This content originally appeared on Level Up Coding - Medium and was authored by Oleks Gorpynich
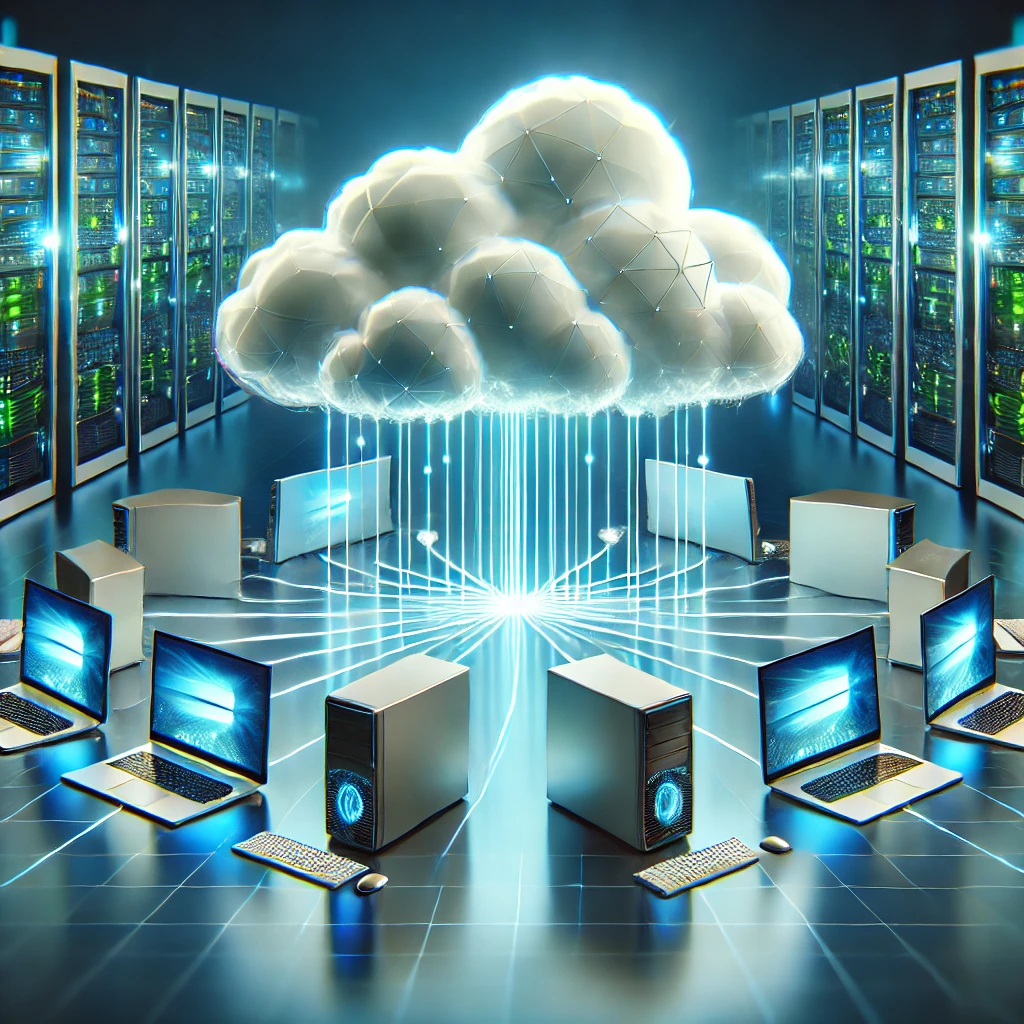
So…what the hell is RPC (Remote Procedure Call), and why should you care?
In short, RPC is a way for one process to communicate with another process. It is a protocol that allows a program to request a service or function to be executed on a remote server as if it were a local function call. I’ll include an example to make this more clear.
Server
import someRpcLibrary
# Define the function to expose as an RPC
def add(x, y):
return x + y
# Create the server and register the "add" function
server = someRpcLibrary.RPCServer("localhost", 5000)
server.register_function(add) # Expose the "add" function
server.run() # Start the server
Client
import someRpcLibrary
# Create the client and connect to the server
client = someRpcLibrary.RPCClient("localhost", 5000)
# Call the "add" function on the server with arguments 5 and 10
result = client.call("add", 5, 10)
print(f"Result from server: {result}") # Output: 15
“Note: someRpcLibrary is a placeholder library used here for illustration. In real-world implementations, libraries such as gRPC, Pyro, or XML-RPC frameworks handle this process."
So what are we looking at?
Well, the client seems to be “calling” an add function. This add function is defined and executed on the server. The server then responds with the result, and the client prints it once it receives it. Pretty simple.
Firstly, it’s important to realize that there are multiple implementations of the RPC protocol, and it can work in a lot of ways. In our toy example, this protocol is implemented by someRpcLibrary which could have done this in a lot of ways.
For example, someRpcLibrary might send the request over HTTP, WebSockets, or even a simple TCP socket connection. The data itself could be formatted as JSON, binary, or something else entirely. The key takeaway is that the details of how the message is sent, received, and processed are abstracted away—what the client sees is just a local-looking function call. This is the magic of RPC: it hides the complexity of remote communication, making distributed systems feel as simple as calling functions within the same application.
However, although there are many various implementations, there are some key aspects of RPC
- Transparency: The client interacts with the remote function as if it were local, without worrying about the underlying network details.
- Client-Server Architecture: The client sends a request to the server, which performs the function and sends back the result.
- Serialization/Deserialization: Data is marshalled (serialized) on the client-side and demarshalled (deserialized) on the server-side.
- Synchronous or Asynchronous: RPC can be blocking (synchronous) or non-blocking (asynchronous).
- Cross-Platform: RPC can work across different operating systems and programming languages.
Marshalling and Demarshalling
One of the most crucial steps in the RPC process is marshalling and demarshalling. These terms might sound complex, but they refer to something quite simple: the process of converting data into a format suitable for transmission and reconstructing it back into its original form.
What is Marshalling?
Marshalling is the process of converting function arguments or data structures into a byte stream or another transferable format that can be sent across the network.
- Why Marshalling? Networks can only transmit raw data, so complex objects (like lists, dictionaries, or custom data types) need to be “flattened” or serialized into a simple format such as:
- JSON (human-readable text format)
- Binary (compact, efficient format like Protocol Buffers)
Example: Imagine a client calls:
add(5, 10)
The arguments 5 and 10 are marshalled into a message format like
{
"procedure": "add",
"params": [5, 10]
}
This JSON string is sent over the network as bytes.
What is Demarshalling
Demarshalling is the process of converting the received byte stream back into usable data — essentially “reversing” the marshalling process.
- Once the server receives the marshalled request, it demarshalls it to extract:
- The function name ("add")
- The arguments (5, 10)
- After processing, the server sends the result -15, back to the client in a marshalled format (e.g., JSON).
The client then demarshalls the server’s response into a Python integer (15), making it usable in the local program.
gRPC
gRPC which stands for Google Remote Procedure Call is a high performance framework which implements the RPC protocol. It allows for high-speed, cross-platform, and cross-language communication between services and applications. It is commonly used as the communication layer in a micro-services architecture. The framework uses uses Protocol Buffers (protobuf) as its default serialization (marshalling/demarshalling) mechanism and supports HTTP/2 as its transport layer.
How gRPC Works
The process of using gRPC can be broken down into key steps:
Define the Service (IDL — Interface Definition Language):
- You define the service and methods using Protocol Buffers (proto files).
- A .proto file specifies the available RPC methods and the request/response message formats.
- Example calculator.proto:
syntax = "proto3";
service Calculator {
rpc Add (AddRequest) returns (AddResponse);
}
message AddRequest {
int32 a = 1;
int32 b = 2;
}
message AddResponse {
int32 result = 1;
}
Generate Client and Server Code:
- You use the Protocol Buffers compiler (protoc) to generate client and server stubs in your preferred language (Python, Go, Java, etc.).
- These stubs handle the marshaling/unmarshaling of data, making remote function calls look like local function calls. They are compiled with the code you wrote for the actual server and client, and actually allow communication to work :)
Implement the Server:
- The server implements the logic of the defined methods.
- Example (Python server):
from concurrent import futures
import grpc
import calculator_pb2
import calculator_pb2_grpc
class CalculatorServicer(calculator_pb2_grpc.CalculatorServicer):
def Add(self, request, context):
result = request.a + request.b
return calculator_pb2.AddResponse(result=result)
server = grpc.server(futures.ThreadPoolExecutor(max_workers=10))
calculator_pb2_grpc.add_CalculatorServicer_to_server(CalculatorServicer(), server)
server.add_insecure_port('[::]:50051')
server.start()
server.wait_for_termination()
Implement the Client:
import grpc
import calculator_pb2
import calculator_pb2_grpc
# Establish a connection to the server
channel = grpc.insecure_channel("localhost:50051")
stub = calculator_pb2_grpc.CalculatorStub(channel)
# Create an AddRequest message with the numbers to add
request = calculator_pb2.AddRequest(a=5, b=10)
# Make the remote call to the "Add" function and get the response
response = stub.Add(request)
print(f"Result from server: {response.result}") # Output: 15
channel.close() # Close the channel when done
So why gRPC over REST for cross-microservice communication?
Performance and efficiency
- gRPC: Uses Protocol Buffers (binary serialization) instead of JSON for data transmission. Protocol Buffers are significantly smaller, faster to serialize/deserialize, and more efficient in terms of bandwidth.
- REST: Uses JSON (a human-readable text format), which is larger in size and slower to parse compared to binary formats.
HTTP/2 Support (vs HTTP/1.1)
- gRPC supports HTTP/2 which is a newer version of HTTP. This comes with a few advantages, including multiplexing, which is where multiple requests can share a single connection without blocking each other.
Streaming Support
gRPC: Supports multiple types of streaming: This can be incredibly useful for microservices that require more methods for communication outside of the classic client/server model.
- Unary RPC: Single request, single response (like REST).
- Server Streaming: Server sends a continuous stream of responses for a single request.
- Client Streaming: Client sends multiple requests in a stream, and the server responds once.
- Bidirectional Streaming: Both client and server can continuously send data streams to each other.
Strongly Typed Contracts
- gRPC: Uses .proto files (Protocol Buffers) to define the service, request, and response messages. This ensures that the client and server adhere to a well-defined, strongly-typed contract.
- With REST, sure there might be API documentation, but this isn’t enforced.
Still, REST is useful in some cases. For one, it is incredibly simple, so getting something to work quick is much easier. Second, public facing APIs are basically impossible with RPC. It is primarily used for micro-service <-> micro-service (or process <-> process) communication. If you don’t have control over your client, REST wins. Finally — browser support. If you need to communicate with a browser, REST apis can be be easily consumed in browsers using JavaScript fetch or XMLHttpRequest
Conclusion
In short, RPC is just a protocol that makes remote calls feel local, and gRPC is a popular implementation of this protocol by Google. It’s built for the modern micro-services world, where low latency and cross-language communication are key. Still, REST APIs win out when simplicity, browser support, and public-facing APIs matter most.
I do often see devs ignoring the advantages of RPC, so hopefully this article gives you some food for thought and avenues for future research :)
Hopefully you can take this knowledge and put it into your own projects.
What is RPC? What is gRPC? was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Oleks Gorpynich
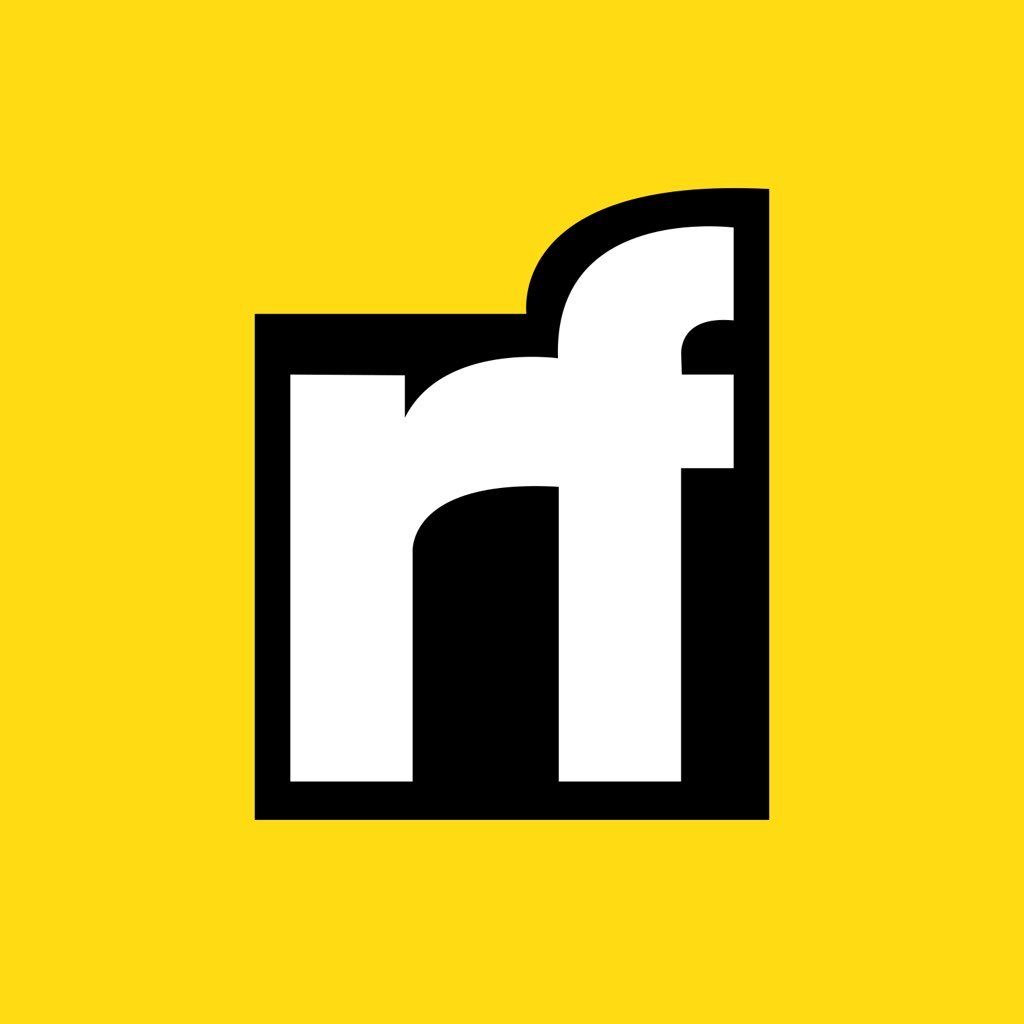
Oleks Gorpynich | Sciencx (2025-01-10T03:00:03+00:00) What is RPC? What is gRPC?. Retrieved from https://www.scien.cx/2025/01/10/what-is-rpc-what-is-grpc/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.