This content originally appeared on DEV Community and was authored by Oluwajubelo
Laravel queues allow you to handle time-consuming tasks asynchronously, meaning they run in the background without slowing down your main application. This is especially useful for tasks like sending emails, processing file uploads, or handling large database operations.
📌 Why Use Queues?
1. Improves Performance
Your application doesn’t have to wait for tasks like email sending
to complete before responding.
2. Enhances User Experience
Users get immediate responses while tasks run in the background.
3. Efficient Resource Management
Heavy tasks can be processed separately, reducing the risk of timeouts.
🛠 Setting Up Laravel Queues
By default, Laravel comes with a queue system that supports different queue drivers like:
sync
(Runs jobs immediately, no real queue)database
(Stores jobs in a database table)redis
(High-performance queue storage)sqs
(Amazon SQS)
1️⃣ Configure the Queue Driver
Open your .env
file and set the queue driver:
QUEUE_CONNECTION=database
For Redis:
QUEUE_CONNECTION=redis
2️⃣ Create a Queue Job
Run the Artisan command to generate a job:
php artisan make:job SendWelcomeEmail
This creates a job file in app/Jobs/SendWelcomeEmail.php
. Inside the handle()
method, you define what the job should do.
namespace App\Jobs;
use App\Mail\WelcomeMail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldBeUnique;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
use Mail;
class SendWelcomeEmail implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
protected $user;
public function __construct($user)
{
$this->user = $user;
}
public function handle()
{
Mail::to($this->user->email)->send(new WelcomeMail($this->user));
}
}
📝 Key Points:
ShouldQueue
tells Laravel to process this job asynchronously.handle()
contains the logic for sending the email.
3️⃣ Dispatching a Job
You can dispatch the job from anywhere in your application:
dispatch(new SendWelcomeEmail($user));
Or use the dispatch()
helper:
SendWelcomeEmail::dispatch($user);
For a delay:
SendWelcomeEmail::dispatch($user)->delay(now()->addMinutes(5));
4️⃣ Processing the Queue
Before running jobs, you must start the queue worker:
php artisan queue:work
This will continuously process new jobs in the queue.
For database queues, you need to create the table:
php artisan queue:table
php artisan migrate
If you want the worker to restart automatically after crashes, use:
php artisan queue:restart
🔧 Queue Management Tips
- Retry Failed Jobs – If a job fails, you can retry it:
php artisan queue:retry all
- Monitor Jobs – Use Horizon for Redis-based queues:
php artisan horizon
🎯 Conclusion
Laravel queues make your application faster and more responsive by handling heavy tasks in the background. Start simple with database queues, then move to Redis or SQS for better scalability.
This content originally appeared on DEV Community and was authored by Oluwajubelo
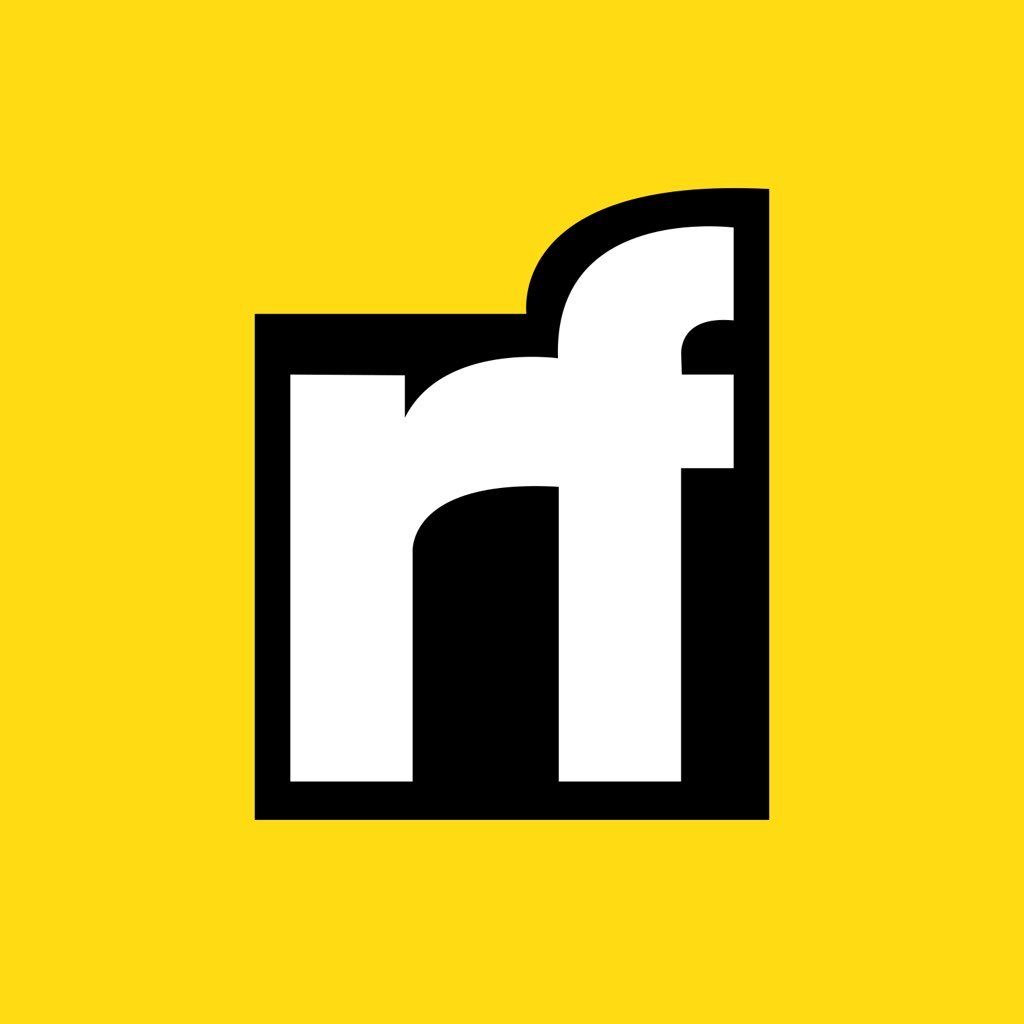
Oluwajubelo | Sciencx (2025-03-19T09:16:23+00:00) Laravel Queues for Beginners 🚀. Retrieved from https://www.scien.cx/2025/03/19/laravel-queues-for-beginners-%f0%9f%9a%80/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.