This content originally appeared on DEV Community and was authored by IgorSusmelj
Hey Dev Community!
As a follow-up to my previous introduction to RustyNum, I want to share a developer-focused update about what I’ve been working on these last few weeks. RustyNum, as you might recall, is my lightweight, Rust-powered alternative to NumPy published on GitHub under MIT license. It uses Rust’s portable SIMD features for faster numerical computations, while staying small (around ~300kB for the Python wheel). In this post, I’ll explore a few insights gained during development, point out where it really helps, and highlight recent additions to the documentation and tutorials.
Brief Recap
If you missed the initial announcement, RustyNum focuses on:
- High performance using Rust’s SIMD
- Memory safety in Rust, without GC overhead
- Small distribution size (much smaller than NumPy wheels)
- NumPy-like interface to reduce friction for Python users
For a more detailed overview, head over to the official RustyNum website or check out my previous post on dev.to.
Developer’s Perspective: What’s New?
1. Working with Matrix Operations
I’ve spent a good chunk of time ensuring matrix operations feel familiar. Being able to do something like matrix-vector or matrix-matrix multiplication with minimal code changes from NumPy was a primary goal. A highlight is the .dot()
function and the @
operator, which both support these operations.
Check out the dedicated tutorial:
Better Matrix Operations with RustyNum
Here’s a quick snippet:
import rustynum as rnp
matrix = rnp.NumArray([i for i in range(16)], dtype=“float32”).reshape([4, 4])
vector = rnp.NumArray([1, 2, 3, 4], dtype=“float32”)
# Use the dot function
result_vec = matrix.dot(vector)
print(“Matrix-Vector Multiplication Result:”, result_vec)
It’s neat to see how close this is to NumPy’s workflow. Benchmarks suggest RustyNum can often handle these tasks at speeds comparable to, and sometimes faster than, NumPy on smaller or medium-sized datasets. For very large matrices, I’m still optimizing the approach.
2. Speeding Up Common Analytics Tasks
Apart from matrix multiplications, I’ve kept refining operations like mean()
, min()
, max()
, and dot()
. These straightforward methods are prime candidates for SIMD acceleration. There’s also a tutorial on how to replace specific NumPy calls with RustyNum for analytics, which might be useful if you’re bottlenecked by Python loops.
Example:
import numpy as np
import rustynum as rnp
# Generate test data
data_np = np.random.rand(1_000_000).astype(np.float32)
data_rn = rnp.NumArray(data_np.tolist(), dtype=“float32”)
# NumPy approach
mean_np = data_np.mean()
# RustyNum approach
mean_rn = data_rn.mean().item()
print(“NumPy mean:”, mean_np)
print(“RustyNum mean:”, mean_rn)
The Python overhead can sometimes offset the raw Rust speed, but in many cases, RustyNum still shows advantages.
New Tutorials: Real-World Examples
One of the best ways to see RustyNum in action is through practical examples. I’ve added several new tutorials with real-world coding scenarios:
- Better Matrix Operations – Focus on dot products, matrix-vector, and matrix-matrix tasks.
- Replacing Core NumPy Calls – Demonstrates how to switch from NumPy’s mean, min, dot to RustyNum.
- Streamlining ML Preprocessing – Explores scaling, normalization, and feature engineering for machine learning.
The last tutorial is a personal favorite. It covers the typical data transformations you’d do in a machine learning pipeline—just swapping out NumPy calls for RustyNum.
Check out a snippet of scaling code from that guide:
def min_max_scale(array):
col_mins = []
col_maxes = []
for col_idx in range(array.shape[1]):
col_data = array[:, col_idx]
col_mins.append(col_data.min())
col_maxes.append(col_data.max())
scaled_data = []
for col_idx in range(array.shape[1]):
col_data = array[:, col_idx]
numerator = col_data - col_mins[col_idx]
denominator = col_maxes[col_idx] - col_mins[col_idx] or 1.0
scaled_col = numerator / denominator
scaled_data.append(scaled_col.tolist())
return rnp.concatenate(
[rnp.NumArray(col, dtype="float32").reshape([array.shape[0], 1]) for col in scaled_data],
axis=1
)
It’s a small snippet, but it shows how RustyNum can do row/column manipulations quite effectively. After scaling, you can still feed the data into your favorite machine learning frameworks. The overhead of converting RustyNum arrays back into NumPy or direct arrays is minimal compared to the cost of big model training steps.
Ongoing Work
1. Large Matrix Optimizations
I’ve noticed that for very large matrices (like 10k×10k), RustyNum’s current code paths aren’t yet fully optimized compared to NumPy. This area remains an active project. RustyNum is still young, and I’m hoping to introduce further parallelization or block-based multiplication techniques for better large-scale performance.
2. Expanded Data Types
RustyNum supports float32 and float64 well, plus some integer types. I’m considering adding stronger integer support for data science tasks like certain indexing or small transformations. Meanwhile, advanced data types (e.g., complex numbers) might appear further down the line if the community needs them.
3. Documentation and API Enhancements
The docs site at rustynum.com has an API reference and a roadmap. I’m continuously adding to it. If you spot anything missing or if you have a specific use case in mind, feel free to open a GitHub issue or submit a pull request.
4. The big goal of Rustynum
RustyNum is simply a learning exercise for me to combine Rust and Python. Since I spend every day around machine learning I would love to have RustyNum replace part of my daily Numpy routines. And we're slowly getting there. I started adding more and more methods around the topic of how to integrate RustyNum in ML pipelines.
Quick Code Example: ML Integration
To demonstrate how RustyNum fits into a data pipeline, here’s a condensed example:
import numpy as np
import rustynum as rnp
from sklearn.linear_model import LogisticRegression
# 1) Create synthetic data in NumPy
train_np = np.random.rand(1000, 10).astype(np.float32)
labels_np = np.random.randint(0, 2, size=1000)
# 2) Convert to RustyNum for fast scaling
train_rn = rnp.NumArray(train_np.flatten().tolist(), dtype="float32").reshape([1000, 10])
# Basic scaling (compute min and max per column)
scaled_rn = []
for col_idx in range(train_rn.shape[1]):
col_data = train_rn[:, col_idx]
mn = col_data.min()
mx = col_data.max()
rng = mx - mn if (mx != mn) else 1.0
scaled_col = (col_data - mn) / rng
scaled_rn.append(scaled_col.tolist())
train_scaled_rn = rnp.concatenate(
[rnp.NumArray(col, dtype="float32").reshape([1000, 1]) for col in scaled_rn],
axis=1
)
# 3) Convert back to NumPy for scikit-learn
train_scaled_np = np.array(train_scaled_rn.tolist(), dtype=np.float32)
# 4) Train a logistic regression model
model = LogisticRegression()
model.fit(train_scaled_np, labels_np)
print("Model Coefficients:", model.coef_)
This script highlights that RustyNum can handle data transformations with a Pythonic feel, after which you can pass the arrays into other libraries.
Final Thoughts
It’s been fun to expand RustyNum’s features and see how well Rust can integrate with Python for high-performance tasks. The recent tutorials are a window into how RustyNum might replace parts of NumPy in data science or ML tasks, especially when smaller array sizes or mid-range tasks are involved.
- Check out the tutorials at rustynum.com
- Contribute or report issues on GitHub
- Share feedback if there’s a feature you’d love to see
Thanks for tuning in to this developer-focused update, and I look forward to hearing how RustyNum helps you in your own projects!
Happy Coding!
Igor
This content originally appeared on DEV Community and was authored by IgorSusmelj
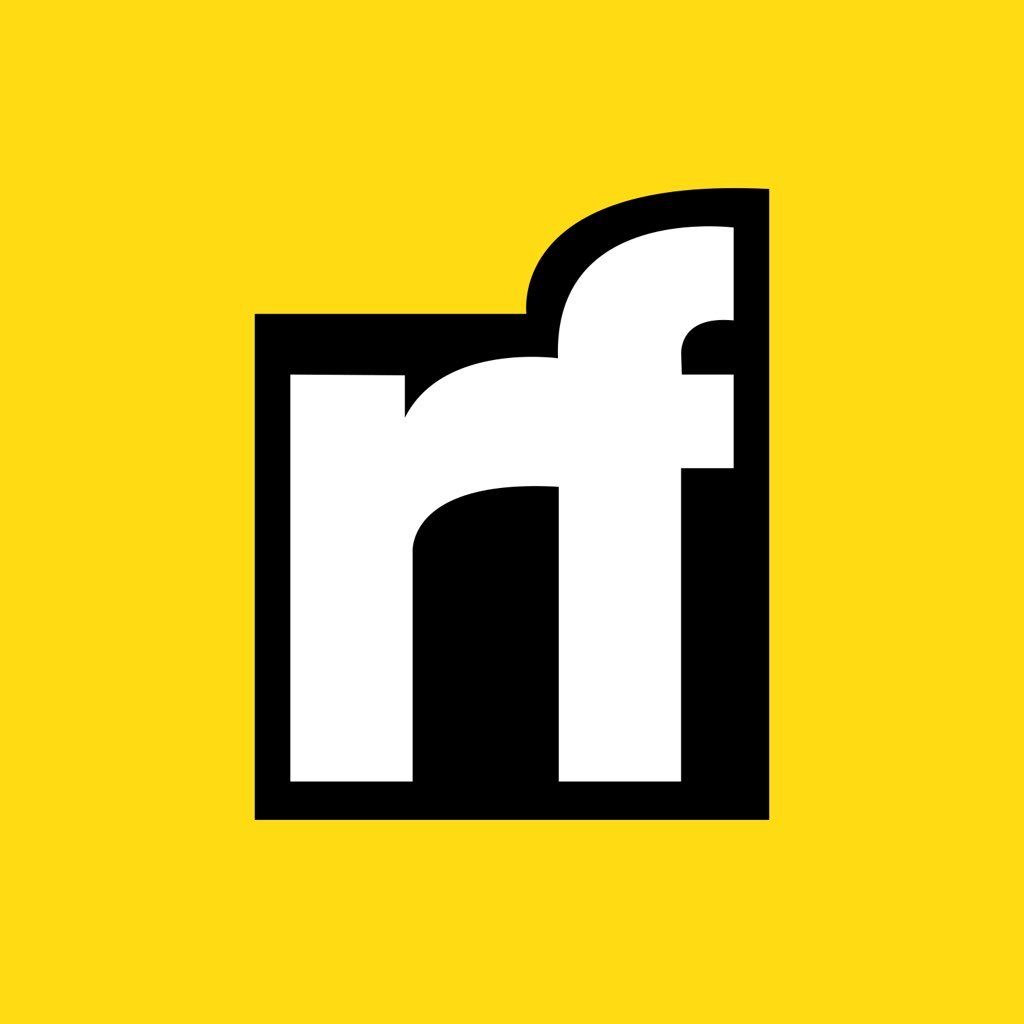
IgorSusmelj | Sciencx (2025-02-16T20:38:17+00:00) RustyNum Follow-Up: Fresh Insights and Ongoing Development. Retrieved from https://www.scien.cx/2025/02/16/rustynum-follow-up-fresh-insights-and-ongoing-development/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.