This content originally appeared on DEV Community and was authored by Kush Parsaniya
Java Record Classes
Java Record Classes were introduced in Java 14 and later made a standard feature in Java 16. They simplify the process of creating classes that mainly hold data.
Why Do We Need Record Classes?
Record classes were introduced to overcome the boilerplate code associated with creating simple data holder classes in Java.
Defining a Record Class
A record class is defined using the record
keyword followed by the name of the record and a parameter list.
public record Person(String name, int age) {}
Key Features of Record Classes
Record classes have the following key features:
- They are final and cannot be subclassed.
- They can have private fields, but they are not required.
- They have a canonical constructor, getters, and
toString
,equals
, andhashCode
methods that are generated by the compiler.
public record Person(String name, int age) {
@Override
public String toString() {
return "Person[name=" + name + ", age=" + age + "]";
}
}
Advantages of Record Classes
Record classes have several advantages over traditional classes:
- They reduce boilerplate code.
- They improve code readability.
- They improve code maintainability.
Example Use Case
Record classes are useful when you need to create simple data holder classes.
public record Address(String street, String city, String state, String zip) {}
public record Person(String name, int age, Address address) {
public static void main(String[] args) {
Person person = new Person("John Doe", 30, new Address("123 Main St", "Anytown", "CA", "12345"));
System.out.println(person);
}
}
Conclusion
Java Record Classes are a powerful feature that simplifies the process of creating simple data holder classes. They reduce boilerplate code, improve code readability, and improve code maintainability.
Share Your Thoughts
What do you think about Java Record Classes? Have you used them in your projects? Share your thoughts in the comments below.
This content originally appeared on DEV Community and was authored by Kush Parsaniya
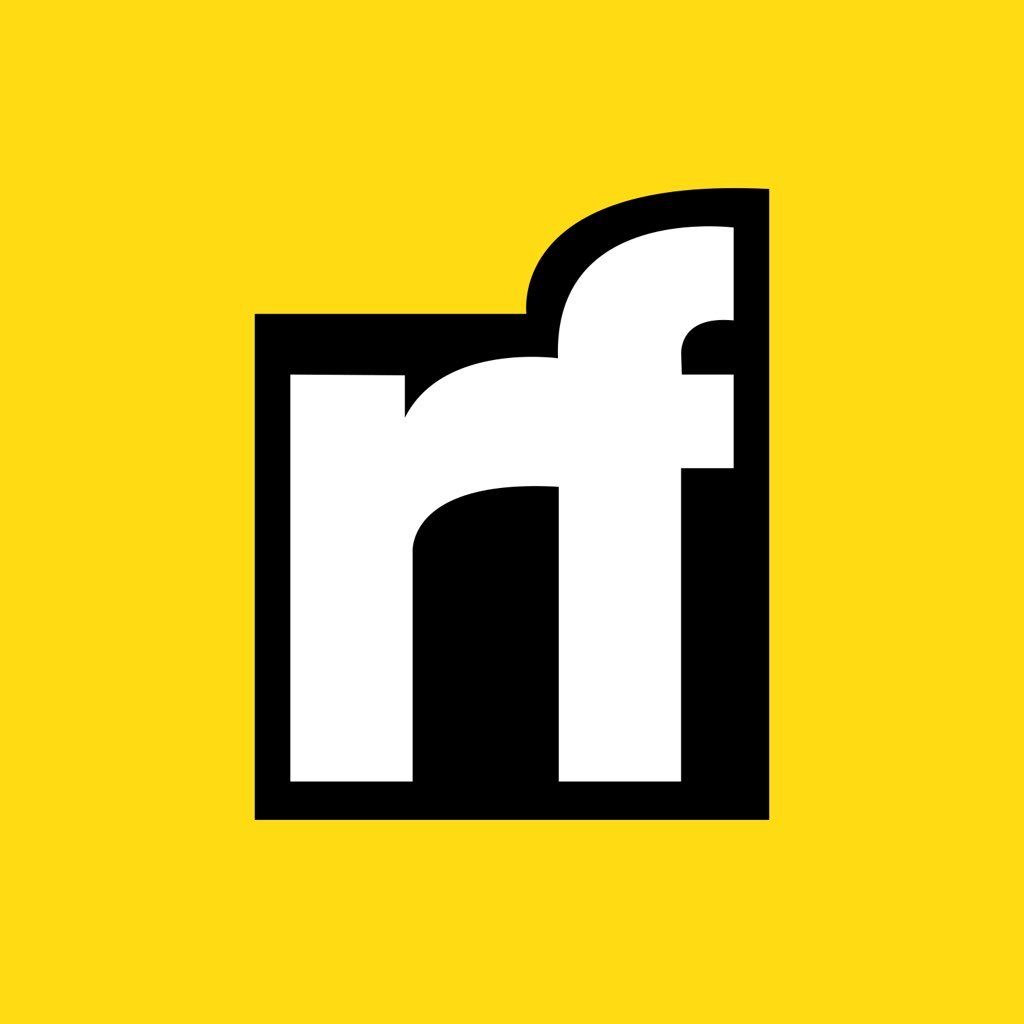
Kush Parsaniya | Sciencx (2025-02-01T07:07:32+00:00) Simplifying Data Holder Classes with Java Record Classes. Retrieved from https://www.scien.cx/2025/02/01/simplifying-data-holder-classes-with-java-record-classes/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.