This content originally appeared on Telerik Blogs and was authored by Hassan Djirdeh
See how to handle asynchronous behavior with Angular resource and rxResource APIs.
Angular 19 introduces two experimental APIs—resource
and rxResource
—designed to simplify handling asynchronous dependencies within Angular’s reactive framework. These APIs elegantly manage evolving data, such as API responses or other async operations, by tightly integrating with Angular signals.
In this article, we’ll explore these new APIs, showcase their use cases through some examples and provide insights into how they enhance asynchronous workflows.
Resource API
The resource API bridges Angular’s signal-based state management with asynchronous operations. Combining request signals, loader functions and resource instances provides a declarative way to manage asynchronous data.
For an introduction to Angular Signals, be sure to check out the articles we’ve recently published—Angular Basics: Signals and Angular Basics: Input, Output, and View Queries.
Imagine a scenario where we want to fetch weather details based on a city name.
import { Component, signal, resource } from '@angular/core';
@Component({
selector: 'app-weather-info',
templateUrl: './weather-info.component.html',
styleUrls: ['./weather-info.component.css']
})
export class WeatherInfoComponent {
city = signal<string>('New York');
weatherData = /* fetch weather details */
}
Using resource
, the setup becomes reactive and streamlined:
import { Component, signal, resource } from "@angular/core";
@Component({
selector: "app-weather-info",
templateUrl: "./weather-info.component.html",
styleUrls: ["./weather-info.component.css"],
})
export class WeatherInfoComponent {
city = signal<string>("New York");
weatherData = resource({
request: this.city,
loader: async ({ request: cityName }) => {
const response = await fetch(
`https://api.weatherapi.com/v1/current.json?q=${cityName}`
);
if (!response.ok) {
throw new Error("Failed to fetch weather details");
}
return await response.json();
},
});
updateCity(newCity: string): void {
this.city.set(newCity);
}
}
In the above example, the city
signal acts as the input, determining which weather details to fetch. Any updates to city
automatically retrigger the loader function, fetching new data based on the updated city name. The weatherData
resource instance tracks the current data (value
), loading status (isLoading
) and any errors (error
).
Dynamic Updates Without Fetching
The update
method allows modifying local resource data without triggering a server request. For instance, we can add a local timestamp to the weather data:
this.weatherData.update((data) => {
if (!data) return undefined;
return { ...data, timestamp: new Date().toISOString() };
});
This capability ensures immediate UI updates while preserving reactivity.
rxResource
For applications deeply integrated with RxJS, rxResource
provides an observable-based counterpart to resource
. It seamlessly connects signals to observables, enabling a more reactive approach to data fetching.
Suppose we want to display a paginated list of books in a library system.
import { Component, signal } from '@angular/core';
import { rxResource } from '@angular/core/rxjs-interop';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-library',
templateUrl: './library.component.html',
styleUrls: ['./library.component.css']
})
export class LibraryComponent {
page = signal<number>(1);
books = /* fetch books */
}
The rxResource
function makes this simple:
import { Component, signal } from "@angular/core";
import { rxResource } from "@angular/core/rxjs-interop";
import { HttpClient } from "@angular/common/http";
@Component({
selector: "app-library",
templateUrl: "./library.component.html",
styleUrls: ["./library.component.css"],
})
export class LibraryComponent {
page = signal<number>(1);
books = rxResource({
request: this.page,
loader: (params) =>
this.http.get<Book[]>(
`https://api.example.com/books?page=${params.request}`
),
});
constructor(private http: HttpClient) {}
updatePage(newPage: number): void {
this.page.set(newPage);
}
}
Here, the page
signal represents the current page number. Changes to page
automatically trigger the loader function to fetch books for the specified page. Using rxResource
enables Angular’s reactive model to integrate smoothly with observable streams.
For an introduction to observables in Angular, check the following articles: Angular Basics: Introduction to Observables (RxJS)—Part 1 and Angular Basics: Introduction to Observables (RxJS)—Part 2.
Wrap-up
The resource
and rxResource
APIs offer a declarative and reactive approach to managing asynchronous workflows in Angular. They address challenges like race conditions, integrated state tracking and request cancellations while enabling seamless updates tied to signal changes.
These APIs enhance the framework’s reactive capabilities by tightly coupling Angular’s signal system with asynchronous data handling. Though experimental, they represent a forward-thinking approach to handling dynamic data and promise to become essential tools in Angular’s asynchronous programming landscape. For more details on resource
and rxResource
, be sure to check out the following resources:
This content originally appeared on Telerik Blogs and was authored by Hassan Djirdeh
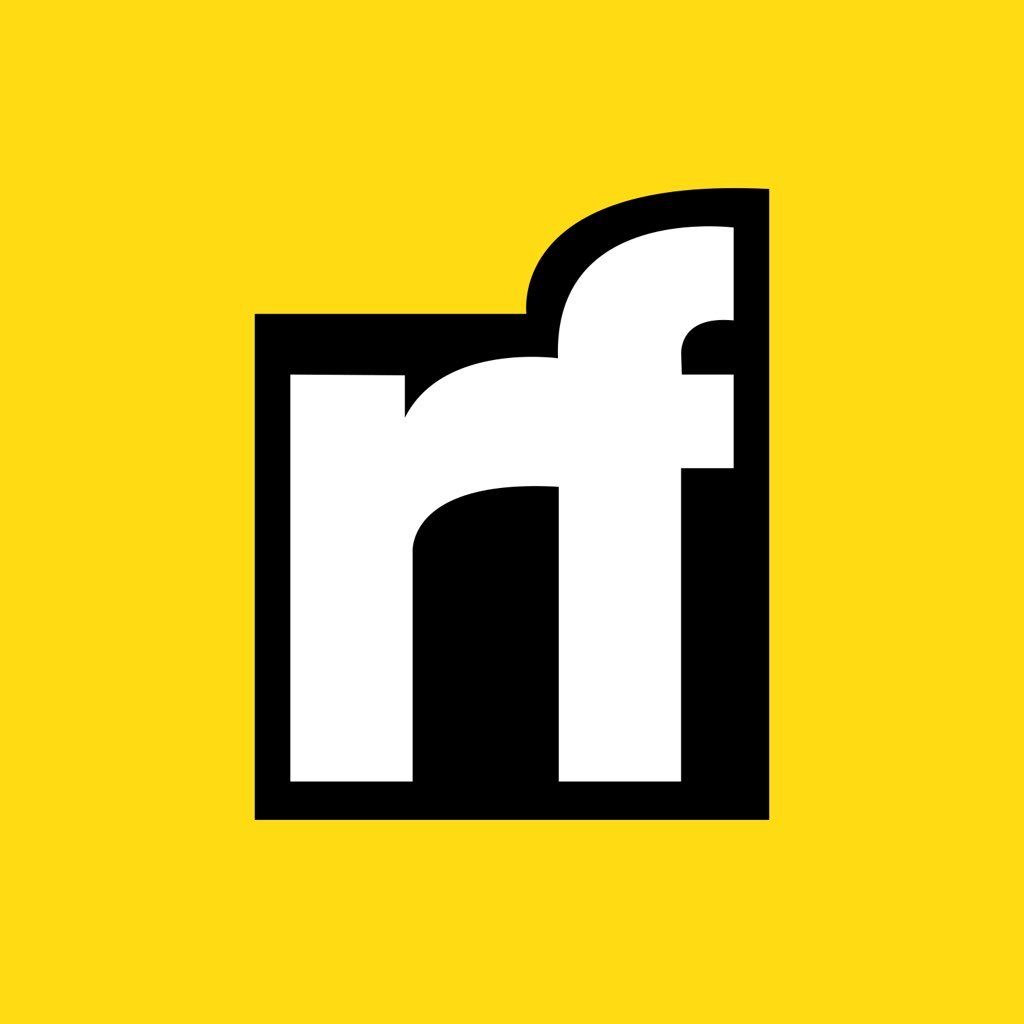
Hassan Djirdeh | Sciencx (2025-02-24T12:19:05+00:00) Angular Resource and rxResource. Retrieved from https://www.scien.cx/2025/02/24/angular-resource-and-rxresource/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.