This content originally appeared on DEV Community and was authored by 0x2e Tech
Let's build a robust Python REST API to fetch real-time stock data. This isn't theoretical; we're building something you can use today. We'll avoid fluff and focus on a practical, plug-and-play solution. This guide assumes you have some Python and REST API experience.
Step 1: Choosing Your Data Source
Forget free, unreliable APIs. You need a reliable source for trading. I recommend using a paid financial data provider like Alpha Vantage, Tiingo, or IEX Cloud. They offer robust APIs and sufficient data for algorithmic trading. Each has its own API key system; get yours before proceeding.
Step 2: Setting up Your Environment
- Install Necessary Libraries:
pip install requests flask python-dotenv
-
Create a
.env
file: This file will store your API key securely. Create a file named.env
in your project directory and add your API key. For example, if you're using Alpha Vantage:
ALPHA_VANTAGE_API_KEY=YOUR_API_KEY_HERE
Remember to replace YOUR_API_KEY_HERE
with your actual key. Ignore this step if you are using a different API.
Step 3: Building the Flask API
Now, let's create the API using Flask. This will be a simple API that takes a stock ticker as input and returns real-time data.
from flask import Flask, jsonify, request
import os
import requests
from dotenv import load_dotenv
app = Flask(__name__)
load_dotenv()
# Function to fetch data from your chosen API
def get_stock_data(symbol):
api_key = os.getenv('ALPHA_VANTAGE_API_KEY') #Remember to change this if you're using a different API
url = f'https://www.alphavantage.co/query?function=GLOBAL_QUOTE&symbol={symbol}&apikey={api_key}'
response = requests.get(url)
data = response.json()
return data
@app.route('/stock', methods=['GET'])
def get_stock():
symbol = request.args.get('symbol')
if not symbol:
return jsonify({'error': 'Symbol parameter is required'}), 400
try:
stock_data = get_stock_data(symbol)
return jsonify(stock_data)
except Exception as e:
return jsonify({'error': str(e)}), 500
if __name__ == '__main__':
app.run(debug=True)
Step 4: Running and Testing Your API
- Save the above code as
app.py
. - Run it from your terminal using:
python app.py
- Test it in your browser or using a tool like Postman by navigating to
http://127.0.0.1:5000/stock?symbol=AAPL
(replace AAPL with your desired stock symbol).
Step 5: Handling Errors Gracefully
The above code includes basic error handling. You'll want to expand on this. Consider:
- Input Validation: Validate that the
symbol
is in the correct format. - Rate Limiting: Implement rate limiting to prevent exceeding API limits.
- Network Errors: Handle potential network issues (e.g., timeouts).
- API Key Management: Securely manage your API keys (do not hardcode them into your code).
Step 6: Advanced Features
Once you have a working API, consider adding features like:
- Historical Data: Fetch historical stock prices.
- Technical Indicators: Calculate and return technical indicators (moving averages, RSI, etc.).
- Real-time Streaming: Use WebSockets for real-time updates.
- Database Integration: Store data in a database for persistence.
- Authentication: Add authentication and authorization to secure your API.
Important Considerations:
- Real-time Data is Expensive: Real-time financial data comes at a price. Factor in the cost of your data provider.
- API Limits: Be aware of your API provider's rate limits to avoid getting your API key blocked.
- Data Integrity: Always validate and sanitize data received from the API.
- Security: Security is paramount in any trading application. Protect your API keys and user data.
This detailed guide provides a foundation for building a robust REST API for real-time stock data. Remember to adapt it to your specific needs and chosen data provider. Good luck building your trading application!
This content originally appeared on DEV Community and was authored by 0x2e Tech
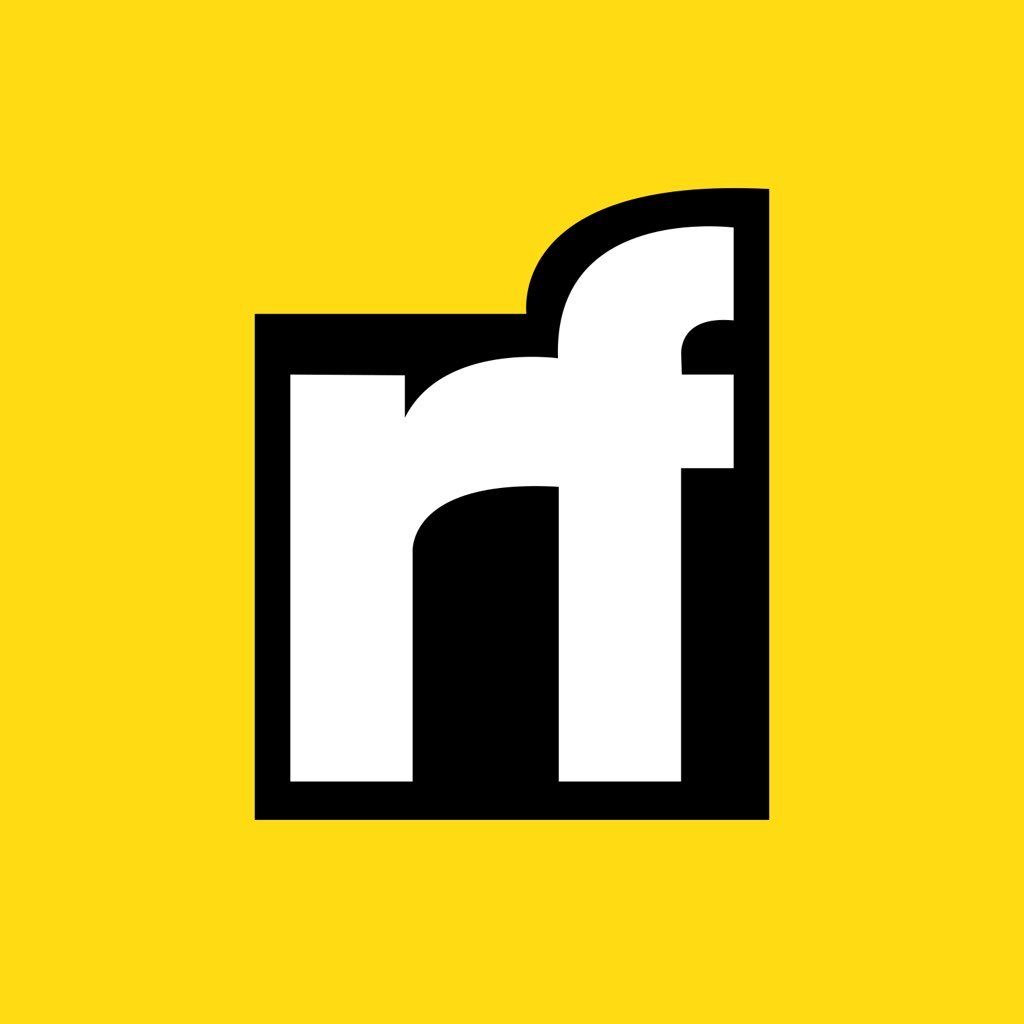
0x2e Tech | Sciencx (2025-01-26T18:53:18+00:00) Python REST API for Real-time Stock Data: A Trader’s Guide. Retrieved from https://www.scien.cx/2025/01/26/python-rest-api-for-real-time-stock-data-a-traders-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.