This content originally appeared on Go Make Things and was authored by Go Make Things
Callback functions are a core part of how a lot of modern JavaScript methods work. But what exactly are they?
A callback function is just a function that another function runs (either immediately or a later time).
For example, consider the Element.addEventListener()
method. You pass in the type of event to listen for, and a callback function that should run when the event happens…
document.addEventListener('click', function (event) {
console.log('The document was clicked!');
});
A lot of methods that modify arrays ([]
) loop over each item in the array and run a callback function on each one.
For example, here the Array.prototype.forEach()
method runs the callback function on every wizard in the wizards
array, and logs their name to the console…
let wizards = ['Merlin', 'Gandalf', 'Radagast'];
wizards.forEach(function (wizard) {
console.log(wizard);
});
The examples above use anonymous callback functions. A new unnamed function is created and passed directly into the method.
But you can also predefine a named function and pass that in as a callback function instead. When you do, omit the the parentheses (()
) at the end. Those cause the function to run, and we only to pass in the function itself.
The method that’s using it will run it when needed.
function handleClicks (event) {
console.log(`The ${event.target} was clicked!`);
}
document.addEventListener('click', handleClicks);
Looking at this code, you might also wonder: where do the arguments passed into the callback functions come from?
We’ll talk about that tomorrow!
Like this? A Lean Web Club membership is the best way to support my work and help me create more free content.
This content originally appeared on Go Make Things and was authored by Go Make Things
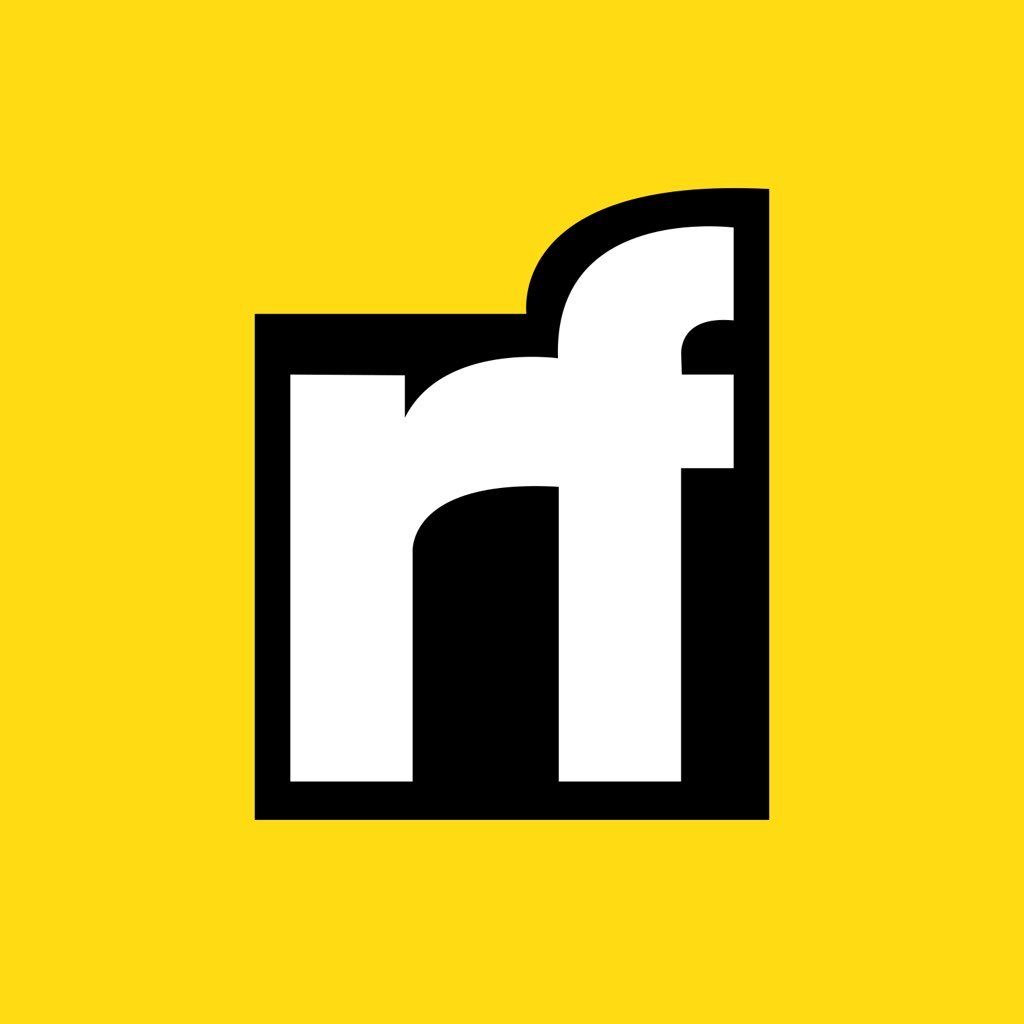
Go Make Things | Sciencx (2025-01-15T14:30:00+00:00) What is a callback function?. Retrieved from https://www.scien.cx/2025/01/15/what-is-a-callback-function-2/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.