This content originally appeared on DEV Community and was authored by Simon Green
Weekly Challenge 307
Each week Mohammad S. Anwar sends out The Weekly Challenge, a chance for all of us to come up with solutions to two weekly tasks. My solutions are written in Python first, and then converted to Perl. It's a great way for us all to practice some coding.
Task 1: Check Order
Task
You are given an array of integers, @ints
.
Write a script to re-arrange the given array in an increasing order and return the indices where it differs from the original array.
My solution
Both this week's tasks a pretty straight forward so don't require too much explanation. For this task, I create a new list (array in Perl) called sorted_ints
. Then then iterate through the list indexes to see if the value at this position is different in the two lists. If it is I add it to the difference
list.
def check_order(ints: list) -> str:
sorted_ints = sorted(ints)
differences = []
for idx in range(len(ints)):
if ints[idx] != sorted_ints[idx]:
differences.append(idx)
return differences
Examples
$ ./ch-1.py 5 2 4 3 1
[0, 2, 3, 4]
$ ./ch-1.py 1 2 1 1 3
[1, 3]
$ ./ch-1.py 3 1 3 2 3
[0, 1, 3]
Task 2: Find Anagrams
Task
You are given a list of words, @words
.
Write a script to find any two consecutive words and if they are anagrams, drop the first word and keep the second. You continue this until there is no more anagrams in the given list and return the count of final list.
My solution
For this task I create a list called sorted_words
which has each word with the letters sorted alphabetically and ignoring the case. I then iterate through the index from one to one less than the length of the list. If the sorted word at this position is the same as the previous one, I add one to the anagrams
counter variable.
As I want the number of words not removed, I subtract the anagrams
variable from the length of the list.
def find_anagrams(words: list) -> list:
sorted_words = [''.join(sorted(word.lower())) for word in words]
anagrams = 0
for idx in range(1, len(words)):
if sorted_words[idx-1] == sorted_words[idx]:
anagrams += 1
return len(words) - anagrams
Examples
$ ./ch-2.py acca dog god perl repl
3
$ ./ch-2.py abba baba aabb ab ab
2
This content originally appeared on DEV Community and was authored by Simon Green
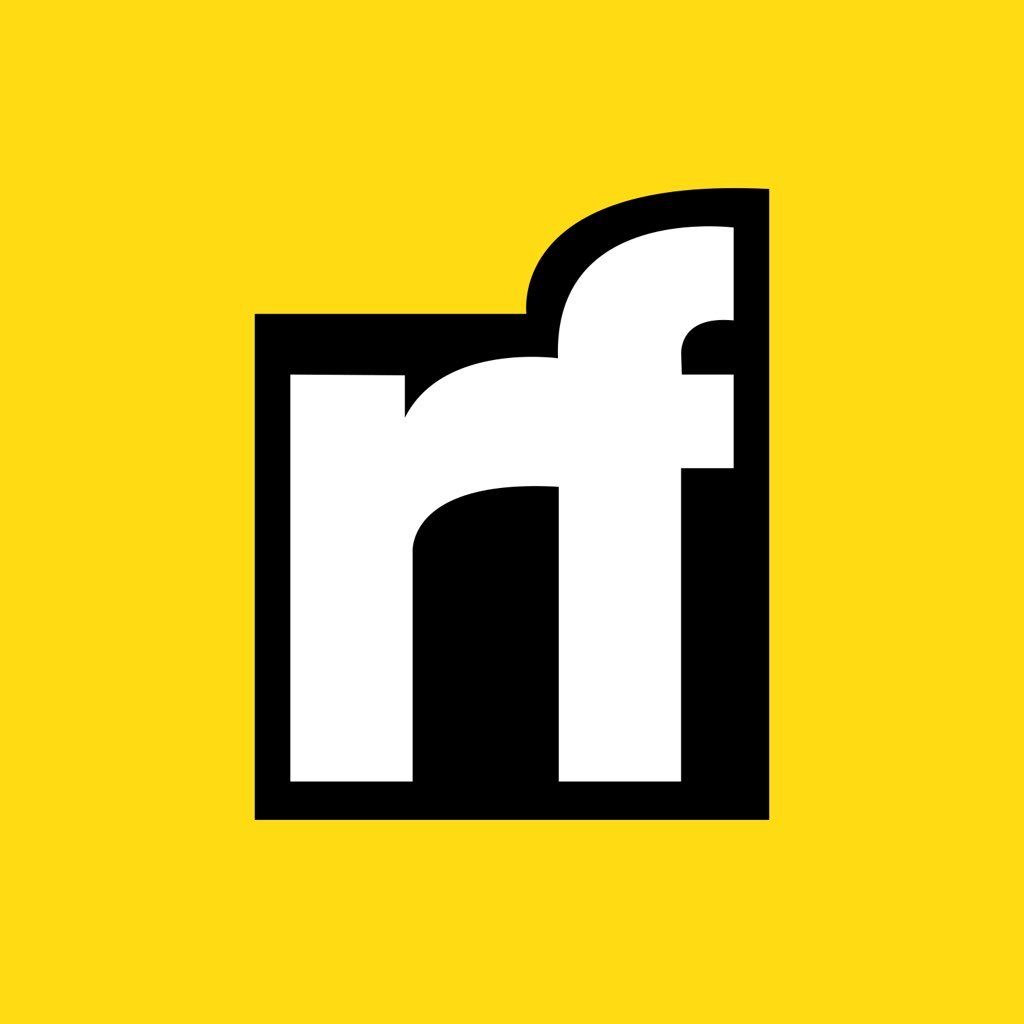
Simon Green | Sciencx (2025-02-09T03:07:08+00:00) Weekly Challenge: Sorting and counting. Retrieved from https://www.scien.cx/2025/02/09/weekly-challenge-sorting-and-counting/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.