This content originally appeared on DEV Community and was authored by Abdullah Al Mamun Akand
Introduction:
Sending emails programmatically is a common requirement for many web applications. In this blog post, we will walk you through how to send emails using Gmail’s SMTP server in a .NET Core Web API application using MailKit, a modern and lightweight email library for .NET.
By the end of this guide, you'll learn how to set up a Web API, integrate MailKit to send emails, and use configuration settings stored in appsettings.json
.
Prerequisites:
Before you begin, ensure you have the following:
- .NET 6 or higher installed.
- A Gmail account with 2-Step Verification enabled and an App Password generated (since Gmail blocks less secure apps).
- Basic knowledge of creating Web API projects in .NET.
Step 1: Set Up Your .NET Web API Project
Open Visual Studio (or your preferred IDE) and create a new Web API project.
Install MailKit via NuGet:
dotnet add package MailKit
MailKit is a fully featured email client library that allows you to send and receive emails using SMTP, POP3, and IMAP.
Step 2: Add Email Configuration in appsettings.json
In your project, open the appsettings.json
file and add the configuration for your email settings, like this:
{
"EmailSettings": {
"Username": "your-mail@gmail.com",
"Password": "gmail-generated-app-password",
"SmtpServer": "smtp.gmail.com",
"Port": 587
}
}
- Username: Your Gmail email address.
- Password: The App Password generated in your Gmail account. (you can create it from your Google Account under Security > App Passwords).
-
SmtpServer: The SMTP server for Gmail (
smtp.gmail.com
). -
Port: Gmail's SMTP port, which is
587
for TLS encryption.
Step 3: Create the Email Service
We will create a service class called EmailService
that will handle email sending.
1. Create an Interface for the service:
In the IRepository
folder (or wherever you prefer), create a new file called IEmailService.cs
:
namespace EmailApp.IRepository
{
public interface IEmailService
{
Task<string> SendEmail(string toEmail, string subject, string body);
}
}
2. Create the Email Service class that implements the IEmailService
interface.
In the Repository
folder, create a new file called EmailService.cs
:
using EmailApp.IRepository;
using MailKit.Net.Smtp;
using MailKit.Security;
using Microsoft.Extensions.Configuration;
using MimeKit;
namespace EmailApp.Repository
{
public class EmailService : IEmailService
{
private readonly IConfiguration _configuration;
public EmailService(IConfiguration configuration)
{
_configuration = configuration;
}
public async Task<string> SendEmail(string toEmail, string mailSubject, string mailBody)
{
try
{
var smtpServer = _configuration["EmailSettings:SmtpServer"];
var port = int.Parse(_configuration["EmailSettings:Port"]);
var fromMail = _configuration["EmailSettings:Username"];
var password = _configuration["EmailSettings:Password"];
var email = new MimeMessage();
email.From.Add(new MailboxAddress("Your Name", fromMail));
email.To.Add(new MailboxAddress("To Name", toEmail));
email.Subject = mailSubject;
email.Body = new TextPart("html") { Text = mailBody };
using var smtp = new SmtpClient();
await smtp.ConnectAsync(smtpServer, port, SecureSocketOptions.StartTls);
await smtp.AuthenticateAsync(fromMail, password);
await smtp.SendAsync(email);
await smtp.DisconnectAsync(true);
return "Email sent successfully!";
}
catch (Exception ex)
{
// Log the exception (not done here for simplicity)
return $"Email sending failed. Error: {ex.Message}";
}
}
}
}
Brief Explanation of the Code:
1. Fetching Configuration Values
var smtpServer = _configuration["EmailSettings:SmtpServer"];
var port = int.Parse(_configuration["EmailSettings:Port"]);
var fromMail = _configuration["EmailSettings:Username"];
var password = _configuration["EmailSettings:Password"];
- Retrieves SMTP server details (server address, port, username, and password) from
appsettings.json
.
2. Creating the Email Message
var email = new MimeMessage();
email.From.Add(new MailboxAddress("Your Name", fromMail));
email.To.Add(new MailboxAddress("To Name", toEmail));
email.Subject = mailSubject;
email.Body = new TextPart("html") { Text = mailBody };
- MimeMessage is used to create an email.
-
From & To Addresses: Sets the sender (
fromMail
) and recipient (toEmail
). - Subject & Body: Sets the subject and body in HTML format.
3. Sending the Email
using var smtp = new SmtpClient();
await smtp.ConnectAsync(smtpServer, port, SecureSocketOptions.StartTls);
await smtp.AuthenticateAsync(fromMail, password);
await smtp.SendAsync(email);
await smtp.DisconnectAsync(true);
-
Creates an SMTP client (
SmtpClient
). - Connects to SMTP server using the given server address and port.
- Enables authentication with Gmail credentials.
- Sends the email.
- Disconnects cleanly after sending.
Step 4: Register the Service in Program.cs
Now, register the EmailService
in the Program.cs
file so that it can be injected into the controller.
In your Program.cs
(or Startup.cs
for older versions), add the following:
builder.Services.AddScoped<IEmailService, EmailService>();
This registers the EmailService
to be available for dependency injection in your controllers.
Step 5: Create the Email Controller
Next, let's create an API controller that will handle HTTP requests for sending emails.
In the Controllers
folder, create a new file called EmailController.cs
:
using EmailApp.IRepository;
using Microsoft.AspNetCore.Mvc;
namespace EmailApp.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class EmailController : ControllerBase
{
private readonly IEmailService _emailService;
public EmailController(IEmailService emailService)
{
_emailService = emailService;
}
[HttpPost]
[Route("SendEmail")]
public async Task<IActionResult> SendEmail(string toEmail, string mailSubject, string mailBody)
{
var result = await _emailService.SendEmail(toEmail, mailSubject, mailBody);
return Ok(new { message = result });
}
}
}
This controller exposes a POST endpoint api/Email/SendEmail
that will accept parameters like toEmail
, mailSubject
, and mailBody
, and send the email accordingly using the EmailService
.
Step 6: Test the API
You can now test the API by sending a POST request using Postman or Swagger (if configured).
Example Request:
-
Endpoint:
POST api/Email/SendEmail
- Body (JSON):
{
"toEmail": "recipient-email@gmail.com",
"mailSubject": "Test Email",
"mailBody": "This is a test email sent from the .NET Web API using MailKit."
}
If everything is set up correctly, you should receive a response like:
{
"message": "Email sent successfully!"
}
Conclusion:
In this blog, you learned how to send emails using Gmail's SMTP server in a .NET Web API application with the MailKit library. You also saw how to structure the solution with the Repository Pattern, and how to manage email configuration settings securely in appsettings.json
.
By following these steps, you can easily integrate email functionality into your .NET Core applications and send emails via Gmail (or any other SMTP service) programmatically.
Next Steps:
- Enhance the email service with additional functionality such as attachments or HTML content formatting.
- Build a UI to trigger email sending from a front-end application.
Happy coding!
Find me on LinkedIn
This content originally appeared on DEV Community and was authored by Abdullah Al Mamun Akand
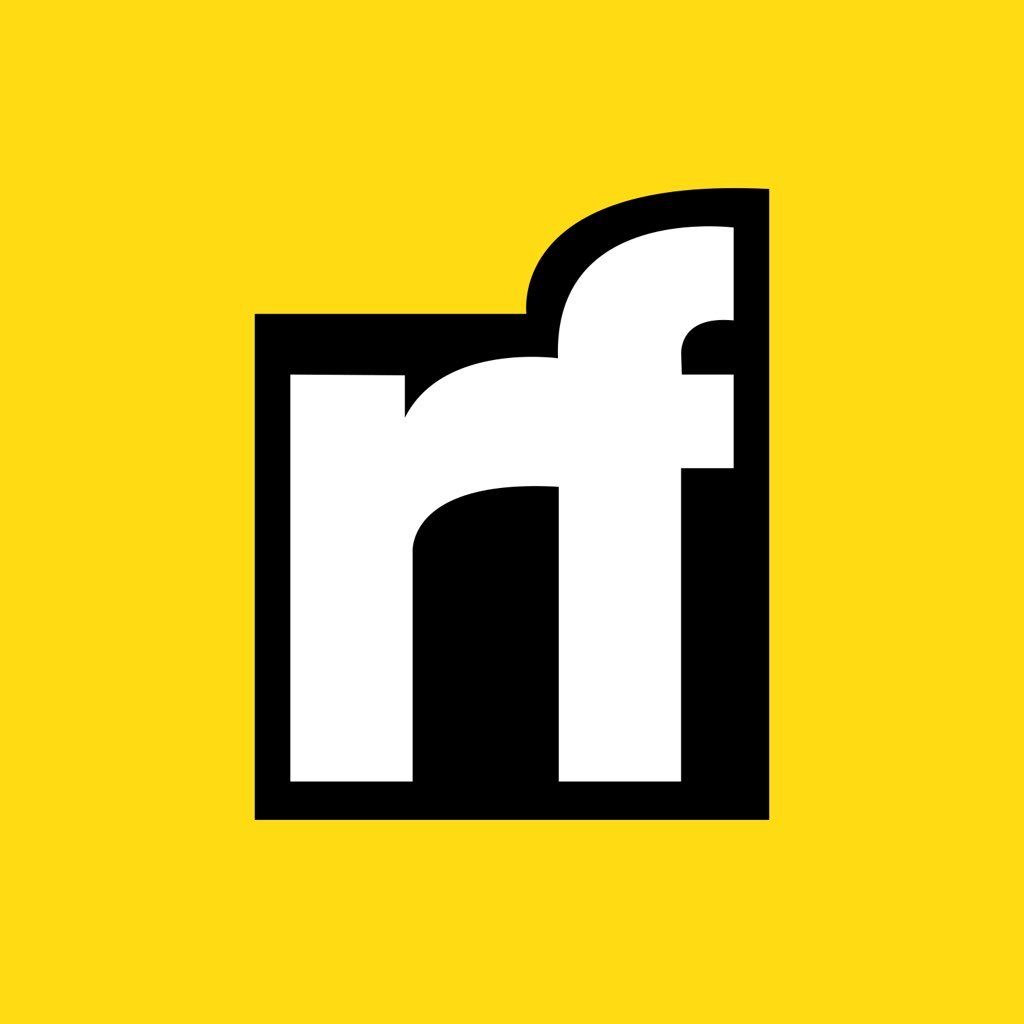
Abdullah Al Mamun Akand | Sciencx (2025-02-18T19:43:13+00:00) Sending Emails with Gmail Using MailKit in .NET Web API: The Complete Guide. Retrieved from https://www.scien.cx/2025/02/18/sending-emails-with-gmail-using-mailkit-in-net-web-api-the-complete-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.