This content originally appeared on DEV Community and was authored by Shahzad Ashraf
Using GroupDocs.Annotation Cloud .NET SDK simplifies the process of adding area annotations to PowerPoint presentations. Developers can emphasize content, provide feedback, and collaborate efficiently without altering the original slides. You can create document management systems or integrate annotation features into your C#, VB.NET, and ASP.NET applications; this Cloud API streamlines the entire process with minimal API calls.
The robust .NET SDK facilitates text, area, and graphical annotations, allowing for accurate document collaboration within .NET applications. It enables developers to personalize annotation styles, assign permissions, and automate annotation processes in PowerPoint presentations—eliminating the need for third-party tools. By utilizing REST API functionalities, you can guarantee a secure, scalable, and effective annotation experience across various platforms.
Begin enhancing collaboration in PowerPoint using a user-friendly Cloud REST API and Cloud SDK by following this step-by-step guide. Construct high-performing presentation review software, e-learning solutions, or business intelligence applications; GroupDocs.Annotation Cloud .NET SDK aids in optimizing workflows, enhancing team collaboration, and increasing productivity in your .NET applications.
Refer to the C# code snippet below for a practical demonstration of annotating PowerPoint presentations in .NET.
using GroupDocs.Annotation.Cloud.Sdk;
using GroupDocs.Annotation.Cloud.Sdk.Api;
using GroupDocs.Annotation.Cloud.Sdk.Client;
using GroupDocs.Annotation.Cloud.Sdk.Model;
using GroupDocs.Annotation.Cloud.Sdk.Model.Requests;
class Program
{
static void Main(string[] args)
{
// Initialize the API configuration with your credentials
string MyClientId = "your-client-id";
string MyClientSecret = "your-client-secret";
var configuration = new Configuration(MyClientId, MyClientSecret);
// Instantiate an AnnotateApi instance to apply the annotation
var annotationApi = new AnnotateApi(configuration);
// Set the input PowerPoint (PPTX) file details
var fileInfo = new GroupDocs.Annotation.Cloud.Sdk.Model.FileInfo
{
// Input file path in cloud storage
FilePath = "SampleFiles/source.pptx"
};
// Create Area annotation
var annotation = new AnnotationInfo
{
// Set annotation properties
AnnotationPosition = new Point { X = 2, Y = 2 },
Box = new Rectangle { X = 150, Y = 150, Width = 250, Height = 120 },
BackgroundColor = 50630,
PenStyle = AnnotationInfo.PenStyleEnum.Solid,
PenWidth = 4,
PenColor = 333333,
Type = AnnotationInfo.TypeEnum.Area, // Annotation type
Text = ".NET REST Annotation API", // Annotation text
PageNumber = 0, // Page index (0-based)
CreatorName = "Cloud API", // Annotation creator
CreatedOn = DateTime.Now,
};
// Set annotation options, including the output file path
var options = new AnnotateOptions
{
FileInfo = fileInfo,
Annotations = new List<AnnotationInfo> { annotation },
OutputPath = "annotation/output.pptx"
};
// Add the area annotation to the PowerPoint file
var result = annotationApi.Annotate(new AnnotateRequest(options));
}
}
This content originally appeared on DEV Community and was authored by Shahzad Ashraf
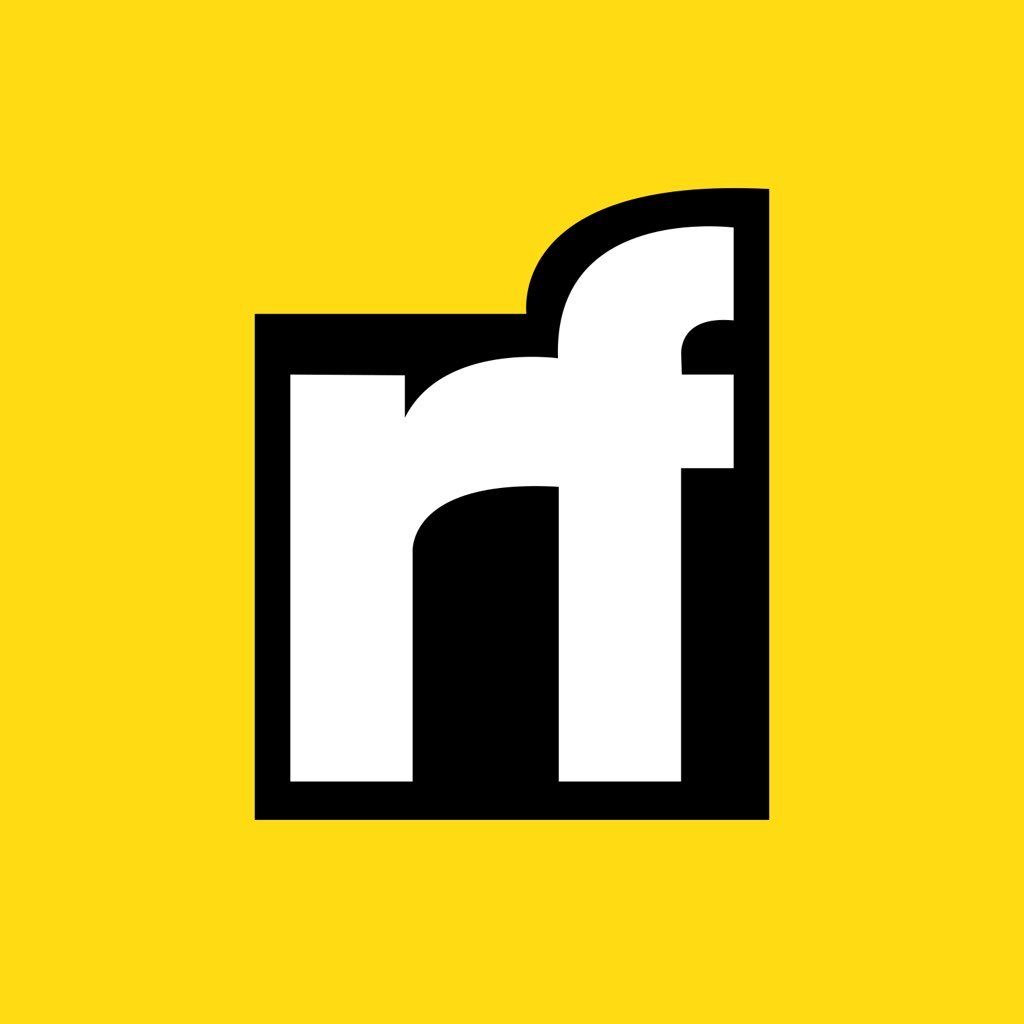
Shahzad Ashraf | Sciencx (2025-03-11T15:07:36+00:00) Seamless Area Annotations in .NET: Unlock Powerful Presentation Workflows. Retrieved from https://www.scien.cx/2025/03/11/seamless-area-annotations-in-net-unlock-powerful-presentation-workflows/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.