This content originally appeared on DEV Community and was authored by Ramu Narasinga
In this article, we will review useIsMobile hook in vercel/ai-chatbot source code.
Following is the entire code picked from this hook:
import * as React from 'react';
const MOBILE_BREAKPOINT = 768;
export function useIsMobile() {
const [isMobile, setIsMobile] = React.useState<boolean | undefined>(
undefined,
);
React.useEffect(() => {
const mql = window.matchMedia(`(max-width: ${MOBILE_BREAKPOINT - 1}px)`);
const onChange = () => {
setIsMobile(window.innerWidth < MOBILE_BREAKPOINT);
};
mql.addEventListener('change', onChange);
setIsMobile(window.innerWidth < MOBILE_BREAKPOINT);
return () => mql.removeEventListener('change', onChange);
}, []);
return !!isMobile;
}
isMobile is set to undefined initially:
const [isMobile, setIsMobile] = React.useState<boolean | undefined>(
undefined,
);
Inside the React.useEffect with empty array, this is what’s happening:
- window.watchMedia is used and is assigned to a variable, mql
const mql = window.matchMedia(`(max-width: ${MOBILE_BREAKPOINT - 1}px)`);
This is the first time I came across window.watchMedia.
window.watchMedia
The Window
interface's matchMedia()
method returns a new MediaQueryList
object that can then be used to determine if the document
matches the media query string, as well as to monitor the document to detect when it matches (or stops matching) that media query.
const onChange = () => {
setIsMobile(window.innerWidth < MOBILE_BREAKPOINT);
};
onChange function sets the isMobile state
mql.addEventListener('change', onChange);
This onChange is registered to detect the viewport changes and when viewport is mobile’s, isMobile is set to true, using the logic below:
setIsMobile(window.innerWidth < MOBILE_BREAKPOINT);
Cleanup is equally important, especially when you have registered listeners, hence the below code:
return () => mql.removeEventListener('change', onChange);
And finally, a boolean is returned from this hook.
return !!isMobile;
About me:
Hey, my name is Ramu Narasinga. I study large open-source projects and create content about their codebase architecture and best practices, sharing it through articles, videos.
I am open to work on interesting projects. Send me an email at ramu.narasinga@gmail.com
My Github — https://github.com/ramu-narasinga
My website — https://ramunarasinga.com
My Youtube channel — https://www.youtube.com/@ramu-narasinga
Learning platform — https://thinkthroo.com
Codebase Architecture — https://app.thinkthroo.com/architecture
Best practices — https://app.thinkthroo.com/best-practices
Production-grade projects — https://app.thinkthroo.com/production-grade-projects
References:
https://github.com/vercel/ai-chatbot/blob/main/hooks/use-mobile.tsx
https://developer.mozilla.org/en-US/docs/Web/API/Window/matchMedia
This content originally appeared on DEV Community and was authored by Ramu Narasinga
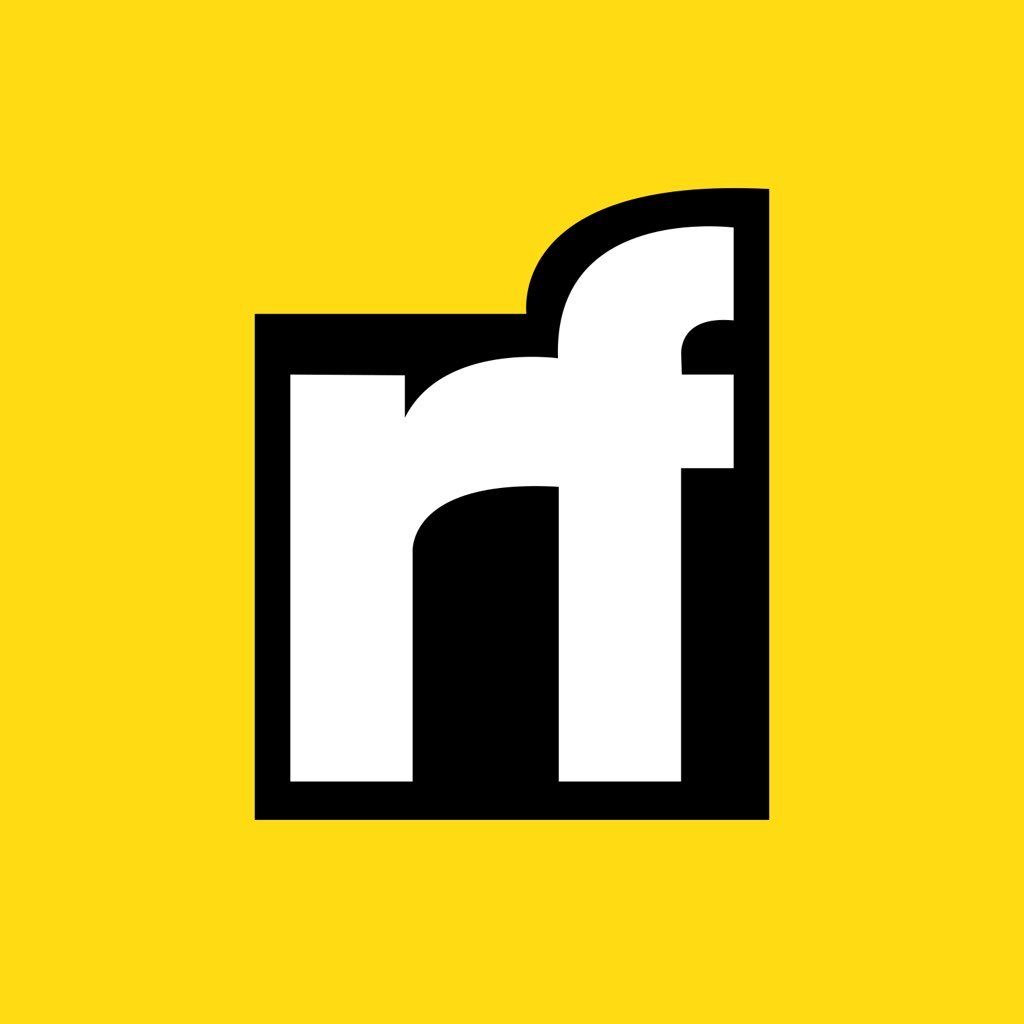
Ramu Narasinga | Sciencx (2025-04-18T03:47:54+00:00) ‘useIsMobile’ hook in vercel/ai-chatbot source code.. Retrieved from https://www.scien.cx/2025/04/18/useismobile-hook-in-vercel-ai-chatbot-source-code/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.