This content originally appeared on DEV Community and was authored by HexShift
React is excellent for building interactive UIs, but it's not meant to handle CPU-heavy tasks like parsing large files or running complex calculations directly in the main thread. This can freeze your UI and result in a poor user experience. To solve this, you can integrate Web Workers into your React app to offload heavy processing to a separate thread.
What Are Web Workers?
Web Workers run scripts in background threads. They can perform tasks without interfering with the main thread, which handles UI rendering. Perfect for processing data in React apps without freezing the interface.
1. Set Up Your React Project
If you’re using Vite or CRA, you can handle Web Workers using built-in or plugin support. Let’s use Vite as an example:
npm create vite@latest react-worker-app --template react
cd react-worker-app
npm install
2. Create a Web Worker
Create a new file called heavyWorker.js
inside src/workers
:
// src/workers/heavyWorker.js
self.onmessage = function (e) {
const input = e.data;
// Simulate heavy processing
const result = fibonacci(input);
self.postMessage(result);
};
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
3. Use the Worker in a React Component
In your React component, create the worker and communicate with it:
// src/App.jsx
import { useState } from "react";
// Vite allows direct imports of worker files with ?worker
import Worker from "./workers/heavyWorker.js?worker";
function App() {
const [number, setNumber] = useState(40);
const [result, setResult] = useState(null);
const [loading, setLoading] = useState(false);
const runWorker = () => {
setLoading(true);
const worker = new Worker();
worker.postMessage(number);
worker.onmessage = function (e) {
setResult(e.data);
setLoading(false);
worker.terminate(); // Clean up
};
};
return (
<div style={{ padding: "2rem" }}>
<h2>React + Web Worker Demo</h2>
<input
type="number"
value={number}
onChange={(e) => setNumber(Number(e.target.value))}
/>
<button onClick={runWorker} disabled={loading}>
{loading ? "Calculating..." : "Run Heavy Task"}
</button>
<div>Result: {result}</div>
</div>
);
}
export default App;
4. Why Use Web Workers with React?
- Offloads expensive computation.
- Prevents UI freezing.
- Improves responsiveness.
5. Notes and Tips
- Workers can’t access the DOM.
- Use transferable objects for large data for performance gains.
- Clean up workers with
worker.terminate()
when done.
Conclusion
Integrating Web Workers in React is a powerful way to maintain UI responsiveness during heavy computation. With tools like Vite or Webpack, it’s easy to manage worker scripts alongside your components.
If this post helped you, consider supporting my work: buymeacoffee.com/hexshift
This content originally appeared on DEV Community and was authored by HexShift
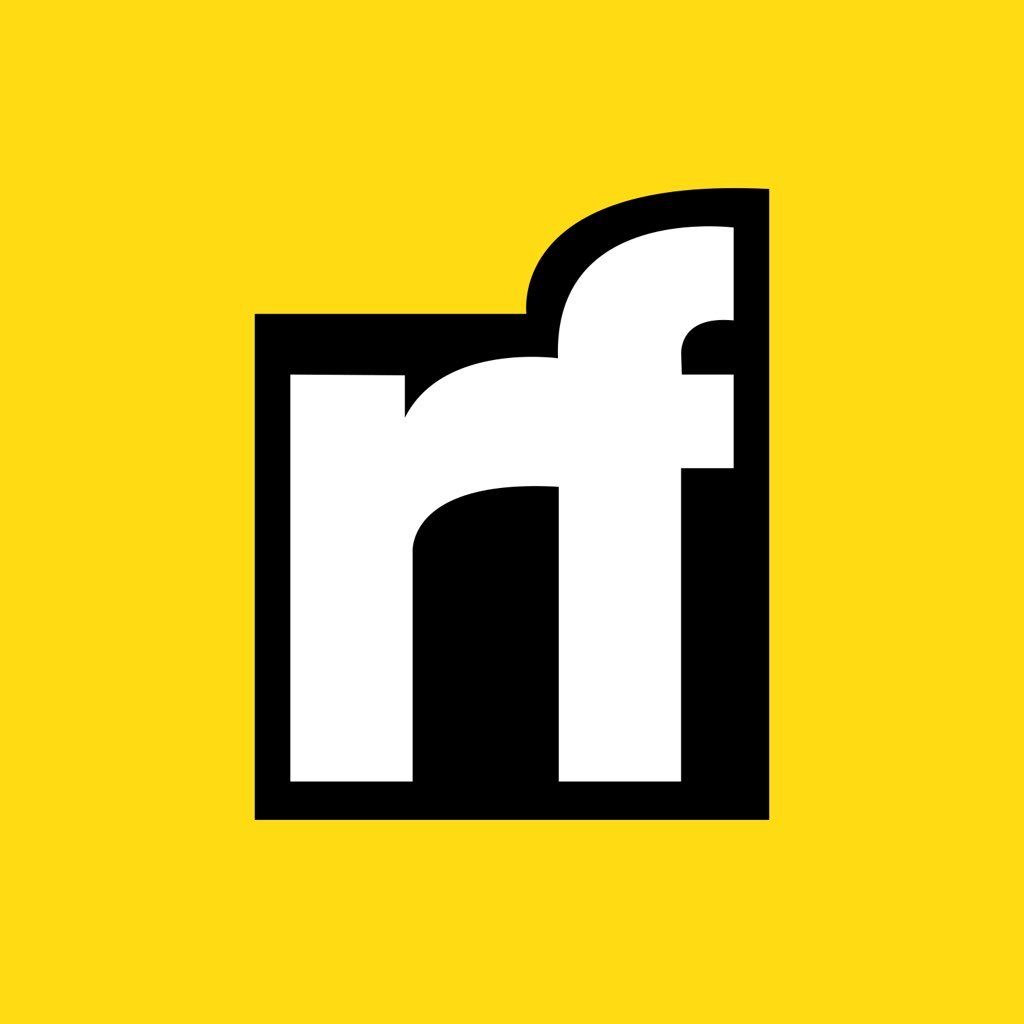
HexShift | Sciencx (2025-04-20T00:04:43+00:00) How to Use React with Web Workers for Offloading Heavy Computation. Retrieved from https://www.scien.cx/2025/04/20/how-to-use-react-with-web-workers-for-offloading-heavy-computation/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.