA
Talles L | Sciencx (2025-01-02T23:24:30+00:00) An Alternating Asynchronous Generator in Python. Retrieved from https://www.scien.cx/2025/01/02/an-alternating-asynchronous-generator-in-python/
" » An Alternating Asynchronous Generator in Python." Talles L | Sciencx - Thursday January 2, 2025, https://www.scien.cx/2025/01/02/an-alternating-asynchronous-generator-in-python/
HARVARDTalles L | Sciencx Thursday January 2, 2025 » An Alternating Asynchronous Generator in Python., viewed ,<https://www.scien.cx/2025/01/02/an-alternating-asynchronous-generator-in-python/>
VANCOUVERTalles L | Sciencx - » An Alternating Asynchronous Generator in Python. [Internet]. [Accessed ]. Available from: https://www.scien.cx/2025/01/02/an-alternating-asynchronous-generator-in-python/
CHICAGO" » An Alternating Asynchronous Generator in Python." Talles L | Sciencx - Accessed . https://www.scien.cx/2025/01/02/an-alternating-asynchronous-generator-in-python/
IEEE" » An Alternating Asynchronous Generator in Python." Talles L | Sciencx [Online]. Available: https://www.scien.cx/2025/01/02/an-alternating-asynchronous-generator-in-python/. [Accessed: ]
rf:citation » An Alternating Asynchronous Generator in Python | Talles L | Sciencx | https://www.scien.cx/2025/01/02/an-alternating-asynchronous-generator-in-python/ |
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.