This content originally appeared on Level Up Coding - Medium and was authored by Gal Ashuach
When Done Correctly — Code Can Be a Form Of Art
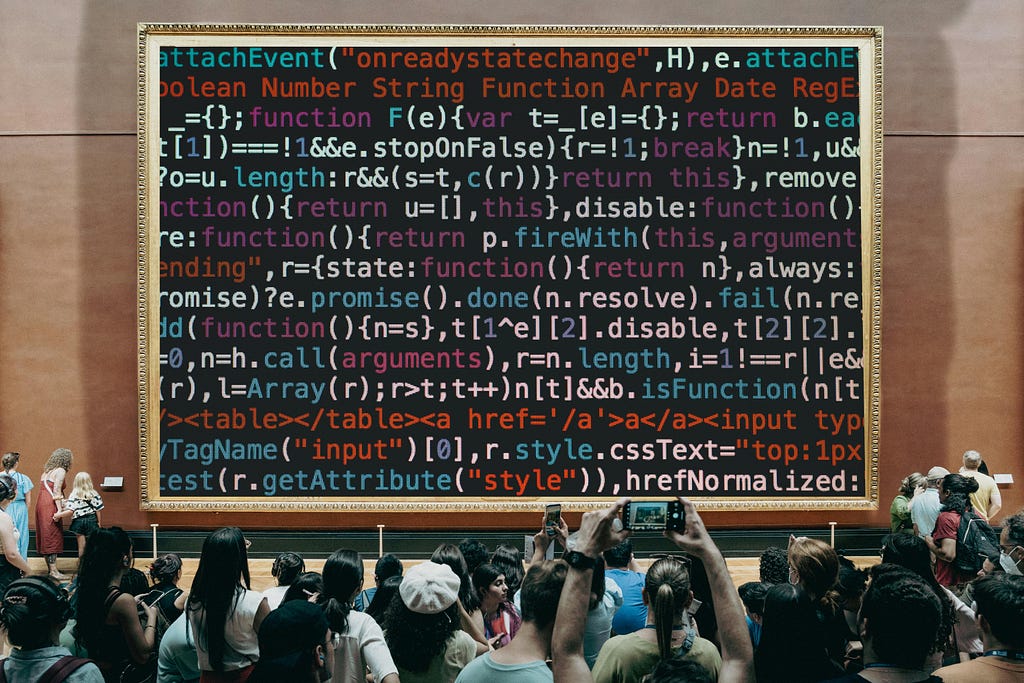
“This was one of the best parts of being a coder, and an artist: the thrill of being in the middle of creating something delightful. It’s like the anticipation of eating freshly baked bread after its aroma fills the room.”
— Dr. Joy Buolamwini, Unmasking AI
The first thing they teach you is that your code should be correct; It should work on all inputs, and handle exceptions and edge cases. Then, you learn about efficiency; You understand different algorithms have different complexities and you try to think about the best way to solve a problem efficiently. As time goes by and you start collaborating with others, you learn different coding conventions and practices used in the industry. But what is the next step? What turns a programmer into a craftsman? What differentiates between simple code and a syntactic masterpiece?
What makes code beautiful?
As we see in all forms of art such as music, painting, or literature, beauty is subjective. In my opinion, several characteristics can make your code beautiful, and here are my top three:
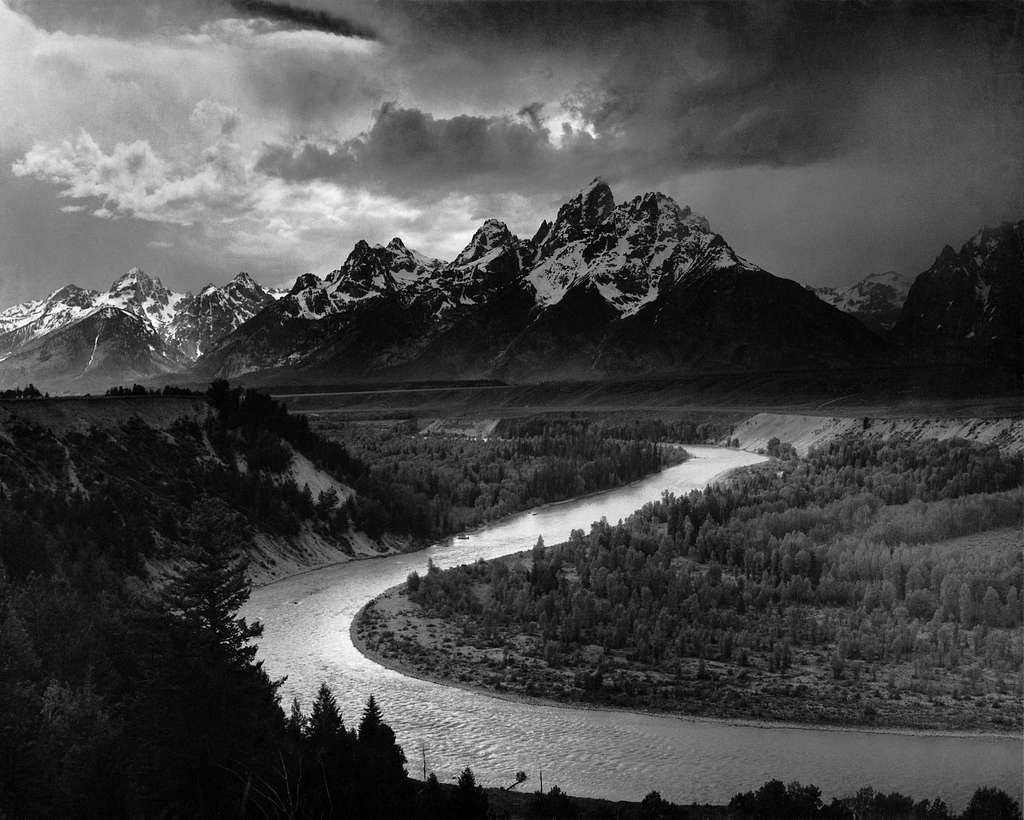
Readability
“The professional understands that clarity is king. Professionals use their powers for good and write code that others can understand.”
― Robert C. Martin, Clean Code: A Handbook of Agile Software Craftsmanship
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
― Martin Fowler
One of the most important traits of an aesthetically appealing code (and a Renaissance painting) is clarity. I always strive to write code others can easily read and hence easily understand. Complicated code wastes programmers’ time and might lead to bugs (if they misunderstand or incorrectly modify it), which can be very costly for the company. In addition, unreadable code is somewhat like a snowball — the next person who will have to modify the code will probably choose the simplest approach possible for completing the task, which will not include a refactor. This means more badly written code will be added, only making it worse for the next person.
Here are five things you can do to keep your code clear:
- Naming: Use descriptive, informative, and concise names for classes, variables, and methods. If possible, make them short. Name anything you can, for example, magic numbers. Good naming can reduce the need for comments. Remember — names are an important part of code, unlike paintings, in which you can call your work “Galacidalacidesoxyribonucleicacid” and still be considered one of the best surrealist artists.
// Don't
public static double bmi(final double w, final double h) {
return w / (h * h);
}
// Do
public static double calculateBMI(final double weight, final double height) {
return weight / (height * height);
}
// don't
public static int size(final int[] arrayOfMoviesFromTheUserBeforeInsertingToDb) {
return arrayOfMoviesFromTheUserBeforeInsertingToDb.length * 4;
}
// do
public static int getSizeInBytes(final int[] arr) {
return arr.length * Integer.BYTES;
}
2. Simplify: Keep your code as simple as possible. Split the logic into classes and methods, with a well-defined single responsibility for each component. Write short lines of code, and make sure the logic is easy to follow.
// don't
final int[] arr = new int[10];
for (int i = 0; i < arr.length - 1; arr[i + 1] = arr[i]++ | 1 << i++) {}
// do
final int[] arr = new int[10];
for (int i = 0; i < arr.length; i++) {
arr[i] = (int) Math.pow(2, i);
}
arr[arr.length -1]--;
3. Avoid premature optimization: It is better to write naive code that works great today, rather than over-engineered code that would support cases/performances you’ll never need. It is very common to improve code when you hit a pain point, but you rarely simplify overcomplicated code left from the past.
// do
public int gcd(final int a, final int b) {
if (b == 0) {
return a;
}
return gcd(b, a % b);
}
// Don't, at the first try, unless you have a really good reason
private final Cache<Pair<Integer, Integer>, Integer> cache = Caffeine.newBuilder()
.maximumSize(100_000)
.build();
public int gcd(final int a, final int b) {
if (b == 0) {
return a;
}
final Pair<Integer, Integer> key = Pair.of(b, a % b);
Integer result = cache.getIfPresent(key);
if (result != null) {
return result;
}
result = gcd(b, a % b);
cache.put(key, result);
return result;
}
4. Avoid deep nesting: If your indentations are getting close to the middle of the screen, consider using the opposite condition. This will often mean starting with error checking and breaking out of the block.
// Don't
public Long getLatestDateInSecondsSinceEpoch(final List<Map<String, LocalDateTime>> list) {
long maxValue = Long.MIN_VALUE;
if (list != null) {
for (final Map<String, LocalDateTime> map : list) {
if (map != null) {
for (final LocalDateTime date : map.values()) {
if (date != null) {
maxValue = Math.max(maxValue, date.atZone(ZoneId.systemDefault()).toEpochSecond());
}
}
}
}
}
return maxValue;
}
// Do
public Long getLatestDateInSecondsSinceEpoch(final List<Map<String, LocalDateTime>> list) {
long maxValue = Long.MIN_VALUE;
if (list == null) {
return maxValue;
}
for (final Map<String, LocalDateTime> map : list) {
if (map == null) {
continue;
}
for (final LocalDateTime date : map.values()) {
if (date == null) {
continue;
}
maxValue = Math.max(maxValue, date.atZone(ZoneId.systemDefault()).toEpochSecond());
}
}
return maxValue;
}
5. DRY (do not repeat yourself): Try to avoid code duplication. If you have the exact (or similar) code in different places, consider extracting it to a shared location and using it for both cases. When there is less code, it is easier to keep track. I know some (the more intellectuals) of you might say that great artists like Andy Warhol, M. C. Esche, and Rene Magritte did repetition artworks - but trust me, programmers do not like repetition.
// Don't
private static Character getFirstLexicographicallyOrderedLetter(final String str) {
if (str == null || str.isEmpty()) {
return null;
}
final String lowerCaseStr = str.toLowerCase();
Character first = null;
for (int i = 0; i < lowerCaseStr.length(); i++) {
final char c = lowerCaseStr.charAt(i);
if (Character.isLetter(c) && (first == null || c < first)) {
first = c;
}
}
return first;
}
private static Character getLastLexicographicallyOrderedLetter(final String str) {
if (str == null || str.isEmpty()) {
return null;
}
final String lowerCaseStr = str.toLowerCase();
Character last = null;
for (int i = 0; i < lowerCaseStr.length(); i++) {
final char c = lowerCaseStr.charAt(i);
if (Character.isLetter(c) && (last == null || c > last)) {
last = c;
}
}
return last;
}
private static Character getFirstLexicographicallyOrderedVowelOrLetter(final String str) {
if (str == null || str.isEmpty()) {
return null;
}
final ToIntFunction<Character> score = "zyxwvtsrqpnmlkjhgfdcbuoiea"::indexOf;
final String lowerCaseStr = str.toLowerCase();
Character first = null;
for (int i = 0; i < lowerCaseStr.length(); i++) {
final char c = lowerCaseStr.charAt(i);
if (Character.isLetter(c) && (first == null || score.applyAsInt(c) > score.applyAsInt(first))) {
first = c;
}
}
return first;
}
// Do
private static Character getFirstLexicographicallyOrderedLetter(final String str) {
return getLetterByComparator(str, Comparator.reverseOrder());
}
private static Character getLastLexicographicallyOrderedLetter(final String str) {
return getLetterByComparator(str, Comparator.naturalOrder());
}
private static Character getFirstLexicographicallyOrderedVowelOrLetter(final String str) {
final ToIntFunction<Character> charToVowelScore = "uoiea"::indexOf;
return getLetterByComparator(str, Comparator.comparingInt(charToVowelScore)
.thenComparing(Comparator.reverseOrder()));
}
private static Character getLetterByComparator(final String str, final Comparator<Character> comparator) {
if (str == null || str.isEmpty()) {
return null;
}
final String lowerCaseStr = str.toLowerCase();
Character result = null;
for (int i = 0; i < lowerCaseStr.length(); i++) {
final char c = lowerCaseStr.charAt(i);
if (Character.isLetter(c) && (result == null || comparator.compare(c, result) > 0)) {
result = c;
}
}
return result;
}
“Code is read more often than it is written.”
— Guido van Rossum
“Code is like humor. When you have to explain it, it’s bad.”
― Cory House
Syntactic sugar and new features
“The code you write makes you a programmer. The code you delete makes you a good one. The code you don’t have to write makes you a great one.”
―Mario Fusco,
“The function of good software is to make the complex appear to be simple.” ―Grady Booch
A good programmer should be familiar with built-in libraries and features and keep track of new developments. It is not uncommon for a new version of a language to provide elegant solutions for existing problems and replace old and ugly syntax. For example in Java: string templates instead of concatenation, records instead of immutable classes, advanced pattern matching, and enhanced switch expressions.
It is always a pleasure to see a line of code and think “Wow, I didn’t know we could do it like that”, or “What a great choice of syntax” as opposed to “Why does this code reinvent the wheel? There’s a standard implementation for it since Java 8.”. Progress in part of the process. Pig bladders to paint tubes, quills to typewriters, analog cameras to digital ones, and brew install are all alike.
// Don't
private void addToCount(final List<String> list) {
for (int i = 0; i < list.size(); ++i) {
final String str = list.get(i);
final Integer count = counter.get(str);
if (count == null) {
counter.put(str, 1);
} else {
counter.put(str, count + 1);
}
}
}
// Do
private void addToCount(final List<String> list) {
list.forEach(str -> counter.merge(str, 1, Integer::sum));
}
“The most dangerous phrase in the language is, ‘We’ve always done it this way.’” — Grace Hopper
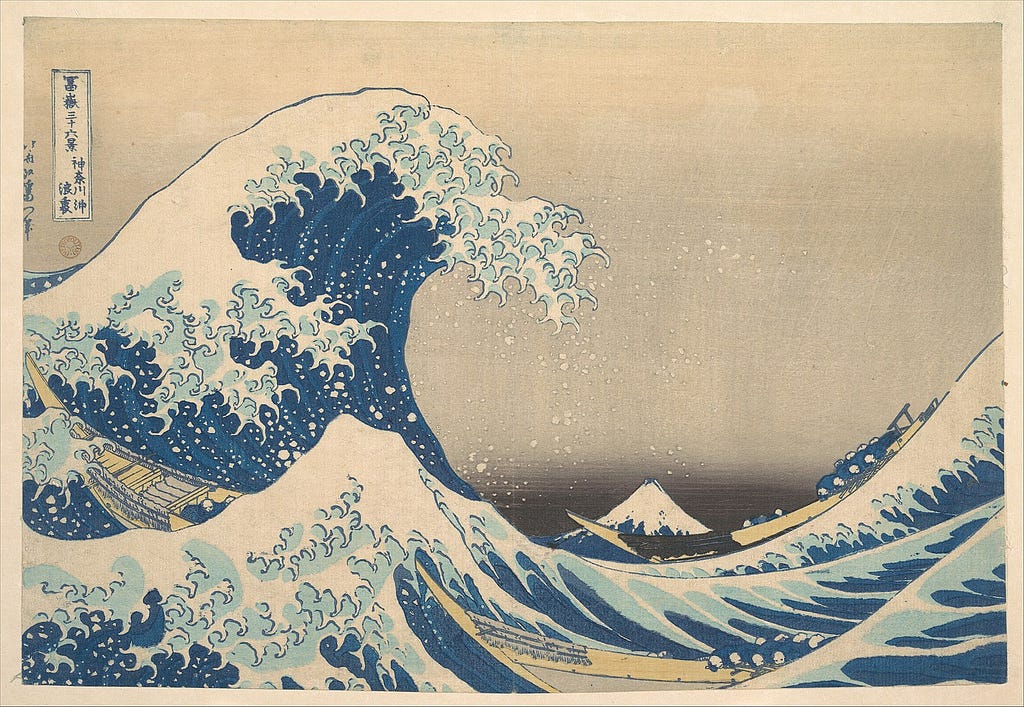
Maintainability
“The only constant in life is change.”
— Heraclitus
As you all know, adding new classes and methods is only a small part of the job, and in many cases, we are required to change the existing ones. It could be something small like a minor bug fix or adding a new metric, but in many cases, we are required to support a whole new functionality or feature that was not originally intended.
There is nothing more beautiful than finding out our old code can be easily changed to support the new requirements without making it look awkward or unnatural. Like people, code should age with grace, and should be able to withstand future tweaks and modifications by yourself and/or others.
Wait, what about efficiency?
Life is all about tradeoffs, and when it comes to performance vs clarity, I would recommend going with the most readable option you can afford to write, without exceeding the performance limitations of your system. Sometimes you don’t have the luxury to write nice code with many object allocations and dynamic calls, and you have no choice but to write efficient (yet primitive) code.
For example, in most cases, I would advise going with i * 2 over i << 1. Shifting is generally a lot faster than multiplying at the instruction level, but the latter might be confusing for other programmers (and the future you). In addition, in many languages, the compiler might optimize multiplication into shifting anyway.
Examples of beautifully written code
As I said earlier — beauty is in the eye of the beholder, and it is hard to bring real-life examples into a blog post as they might involve many files and lines of code. so, I decided to show you a few short examples of something I found quite captivating called “Fluent Interface”. A fluent interface is an object-oriented API using method chaining and cascading to create domain-specific language. In simple words, the different parts of the logic (method calls) are organized as if they were a sentence, making their usage extremely readable and declarative.
- Hamcrest: a framework for asserting matches with many built-in matchers and the ability to write your own.
assertThat("foo", equalToIgnoringCase("FoO"));
assertThat(Cat.class,typeCompatibleWith(Animal.class));
assertThat(person, hasProperty("city", equalTo("New York")));
assertThat(list, containsInAnyOrder("hello", "world"));
assertThat(array, hasItemInArray(42));
assertThat(map, hasEntry(key, value));
assertThat(1, greaterThan(0));
assertThat("test", equalToIgnoringWhiteSpace(" test"));
assertThat("congratulations",stringContainsInOrder(Arrays.asList("con","gratul","ations")));
assertThat("congratulations", startsWith("cong"));
assertThat(list, everyItem(greaterThan(42)));
assertThat("congratulations", anyOf(startsWith("cong"), containsString("ions")));
2. Awaitility: a library for asynchronous systems testing with user-configurable waiting settings.
await()
.atMost(5, SECONDS)
.until(result::isNotEmpty);
with()
.pollInterval(1, SECONDS)
.await("insert row to DB")
.atMost(1, MINUTES)
.until(newRowWasAdded())
3. DataStax Java Driver: Driver for generating CQL queries programmatically.
Select select =
selectFrom("keyspace", "table")
.column("name")
.column("age")
.whereColumn("id").isEqualTo(bindMarker());
// equivalent to SELECT name,age FROM keyspace.table WHERE id=?
deleteFrom("table")
.whereColumn("key").isEqualTo(bindMarker())
.ifColumn("age").isEqualTo(literal(28));
// DELETE FROM table WHERE key=? IF age = 28
insertInto("table")
.value("name", bindMarker())
.value("age", bindMarker())
.usingTtl(60)
// INSERT INTO table (name,age) VALUES (?,?) USING TTL 60
Conclusion
There are many alternative ways to solve the same problem, and each case has its own considerations, leading one to different paths. Your goal is not to pick the easier one to implement, but the one that is easier to understand and maintain down the road. Maybe the choice is similar to that of a spouse - choose for the long run, as you will (hopefully) be stuck with him/her for a while. Francisco de Goya is unarguably one of the greatest Spanish romantic painters, but I wouldn’t put “Saturn Devouring One of His Sons” in a nursery — you should always match the art to the audience.
“There are two ways of constructing a software design: One way is to make it so simple that there are obviously no deficiencies, and the other way is to make it so complicated that there are no obvious deficiencies. The first method is far more difficult.”
- C.A.R. Hoare
When Done Correctly — Code Can Be a Form Of Art was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Gal Ashuach
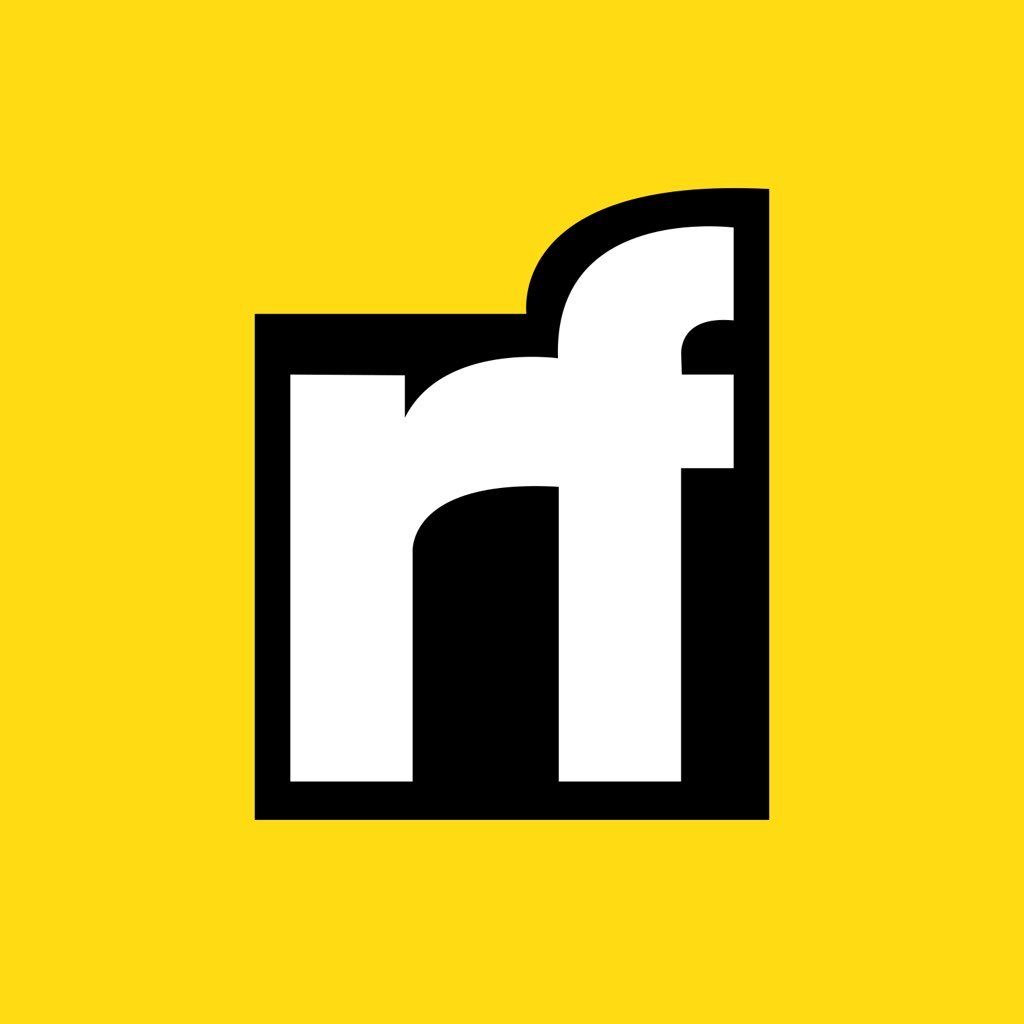
Gal Ashuach | Sciencx (2025-02-17T03:08:05+00:00) When Done Correctly — Code Can Be a Form Of Art. Retrieved from https://www.scien.cx/2025/02/17/when-done-correctly-code-can-be-a-form-of-art/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.