This content originally appeared on DEV Community and was authored by Nwankwo Victor Uzoma
Introduction
JavaScript is a versatile and widely-used programming language that powers the web with the concept of operators and conditionals at its core. Operators in JavaScript are symbols used for mathematical, comparison, and logical operations. Conditionals controls code flows based on specific conditions or value. In this article, we will explore operators, conditionals, and their usage with plenty of examples to help you grasp these concepts easily.
1. What are Operators in JavaScript
Operators are symbols that perform actions on values. Think of them as tools you use to do things like math, comparisons, or decision-making. In JavaScript, and programming in general, there are four major types of operators - Arithmetic operators, assignment operators, comparison operators, and logical operators.
1.1 Arithmetic Operators
These are used for basic math operations.
-
+
(Addition): Adds two numbers or combines strings.
let sum = 5 + 3; // 8
let greeting = "Hello, " + "World!"; // "Hello, World!"
-
-
(Subtraction): Subtracts the second number from the first.
let difference = 10 - 4; // 6
-
*
(Multiplication): Multiplies two numbers.
let product = 2 * 3; // 6
-
/
(Division): Divides the first number by the second.
let quotient = 10 / 2; // 5
-
%
(Modulus): Gives the remainder of a division.
let remainder = 10 % 3; // 1 (because 10 ÷ 3 = 3 with a remainder of 1)
- **(Exponentiation): Raises the first number to the power of the second.
let power = 2 ** 3; // 8 (2 * 2 * 2)
-
++
(Increment): Increases a number by 1.
let x = 5;
x++; // x is now 6
-
--
(Decrement): Decreases a number by 1.
let y = 10;
y--; // y is now 9
1.2 Assignment Operators
These are used to assign values to variables.
-
=
(Assign): Assigns a value to a variable.
let x = 5; // x is now 5
-
+=
(Add and Assign): Adds a value to the variable and assigns the result.
let x = 10;
x += 5; // x is now 15 (same as x = x + 5)
-
-=
(Subtract and Assign): Subtracts a value from the variable and assigns the result.
let x = 20;
x -= 3; // x is now 17 (same as x = x - 3)
-
*=
(Multiply and Assign): Multiplies the variable by a value and assigns the result.
let x = 5;
x *= 4; // x is now 20 (same as x = x * 4)
-
/=
(Divide and Assign): Divides the variable by a value and assigns the result.
let x = 10;
x /= 2; // x is now 5 (same as x = x / 2)
-
%=
(Modulus and Assign): Takes the modulus of the variable by a value and assigns the result.
let x = 10;
x %= 3; // x is now 1 (same as x = x % 3)
1.3 Comparison Operators
These are used to compare two values and return true
or false
.
-
==
(Equal to): Checks if two values are equal (ignores data types).
console.log(5 == "5"); // true (because "5" is converted to 5)
-
===
(Strict Equal to): Checks if two values are equal (including data types).
console.log(5 === "5"); // false (because 5 is a number and "5" is a string)
-
!=
(Not Equal to): Checks if two values are not equal (ignores data types).
console.log(5 != "5"); // false (because "5" is converted to 5)
-
!==
(Strict Not Equal to): Checks if two values are not equal (including data types).
console.log(5 !== "5"); // true (because 5 is a number and "5" is a string)
-
>
(Greater than): Checks if the first value is greater than the second.
console.log(10 > 5); // true
-
<
(Less than): Checks if the first value is less than the second.
console.log(10 < 5); // false
-
>=
(Greater than or Equal to): Checks if the first value is greater than or equal to the second.
console.log(10 >= 10); // true
-
<=
(Less than or Equal to): Checks if the first value is less than or equal to the second.
console.log(10 <= 5); // false
1.4 Logical Operators
These are used to combine or manipulate true
/false
values.
-
&&
(Logical AND): Returnstrue
if both values aretrue
.
console.log(true && false); // false
-
||
(Logical OR): Returnstrue
if at least one value istrue
.
console.log(true || false); // true
-
!
(Logical NOT): Flipstrue
tofalse
andfalse
totrue
.
console.log(!true); // false
2. Conditionals in JavaScript
Conditionals are used to make decisions in your code. They let you run different blocks of code based on whether a condition is true
or false
.
2.1 If Statement
The if
statement runs a block of code if a condition is true
.
Syntax:
if (condition) {
// Code to run if condition is true
}
Example:
let age = 20;
if (age >= 18) {
console.log("You are an adult.");
}
2.2 If-Else Statement
The if-else
statement runs one block of code if the condition is true
and another block if it’s false
.
Syntax:
if (condition) {
// Code to run if condition is true
} else {
// Code to run if condition is false
}
Example:
let age = 15;
if (age >= 18) {
console.log("You are an adult.");
} else {
console.log("You are a minor.");
}
2.3 Else-If Ladder
The else-if
ladder is used to check multiple conditions.
Syntax:
if (condition1) {
// Code to run if condition1 is true
} else if (condition2) {
// Code to run if condition2 is true
} else {
// Code to run if all conditions are false
}
Example:
let score = 85;
if (score >= 90) {
console.log("Grade: A");
} else if (score >= 80) {
console.log("Grade: B");
} else if (score >= 70) {
console.log("Grade: C");
} else {
console.log("Grade: D");
}
Wrapping Up
Operators and conditionals are fundamentals in JavaScript and are responsible for logical decision making and dynamic behavior in JavaScript programs. Understanding these concepts gives you a solid foundation to tackle even more advanced JavaScript code and build powerful applications.
Happy coding!😁
This content originally appeared on DEV Community and was authored by Nwankwo Victor Uzoma
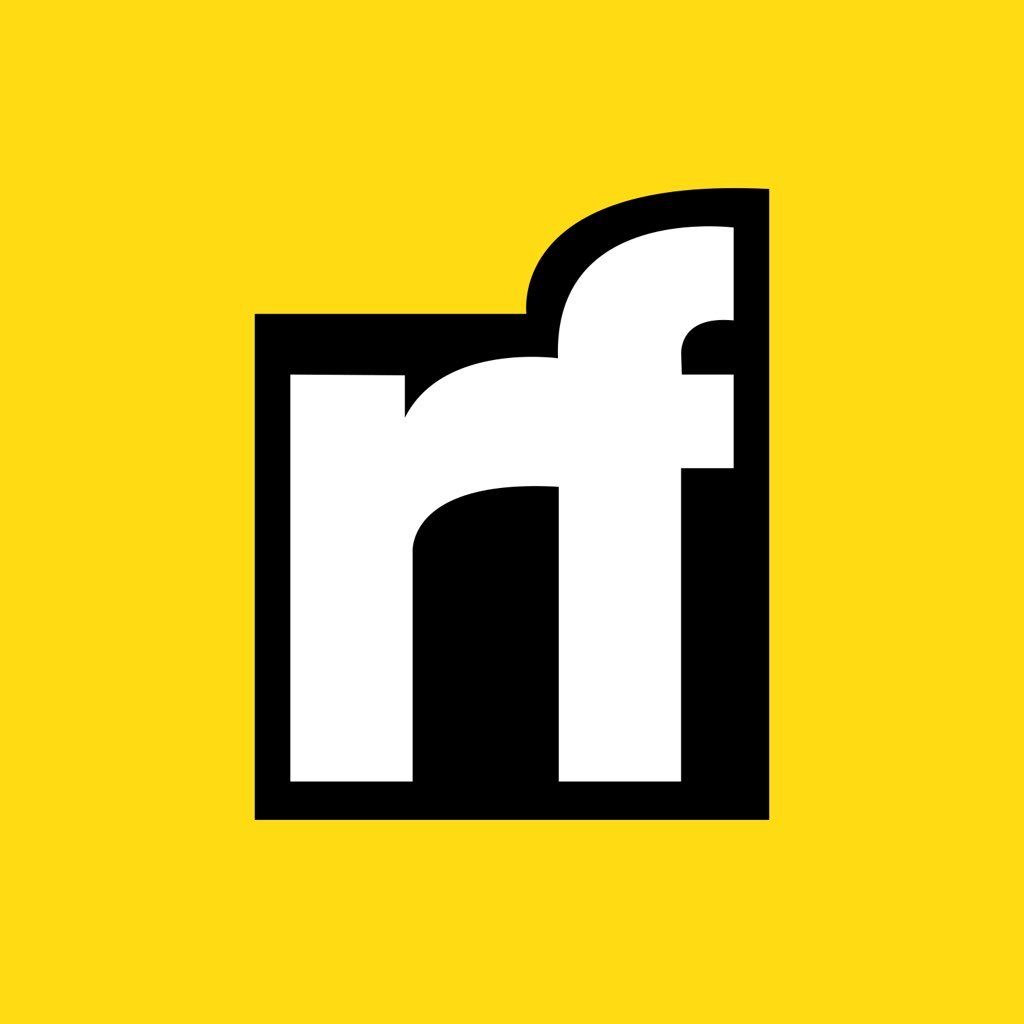
Nwankwo Victor Uzoma | Sciencx (2025-03-28T21:32:27+00:00) Understanding JavaScript with a beginner: Operators and Conditionals. Retrieved from https://www.scien.cx/2025/03/28/understanding-javascript-with-a-beginner-operators-and-conditionals/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.