This content originally appeared on DEV Community and was authored by Sico Spain
Managing finances is a critical task for businesses and individuals alike. Whether you're tracking expenses, recording transactions, or generating financial reports, having a reliable accounting system is essential. In this post, I'll walk you through building a Busy Accounting Software in Python—a simple yet powerful tool to handle basic accounting tasks.
Why Build an Accounting Software?
Accounting software helps automate financial tasks, reduces errors, and provides insights into financial health. While there are many off-the-shelf solutions, building your own software allows you to customize it to your specific needs. Plus, it's a great way to learn Python and software design!
Features of Busy Accounting Software
Our Busy Accounting Software will include the following features:
Account Management: Create and manage multiple accounts (e.g., Cash, Bank, Revenue).
Transaction Recording: Record deposits, withdrawals, and transfers between accounts.
Balance Calculation: Automatically calculate the balance for each account.
Transaction History: Maintain a log of all transactions for auditing and reporting.
Report Generation: Generate a summary of accounts, balances, and transaction history.
How It Works
The software is built using Python and follows an object-oriented design. Here's a breakdown of the key components:
- Account Class The Account class represents a single account. It stores the account name, balance, and transaction history. It also provides methods for depositing, withdrawing, and transferring funds.
python
Copy
class Account:
def init(self, name, initial_balance=0):
self.name = name
self.balance = initial_balance
self.transactions = []
def deposit(self, amount):
if amount <= 0:
raise ValueError("Amount must be positive.")
self.balance += amount
self.transactions.append(f"Deposited: +{amount}")
def withdraw(self, amount):
if amount <= 0:
raise ValueError("Amount must be positive.")
if amount > self.balance:
raise ValueError("Insufficient balance.")
self.balance -= amount
self.transactions.append(f"Withdrew: -{amount}")
def transfer(self, amount, target_account):
if amount <= 0:
raise ValueError("Amount must be positive.")
if amount > self.balance:
raise ValueError("Insufficient balance.")
self.withdraw(amount)
target_account.deposit(amount)
self.transactions.append(f"Transferred: -{amount} to {target_account.name}")
target_account.transactions.append(f"Received: +{amount} from {self.name}")
def get_balance(self):
return self.balance
def get_transaction_history(self):
return self.transactions
- AccountingSoftware Class The AccountingSoftware class manages all accounts and provides functionality to create accounts, retrieve accounts, and generate reports.
python
Copy
class AccountingSoftware:
def init(self):
self.accounts = {}
def create_account(self, name, initial_balance=0):
if name in self.accounts:
raise ValueError("Account already exists.")
self.accounts[name] = Account(name, initial_balance)
print(f"Account '{name}' created with an initial balance of {initial_balance}.")
def get_account(self, name):
if name not in self.accounts:
raise ValueError("Account does not exist.")
return self.accounts[name]
def generate_report(self):
print("\n--- Accounting Report ---")
for account_name, account in self.accounts.items():
print(f"\nAccount: {account_name}")
print(f"Balance: {account.get_balance()}")
print("Transaction History:")
for transaction in account.get_transaction_history():
print(f" - {transaction}")
print("\n--- End of Report ---")
Example Usage
Here's how you can use the Busy Accounting Software:
python
Copy
if name == "main":
software = AccountingSoftware()
# Create accounts
software.create_account("Cash", initial_balance=1000)
software.create_account("Bank", initial_balance=5000)
software.create_account("Revenue", initial_balance=0)
# Perform transactions
cash_account = software.get_account("Cash")
bank_account = software.get_account("Bank")
revenue_account = software.get_account("Revenue")
cash_account.deposit(500)
cash_account.withdraw(200)
cash_account.transfer(300, bank_account)
revenue_account.deposit(1000)
# Generate report
software.generate_report()
Sample Output
When you run the code, you'll get a detailed report like this:
Copy
Account 'Cash' created with an initial balance of 1000.
Account 'Bank' created with an initial balance of 5000.
Account 'Revenue' created with an initial balance of 0.
--- Accounting Report ---
Account: Cash
Balance: 1000
Transaction History:
- Deposited: +500
- Withdrew: -200
- Transferred: -300 to Bank
Account: Bank
Balance: 5300
Transaction History:
- Received: +300 from Cash
Account: Revenue
Balance: 1000
Transaction History:
- Deposited: +1000
--- End of Report ---
How to Expand the Software
This is just the beginning! Here are some ideas to make the software even more powerful:
Persistent Storage: Save accounts and transactions to a database (e.g., SQLite or PostgreSQL).
User Interface: Build a GUI using tkinter or a web-based interface using Flask or Django.
Advanced Reporting: Generate PDF reports or charts using libraries like ReportLab or matplotlib.
Multi-Currency Support: Add support for handling transactions in different currencies.
Authentication: Implement user authentication to secure the software.
Conclusion
Building a Busy Accounting Software in Python is a great way to learn about object-oriented programming, financial management, and software design. This project is simple yet powerful, and it can be expanded to meet your specific needs. Whether you're a beginner or an experienced developer, I hope this post inspires you to create your own accounting tool!
This content originally appeared on DEV Community and was authored by Sico Spain
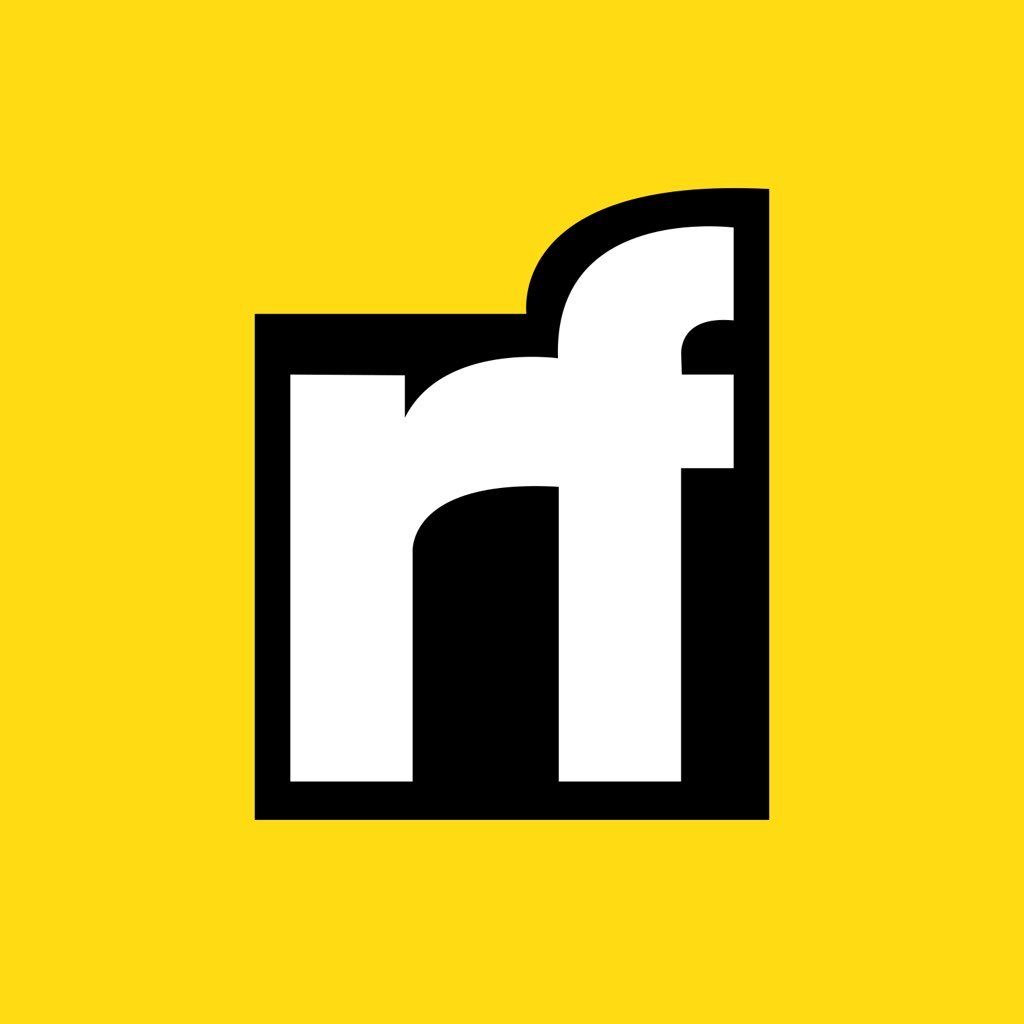
Sico Spain | Sciencx (2025-01-31T09:35:16+00:00) Building a Busy 21 Rel 12 Accounting Software in Business. Retrieved from https://www.scien.cx/2025/01/31/building-a-busy-21-rel-12-accounting-software-in-business/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.