This content originally appeared on DEV Community and was authored by datacollection
Google Scholar is an important tool for academic researchers around the world to find and obtain literature, covering papers, monographs and conference papers in various fields. However, due to its strict anti-crawler mechanism, it is not easy to directly crawl Google Scholar data, especially for users who need large-scale data collection.
In this article, we will introduce two methods for crawling Google Scholar data: manual crawling (Scrapy/Selenium) and Scrapeless API. Manual crawling is suitable for small-scale data collection, but may encounter IP restrictions and verification code problems. Scrapeless API provides a more stable and efficient solution, especially for large-scale data crawling, without the need to maintain additional anti-detection strategies.
By comparing the advantages and disadvantages of the two methods, this article will help you choose the most suitable solution for you to achieve efficient data collection.
Why Scrape Google Scholar?
Google Scholar provides valuable academic resources, including research papers, citations, author profiles, and more. By scraping Google Scholar, you can:
- Collect research papers on a specific topic.
- Extract citation counts for academic impact analysis.
- Retrieve author profiles and their published work.
- Automate literature reviews for research purposes. ## Challenges in Scraping Google Scholar
Scraping Google Scholar comes with challenges such as:
- CAPTCHAs: Frequent requests can trigger Google's anti-bot protection.
- IP Blocking: Google can block IPs making repeated automated requests.
- Dynamic Content: Some results may be dynamically loaded via JavaScript.
To overcome these challenges, we recommend using a dedicated API such as Scrapeless API.
Method 1: How to Scrape Google Scholar - Traditional Web Scraping (Not Recommended)
Web scraping Google Scholar manually using Python (e.g., BeautifulSoup, Selenium) is difficult due to Google's restrictions. Example using requests:
import requests
from bs4 import BeautifulSoup
url = "https://scholar.google.com/scholar?q=machine+learning"
headers = {"User-Agent": "Mozilla/5.0"}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, "html.parser")
results = soup.find_all("div", class_="gs_r")
for result in results:
title = result.find("h3").text if result.find("h3") else "No Title"
print(title)
📌 Disadvantages:
- It is easy to trigger Google's anti-crawling mechanism, resulting in IP blocking or encountering CAPTCHA.
- The data structure is complex, and it is necessary to parse HTML and process dynamically loaded content.
- It is not suitable for large-scale data collection and has low stability.
This approach is not reliable due to Google's anti-bot measures. Instead, using an API like Scrapeless API is the best alternative.
Method 2: Scraping Google Scholar with Scrapeless Scraping API (Recommended)
Scrapeless's Google Scholar API is a tool designed for academic research and data analysis. It can automatically crawl Google Scholar search results to obtain key information such as paper titles, authors, publication dates, and citation counts. It parses Google Scholar's SERP (search engine results page) to provide structured JSON data, avoid IP blocking and verification code verification, and save users from having to deal with complex crawler development.
In addition, Scrapeless also supports real-time search, batch query, and custom parameter filtering, which is suitable for researchers, developers, and data analysts.
Key features of Scrapeless's Google Scholar API
- Automatic parsing: No need to manually write crawlers, directly obtain structured JSON data.
- Real-time data: Support real-time queries to ensure that the Google Scholar results obtained are the latest.
- Anti-crawling mechanism: Automatically bypass Google Scholar's CAPTCHA and IP blocking, without the need for proxy or additional configuration.
- Rich data fields: Provide detailed data such as paper title, author, publication date, number of citations, journal information, related papers, etc.
- Support batch query: You can obtain search results for multiple keywords at one time to improve crawling efficiency.
- Custom search parameters: Support filtering data by time, language, document type, etc., and accurately locate target information.
- Stable API access: Based on cloud architecture, it ensures stability and reliability during high concurrent access.
Sign up for free and start scraping Google search results now!
Log in to Scrapeless now to get a free trial opportunity to easily scrape data from Google search engines to help your projects and analysis. Powerful API functions can help you obtain accurate search information and improve efficiency. Come and experience it!
📌 Scrapeless API key features:
API | Function | Use Case |
---|---|---|
Author API | Retrieves scholar information (H-index, number of papers, etc.) | Scholar impact analysis |
Cite API | Retrieves paper citation formats (BibTeX, APA, etc.) | Paper management |
Organic Results API | Retrieves Google Scholar search results | Academic research |
Profiles API | Retrieves scholar profile data | Collaborative research analysis |
How to use Scrapeless Google Scholar API
Step 1. Obtain Your API Key
To get started, you’ll need to obtain your API Key from the Scrapeless Dashboard:
- Log in to the Scrapeless Dashboard.
- Navigate to API Key Management.
- Click Create to generate your unique API Key.
- Once created, simply click on the API Key to copy it.
Step 2: Use Your API Key in the Code
You can now use your API Key to integrate Scrapeless into your project. Follow these steps to test and implement the API:
- Visit the API Documentation.
- Click "Try it out" for the desired endpoint.
- Enter your API Key in the "Auth" field.
- Click "Send" to get the scraping response.
Below is a sample code snippet that you can directly integrate into your Google Scholar Scraper:
import http.client
import json
conn = http.client.HTTPSConnection("api.scrapeless.com")
payload = json.dumps({
"actor": "scraper.google.scholar",
"input": {
"engine": "google_scholar",
"q": "biology"
}
})
headers = {
'Content-Type': 'application/json'
}
conn.request("POST", "/api/v1/scraper/request", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
In addition, Scrapeless also supports many scraping APIs solutions, such as: Amazon scraping API, Shopee Scraping API, Google Flights scraping API, Google Map scraping API, etc.
Scrapeless Google Scholar API
Scrapeless's Google Scholar API is a powerful tool that can crawl academic papers, journals, books and other resources from Google Scholar through API requests. It allows users to search by specified keywords and obtain detailed information about related documents, such as title, publication information, number of citations, etc.
Google Scholar API Parameters
Parameter | Required | Description |
---|---|---|
engine | TRUE | Set to google_scholar to use this API. |
q | TRUE | Search query (e.g., machine learning). |
cites | FALSE | Unique ID to find citing articles. |
as_ylo | FALSE | Filter results from a specific year. |
as_yhi | FALSE | Filter results up to a specific year. |
hl | FALSE | Language setting (default: en). |
num | FALSE | Number of results (1-20, default: 10). |
Example: Scraping Google Scholar Search Results
Request Code:
import requests
import json
Set the API request URL
url = "https://api.scrapeless.com/api/v1/scraper/request"
Define the payload for the request
payload = json.dumps({
"actor": "scraper.google.scholar",
"input": {
"engine": "google_scholar",
"q": "machine learning", # Search query
"cites": "KNJ0p4CbwgoJ", # Optional: Find articles citing this paper
"as_ylo": 2015, # Optional: Filter results from this year (start year)
"as_yhi": 2023, # Optional: Filter results up to this year (end year)
"hl": "en", # Optional: Language setting (default is English)
"num": 10 # Optional: Number of results (default is 10)
}
})
Set request headers
headers = {
'Content-Type': 'application/json'
}
Send the request
response = requests.request("POST", url, headers=headers, data=payload)
Print the response
print(response.text)
Parameter Explanation:
- engine: Set to google_scholar, indicating the use of Google Scholar for the search.
- q: The search query (e.g., machine learning).
- cites: Optional. Provides the ID of a paper (like KNJ0p4CbwgoJ) to find articles citing it.
- as_ylo: Optional. The starting year (e.g., 2015) to filter the results.
- as_yhi: Optional. The ending year (e.g., 2023) to filter the results.
- hl: Optional. Language setting, default is en for English. Can be set to other languages (e.g., zh for Chinese).
- num: Optional. The number of results to return, between 1 and 20. Default is 10.
Example Response:
{
"search_information": {
"total_results": 5000000,
"time_taken_displayed": 0.05,
"query_displayed": "machine learning"
},
"organic_result": [
{
"position": 1,
"title": "A survey on machine learning methods",
"result_id": "KNJ0p4CbwgoJ",
"link": "https://example.com/article1",
"snippet": "This article provides a comprehensive survey of machine learning methods, including supervised and unsupervised learning.",
"publication_info": {
"summary": "Author1, Author2 - Journal of Machine Learning, 2020"
}
},
{
"position": 2,
"title": "Deep learning in artificial intelligence",
"result_id": "KNJ0p4CbwgoK",
"link": "https://example.com/article2",
"snippet": "This paper discusses the applications of deep learning in various fields, including computer vision and natural language processing.",
"publication_info": {
"summary": "Author3, Author4 - AI Journal, 2021"
}
}
]
}
Response Structure:
- search_information: Contains details about the search query:
- total_results: The total number of results.
- time_taken_displayed: The time it took to display results.
- query_displayed: The search query entered.
- organic_result: A list of the search results, each with:
- position: The rank of the result (e.g., 1st, 2nd).
- title: The title of the paper.
- result_id: The unique ID of the paper.
- link: The URL to the paper.
- snippet: A brief summary or excerpt from the paper.
- publication_info: The publication details.
By adjusting these parameters, you can fine-tune your search to get the most relevant results from Google Scholar, such as limiting results by year, language, or number of results.
Want to start scraping Google Scholar results? Click here to check out our product details and log in now to start using Scrapeless Google Scholar API!
Scrapeless Google Scholar Author API
Scrapeless Google Scholar Author API is a powerful tool for obtaining academic author information on Google Scholar. It can provide basic information about authors, research fields, paper lists, and citation data. This API is particularly suitable for academic researchers and developers to extract academic materials, perform data analysis, or integrate into other applications.
Google Scholar Author API Parameters
Parameter | Required | Description |
---|---|---|
engine | TRUE | Set to google_scholar_author. |
view_op | FALSE | View citations or co-authors. |
sort | FALSE | Sort by title or pubdate. |
start | FALSE | Offset for pagination. |
num | FALSE | Number of results (max: 100). |
"view_op false" | FALSE | It has two options: view_citation - Select to view citations. citation_id is required. list_colleagues - Select to view all co-authors. |
citation_id | FALSE | It is a required parameter when view_op=view_citation is selected. You can access IDs inside our structured JSON response. |
Example: Fetching an Author’s Publications
Here is a code example written in Python that shows how to use the Scrapeless Google Scholar Author API to query information about academic authors:
import http.client
import json
conn = http.client.HTTPSConnection("api.scrapeless.com")
# Define the request payload, specify the crawler engine and author ID
payload = json.dumps({
"actor": "scraper.google.scholar",
"input": {
"engine": "google_scholar_author",
"author_id": "LSsXyncAAAAJ" # Example author ID
}
})
# Set request headers
headers = {
'Content-Type': 'application/json'
}
# Making a POST request
conn.request("POST", "/api/v1/scraper/request", payload, headers)
# Get the response result
res = conn.getresponse()
# Read and output data
data = res.read()
print(data.decode("utf-8"))
The result returned by the API request is a JSON-formatted response containing detailed information about the academic author, such as name, academic field, institution, etc. The returned data also includes a list of the author's papers and citations. The following is a simplified example showing some of the results returned by the API:
{
"name": "John Doe",
"institution": "Harvard University",
"research_areas": [
{
"title": "Epigenetics",
"link": "https://scholar.google.com/citations?view_op=search_authors&hl=en&mauthors=label:epigenetics"
},
{
"title": "Gene Regulation",
"link": "https://scholar.google.com/citations?view_op=search_authors&hl=en&mauthors=label:gene_regulation"
},
{
"title": "Genomics",
"link": "https://scholar.google.com/citations?view_op=search_authors&hl=en&mauthors=label:genomics"
},
{
"title": "Transcription Factors",
"link": "https://scholar.google.com/citations?view_op=search_authors&hl=en&mauthors=label:transcription_factors"
}
],
"thumbnail": "https://www.google.com/citations/images/avatar_scholar_128.png",
"articles": [
{
"title": "Model-based analysis of ChIP-Seq (MACS)",
"link": "https://scholar.google.com/citations?view_op=view_citation&hl=en&user=LSsXyncAAAAJ&citation_for_view=LSsXyncAAAAJ:2osOgNQ5qMEC",
"citation_id": "LSsXyncAAAAJ:2osOgNQ5qMEC",
"authors": "Y Zhang, T Liu, CA Meyer, J Eeckhoute, DS Johnson, BE Bernstein, ...",
"publication": "Genome biology 9, 1-9, 2008",
"cited_by": {
"value": 17091,
"link": "https://scholar.google.com/scholar?oi=bibs&hl=en&cites=14252090027271643524"
},
"year": "2008"
},
{
"title": "Genome-wide analysis of estrogen receptor binding sites",
"link": "https://scholar.google.com/citations?view_op=view_citation&hl=en&user=LSsXyncAAAAJ&citation_for_view=LSsXyncAAAAJ:9yKSN-GCB0IC",
"citation_id": "LSsXyncAAAAJ:9yKSN-GCB0IC",
"authors": "JS Carroll, CA Meyer, J Song, W Li, TR Geistlinger, J Eeckhoute, ...",
"publication": "Nature genetics 38 (11), 1289-1297, 2006",
"cited_by": {
"value": 1653,
"link": "https://scholar.google.com/scholar?oi=bibs&hl=en&cites=7951096779388712529"
},
"year": "2006"
}
]
}
The response data contains detailed author information and research results. The specific result fields include:
- research_areas: Lists the author's research areas, each of which has a corresponding link pointing to the search results on Google Scholar.
- thumbnail: URL of the author's avatar image.
- articles: Contains the author's main academic articles. The information of each article includes title, link, author, publication journal, number of citations, etc.
For example, the detailed information of an article is as follows:
- title: Article title
- link: Article link, pointing to the detailed citation page on Google Scholar
- citation_id: The unique citation ID of the article in Google Scholar
- authors: List of all participating authors
- publication: The journal in which the article is published and its volume and issue number
- cited_by: The number of times the article is cited, and a link to the citation page is provided
This information is very helpful for academic researchers and developers to analyze the author's academic contributions.
Scrapeless Google Scholar Cite API
Scrapeless's Google Scholar Cite API is a powerful tool that allows users to extract citation information for scholarly articles directly from Google Scholar. It can retrieve formatted citations in multiple formats, including MLA, APA, Chicago, Harvard, and Vancouver, providing convenience for researchers, students, and developers.
In addition, Scrapeless's Google Scholar Cite API also provides export links for different citation management tools (such as BibTeX, EndNote, RefMan, and RefWorks), enabling seamless integration with reference workflows. With real-time data retrieval and structured JSON output, Scrapeless's Google Scholar Cite API simplifies the citation process and improves academic research efficiency.
Google Scholar Cite API Parameters
Parameter | Required | Description |
---|---|---|
engine | TRUE | Set to google_scholar_cite. |
q | TRUE | ID of an article to retrieve citations. |
hl | FALSE | Language setting (default: en). |
Example: Extracting Citation Details
Here is a Python code example demonstrating how to make a request to the Scrapeless Google Scholar Cite API.
import http.client
import json
conn = http.client.HTTPSConnection("api.scrapeless.com")
payload = json.dumps({
"actor": "scraper.google.scholar",
"input": {
"engine": "google_scholar_cite",
"q": "s1QWFy06YAYJ"
}
})
headers = {
'Content-Type': 'application/json'
}
conn.request("POST", "/api/v1/scraper/request", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Example Result Code
The API responds with a JSON object containing citation details in multiple formats:
{
"citations": [
{
"title": "MLA",
"snippet": "Masters, Paul S. \"The molecular biology of coronaviruses.\" Advances in virus research 66 (2006): 193-292."
},
{
"title": "APA",
"snippet": "Masters, P. S. (2006). The molecular biology of coronaviruses. Advances in virus research, 66, 193-292."
},
{
"title": "Chicago",
"snippet": "Masters, Paul S. \"The molecular biology of coronaviruses.\" Advances in virus research 66 (2006): 193-292."
},
{
"title": "Harvard",
"snippet": "Masters, P.S., 2006. The molecular biology of coronaviruses. Advances in virus research, 66, pp.193-292."
},
{
"title": "Vancouver",
"snippet": "Masters PS. The molecular biology of coronaviruses. Advances in virus research. 2006 Jan 1;66:193-292."
}
],
"links": [
{
"name": "BibTeX",
"link": "https://scholar.googleusercontent.com/scholar.bib?q=info:s1QWFy06YAYJ:scholar.google.com/&output=citation"
},
{
"name": "EndNote",
"link": "https://scholar.googleusercontent.com/scholar.enw?q=info:s1QWFy06YAYJ:scholar.google.com/&output=citation"
},
{
"name": "RefMan",
"link": "https://scholar.googleusercontent.com/scholar.ris?q=info:s1QWFy06YAYJ:scholar.google.com/&output=citation"
},
{
"name": "RefWorks",
"link": "https://scholar.googleusercontent.com/scholar.rfw?q=info:s1QWFy06YAYJ:scholar.google.com/&output=citation"
}
]
}
The Scrapeless Google Scholar Cite API returns a JSON structure containing two main sections: citations (citation information) and links (citation format export links). Below is a detailed analysis of each field:
1. Citations
This field is an array where each element represents a different citation format, including MLA, APA, Chicago, Harvard, and Vancouver. Each format includes the following fields:
| Field Name | Data Type | Description |
|------------|-----------|-------------------------------------------------------------------|
| title | String | The name of the citation format, such as "MLA" or "APA" |
| snippet | String | The citation text in the respective format, including article title, author, journal information, etc. |
Example Data:
"citations": [
{
"title": "MLA",
"snippet": "Masters, Paul S. \"The molecular biology of coronaviruses.\" Advances in virus research 66 (2006): 193-292."
},
{
"title": "APA",
"snippet": "Masters, P. S. (2006). The molecular biology of coronaviruses. Advances in virus research, 66, 193-292."
}
]
Data Analysis:
- title: "MLA" indicates that this entry follows the MLA citation format.
- snippet: The citation text includes the author Paul S. Masters, the article title The molecular biology of coronaviruses, the journal Advances in Virus Research, volume 66, and pages 193-292.
2. Links (Citation Export Links)
This field is an array where each element provides a link for exporting the citation in different formats such as BibTeX, EndNote, RefMan, and RefWorks. Each format includes the following fields:
| Field Name | Data Type | Description |
|------------|-----------|-------------------------------------------------------------------|
| name | String | The name of the citation format, such as "BibTeX" or "EndNote" |
| link | String | A URL that allows users to directly download the citation in the specified format |
Example Data:
"links": [
{
"name": "BibTeX",
"link": "https://scholar.googleusercontent.com/scholar.bib?q=info:s1QWFy06YAYJ..."
},
{
"name": "EndNote",
"link": "https://scholar.googleusercontent.com/scholar.enw?q=info:s1QWFy06YAYJ..."
}
]
Data Analysis:
- name: "BibTeX" indicates that this entry is a BibTeX citation export link.
- link: "https://scholar.googleusercontent.com/scholar.bib?q=info:s1QWFy06YAYJ..." is a direct URL to access the BibTeX citation, which can be used in LaTeX or other reference management tools.
Scrapeless Google Scholar Profiles API
The Scrapeless Google Scholar Profiles API allows users to search for Google Scholar profiles based on author names and fetch detailed information, including citations, interests, affiliations, and more. Below is an overview of how to use the API, the parameters involved, and how to interpret the results.
Google Scholar Profiles API Parameters
Parameter | Required | Description |
---|---|---|
engine | TRUE | Set to google_scholar_profiles. |
mauthors | TRUE | Author name for profile search. |
hl | FALSE | Language setting (default: en). |
after_author | FALSE | Token for pagination. |
Example: Searching for an Author Profile
The following Python code demonstrates how to make an API request using the Scrapeless service:
import http.client
import json
conn = http.client.HTTPSConnection("api.scrapeless.com")
payload = json.dumps({
"actor": "scraper.google.scholar",
"input": {
"engine": "google_scholar_author",
"author_id": "LSsXyncAAAAJ"
}
})
headers = {
'Content-Type': 'application/json'
}
conn.request("POST", "/api/v1/scraper/request", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
The API returns a JSON object with information about the search results. Here is an example:
{
"pagination": {
"next": "https://scholar.google.com//citations?view_op=search_authors&hl=en&mauthors=Mike&after_author=pnnfAUQM__8J&astart=10",
"next_page_token": "pnnfAUQM__8J"
},
"profiles": [
{
"name": "Mike Robb",
"link": "https://scholar.google.com//citations?hl=en&user=kq0NYnMAAAAJ",
"author_id": "kq0NYnMAAAAJ",
"affiliations": "Chemistry Department Imperial College",
"email": "Verified email at imperial.ac.uk",
"cited_by": 230346,
"interests": [
{
"title": "Computational chemistry",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:computational_chemistry"
},
{
"title": "Theoretical Chemistry",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:theoretical_chemistry"
},
{
"title": "conical intersections",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:conical_intersections"
},
{
"title": "non adiabatic dynamics",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:non_adiabatic_dynamics"
}
],
"thumbnail": "https://scholar.google.com//citations?hl=en&user=kq0NYnMAAAAJ"
},
{
"name": "Mike A. Nalls",
"link": "https://scholar.google.com//citations?hl=en&user=ZjfgPLMAAAAJ",
"author_id": "ZjfgPLMAAAAJ",
"affiliations": "Founder/consultant with Data Tecnica International + Data science lead at NIH's Center for …",
"email": "Verified email at mail.nih.gov",
"cited_by": 175760,
"interests": [
{
"title": "statistical genetics",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:statistical_genetics"
},
{
"title": "neurodegeneration",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:neurodegeneration"
},
{
"title": "data science",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:data_science"
},
{
"title": "biostatistics",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:biostatistics"
},
{
"title": "genomics",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:genomics"
}
],
"thumbnail": "https://scholar.google.com//citations?hl=en&user=ZjfgPLMAAAAJ"
},
{
"name": "mike wright",
"link": "https://scholar.google.com//citations?hl=en&user=RIg9DVEAAAAJ",
"author_id": "RIg9DVEAAAAJ",
"affiliations": "imperial college",
"email": "Verified email at imperial.ac.uk",
"cited_by": 131474,
"interests": [
{
"title": "entrepreneurship",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:entrepreneurship"
}
],
"thumbnail": "https://scholar.google.com//citations?hl=en&user=RIg9DVEAAAAJ"
},
{
"name": "Mike Lean (MEJ Lean)",
"link": "https://scholar.google.com//citations?hl=en&user=R8PPdbQAAAAJ",
"author_id": "R8PPdbQAAAAJ",
"affiliations": "Professor of Human Nutrition, University of Glasgow",
"email": "Verified email at glasgow.ac.uk",
"cited_by": 98488,
"interests": [
{
"title": "Food",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:food"
},
{
"title": "Nutrition",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:nutrition"
},
{
"title": "Obesity",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:obesity"
},
{
"title": "Diabetes",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:diabetes"
},
{
"title": "CHD",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:chd"
}
],
"thumbnail": "https://scholar.google.com//citations?hl=en&user=R8PPdbQAAAAJ"
},
{
"name": "Mike Schuster",
"link": "https://scholar.google.com//citations?hl=en&user=L9lS9_AAAAAJ",
"author_id": "L9lS9_AAAAAJ",
"affiliations": "Two Sigma",
"email": "Verified email at twosigma.com",
"cited_by": 96241,
"interests": [
{
"title": "machine learning",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:machine_learning"
},
{
"title": "neural networks",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:neural_networks"
},
{
"title": "deep learning",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:deep_learning"
},
{
"title": "reinforcement learning",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:reinforcement_learning"
}
],
"thumbnail": "https://scholar.google.com//citations?hl=en&user=L9lS9_AAAAAJ"
},
{
"name": "prof dr ir Mike SM Jetten",
"link": "https://scholar.google.com//citations?hl=en&user=iXjCKTgAAAAJ",
"author_id": "iXjCKTgAAAAJ",
"affiliations": "Radboud University, Microbiology, Nijmegen, Netherlands",
"email": "Verified email at science.ru.nl",
"cited_by": 88336,
"interests": [
{
"title": "anaerobic microbiology",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:anaerobic_microbiology"
},
{
"title": "nitrogen cycle",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:nitrogen_cycle"
},
{
"title": "methane archaea",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:methane_archaea"
},
{
"title": "anammox",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:anammox"
}
],
"thumbnail": "https://scholar.google.com//citations?hl=en&user=iXjCKTgAAAAJ"
},
{
"name": "Mike Wingfield",
"link": "https://scholar.google.com//citations?hl=en&user=wT4V7isAAAAJ",
"author_id": "wT4V7isAAAAJ",
"affiliations": "Professor, Forestry and Agricultural Biotechnology Institute (FABI), University of Pretoria",
"email": "Verified email at fabi.up.ac.za",
"cited_by": 82076,
"interests": [
{
"title": "forest protection",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:forest_protection"
},
{
"title": "mycology",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:mycology"
},
{
"title": "entomology",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:entomology"
},
{
"title": "biotechnology",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:biotechnology"
}
],
"thumbnail": "https://scholar.google.com//citations?hl=en&user=wT4V7isAAAAJ"
},
{
"name": "Mike Lewis",
"link": "https://scholar.google.com//citations?hl=en&user=SnQnQicAAAAJ",
"author_id": "SnQnQicAAAAJ",
"affiliations": "Facebook AI Research",
"email": "Verified email at fb.com",
"cited_by": 73932,
"interests": [
{
"title": "Natural language processing",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:natural_language_processing"
},
{
"title": "machine learning",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:machine_learning"
},
{
"title": "linguistics",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:linguistics"
}
],
"thumbnail": "https://scholar.google.com//citations?hl=en&user=SnQnQicAAAAJ"
},
{
"name": "Mike W. Peng",
"link": "https://scholar.google.com//citations?hl=en&user=z1Kz8gQAAAAJ",
"author_id": "z1Kz8gQAAAAJ",
"affiliations": "Jindal Chair of Global Strategy, University of Texas at Dallas",
"email": "Verified email at utdallas.edu",
"cited_by": 67576,
"interests": [
{
"title": "International Business",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:international_business"
},
{
"title": "Global Strategy",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:global_strategy"
},
{
"title": "Strategic Management",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:strategic_management"
}
],
"thumbnail": "https://scholar.google.com//citations?hl=en&user=z1Kz8gQAAAAJ"
},
{
"name": "Mike Hulme",
"link": "https://scholar.google.com//citations?hl=en&user=uQJsUvEAAAAJ",
"author_id": "uQJsUvEAAAAJ",
"affiliations": "University of Cambridge",
"email": "Verified email at cam.ac.uk",
"cited_by": 62393,
"interests": [
{
"title": "Climate Change",
"link": "https://scholar.google.com//citations?hl=en&view_op=search_authors&mauthors=label:climate_change"
}
],
"thumbnail": "https://scholar.google.com//citations?hl=en&user=uQJsUvEAAAAJ"
}
]
}
The results are structured as follows:
- pagination: Contains the next URL and next_page_token, which can be used for paginated results.
- profiles: An array of profiles matching the author's search. Each profile includes:
- name: The name of the author.
- link: The link to the author's full Google Scholar profile.
- author_id: A unique identifier for the author.
- affiliations: The author's institutional affiliation.
- email: The author's verified email address.
- cited_by: The total number of citations for the author.
- interests: A list of topics or research areas with links to relevant author searches.
- thumbnail: The URL of the author's profile picture.
By using Scrapeless Google Scholar Profiles API, developers can easily integrate Google Scholar profile data into their applications, allowing for the exploration of academic research and author-specific information.
Conclusion
Scraping Google Scholar manually is difficult due to Google’s strict anti-bot measures. Instead, Scrapeless Google Scholar API provides an efficient, reliable, and CAPTCHA-free way to extract Google Scholar data. Whether you need search results, author profiles, citation details, or publication metadata, Scrapeless Google Scholar API covers all use cases.
🔹 Why Use Scrapeless API?
âś… Avoids IP bans and CAPTCHAs
âś… Provides structured JSON output
âś… Supports various endpoints for citations, authors, and search results
🚀 Start using Scrapeless API today to extract Google Scholar data seamlessly!
FAQ about Google Scholar API
Q1: Is Scrapeless API free?
Answer:Scrapeless offers a free trial, after which a subscription is required. You can join our Discord and contact our team to claim your free trial of Scrapeless. After the trial, a subscription will be required.
Q2: Can I scrape Google Scholar manually?
Answer: Yes, but it is easy to be blocked. Scrapeless API is more stable.
Q3: What kind of data can I scrape from Google Scholar?
Answer: With the right tools, you can scrape various types of data from Google Scholar, including publication titles, authors, citation counts, publication years, and abstracts. This data is particularly useful for researchers, analysts, and developers who need access to large volumes of academic information for analysis, trend monitoring, or citation tracking.
This content originally appeared on DEV Community and was authored by datacollection
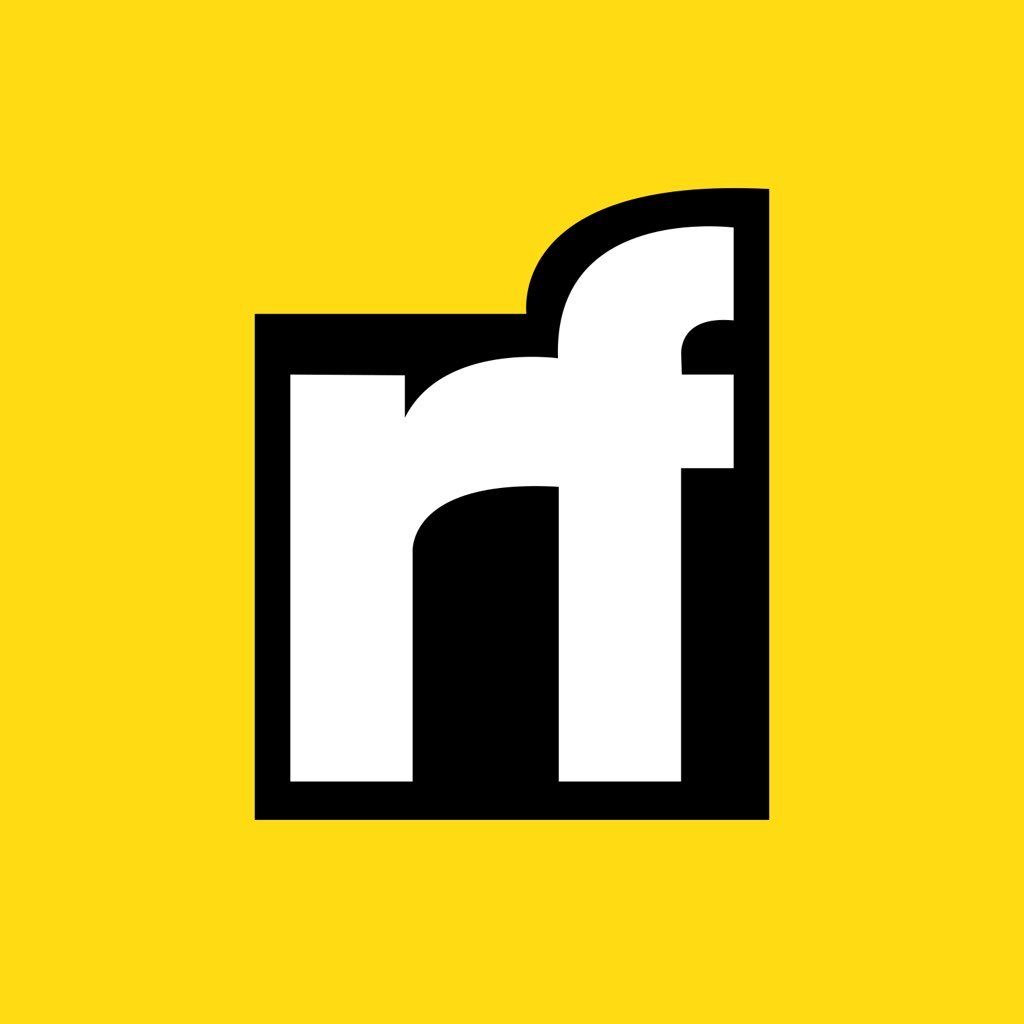
datacollection | Sciencx (2025-02-20T04:28:26+00:00) How to Scrape Google Scholar Results. Retrieved from https://www.scien.cx/2025/02/20/how-to-scrape-google-scholar-results/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.