This content originally appeared on DEV Community and was authored by Yeauty YE
Introduction: The Video Effects Struggle Is Real
Video effects are everywhere—think short-form video filters, live-stream beauty enhancements, cinematic color grading, or even AI-generated dynamic visuals. They’re the secret sauce that hooks viewers. But let’s be honest: building them as a developer can feel like a nightmare. Sound familiar?
- Bulk Processing Woes: Need to slap a blur on hundreds of videos? Manual tools like Premiere or After Effects laugh in your face—way too slow.
- FFmpeg Command-Line Hell: Sure, it’s powerful, but those endless cryptic flags? One typo, and you’re debugging for hours.
- C API Madness: Calling FFmpeg’s C API directly? Good luck with FFI headaches and memory leaks that haunt your dreams.
- Dynamic Effects Bottleneck: Want real-time wave distortions or cyberpunk flickers? CPU-only processing crawls, and GPU acceleration feels like a distant dream.
If you’re a Rust developer, you’ve likely hit these walls. In this post, I’ll show you how to wield Rust, FFmpeg, and OpenGL to craft video effects with elegance and speed—basic filters to hardcore dynamic visuals, all in a few minutes. Let’s dive in.
Why Rust + FFmpeg + OpenGL? The Holy Trinity of Video Magic
Rust brings safety and performance. FFmpeg is the Swiss Army knife of multimedia. OpenGL unlocks GPU power. Together, they’re a dream team for video effects—efficient, scalable, and developer-friendly. Here’s how to make them work for you.
Get Started: Video Effects in 3 Steps
Let’s say you’ve got an input.mp4
and want to add a Gaussian blur, saving it as output.mp4
. Here’s the no-nonsense breakdown:
1. Set Up FFmpeg
Not installed yet? It’s quick:
- macOS:
brew install ffmpeg
- Windows:
vcpkg install ffmpeg
# First-time vcpkg users: set the VCPKG_ROOT env variable
2. Add Rust Dependencies
In your Cargo.toml
:
[dependencies]
ez-ffmpeg = "*"
3. Write the Code
Blur that video in a handful of lines:
use ez_ffmpeg::{FfmpegContext, Output};
fn main() {
FfmpegContext::builder()
.input("input.mp4") // Input file
.filter_desc("boxblur=10:10") // Gaussian blur
.output("output.mp4") // Output file
.build().unwrap()
.start().unwrap()
.wait().unwrap();
}
Run it, and boom—output.mp4
has a slick blur. Chained API calls, no FFmpeg flag memorization required. Done.
5 Real-World Use Cases and How to Nail Them
Video effects vary wildly by project. Here are five practical scenarios with code snippets every dev can relate to:
1. Privacy Blur: Gaussian Effect
.filter_desc("boxblur=10:10")
- Problem: Live streams or videos need obscured backgrounds or sensitive areas.
- Result: Smooth, professional blur—privacy secured, visuals intact.
2. Retro Vibes: Black-and-White Filter
.filter_desc("format=gray")
- Problem: Need a nostalgic look for a video reel, but manual grading takes forever.
- Result: Instant monochrome magic, old-school feels in one line.
3. Cinematic Polish: Color Grading
.filter_desc("curves=all='0/0 0.5/0.75 1/1'")
- Problem: Post-production color tweaks are a slog, especially in bulk.
- Result: Film-like brightness and contrast, effortlessly applied.
4. Pixel Art Style: Mosaic
.filter_desc("scale=iw/10:ih/10,scale=iw*10:ih*10")
- Problem: Want a stylized look or subtle obfuscation without heavy tools.
- Result: Pixelated charm, perfect for art or privacy.
5. Sci-Fi Twist: Negative (Night Vision)
.filter_desc("negate")
- Problem: Craving a futuristic vibe but stuck on implementation.
- Result: Colors inverted, night-vision style—done in a snap.
These FFmpeg filters are lightweight, fast, and ideal for batch jobs or quick prototypes.
Level Up: Dynamic Effects with OpenGL
Static filters not cutting it? Crank things up with OpenGL and GLSL shaders for real-time, GPU-accelerated effects.
Use Case 1: Wavy Distortion
GLSL shader (fragment.glsl
):
#version 330 core
in vec2 TexCoord;
out vec4 FragColor;
uniform sampler2D screenTexture;
uniform float playTime;
void main()
{
vec2 uv = TexCoord;
uv.x += sin(playTime + uv.y * 10.0) * 0.02; // Wavy motion
vec3 texColor = texture(screenTexture, uv).rgb;
FragColor = vec4(texColor, 1.0);
}
Rust code:
use ez_ffmpeg::opengl::opengl_frame_filter::OpenGLFrameFilter;
use ez_ffmpeg::{FfmpegContext, Output, AVMediaType, filter::frame_pipeline_builder::FramePipelineBuilder};
fn main() {
let fragment_shader = include_str!("../fragment.glsl");
let frame_pipeline: FramePipelineBuilder = AVMediaType::AVMEDIA_TYPE_VIDEO.into();
let filter = OpenGLFrameFilter::new_simple(fragment_shader.to_string()).unwrap();
let frame_pipeline = frame_pipeline.filter("wave", Box::new(filter));
FfmpegContext::builder()
.input("input.mp4")
.output(Output::from("output.mp4").add_frame_pipeline(frame_pipeline))
.build().unwrap()
.start().unwrap()
.wait().unwrap();
}
- Problem: Static effects feel flat; users want motion.
- Result: A rippling wave effect—think water or dreamy sequences.
Use Case 2: Cyberpunk Flicker
GLSL shader:
#version 330 core
in vec2 TexCoord;
out vec4 color;
uniform sampler2D screenTexture;
uniform float playTime;
void main()
{
vec3 texColor = texture(screenTexture, TexCoord).rgb;
float r_shift = sin(playTime * 1.5) * 0.2 + 0.8;
float g_shift = cos(playTime * 2.0) * 0.2 + 0.8;
float b_shift = sin(playTime * 1.8) * 0.2 + 0.8;
texColor.r *= r_shift;
texColor.g *= g_shift;
texColor.b *= b_shift;
float brightness = sin(playTime * 2.5) * 0.1 + 0.95;
texColor *= brightness;
color = vec4(texColor, 1.0);
}
- Problem: Short-form content needs eye-catching flair; traditional tools are clunky.
- Result: Pulsing colors and flickers—pure cyberpunk energy.
Why This Combo Wins
- Scalability: Process hundreds of videos with code, not clicks.
- Developer Joy: Chained APIs beat command-line chaos.
- Performance: GPU via OpenGL smokes CPU-only workflows.
- Safety: Rust handles memory, sparing you C’s nightmares.
Wrap-Up: Your New Rust Superpower
Whether it’s filters for TikTok-style apps, live-stream enhancements, or AI video pipelines, Rust with FFmpeg and OpenGL delivers. Professional effects in a few lines, GPU speed boosts, and no low-level grunt work—every dev’s dream.
Curious to dig deeper? Check out this open-source gem:
🔗 https://github.com/YeautyYE/ez-ffmpeg
This content originally appeared on DEV Community and was authored by Yeauty YE
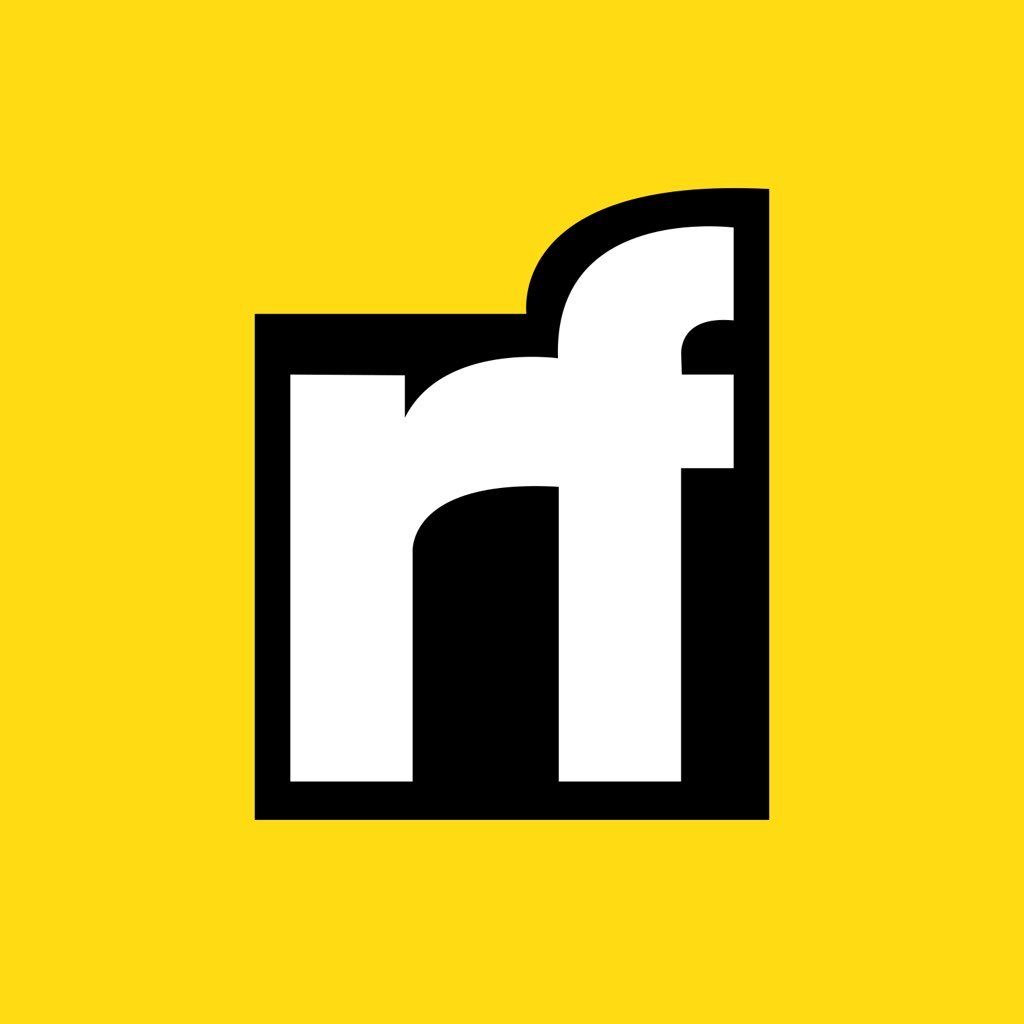
Yeauty YE | Sciencx (2025-03-31T13:51:27+00:00) Mastering Video Effects in Rust: Unleashing FFmpeg and OpenGL Like a Pro. Retrieved from https://www.scien.cx/2025/03/31/mastering-video-effects-in-rust-unleashing-ffmpeg-and-opengl-like-a-pro/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.