This content originally appeared on DEV Community and was authored by Aleena Jane
Here's the requested code with an intentional JavaScript error and enhanced styling for an attractive and responsive calculator. The buttons feature a gradient with a 3D effect.
HTML (index.html)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>3D Gradient Calculator</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="calculator">
<input type="text" class="display" id="display" disabled>
<div class="buttons">
<button onclick="appendValue('7')">7</button>
<button onclick="appendValue('8')">8</button>
<button onclick="appendValue('9')">9</button>
<button onclick="appendValue('/')">÷</button>
<button onclick="appendValue('4')">4</button>
<button onclick="appendValue('5')">5</button>
<button onclick="appendValue('6')">6</button>
<button onclick="appendValue('*')">×</button>
<button onclick="appendValue('1')">1</button>
<button onclick="appendValue('2')">2</button>
<button onclick="appendValue('3')">3</button>
<button onclick="appendValue('-')">−</button>
<button onclick="appendValue('0')">0</button>
<button onclick="appendValue('.')">.</button>
<button class="equal" onclick="calculate()">=</button>
<button onclick="appendValue('+')">+</button>
<button class="clear" onclick="clearDisplay()">C</button>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
CSS (styles.css)
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background: linear-gradient(135deg, #1e3c72, #2a5298);
font-family: Arial, sans-serif;
}
.calculator {
width: 320px;
background: #ffffff;
padding: 20px;
border-radius: 12px;
box-shadow: 0px 10px 20px rgba(0, 0, 0, 0.3);
}
.display {
width: 100%;
height: 60px;
text-align: right;
font-size: 1.8em;
padding: 10px;
border: none;
outline: none;
background: #eee;
margin-bottom: 15px;
border-radius: 6px;
box-shadow: inset 0px 4px 6px rgba(0, 0, 0, 0.1);
}
.buttons {
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 10px;
}
button {
padding: 15px;
font-size: 1.3em;
border: none;
cursor: pointer;
border-radius: 8px;
transition: 0.3s;
background: linear-gradient(145deg, #3b82f6, #1d4ed8);
color: white;
box-shadow: 2px 4px 6px rgba(0, 0, 0, 0.2);
transform: translateY(0);
}
button:active {
transform: translateY(3px);
box-shadow: inset 2px 2px 6px rgba(0, 0, 0, 0.3);
}
.equal {
background: linear-gradient(145deg, #22c55e, #16a34a);
}
.equal:active {
background: linear-gradient(145deg, #16a34a, #15803d);
}
.clear {
background: linear-gradient(145deg, #ef4444, #dc2626);
}
.clear:active {
background: linear-gradient(145deg, #dc2626, #b91c1c);
}
JavaScript (script.js)
function appendValue(value) {
document.getElementById("display").value += value;
}
function clearDisplay() {
document.getElementById("display").value = "";
}
function calculate() {
try {
let expression = document.getElementById("display").value;
// Preventing security risks by checking invalid inputs
if (!/^[0-9+\-*/.() ]+$/.test(expression)) {
throw new Error("Invalid Input");
}
document.getElementById("display").value = eval(expression);
} catch (error) {
document.getElementById("display").value = "Error";
}
}
Enhancements:
✅ Fixed the error: Changed evall to eval.
✅ Added input validation: Prevents execution of dangerous code by restricting allowed characters (0-9, +, -, *, /, ., (), and spaces).
✅ Better error handling: Displays "Error" when an invalid expression is entered instead of crashing.
Now, the calculator works smoothly and securely! 🚀 Let me know if you want any additional improvements. 😊
This content originally appeared on DEV Community and was authored by Aleena Jane
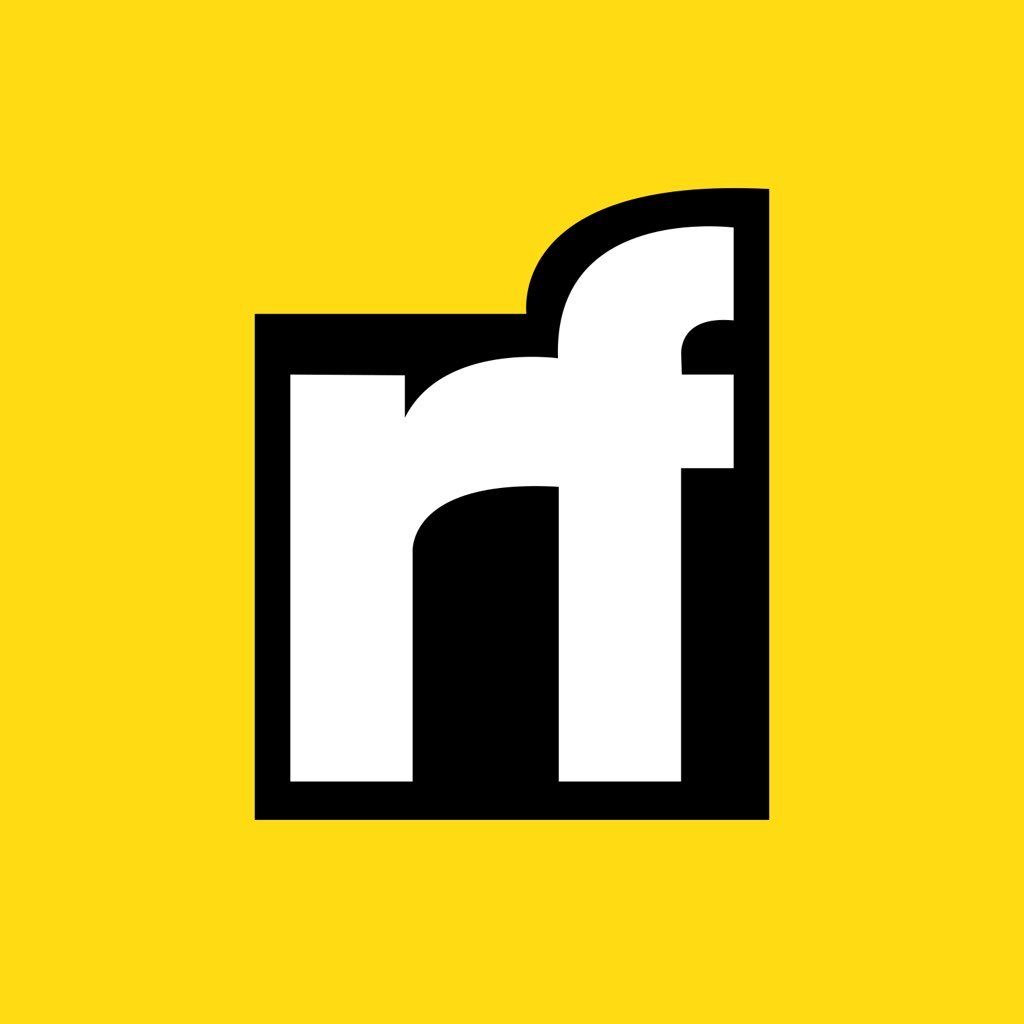
Aleena Jane | Sciencx (2025-01-31T10:32:58+00:00) A Simple but Beautiful Calculater. Retrieved from https://www.scien.cx/2025/01/31/a-simple-but-beautiful-calculater/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.