This content originally appeared on DEV Community and was authored by Hrishikesh Ghule
Intro
Handling SEPA (Single Euro Payments Area) XML files manually is a hassle. I recently automated this task using PowerShell, eliminating manual updates for fields like MsgId, CredDtTm, and others. This article explains the problem, the PowerShell solution, and how it made my life easier.
My Problem
I had been working in the FinTech sector for several months now, where I came across SEPA XML files and how they are used to initiate and complete transactions.
In financial processing, the SEPA XML files contain structured transaction data.
SEPA files are of two different types: SCT and SDD
🔹 SDD (SEPA Direct Debit) – Used for pull payments, where the creditor withdraws money from the debtor’s account (e.g., subscriptions, utility bills).
🔹 SCT (SEPA Credit Transfer) – Used for push payments, where the payer sends money to the recipient (e.g., salary payments, vendor payments).
đź’ˇ SDD = Auto-debits, SCT = Manual transfers. Simple.
Now each of these files contained different tags, namely:
CstmrCdtTrfInitn (Customer Credit Transfer Initiation) --> for SCT
CstmrDrctDbtInitn (Customer Direct Debit Initiation) --> for SDD
To initiate these payments everyday for testing purposes,
each file required:
âś… Updating timestamps to the current date
âś… Modifying the MsgId field dynamically
âś… Renaming the files based on updates
Was I gonna do this manually? Not happening.
The Solution: PowerShell Script
I wrote a PowerShell script that:
âś… Scans the directory for all XML files
âś… Checks if it's a credit transfer or direct debit file
âś… Updates the necessary fields dynamically
âś… Renames the files for better tracking
Here’s the full script for your reference(skip this to read the explanation below):
Set-Location -Path $PSScriptRoot
$xmlfiles = Get-ChildItem -Path $PSScriptRoot -Filter "*.xml"
Write-Output "Current directory: $PWD"
$currentMonthDate = (Get-Date).ToString("MMMdd").ToUpper()
$currentDateISO = (Get-Date -Format "yyyy-MM-ddTHH:mm:ss").ToString()
$currentDate = (Get-Date -Format "yyyy-MM-dd").ToString()
foreach($file in $xmlfiles){
[xml]$xml = Get-Content -Path $file.FullName -Raw
$firstChildNode = $xml.Document.ChildNodes[0]
# Handle Credit Transfer Files
if($firstChildNode.LocalName -eq "CstmrCdtTrfInitn"){
$xml.Document.CstmrCdtTrfInitn.GrpHdr.CredDtTm = $currentDateISO
$xml.Document.CstmrCdtTrfInitn.PmtInf.ReqdExctnDt = $currentDate
$msgid = $xml.Document.CstmrCdtTrfInitn.GrpHdr.MsgId
$updatedMsgid = $msgid -replace "(?i)([A-Z]{3}\d{2})", $currentMonthDate
$xml.Document.CstmrCdtTrfInitn.GrpHdr.MsgId = $updatedMsgid
$xml.Document.CstmrCdtTrfInitn.PmtInf.PmtInfId = $updatedMsgid
$xml.Save($file.FullName)
$oldname = $file.Name
$newFilename = "UpdatedMsgId.xml"
Rename-Item -Path $file.FullName -NewName $newFilename -Force
Write-Output "Updated File: $oldname ---> $newFilename"
}
# Handle Direct Debit Files
if($firstChildNode.LocalName -eq "CstmrDrctDbtInitn"){
$xml.Document.CstmrDrctDbtInitn.GrpHdr.CredDtTm = $currentDateISO
$xml.Document.CstmrDrctDbtInitn.PmtInf.ReqdCollDtt = $currentDate
$msgid = $xml.Document.CstmrDrctDbtInitn.GrpHdr.MsgId
$updatedMsgid = $msgid -replace "(?i)([A-Z]{3}\d{2})", $currentMonthDate
$xml.Document.CstmrDrctDbtInitn.GrpHdr.MsgId = $updatedMsgid
$xml.Document.CstmrDrctDbtInitn.PmtInf.PmtInfId = $updatedMsgid
$xml.Save($file.FullName)
$oldname = $file.Name
$newFilename = "UpdatedMsgId.xml"
Rename-Item -Path $file.FullName -NewName $newFilename -Force
Write-Output "Updated File: $oldname ---> $newFilename"
}
}
Write-Output "All the files have been updated successfully."
*I know none of you are interested in reading just a raw script, instead, I'll explain what I did here: *
So basically, it
🔹 Identifies the XML type (CstmrCdtTrfInitn or CstmrDrctDbtInitn)
🔹 Updates the timestamps and dates dynamically wherever needed
🔹 Modifies MsgId using regex to include the current date
🔹 Saves the updated file
🔹 Renames it to ensure changes are visible
Making It Easy to Run
Nobody likes to open PowerShell, navigate to the script directory, and run the script manually. It just doesn't feel right to my lazy brain. So instead, I paired the script with a batch file to allow easy execution:
@echo off
powershell -ExecutionPolicy Bypass -File "%~dp0\AutoUpdateXMLs.ps1"
pause
Here, "%~dp0" makes sure that it searches for the script in the current directory of the batch file.
Now, anyone in my team can double-click and execute the script without touching PowerShell. The only pre-requisite is that both the files (the batch and script file) should be in the same directory where the payment files are present.
Impact of This Automation
🚀 Eliminated manual effort – No more repetitive edits
⚡ Zero human errors – Updates are automated
📂 Better tracking – Files are renamed systematically
This script saves hours of work every week. If you're dealing with structured XML data regularly, automating updates using PowerShell is a game-changer.
Final Thoughts
This automation started as a small task but turned into a huge time-saver. Now, I can extend it to other XML processing tasks beyond SEPA payments. If you're dealing with repetitive file modifications, PowerShell is your best friend.
đź’¬ Have you automated XML updates before? Let me know your thoughts in the comments!
This content originally appeared on DEV Community and was authored by Hrishikesh Ghule
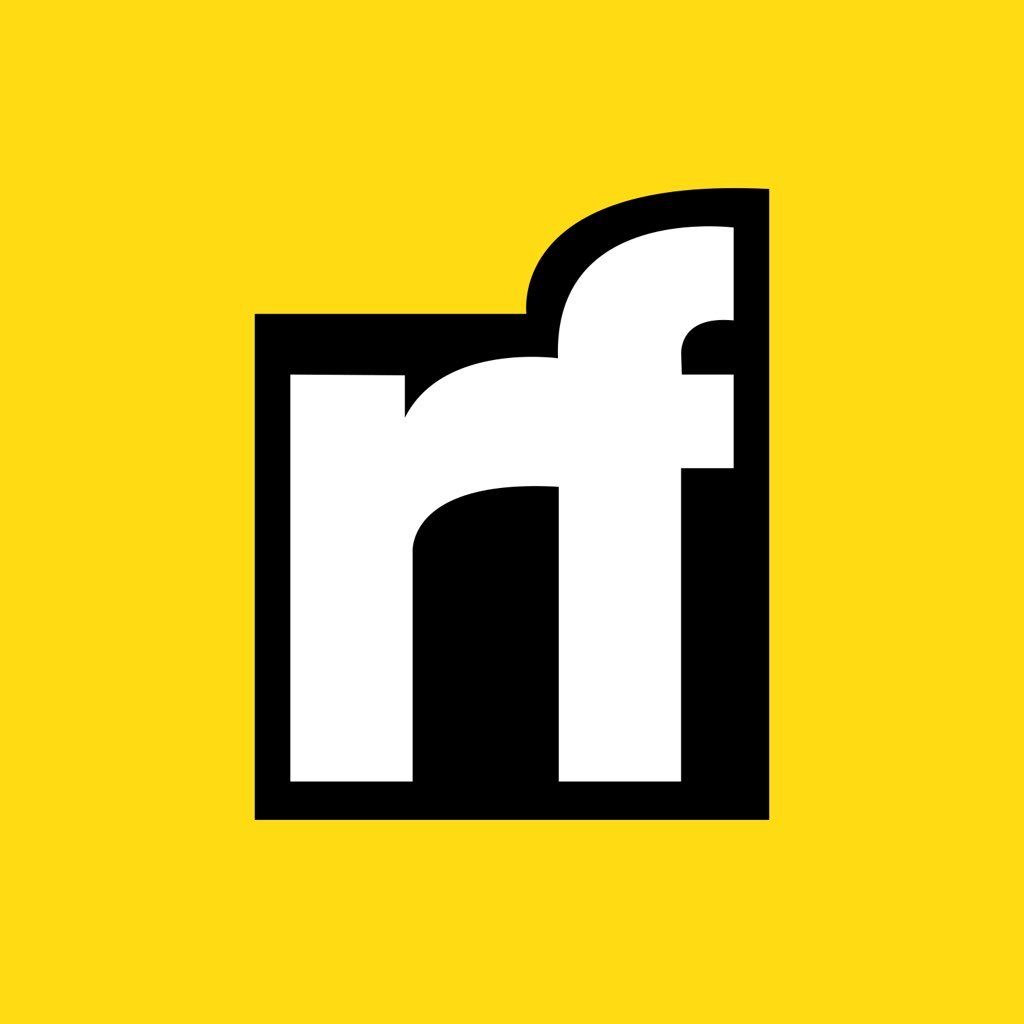
Hrishikesh Ghule | Sciencx (2025-03-18T17:06:32+00:00) Automating SEPA XML File Updates with PowerShell. Retrieved from https://www.scien.cx/2025/03/18/automating-sepa-xml-file-updates-with-powershell/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.