This content originally appeared on DEV Community and was authored by Rowsan Ali
In today's web development landscape, creating a responsive navigation bar is a important part of building a user-friendly website. A well-designed navigation bar not only enhances the user experience but also ensures that your website is accessible on all devices, from desktops to mobile phones. In this blog post, we'll walk through the process of building a responsive navigation bar with dropdowns using Tailwind CSS, a utility-first CSS framework that makes styling a breeze.
What is Tailwind CSS?
Tailwind CSS is a highly customizable, low-level CSS framework that provides utility classes to build designs directly in your markup. Unlike traditional CSS frameworks that come with pre-designed components, Tailwind gives you the building blocks to create custom designs without writing a single line of CSS.
Prerequisites
Before we dive into the code, make sure you have the following set up:
- Node.js installed on your machine.
- Tailwind CSS installed in your project. If you haven't set up Tailwind yet, you can follow the official installation guide.
Step 1: Setting Up the HTML Structure
Let's start by creating the basic HTML structure for our navigation bar. We'll use a <nav>
element to wrap our navigation items.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Navbar with Tailwind CSS</title>
<link href="https://cdn.jsdelivr.net/npm/tailwindcss@2.2.19/dist/tailwind.min.css" rel="stylesheet">
</head>
<body>
<nav class="bg-blue-600 p-4">
<div class="container mx-auto flex justify-between items-center">
<a href="#" class="text-white text-2xl font-bold">Logo</a>
<ul class="hidden md:flex space-x-4">
<li><a href="#" class="text-white hover:text-blue-200">Home</a></li>
<li><a href="#" class="text-white hover:text-blue-200">About</a></li>
<li class="relative">
<a href="#" class="text-white hover:text-blue-200">Services</a>
<ul class="absolute hidden bg-blue-600 mt-2 space-y-2">
<li><a href="#" class="block px-4 py-2 text-white hover:bg-blue-700">Web Design</a></li>
<li><a href="#" class="block px-4 py-2 text-white hover:bg-blue-700">SEO</a></li>
<li><a href="#" class="block px-4 py-2 text-white hover:bg-blue-700">Marketing</a></li>
</ul>
</li>
<li><a href="#" class="text-white hover:text-blue-200">Contact</a></li>
</ul>
<button class="md:hidden text-white focus:outline-none">
<svg class="w-6 h-6" fill="none" stroke="currentColor" viewBox="0 0 24 24" xmlns="http://www.w3.org/2000/svg">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M4 6h16M4 12h16m-7 6h7"></path>
</svg>
</button>
</div>
</nav>
<div class="md:hidden">
<ul class="bg-blue-600 space-y-2 p-4">
<li><a href="#" class="block text-white hover:text-blue-200">Home</a></li>
<li><a href="#" class="block text-white hover:text-blue-200">About</a></li>
<li>
<a href="#" class="block text-white hover:text-blue-200">Services</a>
<ul class="pl-4 mt-2 space-y-2">
<li><a href="#" class="block text-white hover:text-blue-200">Web Design</a></li>
<li><a href="#" class="block text-white hover:text-blue-200">SEO</a></li>
<li><a href="#" class="block text-white hover:text-blue-200">Marketing</a></li>
</ul>
</li>
<li><a href="#" class="block text-white hover:text-blue-200">Contact</a></li>
</ul>
</div>
<script>
const mobileMenuButton = document.querySelector('button');
const mobileMenu = document.querySelector('.md\\:hidden ul');
mobileMenuButton.addEventListener('click', () => {
mobileMenu.classList.toggle('hidden');
});
</script>
</body>
</html>
Explanation:
-
Navbar Container: The
<nav>
element is styled with a blue background (bg-blue-600
) and padding (p-4
). -
Logo: The logo is a simple text link styled with white color (
text-white
), larger font size (text-2xl
), and bold font weight (font-bold
). -
Navigation Links: The navigation links are wrapped in a
<ul>
element. The links are hidden on mobile screens (hidden
) and displayed on medium screens and above (md:flex
). -
Dropdown Menu: The "Services" link has a dropdown menu that is hidden by default (
hidden
). The dropdown menu is positioned absolutely (absolute
) below the "Services" link. -
Mobile Menu Button: A button with a hamburger icon is displayed on mobile screens (
md:hidden
). When clicked, it toggles the visibility of the mobile menu.
Step 2: Making the Navbar Responsive
The navbar we've created so far is responsive, but the dropdown menu doesn't work on mobile screens. Let's fix that by adding some JavaScript to toggle the dropdown menu.
JavaScript for Dropdown Toggle
We'll use JavaScript to toggle the visibility of the dropdown menu when the "Services" link is clicked on mobile screens.
document.addEventListener('DOMContentLoaded', () => {
const servicesLink = document.querySelector('li.relative > a');
const dropdownMenu = document.querySelector('li.relative ul');
servicesLink.addEventListener('click', (e) => {
e.preventDefault();
dropdownMenu.classList.toggle('hidden');
});
});
Explanation:
-
Event Listener: We add an event listener to the "Services" link to prevent the default link behavior (
e.preventDefault()
) and toggle the visibility of the dropdown menu (dropdownMenu.classList.toggle('hidden')
).
Step 3: Enhancing the Dropdown Menu
To make the dropdown menu more user-friendly, we can add a smooth transition when it appears and disappears. We'll use Tailwind's transition
and transform
utilities to achieve this.
<ul class="absolute hidden bg-blue-600 mt-2 space-y-2 transition-transform transform translate-y-2">
<li><a href="#" class="block px-4 py-2 text-white hover:bg-blue-700">Web Design</a></li>
<li><a href="#" class="block px-4 py-2 text-white hover:bg-blue-700">SEO</a></li>
<li><a href="#" class="block px-4 py-2 text-white hover:bg-blue-700">Marketing</a></li>
</ul>
Explanation:
-
Transition: The
transition-transform
class adds a smooth transition effect when the dropdown menu appears or disappears. -
Transform: The
transform translate-y-2
class moves the dropdown menu slightly down when it appears, creating a smooth animation.
Step 4: Final Touches
To ensure that the dropdown menu works seamlessly on both desktop and mobile screens, we can add some additional styles and JavaScript to handle different screen sizes.
JavaScript for Responsive Dropdown
document.addEventListener('DOMContentLoaded', () => {
const servicesLink = document.querySelector('li.relative > a');
const dropdownMenu = document.querySelector('li.relative ul');
const mobileMenuButton = document.querySelector('button');
const mobileMenu = document.querySelector('.md\\:hidden ul');
servicesLink.addEventListener('click', (e) => {
if (window.innerWidth < 768) {
e.preventDefault();
dropdownMenu.classList.toggle('hidden');
}
});
mobileMenuButton.addEventListener('click', () => {
mobileMenu.classList.toggle('hidden');
});
});
Explanation:
-
Conditional Toggle: The dropdown menu is only toggled on mobile screens (
window.innerWidth < 768
). On larger screens, the dropdown menu will appear on hover (handled by Tailwind'sgroup-hover
utility).
Conclusion
Congratulations! You've successfully built a responsive navigation bar with dropdowns using Tailwind CSS. This navigation bar is fully responsive, works seamlessly on all devices, and includes smooth transitions for a polished user experience.
Tailwind CSS makes it easy to create custom, responsive designs without writing a lot of custom CSS. By leveraging Tailwind's utility classes, you can quickly build complex components like navigation bars with dropdowns. Happy coding!
This content originally appeared on DEV Community and was authored by Rowsan Ali
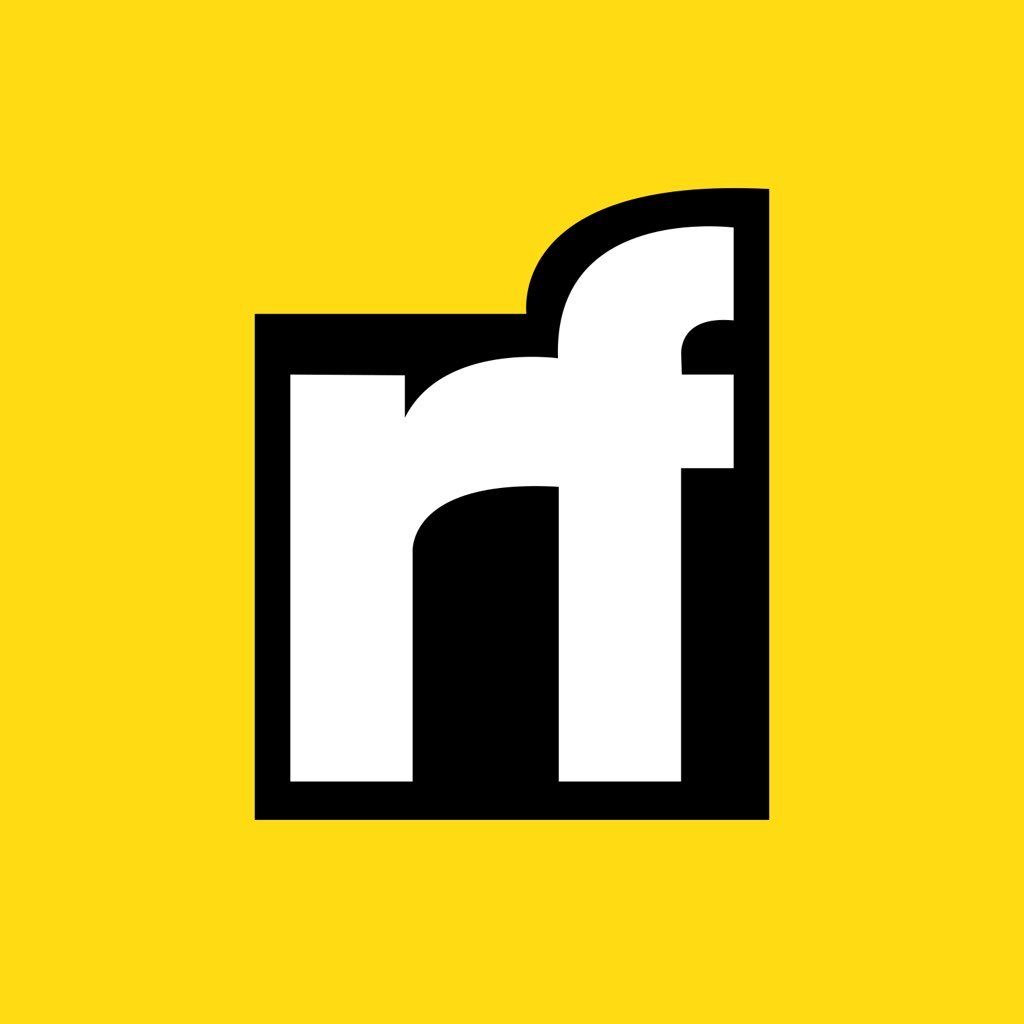
Rowsan Ali | Sciencx (2025-02-19T08:16:19+00:00) How to Build a Responsive Navigation Bar with Dropdowns Using Tailwind. Retrieved from https://www.scien.cx/2025/02/19/how-to-build-a-responsive-navigation-bar-with-dropdowns-using-tailwind/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.