This content originally appeared on Level Up Coding - Medium and was authored by Mundhraumang
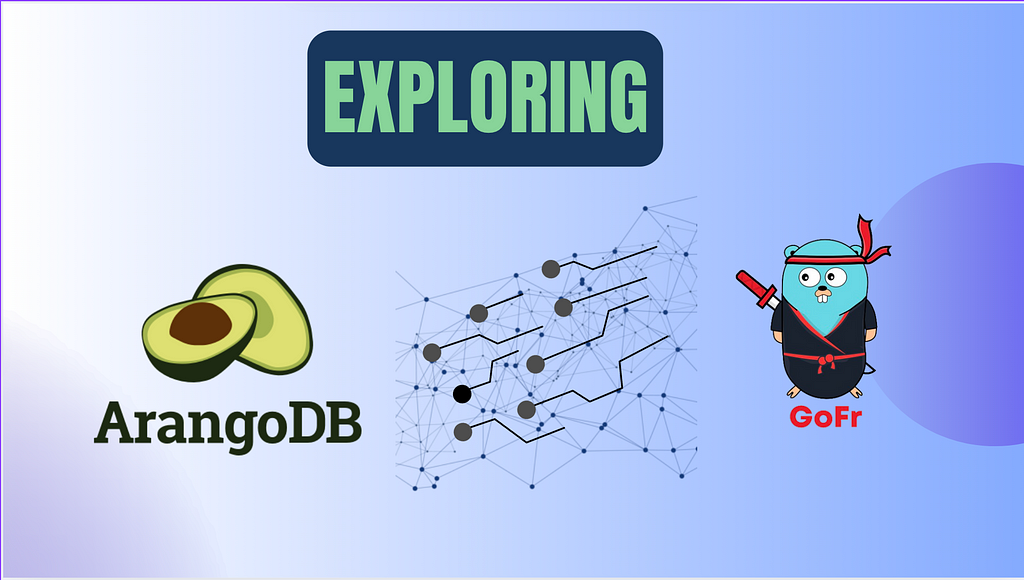
Introduction:
In today’s fast-paced development landscape, applications often demand a diverse range of database solutions to address their unique challenges. The choice of database plays a crucial role in determining the scalability, performance, and flexibility of an application. While traditional relational databases work well for structured data, many modern use cases demand more than just tables and rows.
In this article, we explore ArangoDB, a powerful multi-model database that supports document, key-value, and graph-based data storage within a single system. We will break down its core components — databases, collections, documents, graphs, and edges — and understand how it can be used in real-world scenarios. Finally, we’ll get hands-on and demonstrate how seamlessly you can integrate and use ArangoDB within the GoFr framework, making your development process even smoother. Whether you’re a seasoned developer or just starting your database journey, this article will provide you with a solid understanding of ArangoDB and its potential.
What is ArangoDB?
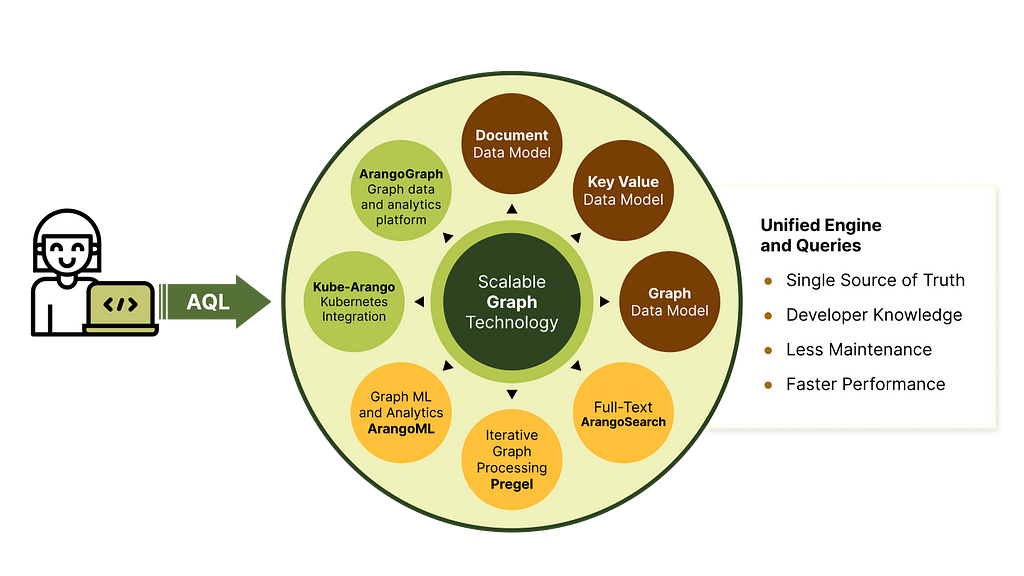
ArangoDB is a powerful, multi-model NoSQL database that offers a unique blend of flexibility and scalability. It’s designed to handle various data types and relationships efficiently, making it a great choice for modern applications. It enables developers to work with connected data faster and more efficiently through a single, composable query language. What sets ArangoDB apart is its ability to seamlessly integrate graph, document, and key/value data models within a single database. This means you can manage different types of data without juggling multiple database systems.
ArangoDB is a native multi-model database supporting three data models:
Key-Value Model
Each document has a unique _key that serves as a primary identifier, making it easy to retrieve data.
Document Model
Documents are self-contained JSON objects stored in collections. Queries can access attributes granularly, filter, and aggregate data.
Graph Model
ArangoDB supports labeled property graphs where:
- Vertices are documents stored in document collections.
- Edges are documents stored in edge collections, containing _from and _to references.
Edges are directed, meaning relationships follow a specific direction. However, queries can traverse graphs in inbound, outbound, or any direction.
Think of it like this: Imagine you’re building a social network application. You have users (documents), their connections (edges in a graph), and perhaps some simple key-value pairs for user settings. ArangoDB can handle all of this within one database.
ArangoDB Core Structure:
At its heart, ArangoDB stores information as JSON objects, which are organized in a hierarchical structure:Let’s break down the key components using our social network example:
- Databases: Collections are grouped within databases, allowing logical separation of data. Each ArangoDB instance contains at least one database, _system, which manages database-related operations. In our example, we might have a database called social_network. Databases provide isolation, so you can have multiple, separate sets of data on the same ArangoDB server.
- Collections: Collections are like tables in a traditional relational database. Documents are organized in collections, which function like folders. In our social network, we might have a users collection (for user profiles) and a friendships collection (for connections between users). Collections can be of two types:
- Document Collections: Store JSON documents (vertices in graphs)
- Edge Collections: Store relationships between documents using _from and _to attributes. - Documents: Documents are the heart of ArangoDB. ArangoDB stores. data as JSON objects, each referred to as a document. Every document contains key-value pairs known as attributes, which can hold various data types. A user document might look like this:
{ "name": "Alice",
"age": 30,
"city": "New York",
"interests": ["reading", "hiking"]
}
Documents are stored internally in a binary format called VelocyPack for efficiency.
Use Cases: How Companies Leverage ArangoDB in Production:
ArangoDB’s flexibility and performance have made it the database of choice for organizations across various industries. Let’s explore how real companies are solving complex data challenges with ArangoDB.
- InfoCamere — Analyzing Company Relationships: InfoCamere needed to understand the complex ownership relationships between Italian companies. After evaluating various databases, they chose ArangoDB’s graph database for its ability to handle complex graph data efficiently. It outperformed alternatives like Neo4j and PostgreSQL, making it their preferred choice for managing company equity data.
- Boosting Application Performance (IC Manage): IC Manage, a provider of design management solutions, struggled with performance limitations in their existing relational database. They switched to ArangoDB to power their next-generation product, nGDP, benefiting from its graph and document model. This transition led to a 2000x performance improvement, simplified their codebase, and enabled faster feature development.
- Key2Publish — Scaling Product Information Management: Key2Publish developed a webshop and Product Information Management (PIM) system for ITK Diagnostics, managing over 200,000 products. They chose ArangoDB for its speed, flexibility, and ability to handle large datasets efficiently. ArangoDB’s fast query response times and extensive documentation made it the perfect fit.
- FlightStats — Harmonizing Aviation Data: FlightStats, a data services company in the aviation industry, needed a database that could store and process fragmented reference data from multiple sources. ArangoDB’s multi-model approach allowed them to structure data efficiently, improve API access, and scale as their data needs evolved. It became a crucial component in ensuring accurate and up-to-date aviation information.
These examples highlight how ArangoDB has helped businesses across different industries solve complex data challenges with its flexible and high-performance architecture.
ArangoDB with GoFr: Simplifying Database Interactions
When building applications with ArangoDB, developers need a framework that makes database interactions seamless while providing enterprise-grade features. GoFr, a lightweight cloud-native framework for Go, offers exactly that. GoFr prioritizes simplicity, ease of use, and developer productivity.
Why Use GoFr with ArangoDB?
GoFr makes working with ArangoDB easier and more efficient, providing built-in features that handle common tasks like observability and database migrations. This allows you to focus on building your application logic, not managing database infrastructure. Let’s explore how GoFr simplifies working with ArangoDB and why it’s an excellent choice for your next project.
- Built-in Observability: GoFr’s ArangoDB client has observability integrated right out of the box. This means metrics, tracing, and logging are already set up, allowing you to easily monitor and debug your database interactions. No more manual configuration — just use GoFr’s logging and tracing capabilities.
- Simplified Migrations: GoFr supports ArangoDB migrations, making it easy to manage database schema changes. You can define your database structure (collections, graphs, etc.) in migration files, and GoFr will handle the creation and updates. This keeps your database schema organized and ensures consistency across environments.
- Clean Abstraction: GoFr provides a clean and consistent interface for interacting with ArangoDB. The gofr.Context()gives you access to the ArangoDB client, simplifying database operations within your handlers. This abstraction makes your code cleaner and easier to maintain.
- Easy Dependency Injection: GoFr supports dependency injection, making it easy to swap out different ArangoDB clients or even your own arangoDB client if needed. This promotes testability and flexibility in your application’s architecture.
Example Usage:
Let’s illustrate how easy it is to use ArangoDB with GoFr using a simple social network example. We’ll create users, define friendships (edges), and retrieve friends.
- Prerequisites:
Before we begin, ensure you have the following:
- Go 1.21 or above: Verify your Go installation with:
go version
2. Docker installed for running ArrangoDB locally.
- Setting up the project:
Let’s start by initializing a new Go module and adding the required dependencies:
# Initialize a new Go module
go mod init example.com
# Add GoFr to your project
go get gofr.dev
# Add GoFr's ArangoDB driver
go get gofr.dev/pkg/gofr/datasource/arangodb@latest
- Run ArangoDB using Docker:
docker run -d --name arangodb \
-p 8529:8529 \
-e ARANGO_ROOT_PASSWORD=root \
--pull always \
arangodb:latest
- Creating the Main Application File:
Create a file named main.go with the following content:
package main
import (
"fmt"
"example.com/migrations"
"gofr.dev/pkg/gofr"
"gofr.dev/pkg/gofr/datasource/arangodb"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
func main() {
app := gofr.New()
// Configure the ArangoDB client
arangoClient := arangodb.New(arangodb.Config{
Host: "localhost",
User: "root",
Password: "root",
Port: 8529,
})
app.AddArangoDB(arangoClient)
app.Migrate(migrations.All())
// Example routes demonstrating different types of operations
app.POST("/setup", Setup)
app.POST("/users/{name}", CreateUserHandler)
app.POST("/friends", CreateFriendship)
app.GET("/friends/{collection}/{vertexID}", GetEdgesHandler)
app.Run()
}
// Setup demonstrates database and collection creation
func Setup(ctx *gofr.Context) (interface{}, error) {
// Define and create the graph
edgeDefs := arangodb.EdgeDefinition{
{Collection: "friendships", From: []string{"employees"}, To: []string{"employees"}},
}
err := ctx.ArangoDB.CreateGraph(ctx, "employee_db", "social_graph", &edgeDefs)
if err != nil {
return nil, fmt.Errorf("failed to create graph: %w", err)
}
return "Setup completed successfully", nil
}
// CreateUserHandler demonstrates user management and document creation
func CreateUserHandler(ctx *gofr.Context) (interface{}, error) {
name := ctx.PathParam("name")
// Create a person document
person := Person{
Name: name,
Age: 25,
}
docID, err := ctx.ArangoDB.CreateDocument(ctx, "employee_db", "employees", person)
if err != nil {
return nil, fmt.Errorf("failed to create person document: %w", err)
}
return map[string]string{
"message": "User created successfully",
"docID": docID,
}, nil
}
// CreateFriendship demonstrates edge document creation
func CreateFriendship(ctx *gofr.Context) (interface{}, error) {
var req struct {
From string `json:"from"`
To string `json:"to"`
StartDate string `json:"startDate"`
}
if err := ctx.Bind(&req); err != nil {
return nil, err
}
edgeDocument := map[string]any{
"_from": fmt.Sprintf("employees/%s", req.From),
"_to": fmt.Sprintf("employees/%s", req.To),
"startDate": req.StartDate,
}
// Create an edge document for the friendship
edgeID, err := ctx.ArangoDB.CreateDocument(ctx, "employee_db", "friendships", edgeDocument)
if err != nil {
return nil, fmt.Errorf("failed to create friendship: %w", err)
}
return map[string]string{
"message": "Friendship created successfully",
"edgeID": edgeID,
}, nil
}
// GetEdgesHandler demonstrates fetching edges connected to a vertex
func GetEdgesHandler(ctx *gofr.Context) (interface{}, error) {
collection := ctx.PathParam("collection")
vertexID := ctx.PathParam("vertexID")
fullVertexID := fmt.Sprintf("%s/%s", collection, vertexID)
// Prepare a slice to hold edge details
edges := make(arangodb.EdgeDetails, 0)
// Fetch all edges connected to the given vertex
err := ctx.ArangoDB.GetEdges(ctx, "employee_db", "social_graph", "friendships",
fullVertexID, &edges)
if err != nil {
return nil, fmt.Errorf("failed to get edges: %w", err)
}
return map[string]interface{}{
"vertexID": vertexID,
"edges": edges,
}, nil
}
- Defining Migrations:
Create a directory called migrations and add a file named 20250210153000_create_collection.go:
package migrations
import (
"context"
"gofr.dev/pkg/gofr/migration"
)
func createCollection() migration.Migrate {
return migration.Migrate{
UP: func(d migration.Datasource) error {
// Create the database
err := d.ArangoDB.CreateDB(context.Background(), "employee_db")
if err != nil {
return err
}
// Create a vertex collection for employees
err = d.ArangoDB.CreateCollection(context.Background(), "employee_db", "employees", false)
if err != nil {
return err
}
// Create an edge collection for friendships
err = d.ArangoDB.CreateCollection(context.Background(), "employee_db", "friendships", true)
if err != nil {
return err
}
return nil
},
}
}
func All() []migration.Migrate {
return []migration.Migrate{
createCollection(),
}
}
- Running and Testing Your Application:
Run your application using following command:
go run main.go
Now, you can interact with your application using curl commands:
# Run the setup
curl -X POST http://localhost:9000/setup
# Create two users
curl -X POST http://localhost:9000/users/john
curl -X POST http://localhost:9000/users/jane
# Create a friendship between them
curl --location 'http://localhost:9000/friends' \
--header 'Content-Type: application/json' \
--data '{
"from": "john",
"to": "jane",
"startDate": "2023-01-01"
}'
# Get all of John's friendships
curl -X GET http://localhost:9000/friends/employees/john
Below is a screenshot of the traces for a GetByID request, captured from GoFr's tracer. This trace captures the entire lifecycle of the request, showing database interactions, latency, and execution flow:
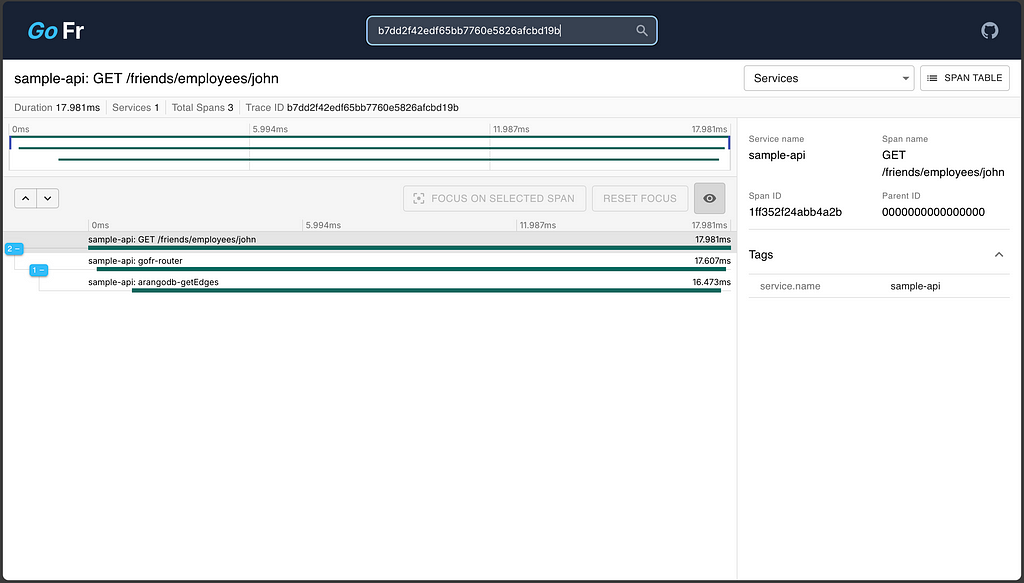
GoFr logs every request in a structured format, helping with debugging and monitoring. The following screenshot shows logs for different requests, demonstrating various log levels (INFO, ERROR, DEBUG, etc.):
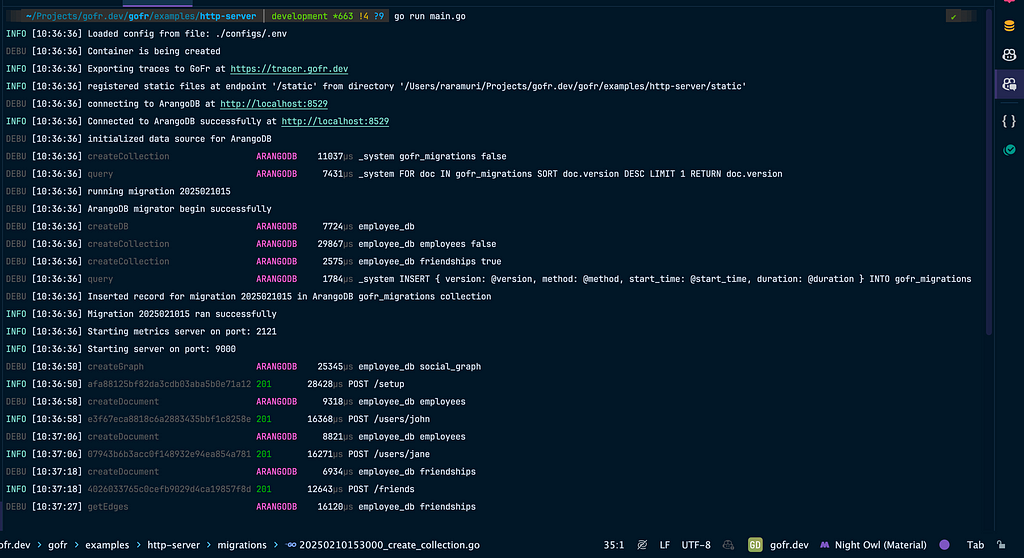
Conclusion:
In this article, we’ve explored ArangoDB, a powerful multi-model NoSQL database that offers a unique blend of flexibility and scalability. We’ve seen how ArangoDB’s ability to handle graph, document, and key/value data within a single database can simplify development and improve performance. Real-world examples illustrated its diverse applications, from managing complex relationships to scaling for massive datasets.
GoFr’s integration with ArangoDB demonstrates how a well-designed framework can simplify complex database operations. By providing a clean, consistent interface with built-in observability and migration support, GoFr enables developers to focus on business logic rather than database plumbing.
Whether you’re dealing with intricate data relationships, massive data volumes, or the need for flexible data models, ArangoDB, combined with the ease of use provided by GoFr, offers a compelling solution for modern application development. We encourage you to explore ArangoDB and GoFr further and discover how they can empower your next project.
Do checkout GoFr and it’s Github Repo and support it by giving it a ⭐.
Thank you for reading, Happy coding!
Navigating ArangoDB’s Multi-Model Magic with GoFr: An Informative and Practical Approach was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Mundhraumang
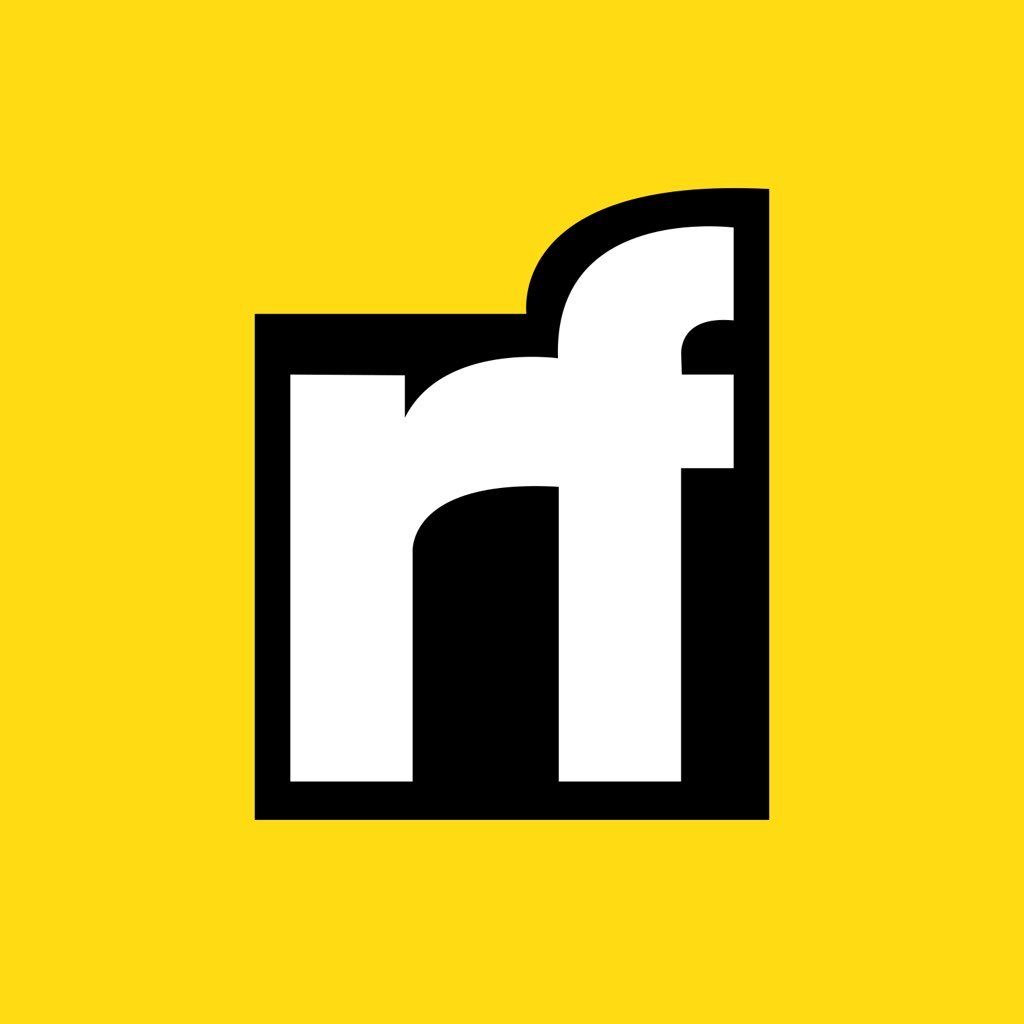
Mundhraumang | Sciencx (2025-02-24T13:41:55+00:00) Navigating ArangoDB’s Multi-Model Magic with GoFr: An Informative and Practical Approach. Retrieved from https://www.scien.cx/2025/02/24/navigating-arangodbs-multi-model-magic-with-gofr-an-informative-and-practical-approach/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.