This content originally appeared on DEV Community and was authored by abdessamad idboussadel
On my journey of learning the basics of Linux and server management essential skills that every developer should have, even front-end ones.I’ve taken notes that might help others as well. In this blog, I’ll be sharing my insights on setting up an Apache server with PHP on Linux. If you find this helpful, let me know—I have plenty more to share! 😊
Let's begin by running the following command to install the necessary packages:
Run the following command to install the necessary packages:
sudo apt update && sudo apt upgrade -y
sudo apt install apache2 mysql-server php libapache2-mod-php php-mysql -y
1. Why php-mysql
and not just mysql
?
-
php-mysql
is a PHP extension that allows PHP to communicate with MySQL databases. - Without
php-mysql
, PHP scripts won't be able to interact with MySQL.
2. What is libapache2-mod-php
?
-
libapache2-mod-php
is an Apache module that enables PHP processing within the Apache web server. - It ensures that Apache can handle
.php
files and execute PHP scripts.
then run the following commands:
sudo systemctl start apache2
sudo systemctl enable apache2
sudo systemctl start mysql
sudo systemctl enable mysql
sudo systemctl start apache2
- Starts the Apache service immediately.
- However, it will not start automatically on reboot.
sudo systemctl enable apache2
- Enables the Apache service to start automatically at boot.
Configure the MySQL Database
Run the MySQL security script to secure your database:
sudo mysql_secure_installation
- Set a root password
- Remove anonymous users
- Disallow remote root login
- Remove test databases
- Reload privilege tables
Then, log in to MySQL and create a database:
sudo mysql -u root -p
Inside MySQL:
CREATE DATABASE mydatabase;
CREATE USER 'abdessamad'@'%' IDENTIFIED BY '123456789';
# Creates a new MySQL user myuser with the password mypassword.
# The @'%' part allows the user to connect from any host (% is a wildcard representing any IP address).
GRANT ALL PRIVILEGES ON mydatabase.* TO 'abdessamad'@'%';
# Refreshes the MySQL server's internal cache of user privileges. This is necessary after creating users or changing privileges to apply the changes.
FLUSH PRIVILEGES;
EXIT;
Configure Apache to Serve Your PHP Site
Move your PHP files to the web root directory:
sudo rm -rf /var/www/html/*
sudo nano /var/www/html/index.php
Add this test PHP code:
<?php
echo "its finaly working ..."
?>
Save and exit.
Set proper permissions:
# Change ownership of all files and directories in /var/www/html recursively
# The user and group ownership is set to 'www-data' which is the default user/group for web servers (Nginx/Apache)
sudo chown -R www-data:www-data /var/www/html
# - Owner (www-data) has read, write, and execute permissions (7)
# - Group (www-data) and Others have read and execute permissions, but no write permissions
sudo chmod -R 755 /var/www/html
Restart Apache:
sudo systemctl restart apache2
it should be working on the localhost now :
6. Configure Apache for Network Access
Edit the default Apache config:
sudo nano /etc/apache2/sites-available/000-default.conf
Find DocumentRoot /var/www/html
and add:
<VirtualHost *:80>
DocumentRoot /var/www/html
<Directory /var/www/html>
AllowOverride All
Require all granted
</Directory>
</VirtualHost>
Save and exit.
Allow traffic through the firewall:
sudo ufw allow 80/tcp
sudo ufw allow 3306/tcp
sudo ufw reload
Enable mod_rewrite
:
sudo a2enmod rewrite
sudo systemctl restart apache2
now check your server ip using :
ip a
now you can access it outside the vm and access on the network :
If it’s not working, or if you want to access the server from outside the local machine on the network, try using a bridged network instead of NAT in VMware or VirtualBox. This will allow you to use a local IP address (e.g., 192.x.x.x) instead of a private IP.
if your apache server and mysql will be on separate servers then you need to :
Allow Remote MySQL Access
Edit the MySQL config file:
sudo nano /etc/mysql/mysql.conf.d/mysqld.cnf
Find bind-address = 127.0.0.1
and change it to:
bind-address = 0.0.0.0
Save and exit (CTRL + X
, then Y
, then ENTER
).Now any one can access your db remotely.
Restart MySQL:
sudo systemctl restart mysql
Now lets test it with dynamic php page :
sudo mysql -u root -p
CREATE DATABASE mydatabase;
USE mydatabase;
CREATE TABLE books (
id INT AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255) NOT NULL,
author VARCHAR(255) NOT NULL,
genre VARCHAR(100),
published_date DATE,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
<?php
$servername = "localhost";
$username = "myuser";
$password = "mypassword";
$dbname = "mydatabase";
$conn = new mysqli($servername, $username, $password, $dbname);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$title = $_POST['title'];
$author = $_POST['author'];
$genre = $_POST['genre'];
$published_date = $_POST['published_date'];
$sql = "INSERT INTO books (title, author, genre, published_date)
VALUES ('$title', '$author', '$genre', '$published_date')";
if ($conn->query($sql) === TRUE) {
echo "New book added successfully";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
}
// Fetching all books
$sql = "SELECT id, title, author, genre, published_date FROM books";
$result = $conn->query($sql);
$conn->close();
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Create a New Book</title>
</head>
<body>
<h1>Create a New Book</h1>
<form action="index.php" method="POST">
<label for="title">Book Title:</label>
<input type="text" id="title" name="title" required><br><br>
<label for="author">Author:</label>
<input type="text" id="author" name="author" required><br><br>
<label for="genre">Genre:</label>
<input type="text" id="genre" name="genre"><br><br>
<label for="published_date">Published Date:</label>
<input type="date" id="published_date" name="published_date"><br><br>
<input type="submit" value="Create Book">
</form>
<h2>Book List</h2>
<?php
if ($result->num_rows > 0) {
echo "<table border='1'>
<tr>
<th>Title</th>
<th>Author</th>
<th>Genre</th>
<th>Published Date</th>
</tr>";
while($row = $result->fetch_assoc()) {
echo "<tr>
<td>" . $row["title"] . "</td>
<td>" . $row["author"] . "</td>
<td>" . $row["genre"] . "</td>
<td>" . $row["published_date"] . "</td>
</tr>";
}
echo "</table>";
} else {
echo "No books found";
}
?>
</body>
</html>
now lets set up ssh :
sudo apt install openssh-server
sudo systemctl start ssh
sudo ufw allow ssh
sudo ufw reload
ssh-keygen -t rsa -b 4096 -C "[your_email@example.com](mailto:your_email@example.com)"
# to get your server username or create a new user if you want with sudo useradd -m -s /bin/bash newusername
whoami
# to get your server ip
hostname -I
ssh-copy-id username@server_ip
then try :
ssh username@server_ip
Conclusion
By following these steps, you now have a fully functional web server capable of handling PHP applications, managing database interactions, and enabling secure remote access via SSH. This setup not only supports development but also lays the foundation for deploying real-world applications.
If you found this guide helpful, let me know! There are plenty more notes and articles on various topics to help you take your programming skills to the next level. I’d be happy to share more insights! 🚀
This content originally appeared on DEV Community and was authored by abdessamad idboussadel
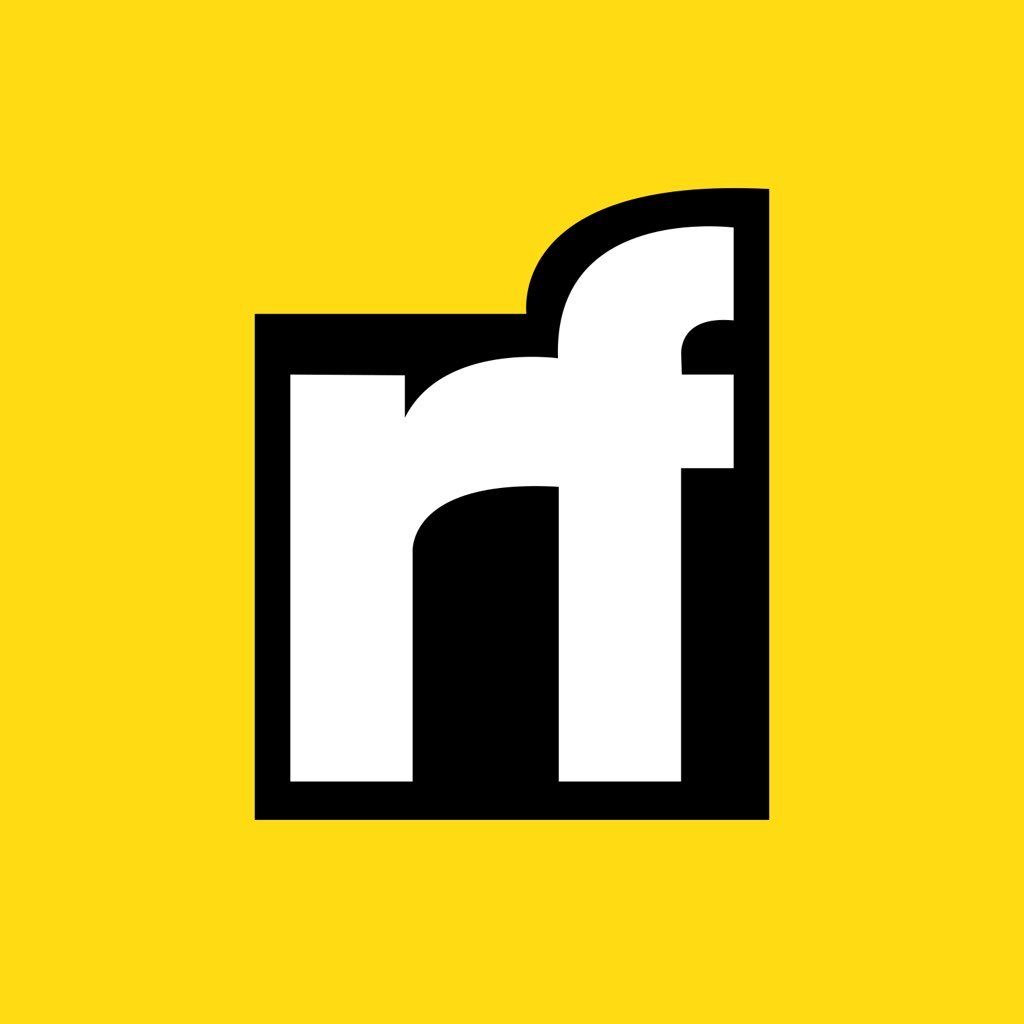
abdessamad idboussadel | Sciencx (2025-02-09T22:49:13+00:00) Setting Up an Apache Server with PHP and SSH on Linux. Retrieved from https://www.scien.cx/2025/02/09/setting-up-an-apache-server-with-php-and-ssh-on-linux/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.