This content originally appeared on DEV Community and was authored by Leandro Veiga
Debugging complex .NET applications is an art that combines technical know-how, patience, and the right set of tools. In this article, I'll share my most valuable lessons learned when tackling challenging debugging scenarios in large-scale .NET projects. From utilizing advanced Visual Studio features to employing robust logging libraries, this guide details practical strategies, code examples, and best practices that can help you resolve even the trickiest issues.
Table of Contents
- Introduction
- The Importance of Effective Debugging
- Essential Tools for Debugging .NET Applications
-
Techniques and Strategies for Debugging Complex Issues
- Breakpoints and Step Debugging
- Advanced Logging and Exception Handling
- Performance Profiling and Memory Analysis
- Real-World Debugging Scenarios and Lessons Learned
- Best Practices for Maintaining a Healthy Codebase
- Conclusion
Introduction
Debugging is more than just finding and fixing bugs; it's about understanding the behavior of your application under various conditions and uncovering hidden issues that could affect the performance and stability of your software. Through my experiences debugging complex .NET applications, I've learned that combining systematic approaches with modern tools can make the process both efficient and enlightening.
The Importance of Effective Debugging
Effective debugging can:
- Reduce downtime by quickly resolving production issues.
- Enhance code quality and maintainability.
- Provide insights into potential edge cases and performance bottlenecks.
- Improve overall team productivity by promoting best practices and knowledge sharing.
Essential Tools for Debugging .NET Applications
Using the right tools can be a game-changer. Here are some that I've found indispensable:
- Visual Studio Debugger: Provides powerful breakpoint, watch, and immediate window functionalities.
- Visual Studio Code with .NET Extensions: Lightweight alternative for quick debugging sessions.
- Exception and Logging Libraries: Libraries like Serilog, NLog, or log4net help record detailed logs.
- Profilers and Memory Analyzers: Tools such as JetBrains DotTrace, Visual Studio Diagnostic Tools, or PerfView are crucial for performance tuning.
- Decompilers: Tools like dotPeek or ILSpy help examine compiled assemblies when source code isn’t available.
Techniques and Strategies for Debugging Complex Issues
Breakpoints and Step Debugging
Breakpoints allow you to pause execution and inspect the state of your application line by line. This is the foundation of effective debugging. Here’s an example using Visual Studio:
public class OrderProcessor
{
public void Process(Order order)
{
// Set a breakpoint on the following line to inspect the order details.
if (order == null)
{
throw new ArgumentNullException(nameof(order));
}
// Step through the loop to monitor how each item is handled.
foreach (var item in order.Items)
{
ProcessItem(item);
}
}
private void ProcessItem(Item item)
{
// Use the Immediate Window to evaluate expressions or further step into this method.
// Additional processing logic here...
}
}
When debugging, use features such as conditional breakpoints to pause execution only under specific conditions.
Advanced Logging and Exception Handling
Logging is critical for reproducing issues that occur in production. Implementing a structured logging mechanism can make it easier to track down bugs. For example, using Serilog:
using Serilog;
public class PaymentService
{
public PaymentService()
{
Log.Logger = new LoggerConfiguration()
.WriteTo.Console()
.WriteTo.File("logs/payment_log.txt", rollingInterval: RollingInterval.Day)
.CreateLogger();
}
public void ProcessPayment(Payment payment)
{
try
{
// Payment processing logic
Log.Information("Processing payment for {CustomerId}", payment.CustomerId);
}
catch (Exception ex)
{
Log.Error(ex, "Error processing payment for {CustomerId}", payment.CustomerId);
throw;
}
}
}
This code not only logs operations but also captures exceptions with valuable context, making it easier to trace the root cause when something goes wrong.
Performance Profiling and Memory Analysis
When dealing with performance issues or memory leaks, profiling is indispensable. For example:
- Profiling CPU Usage: Use Visual Studio Diagnostic Tools or DotTrace to identify long-running methods and optimize them.
- Memory Analysis: Using tools like PerfView or the built-in memory diagnostic in Visual Studio helps find memory allocations and garbage collection issues.
Real-World Debugging Scenarios and Lessons Learned
Scenario 1: Intermittent API Timeouts
In a large-scale microservices architecture, we encountered intermittent API timeouts during peak loads. By enabling detailed logging and using a profiling tool, we discovered that a specific service had a misconfigured connection pool. The fix involved updating the configuration and adding better error handling to retry failed connections.
Scenario 2: Memory Leak in a Long-Running Application
A long-running background service experienced a gradual memory leak. Using a combination of memory profiling and logging, we identified that unmanaged resources were not being released. The solution was to implement the IDisposable pattern properly and ensure all unmanaged resources were disposed of in the service's shutdown routine.
Scenario 3: Data Inconsistencies in a Transactional System
Debugging data inconsistencies required deep analysis of the transaction flow. Debuggers and step-through inspection revealed timing issues in parallel processing, leading to race conditions. By applying locking and reviewing the transaction boundaries, we fixed the issue and ensured data integrity.
Best Practices for Maintaining a Healthy Codebase
- Write Structured, Meaningful Logs: Use logging frameworks to provide clarity when issues occur.
- Adopt Defensive Programming: Protect your code with proper null checks, exception handling, and input validations.
- Use Automated Testing and CI/CD Pipelines: Ensure that tests catch issues early and that debugging doesn't become reactive maintenance.
- Regular Code Reviews: Share debugging insights and best practices with your team to prevent recurring issues.
- Document Known Issues and Fixes: Maintain a centralized document for lessons learned, making it easier for the team to handle similar issues in the future.
Conclusion
Debugging complex .NET applications is challenging, but with the right tools, techniques, and experiences, you can manipulate even the most stubborn bugs. Remember, efficient debugging is about preparation—leveraging advanced IDE tools, logging frameworks, and profiling utilities to get to the root of any issue.
By applying these lessons learned and best practices, you'll not only solve immediate problems but also build a more robust and maintainable codebase for the future. Happy debugging!
Feel free to share your thoughts or additional debugging tips in the comments below. Let's learn together!
This content originally appeared on DEV Community and was authored by Leandro Veiga
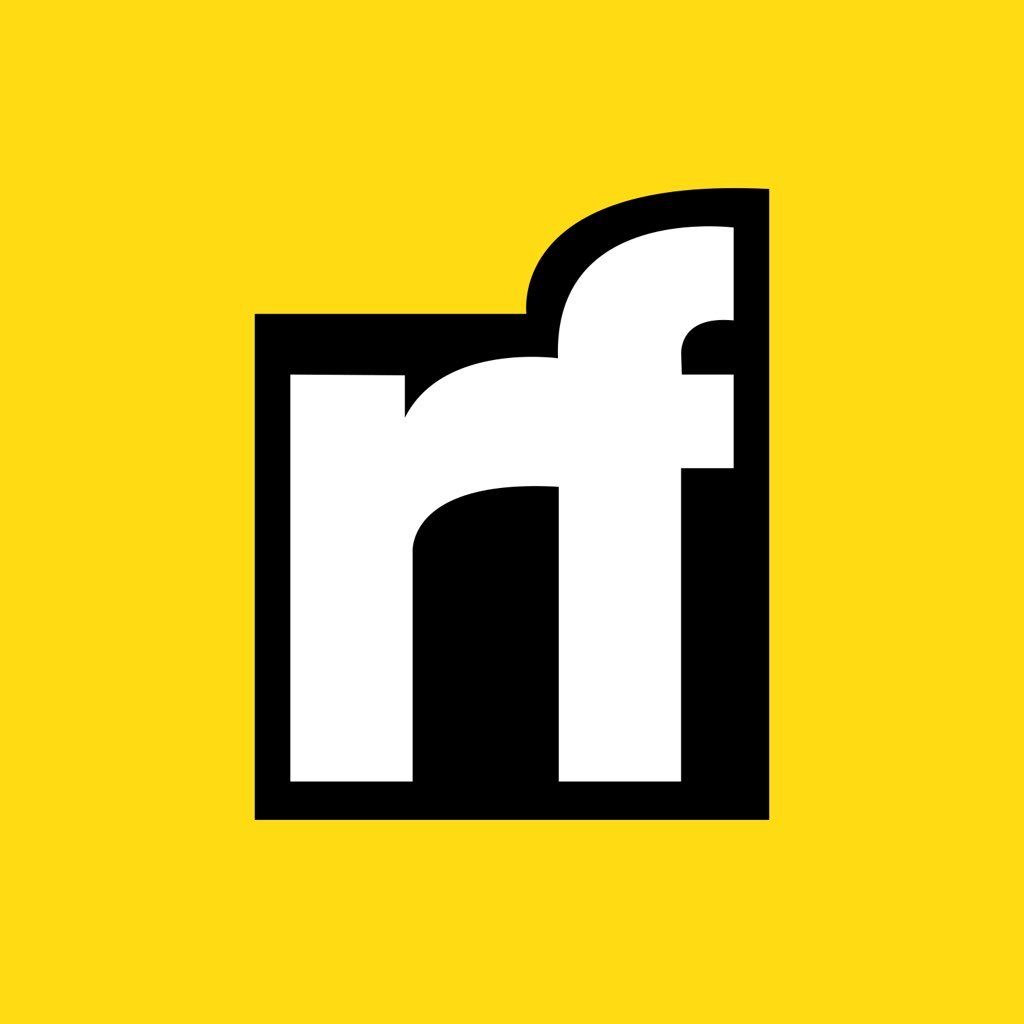
Leandro Veiga | Sciencx (2025-03-01T21:32:23+00:00) Top Lessons Learned from Debugging Complex .NET Applications: Tools, Techniques, and Best Practices. Retrieved from https://www.scien.cx/2025/03/01/top-lessons-learned-from-debugging-complex-net-applications-tools-techniques-and-best-practices/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.