This content originally appeared on Envato Tuts+ Tutorials and was authored by Esther Vaati
So what are we making?! This:
We’ll be getting familiar with color pickers, the canvas elements, curves.. you name it.
Building the interface
Let’s start with a minimal interface consisting of:
- A preview area containing a canvas element to display the blob.
- A color picker element to change the blob’s color (we’ll give it a default value of a nice Envato green #9cee69).
- 2 range slider elements for changing the variation and complexity of the blob.
- A download button to save the generated blob
The HTML structure will look like this:
1 |
<main class="container"> |
2 |
<header>
|
3 |
<h1>Blob Generator</h1> |
4 |
</header>
|
5 |
|
6 |
<section class="preview"> |
7 |
<canvas id="canvas" width="300" height="300"></canvas> |
8 |
</section>
|
9 |
<section class="customize-container"> |
10 |
<label for="colorPicker">Color:</label> |
11 |
<input
|
12 |
type="color" |
13 |
id="colorPicker" |
14 |
class="color-picker" |
15 |
value="#9cee69" |
16 |
/>
|
17 |
|
18 |
<div class="slider-container"> |
19 |
<label for="complexitySlider" class="slider-label">Complexity:</label> |
20 |
<input
|
21 |
type="range" |
22 |
id="complexitySlider" |
23 |
class="slider" |
24 |
min="3" |
25 |
max="12" |
26 |
value="6" |
27 |
/>
|
28 |
</div>
|
29 |
|
30 |
<div class="slider-container"> |
31 |
<label for="Variation" class="slider-label">Variation:</label> |
32 |
<input
|
33 |
type="range" |
34 |
id="variation-slider" |
35 |
class="slider" |
36 |
min="0" |
37 |
max="100" |
38 |
value="50" |
39 |
/>
|
40 |
</div>
|
41 |
|
42 |
<button type="button" id="downloadBtn">Download Blob</button> |
43 |
</section>
|
Styling with CSS
Time to snazz things up. Here are the base styles for enhancing the design of the UI.
1 |
body { |
2 |
display: flex; |
3 |
flex-direction: column; |
4 |
align-items: center; |
5 |
min-height: 100vh; |
6 |
background-color: #f5f5f5; |
7 |
font-family: "DM Mono", monospace; |
8 |
}
|
9 |
.container { |
10 |
width: 90%; |
11 |
max-width: 600px; |
12 |
height: fit-content; |
13 |
display: flex; |
14 |
flex-direction: column; |
15 |
align-items: center; |
16 |
border-radius: 20px; |
17 |
background: white; |
18 |
}
|
19 |
header { |
20 |
width: 100%; |
21 |
text-align: center; |
22 |
}
|
Preview area:
1 |
.preview { |
2 |
position: relative; |
3 |
width: 350px; |
4 |
height: 300px; |
5 |
display: flex; |
6 |
align-items: center; |
7 |
justify-content: center; |
8 |
margin: 1rem 0; |
9 |
overflow: hidden; |
10 |
border: 2px dashed #e0e0e0; |
11 |
border-radius: 8px; |
12 |
}
|
Customize container
We have a customize container that houses the color picker and range elements. To ensure these elements are aligned neatly, set a maximum width to the customize container.
1 |
.customize-container { |
2 |
width: 100%; |
3 |
max-width: 300px; |
4 |
}
|
Color picker and range elements
Add these styles to ensure consistency in the color picker and range elements.
1 |
input[type="color"] { |
2 |
width: 100%; |
3 |
height: 40px; |
4 |
border: none; |
5 |
padding: 4px; |
6 |
border-radius: 8px; |
7 |
cursor: pointer; |
8 |
}
|
9 |
.slider-label, |
10 |
.customize-container, |
11 |
p { |
12 |
display: block; |
13 |
margin-bottom: 8px; |
14 |
font-size: 0.75rem; |
15 |
color: #403e3e; |
16 |
}
|
17 |
|
18 |
.slider { |
19 |
width: 100%; |
20 |
height: 4px; |
21 |
background: #e0e0e0; |
22 |
border-radius: 2px; |
23 |
appearance: none; |
24 |
outline: none; |
25 |
}
|
26 |
.slider-container { |
27 |
margin-bottom: 5px; |
28 |
width: 300px; |
29 |
min-width: 300px; |
30 |
}
|
To also ensure consistency in the appearance of range sliders across multiple browsers, let’s apply custom styles for both Chromium-based browsers and Firefox. In Chromium browsers, we use the -webkit-slider-thumb
pseudo-element, while in Firefox, we use the -moz-range-thumb
pseudo-element.
1 |
.slider { |
2 |
width: 100%; |
3 |
height: 4px; |
4 |
background: #e0e0e0; |
5 |
border-radius: 2px; |
6 |
appearance: none; |
7 |
outline: none; |
8 |
}
|
9 |
.slider-container { |
10 |
margin-bottom: 5px; |
11 |
width: 300px; |
12 |
min-width: 300px; |
13 |
}
|
14 |
.slider::-webkit-slider-thumb { |
15 |
appearance: none; |
16 |
width: 16px; |
17 |
height: 16px; |
18 |
background: #147ccb; |
19 |
border-radius: 50%; |
20 |
cursor: pointer; |
21 |
transition: transform 0.2s; |
22 |
}
|
23 |
|
24 |
.slider::-moz-range-thumb { |
25 |
width: 16px; |
26 |
height: 16px; |
27 |
background: #147ccb; |
28 |
border-radius: 50%; |
29 |
cursor: pointer; |
30 |
border: none; |
31 |
transition: transform 0.2s; |
32 |
}
|
Lastly, the download button will have the following styles.
1 |
#downloadBtn { |
2 |
margin-top: 10px; |
3 |
color: white; |
4 |
background-color: #147ccb; |
5 |
border: none; |
6 |
padding: 10px 20px; |
7 |
border-radius: 5px; |
8 |
cursor: pointer; |
9 |
}
|
Blob generation with JavaScript
Let’s dive in to the logic of creating blobs. But first, let’s get all the elements that need manipulation.
1 |
const canvas = document.getElementById("canvas"); |
2 |
const variationSlider = document.getElementById("variation-slider"); |
3 |
const complexitySlider = document.getElementById("complexitySlider"); |
4 |
const downloadBtn = document.getElementById("downloadBtn"); |
5 |
const colorPicker = document.getElementById("colorPicker"); |
Drawing with the canvas API
The Canvas API is a powerful tool for creating and manipulating 2D graphics on the browser. It allows us to draw different shapes, ranging from basic lines, circles, and rectangles to even more complex ones like hexagons and blobs.
To get started, we need to access the canvas’ 2D context which provides the properties and methods needed for drawing.
1 |
const ctx = canvas.getContext("2d"); |
The context acts as the surface on which graphics are drawn.
Some of the common methods for drawing from the canvas API include:
-
lineTo(x,y)
: This method draws a line from the current position to the specified x and y coordinates. -
rect(x,y, width, height)
: This method draws a rectangle at the specified position with the specified dimensions. -
quadraticCurveTo(cp1x, cp1y, x, y )
: This method creates a smooth curve using cp1x and cp1y as the control point and x and y as the end point. -
bezierCurveTo(cp1x, cp1x,cp1x,cp1x, x,y):
This method draws a sharp curve using the specified central and end points(x, y).
The quadraticCurveTo
method is perfect for creating smooth organic shapes. By connecting multiple random points with curves, we can generate blobs of varying complexity and shape.
For example , here is the code needed to generate a simple curve from one point to another.
1 |
ctx.moveTo(100, 100); |
2 |
ctx.quadraticCurveTo(200, 50, 300, 100); |
The points (100,100) represent the starting point and the curve is drawn to (300,100) with (200, 50) as the control point. The control point is the points to which the curve pulls towards.
Here is the generated curve:



To clarify things, here is an illustration showing how the control point pulls the curve towards it.



Generate Blobs with quadraticCurveTo method
Create a function called createBlob
.
1 |
const createBlob = () => {} |
Inside this function, we will do the following:
- Clear the canvas
- Generate an array of points based on variation and complexity values.
- Connect the points using curves with the
quadraticCurveTo
method.
Define a standard size for the blob and get the currently selected complexity and variation values .
1 |
const createBlob = () => { |
2 |
const color = colorPicker.value; |
3 |
const size = 100; |
4 |
const complexity = parseInt(complexitySlider.value); |
5 |
const variation = parseInt(variationSlider.value) / 100; |
6 |
|
7 |
}
|
Before we begin drawing, clear the canvas, load the currently selected color, and reset the current path to ensure no drawings are on the context’s active path.
1 |
ctx.clearRect(0, 0, canvas.width, canvas.height); |
2 |
ctx.fillStyle = color; |
3 |
ctx.beginPath(); |
Define the center of the canvas to ensure the blob is positioned symmetrically.
1 |
const centerX = canvas.width / 2; |
2 |
const centerY = canvas.height / 2; |
Define a value angleStep
that represents the separation in radians between each point on the blob. This value defines the shape of the blob. A higher complexity value results in more points around the center, hence making the blob more detailed. Smaller values will result in an almost circular-looking blob.
1 |
const angleStep = (Math.PI * 2) / complexity; |
This value, as you can see, is calculated by dividing the full circle (2 *PI radians) by the complexity value. Next, create an empty array called points for storing the blob’s coordinates.
1 |
const points = []; |
Create a for loop to iterate over the value of complexity
, which determines the number of points on the blob. At each iteration generate x
and y
coordinates for each point based on a randomly generated radius
. Then push each pair of coordinates to the points
array. At any given point, the array will contain different values for x and y which when drawn and connected will form a blob shape.
1 |
const points = []; |
2 |
for (let i = 0; i < complexity; i++) { |
3 |
const angle = i * angleStep; |
4 |
const radius = size * (1 + (Math.random() - 0.5) * variation); |
5 |
const x = centerX + radius * Math.cos(angle); |
6 |
const y = centerY + radius * Math.sin(angle); |
7 |
points.push([x, y]); |
8 |
}
|
To ensure a smooth transition on the start and endpoints of any path, we need to move the starting point to the midpoint of the first and last points;
1 |
ctx.moveTo( |
2 |
(points[0].x + points[points.length - 1].x) / 2, |
3 |
(points[0].y + points[points.length - 1].y) / 2 |
4 |
);
|
Finally draw the blob with quadraticCurveTo
method.
1 |
for (let i = 0; i < points.length; i++) { |
2 |
const [x1, y1] = points[i]; |
3 |
const [x2, y2] = points[(i + 1) % points.length]; |
4 |
const midX = (x1 + x2) / 2; |
5 |
const midY = (y1 + y2) / 2; |
6 |
ctx.quadraticCurveTo(x1, y1, midX, midY); |
7 |
}
|
Here we are using the coordinates in the points
array to create curves connecting between all the points. To ensure smooth curves between points, we have defined a midpoint between each pair of consecutive points. This midpoint acts as the control point ensuring the curve is smoothly pulled towards the center of the line connecting the 2 points.
And since the midpoint is placed between 2 points, this helps to form rounded blobs. Finally, invoke the createBlob
function
1 |
createBlob(); |
Invoking the createBlob
function will draw a blob matching the selected color , variation
and complexity
values. When these values change, the shape of the blob will also change.
1 |
colorPicker.addEventListener("input", createBlob); |
2 |
variationSlider.addEventListener("input", createBlob); |
3 |
complexitySlider.addEventListener("input", createBlob); |
Here is the final code for the createBlob
function.
1 |
const createBlob = () => { |
2 |
const color = colorPicker.value; |
3 |
const size = 100; |
4 |
const complexity = parseInt(complexitySlider.value); |
5 |
const variation = parseInt(variationSlider.value) / 100; |
6 |
|
7 |
ctx.clearRect(0, 0, canvas.width, canvas.height); |
8 |
ctx.fillStyle = color; |
9 |
ctx.beginPath(); |
10 |
|
11 |
const centerX = canvas.width / 2; |
12 |
const centerY = canvas.height / 2; |
13 |
const angleStep = (Math.PI * 2) / complexity; |
14 |
|
15 |
const points = []; |
16 |
|
17 |
for (let i = 0; i < complexity; i++) { |
18 |
const angle = i * angleStep; |
19 |
const radius = size * (1 + (Math.random() - 0.5) * variation); |
20 |
const x = centerX + radius * Math.cos(angle); |
21 |
const y = centerY + radius * Math.sin(angle); |
22 |
points.push([x, y]); |
23 |
}
|
24 |
|
25 |
ctx.moveTo( |
26 |
(points[0].x + points[points.length - 1].x) / 2, |
27 |
(points[0].y + points[points.length - 1].y) / 2 |
28 |
);
|
29 |
|
30 |
for (let i = 0; i < points.length; i++) { |
31 |
const [x1, y1] = points[i]; |
32 |
const [x2, y2] = points[(i + 1) % points.length]; |
33 |
const midX = (x1 + x2) / 2; |
34 |
const midY = (y1 + y2) / 2; |
35 |
ctx.quadraticCurveTo(x1, y1, midX, midY); |
36 |
}
|
37 |
|
38 |
ctx.closePath(); |
39 |
ctx.fill(); |
40 |
};
|
Download the blob
Now that we can change the shape, color, and complexity of the blob, let’s add the ability to download the blob for use. To achieve this, we will add a click event listener to the download button. When the button is clicked, the generated blob will be downloaded.
Luckily, the canvas API provides a simple way to download the entire canvas using the canvas.toDataURL
. This method will convert the canvas contents into a data URL of the image.
1 |
downloadBtn.addEventListener("click", () => { |
2 |
const link = document.createElement("a"); |
3 |
const dataURL = canvas.toDataURL("image/png"); |
4 |
link.download = "blob/png"; |
5 |
link.href = dataURL; |
6 |
link.click(); |
And we’re done!
Here is the final working demo.
This blob generator will give you the flexibility to create and customize blobs to suit your creative needs. But besides that, this has been a detailed and in-depth exercise in JavaScript—I hope you learned something!
This content originally appeared on Envato Tuts+ Tutorials and was authored by Esther Vaati
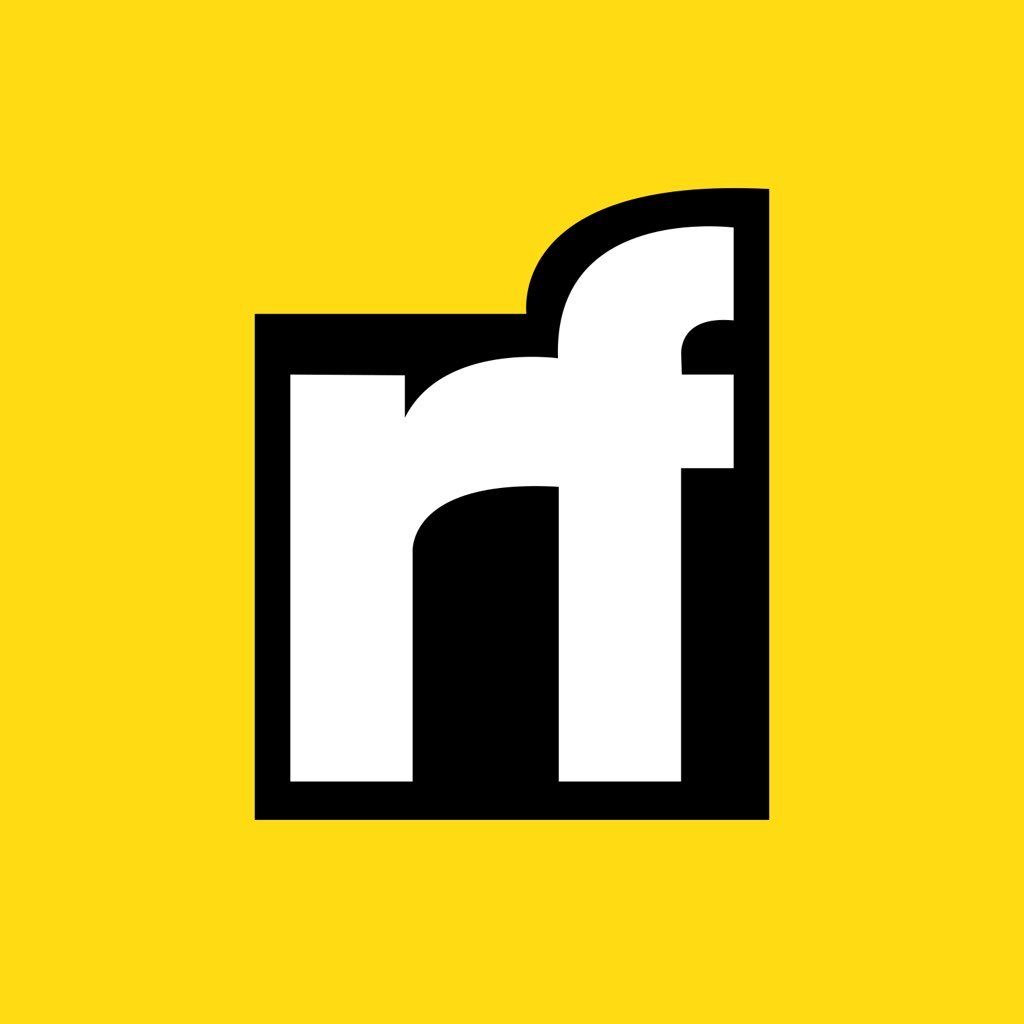
Esther Vaati | Sciencx (2025-01-20T19:14:57+00:00) How to create a “blob generator” tool in JavaScript (Canvas tutorial). Retrieved from https://www.scien.cx/2025/01/20/how-to-create-a-blob-generator-tool-in-javascript-canvas-tutorial/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.